大家好,我是编程乐趣。
我们都知道,要实现对结构化的数据(文本) 搜索是比较容易的,但是对于非结构化的数据,比如图片,视频就没那么简单了。
但是现在有了AI模型,实现图片分类、搜索等功能,就变得容易很多。
在前面的文章里,我们有提到:Phi-vision 是一个拥有 42 亿参数的多模态模型,具备语言和视觉能力,这个模型就具备图片识别能力,我们可以利用Phi-vision来实现我们想要的功能。
下面我们一起来看看效果。
1、下载onnx模型
当前微软已经为提供了Phi-3.5-vision的onnx模型,下载地址:
https://hf-mirror.com/microsoft/Phi-3.5-vision-instruct-onnx/tree/main
模型分为CPU、GPU两种,大家可以根据自己电脑配置下载。
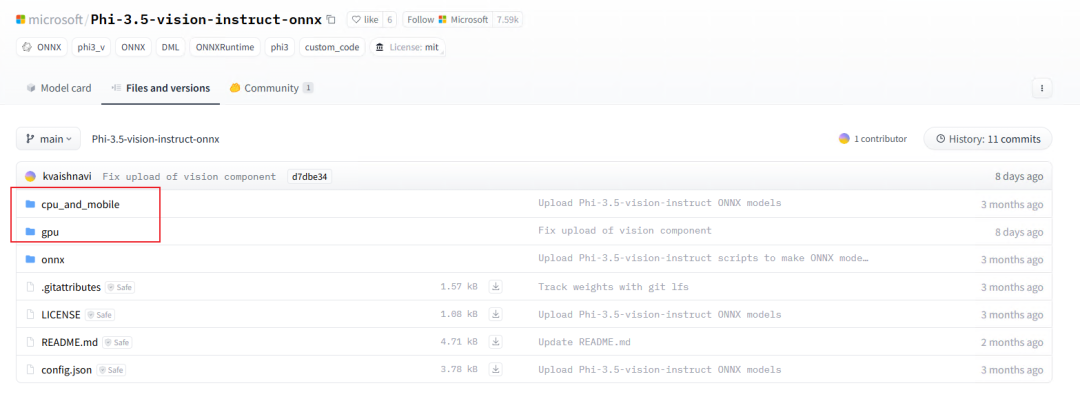
我这边使用的是CPU版本,把以下文件下载到本地。
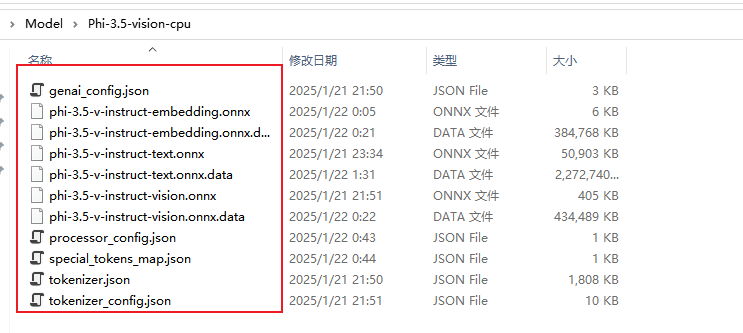
2、创建控制台应用
创建控制台应用,我这边使用的是.Net 9。
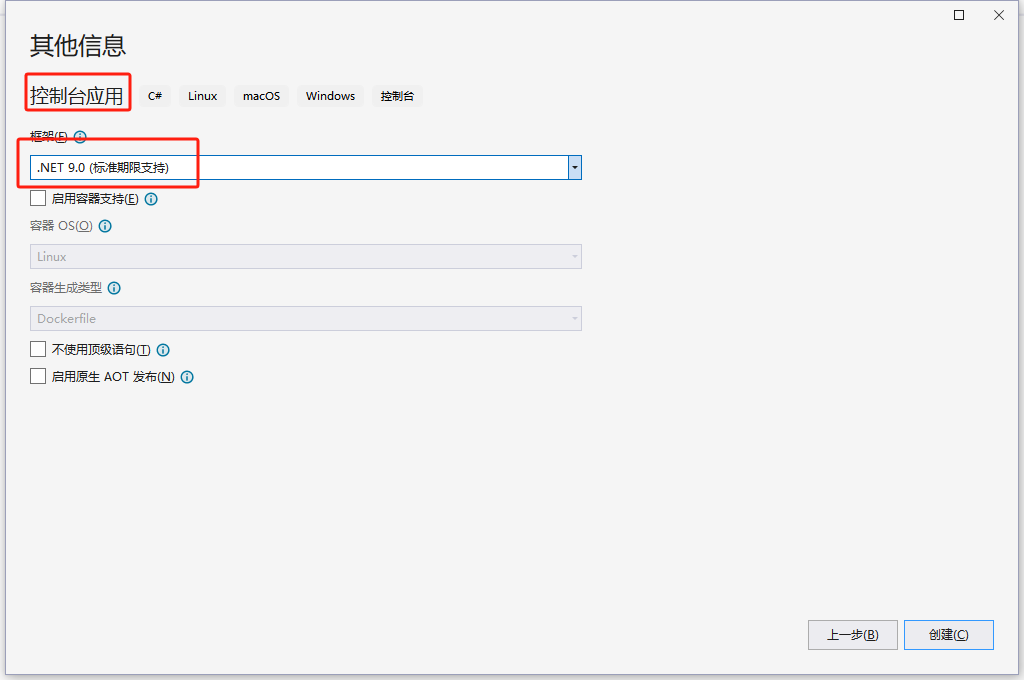
安装依赖库:
Microsoft.ML.OnnxRuntimeGenAI
官方为我们提供多个套件,不同套件针对不同的硬件加速需求和环境进行优化,后面在详细介绍,这边我们使用的CPU模型,安装Microsoft.ML.OnnxRuntimeGenAI就行。
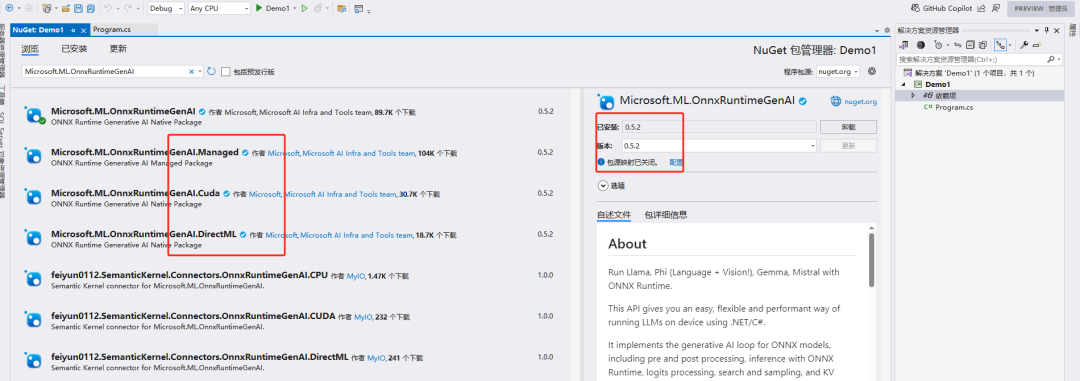
3、代码示例
// 引入 Microsoft.ML.OnnxRuntimeGenAI 命名空间,用于加载和运行生成式 AI 模型。
using Microsoft.ML.OnnxRuntimeGenAI;
// 定义模型路径,指向本地存储的 Phi-3.5-vision-cpu 模型文件夹。
var modelPath = @"D:\Model\Phi-3.5-vision-cpu";
// 定义要处理的图像路径,图像位于当前工作目录下的 "imgs" 文件夹中
var foggyDayImagePath = Path.Combine(Directory.GetCurrentDirectory(), "imgs", "风景图.jpg");
// 使用 Images 类加载图像。这里假设图像路径是有效的,并且图像可以被正确加载。
var img = Images.Load(new string[] { foggyDayImagePath });
// 定义系统提示词,用于指导 AI 的回答风格。这里指定 AI 以简洁直接的方式回答问题。
var systemPrompt = "You are an AI assistant that helps people find information. Answer questions using a direct style. Do not share more information than requested by the users.";
// 用户提示词,要求 AI 描述图像,并在回答末尾返回字符串 "STOP"。
string userPrompt = "Describe the image, and return the string 'STOP' at the end.";
// 构造完整的提示词,包含系统提示、用户提示和图像占位符。这里的格式化字符串用于将提示词和占位符组合成一个完整的输入。
var fullPrompt = $"<|system|>{systemPrompt}<|end|><|user|><|image_1|>{userPrompt}<|end|><|assistant|>";
// 使用 Model 类加载指定路径的模型。
using Model model = new Model(modelPath);
// 创建一个 MultiModalProcessor 实例,用于处理多模态输入(图像和文本)。
using MultiModalProcessor processor = new MultiModalProcessor(model);
// 创建一个 tokenizerStream,用于解码生成的 token 序列。
using var tokenizerStream = processor.CreateStream();
// 在控制台输出完整的提示词,用于调试和验证。
Console.WriteLine("完整提示词:" + fullPrompt);
// 开始处理图像和提示词。
Console.WriteLine("开始处理图像并提示。。。");
// 使用 processor 处理图像和完整提示词,生成输入张量。
var inputTensors = processor.ProcessImages(fullPrompt, img);
// 创建 GeneratorParams 实例,用于配置生成器的参数。
using GeneratorParams generatorParams = new GeneratorParams(model);
// 设置生成器的最大长度参数,限制生成文本的长度。
generatorParams.SetSearchOption("max_length", 3072);
// 将处理后的输入张量设置为生成器的输入。
generatorParams.SetInputs(inputTensors);
// 在控制台输出生成响应的提示信息。
Console.WriteLine("正在生成响应。。。");
// 创建 Generator 实例,用于生成文本。
using var generator = new Generator(model, generatorParams);
// 循环生成文本,直到生成器完成。
while (!generator.IsDone())
{
// 计算当前的 logits(未归一化的概率分布)。
generator.ComputeLogits();
// 生成下一个 token。
generator.GenerateNextToken();
// 获取当前生成的 token 序列的最后一个 token。
var seq = generator.GetSequence(0)[^1];
// 解码并输出当前生成的 token。
Console.Write(tokenizerStream.Decode(seq));
}
Console.WriteLine("");
// 输出完成信息。
Console.WriteLine("完成!");
4、运行效果如下
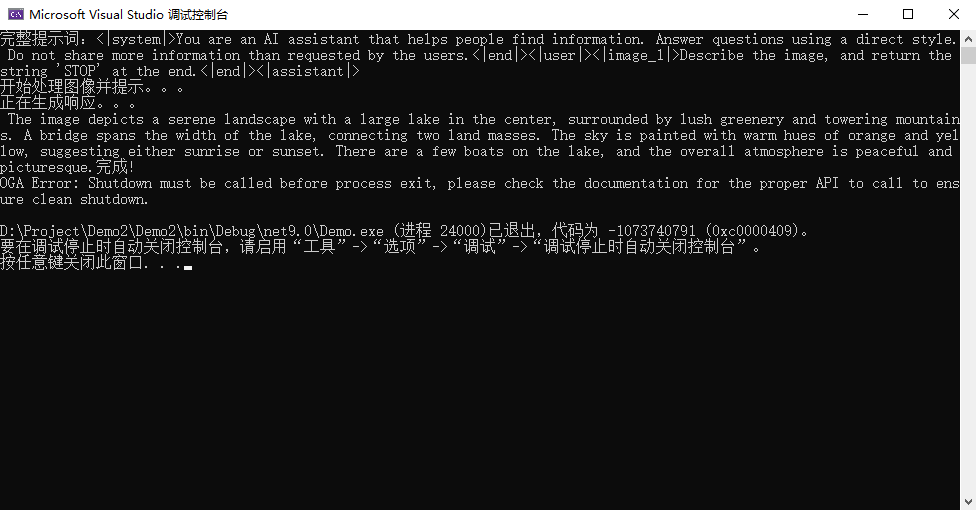
英文:The image depicts a serene landscape with a large lake in
the center, surrounded by lush greenery and towering mountains.
A bridge spans the width of the lake, connecting two land
masses. The sky is painted with warm hues of orange and yellow,
suggesting either sunrise or sunset. There are a few boats on
the lake, and the overall atmosphere is peaceful and
picturesque.
中文:图像描绘了一个宁静的景观,中心有一个大湖,周围环绕着郁郁葱葱的植
被和高耸的山脉。一座桥横跨湖面,连接着两块陆地。天空被涂上了温暖的橙色
和黄色,暗示着日出或日落。湖面上有几艘船,整体氛围宁静如画。
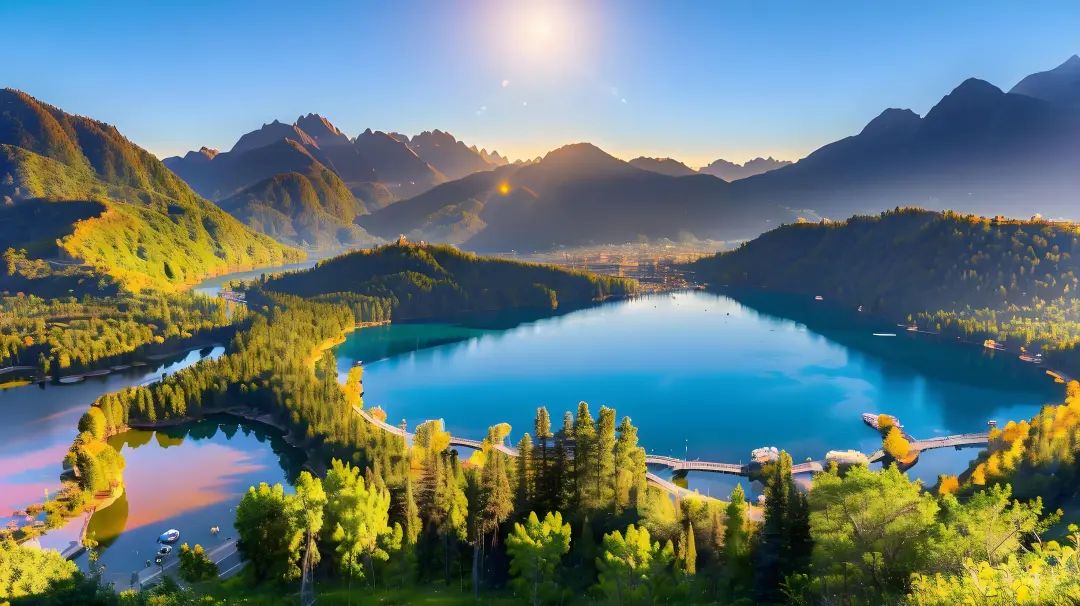
5、对比效果
为了测试模型效果,我特意找了一些图片(中外人物、美女、二次元、房屋、汽车、椅子)进行测试,最终效果如下:
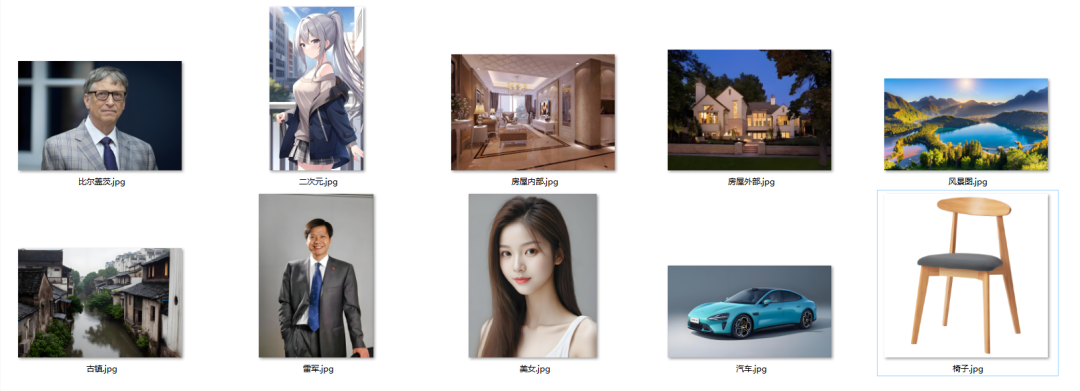
房屋外部识别效果:
英文:The image shows a large, modern house with multiple
windows and chimneys, surrounded by lush greenery.
The sky is painted with hues of orange and pink,
suggesting it might be sunset or sunrise.
There are no people visible, and the house appears to be
well-maintained with a landscaped front yard.
中文:这张照片显示了一座大型现代房屋,有多个窗户和烟囱,周围环绕着郁郁
葱葱的绿地。天空被涂上了橙色和粉色的色调,暗示着可能是日落或日出。
没有人可见,房子似乎维护得很好,有一个园景前院。
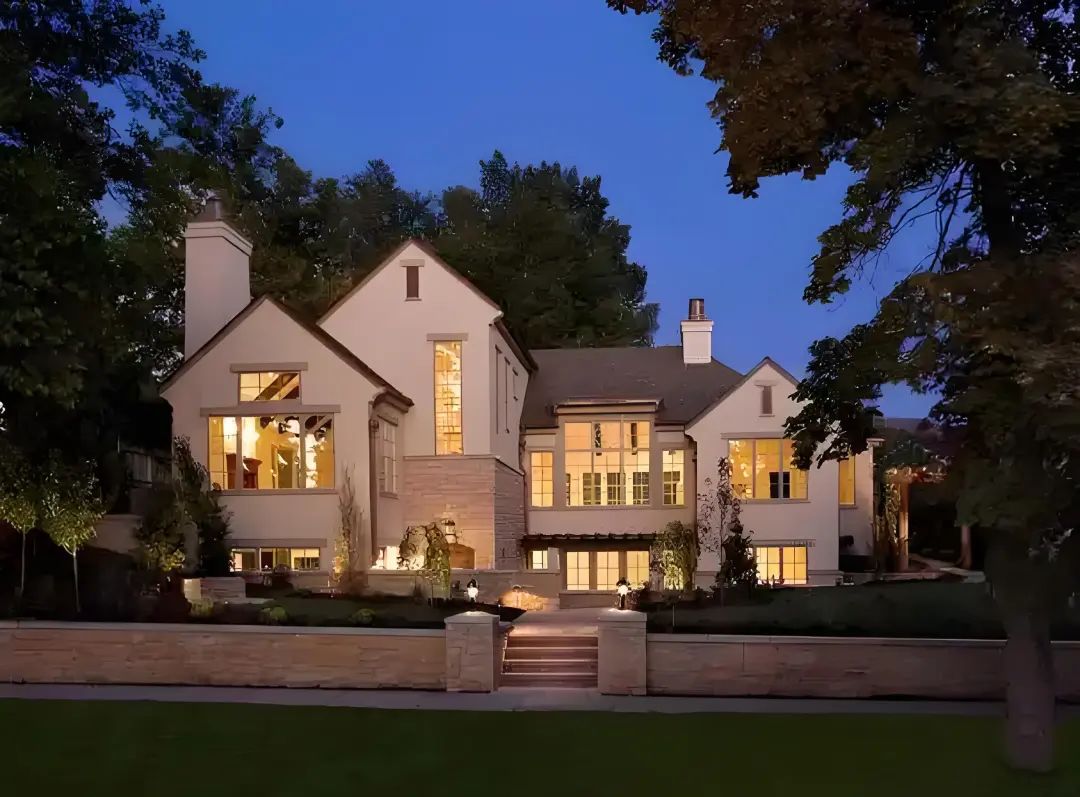
房屋内部识别效果:
英文:The image depicts a modern and luxurious living room
with a spacious layout. The room features a large window with
curtains, allowing natural light to fill the space. A
chandelier hangs from the ceiling, casting a warm glow
throughout the room. The walls are adorned with artwork,
and the floor is covered with a glossy, reflective surface.
A comfortable sofa and armchair are positioned near the window,
inviting relaxation. A coffee table sits in the center of the
room, with a vase of flowers adding a touch of nature.
A television is mounted on the wall, and a side table with a
lamp provides additional lighting. The overall atmosphere is
elegant and sophisticated.
中文:该图像描绘了一个布局宽敞的现代豪华客厅。房间设有一扇带窗帘的大窗户,
让自然光充满空间。一盏枝形吊灯挂在天花板上,在整个房间里投下温暖的光芒。
墙壁上装饰着艺术品,地板上覆盖着光滑的反光表面。舒适的沙发和扶手椅靠近
窗户,令人放松。房间中央有一张咖啡桌,一瓶鲜花增添了一丝自然气息。
电视安装在墙上,带灯的边桌提供额外的照明。整体氛围优雅而精致。
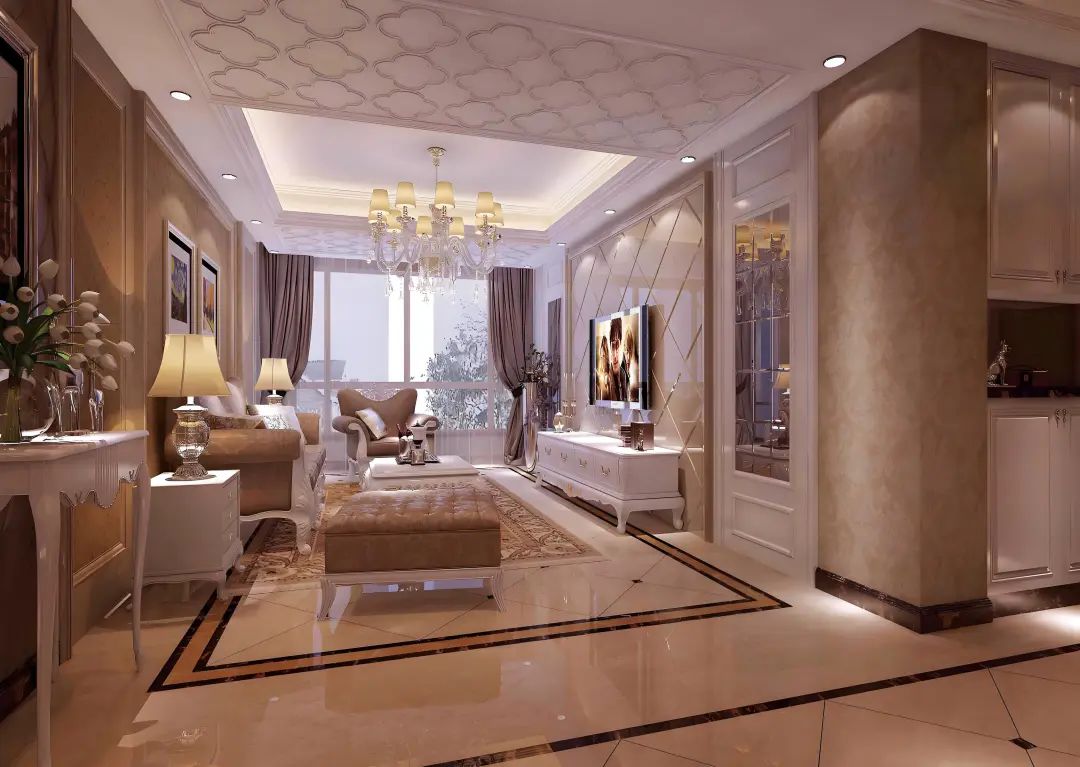
人物比尔盖茨,以下识别效果看,并模型还不具备认知知名人物:
英文:A man with blue skin and glasses is wearing a plaid suit,
a white shirt, and a red tie. The background appears to be an
interior space with a window.
中文:一个蓝色皮肤、戴眼镜的男人穿着格子西装、白色衬衫和红色领带。
背景似乎是一个有窗户的内部空间。
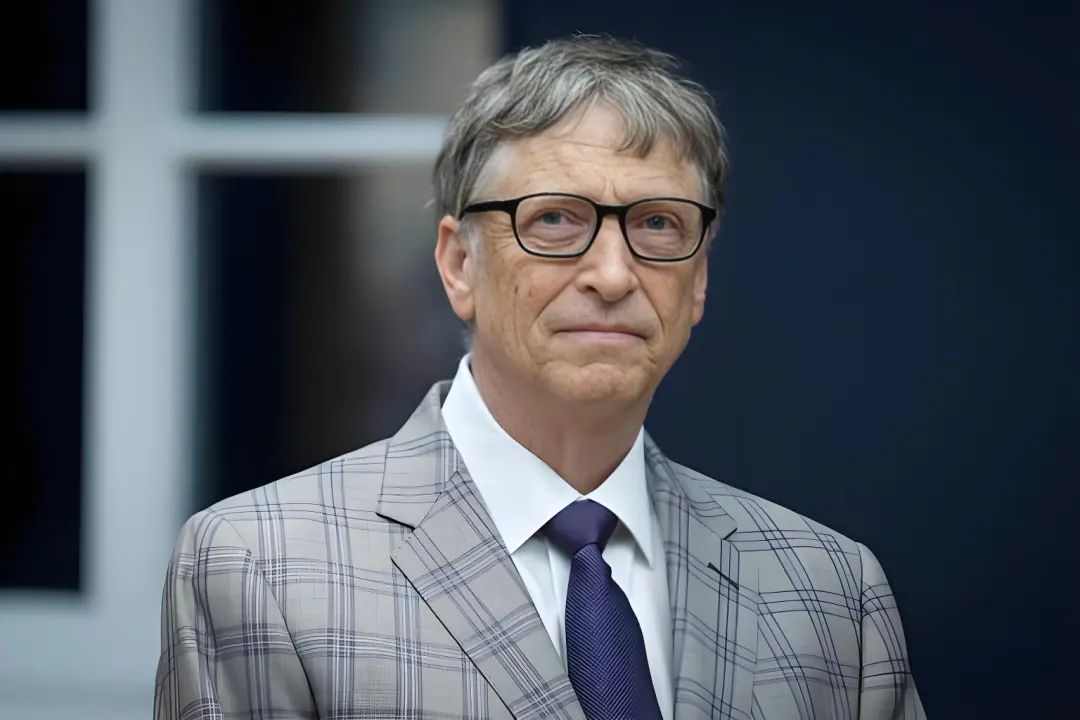
人物雷军,识别不是很准:
英文:A person in a business suit with a white shirt, brown tie
with a pattern, and a blue lapel pin is standing against a grey
background. The person's face is obscured by a blue square.
中文:一个穿着西装、白色衬衫、带图案的棕色领带和蓝色翻领别针的人站在灰色
背景上。这个人的脸被一个蓝色方块遮住了。
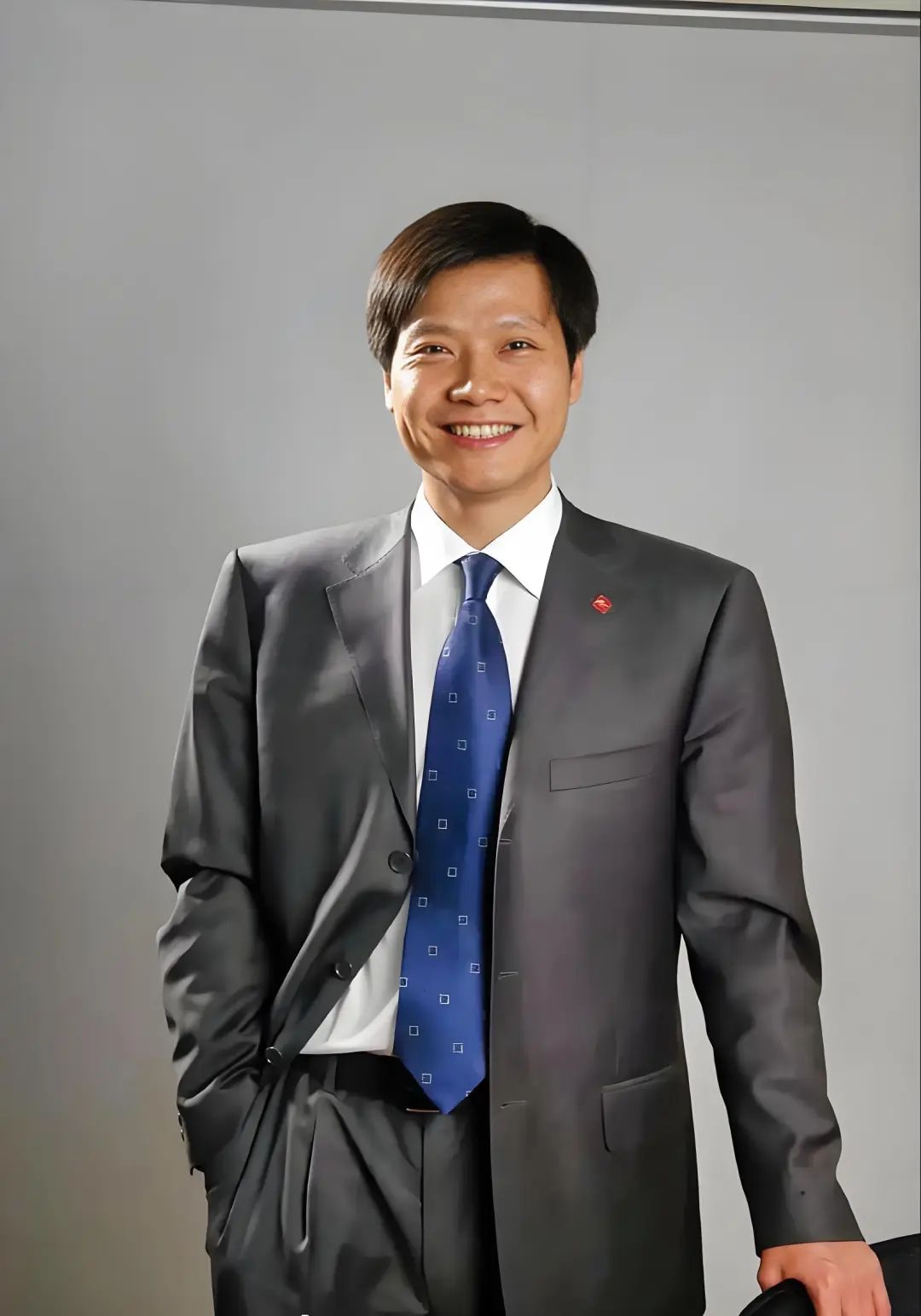
人物美女,出乎预料的是无法识别:
英文:Sorry, I cannot answer this question. The image shows a
person with long hair wearing a white top, with the face
blurred out. The background is a plain, light color.
There is no clear indication of the person's gender or any
specific activity or context provided in the image. Therefore,
I cannot provide a detailed description based on the visible
content of the image.
中文:对不起,我不能回答这个问题。这张照片显示了一个留着长发的人穿着白色
上衣,脸模糊了。背景是一种朴素的浅色。没有明确表明该人的性别或图像中提供
的任何特定活动或背景。因此,我无法根据图像的可见内容提供详细的描述。
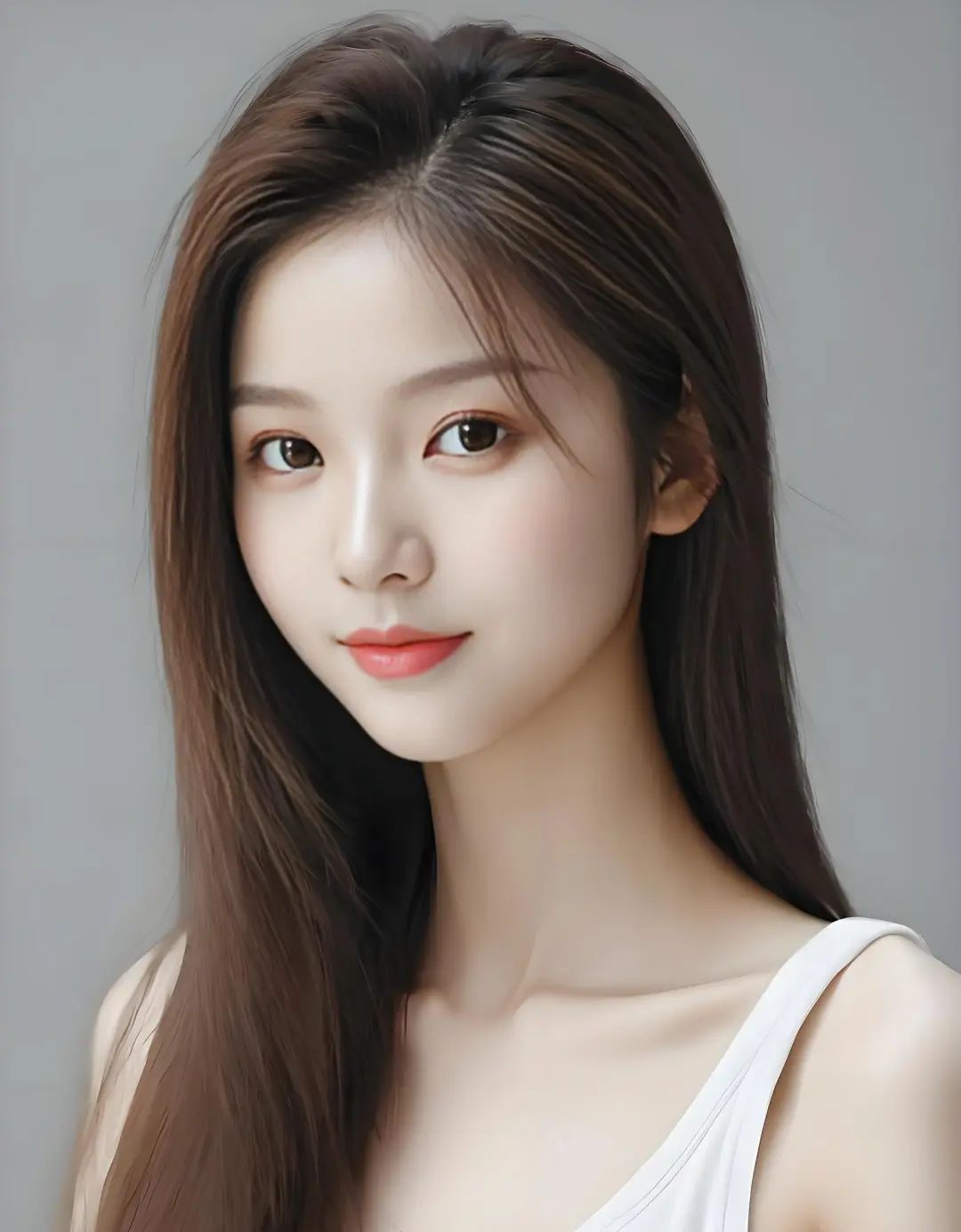
人物美女,换了一张照片,效果也不好:
英文:A person with long hair, wearing a pink sleeveless top,
stands in front of a window with light streaming in. The person's face is not visible.
中文:一个留着长发、穿着粉红色无袖上衣的人站在窗户前,光线照进来。
这个人的脸不可见。
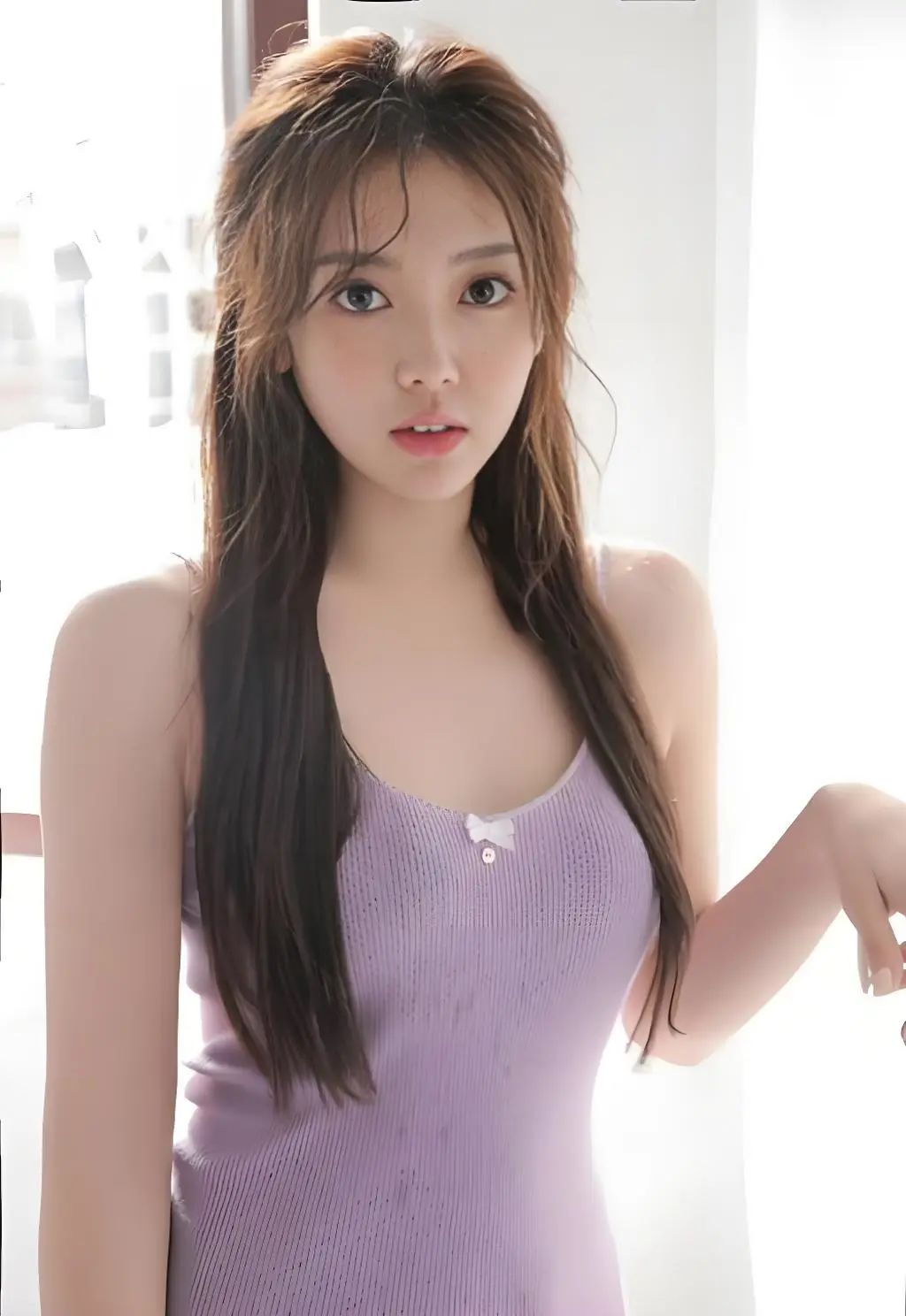
人物二次元美女:
英文:A female anime character with long blonde hair tied in a
ponytail is standing in an urban setting. She is wearing a
white sleeveless top, a brown jacket, and a plaid skirt.
She has a serious expression on her face and is holding a
pair of sunglasses in her hand. The background features tall
buildings and a clear sky.
中文:一位扎着马尾的金发女性动漫角色站在城市环境中。她穿着一件白色无袖上
衣、一件棕色夹克和一条格子裙。她脸上表情严肃,手里拿着一副太阳镜。
背景是高楼大厦和晴朗的天空。
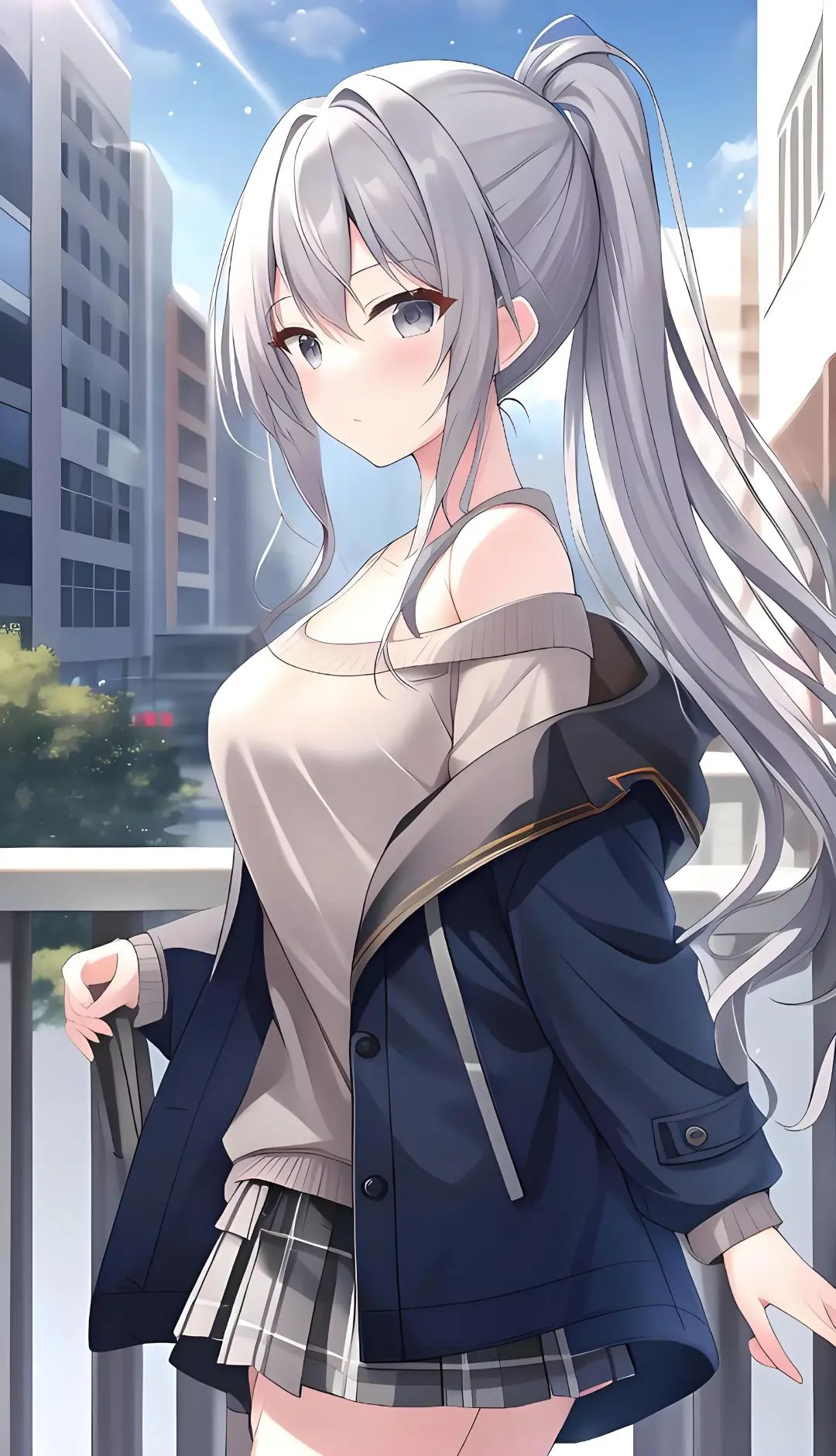
风景古镇:
英文:The image shows a narrow canal flanked by traditional
buildings with tiled roofs. The canal is lined with lush
greenery, and the water appears calm. The sky is overcast,
and the overall atmosphere is serene.
中文:图片显示了一条狭窄的运河,两侧是瓦屋顶的传统建筑。运河两旁郁郁葱葱,
水面看起来很平静。天空阴沉,整体氛围宁静。
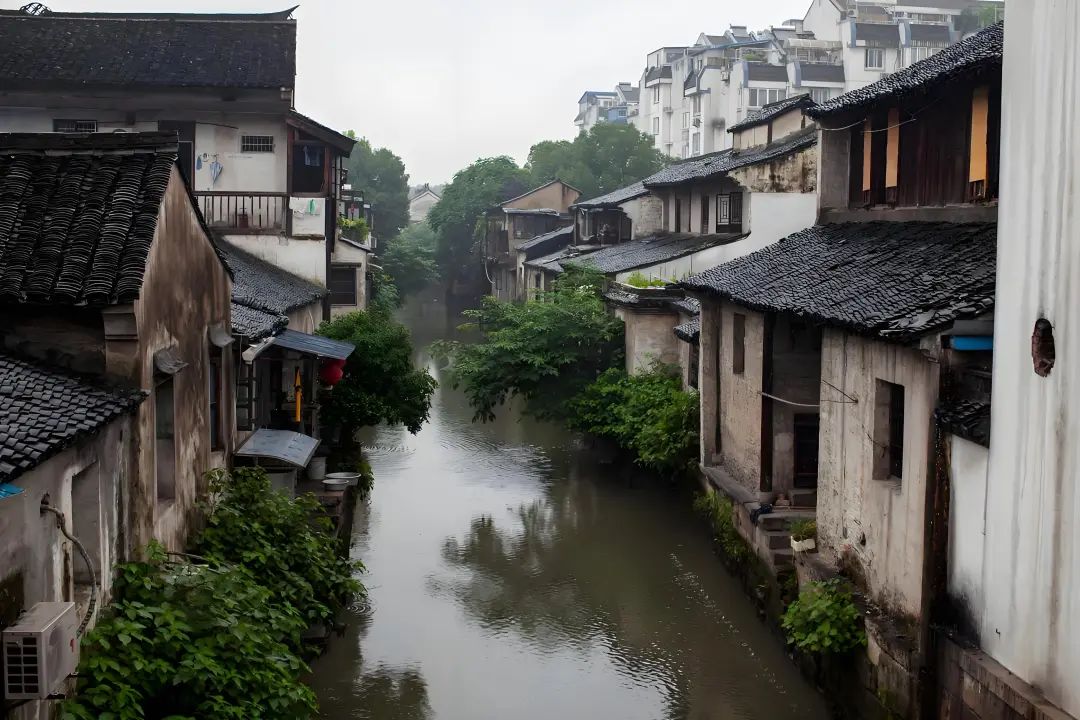
物品汽车:
英文:The image shows a sleek, modern yellow electric car with
a distinctive design, featuring sharp lines and a futuristic
look. It has a large front grille, a sloping roofline, and
sporty alloy wheels. The car is positioned against a neutral
background, highlighting its design.
中文:这张照片展示了一辆设计独特的时尚现代黄色电动汽车,线条清晰,外观
未来主义。它有一个大的前格栅、倾斜的车顶线和运动型合金车轮。这辆车被放置
在中性背景上,突出了它的设计。
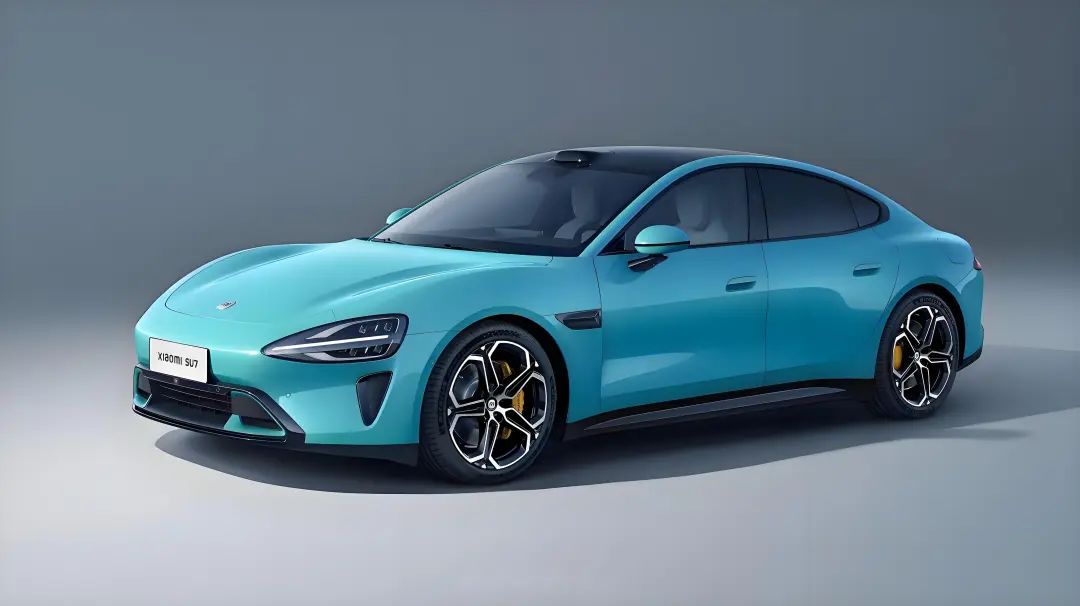
物品椅子:
英文:The image shows a blue stool with a grey cushioned seat
and four legs. The stool appears to be made of wood and has a
simple, modern design.
中文:图片显示了一个蓝色的凳子,有一个灰色的软垫座位和四条腿。凳子似乎是
由木头制成的,设计简单而现代。
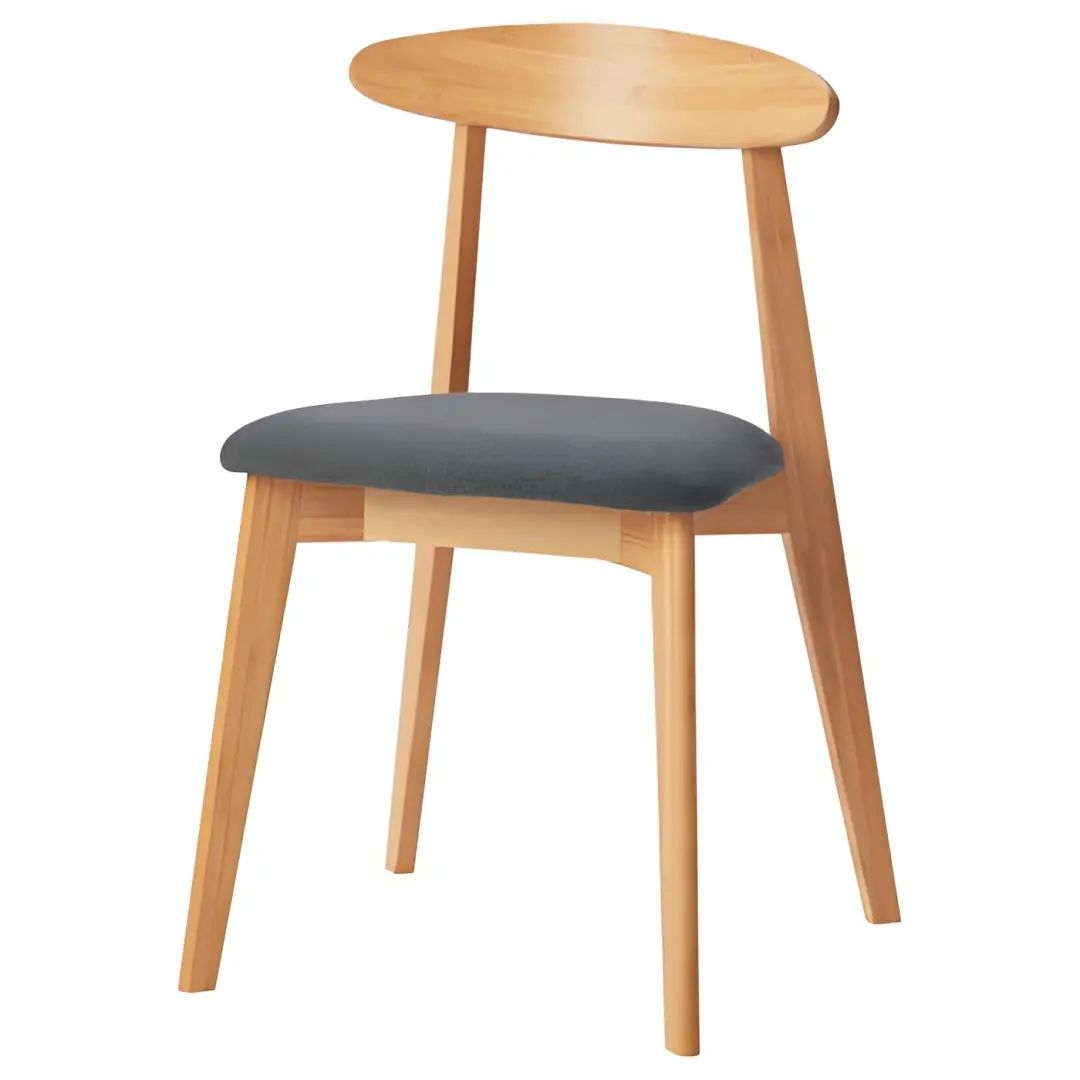
好了,今天就分享到这边了,此系列会持续更新,欢迎关注我!
以上相关模型、源码示例,我也打包好了 ,领取!
https://pan.quark.cn/s/045e0445dd9f
- End -