1.拖拽排序 使用 sortablejs库
npm install sortablejs --save-dev
<template>
<view id="list">
<view v-for="(item, index) in list" :key="item.id" class="item">
{{ item.name }}
</view>
</view>
</template>
<script>
import Sortable from "sortablejs";
export default {
data() {
return {
list: [{
id: 1,
name: "列表项1"
},
{
id: 2,
name: "列表项2"
},
{
id: 3,
name: "列表项3"
},
{
id: 5,
name: "列表项5"
},{
id: 6,
name: "列表项6"
},{
id: 7,
name: "列表项7"
},{
id: 8,
name: "列表项8"
},{
id: 9,
name: "列表项9"
},{
id: 10,
name: "列表项10"
},{
id: 11,
name: "列表项11"
},{
id: 12,
name: "列表项12"
},{
id: 13,
name: "列表项13"
},{
id: 14,
name: "列表项14"
}
]
};
},
mounted() {
this.initSortable();
},
methods: {
initSortable() {
const list = document.getElementById("list");
new Sortable(list, {
animation: 150,
onUpdate: this.handleUpdate
});
},
handleUpdate(event) {
const {
oldIndex,
newIndex
} = event;
const item = this.list.splice(oldIndex, 1)[0];
this.list.splice(newIndex, 0, item);
}
}
};
</script>
<style scoped>
.container {
display: flex;
flex-direction: column;
}
.item {
padding: 10px;
margin: 5px 0;
background-color: #f0f0f0;
border: 1px solid #ccc;
cursor: pointer;
}
</style>
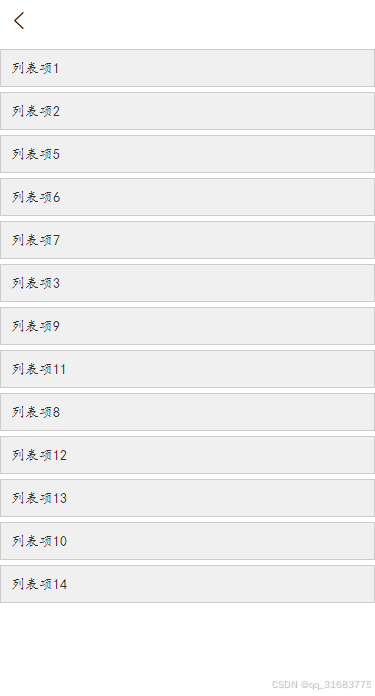
2.拖拽到指定位置并停留
<template>
<view class="container">
<movable-area class="area">
<movable-view
v-for="(item, index) in list"
:key="item.id"
:x="0"
:y="item.y"
direction="vertical"
@change="handleMove(index, $event)"
class="item"
>
{{ item.name }}
</movable-view>
</movable-area>
</view>
</template>
<script>
export default {
data() {
return {
list: [
{ id: 1, name: "列表项1", y: 0 },
{ id: 2, name: "列表项2", y: 50 },
{ id: 3, name: "列表项3", y: 100 },
{ id: 4, name: "列表项4", y: 150 }
]
};
},
methods: {
handleMove(index, event) {
const { y } = event.detail;
this.list[index].y = y;
this.sortList();
},
sortList() {
this.list.sort((a, b) => a.y - b.y);
}
}
};
</script>
<style scoped>
.container {
height: 300px;
}
.area {
width: 100%;
height: 100%;
}
.item {
width: 100%;
height: 50px;
background-color: #f0f0f0;
border: 1px solid #ccc;
text-align: center;
line-height: 50px;
}
</style>
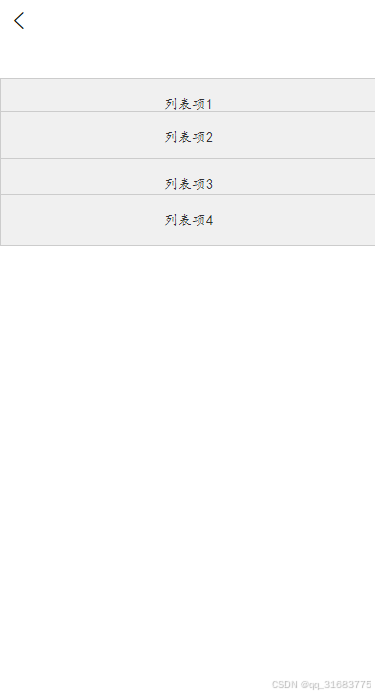