B2093 查找特定的值 - 洛谷
- 题⽬要求下标是从0开始的,和数组的下标是吻合的,存放数据应该从下标0开始
- n的取值范围是1~10000
- 数组中存放的值的绝对值不超10000,说明int类型就⾜够了
- 找到了输出下标,找不到要输出-1,这⼀点要注意,很容易忽略
c++
#include <bits/stdc++.h>
using namespace std;
int main()
{
ios::sync_with_stdio(false);
cin.tie(nullptr);
int n;
cin >> n;
vector<int> arr(n);
for(auto &x : arr)
{
cin >> x;
}
int a;
cin >> a;
int i = 0;
int flg = true;
for(auto x : arr)
{
if (a == x)
{
cout << i << '\n';
flg = false;
break;
}
i++;
}
if (flg)
{
cout << -1 << '\n';
}
return 0;
}
c++
#include <iostream>
using namespace std;
const int N = 10010;
int arr[N];
int main()
{
int n = 0;
cin >> n;
for (int i = 0; i < n; i++)
{
cin >> arr[i];
}
int k = 0;
cin >> k;
int i = 0;
for (i = 0; i < n; i++)
{
if (k == arr[i])
{
cout << i << endl;
break;
}
}
if (i == n)
cout << -1 << endl;
return 0;
}
- 有的题⽬要求数据从下标0的位置开始存放,也有些题⽬要求数据是从下标1的位置开始存放,要仔细阅读题⽬。让从下标1开始存放的时候,数组的开辟必须要有多余的空间使⽤,如果开辟的刚刚好就会越界。
- 数组空间的开辟要⾜够,以免数据越界,所以经常题⽬需要存放n个数据,就开辟n+10个空间,这样空间就⾮常充⾜,⽐较保险。其实在空间⾜够的情况下,浪费⼀点空间是不影响的。动态规划相关算法,⼀般都会预留好空间。
- ⼀般数组较⼤的时候,建议将数组创建成全局数组,因为局部的数组太⼤的时候,可能会导致程序⽆法运⾏,刷题多了就⻅怪不怪了。全局变量(数组)是在内存的静态区开辟空间,但是局部的变量(数组)是在内存的栈区开辟空间的,每个程序的栈区空间是有限的,不会很⼤。
B2089 数组逆序重存放 - 洛谷
c++
#include <bits/stdc++.h>
using namespace std;
int main()
{
ios::sync_with_stdio(false);
cin.tie(nullptr);
int n;
cin >> n;
vector<int> a(n);
for (auto &x : a)
{
cin >> x;
}
int left = 0, right = n - 1;
while (left <= right)
{
int tmp = a[left];
a[left] = a[right];
a[right] = tmp;
left++;
right--;
}
for (auto x : a)
{
cout << x << ' ';
}
return 0;
}
c++
#include <iostream>
using namespace std;
int arr[110] = { 0 };
int main()
{
int n = 0;
cin >> n;
int i = 0;
for (i = 0; i < n; i++)
cin >> arr[i];
//逆序
int left = 0;
int right = n - 1;
while (left < right)
{
int tmp = arr[left];
arr[left] = arr[right];
arr[right] = tmp;
left++;
right--;
}
for (i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
c++
#include <iostream>
using namespace std;
int arr[110] = { 0 };
int main()
{
int n = 0;
cin >> n;
int i = 0;
for (i = 0; i < n; i++)
cin >> arr[i];
//逆序输出
for (i = n - 1; i >= 0; i--)
cout << arr[i] << " ";
return 0;
}
B2091 向量点积计算 - 洛谷
c++
#include <bits/stdc++.h>
using namespace std;
int main()
{
ios::sync_with_stdio(false);
cin.tie(nullptr);
int n;
cin >> n;
vector<int> a(n);
for (auto &x : a)
{
cin >> x;
}
vector<int> b(n);
for (auto &x : b)
{
cin >> x;
}
int ans = 0;
for (int i = 0; i < n; i++)
{
ans += a[i] * b[i];
}
cout << ans << '\n';
return 0;
}
c++
#include <iostream>
using namespace std;
const int N = 1010;
int arr1[N];
int arr2[N];
int main()
{
int n = 0;
cin >> n;
for (int i = 0; i < n; i++)
{
cin >> arr1[i];
}
for (int i = 0; i < n; i++)
{
cin >> arr2[i];
}
int ret = 0;
for (int i = 0; i < n; i++)
{
ret += arr1[i] * arr2[i];
}
cout << ret << endl;
return 0;
}
c++
#include <iostream>
using namespace std;
const int N = 1010;
int arr1[N];
int main()
{
int n = 0;
int m = 0;
cin >> n;
for (int i = 0; i < n; i++)
{
cin >> arr1[i];
}
int ret = 0;
for (int i = 0; i < n; i++)
{
cin >> m;
ret += arr1[i] * m;
}
cout << ret << endl;
return 0;
}
B2090 年龄与疾病 - 洛谷
c++
#include <bits/stdc++.h>
using namespace std;
int main()
{
int n;
cin >> n;
vector<int> p(n);
for (auto &x : p)
{
cin >> x;
}
//0-18 、 19-35 、 36-60、 61
int c = 0, t = 0, a = 0, o = 0;
for (auto x : p)
{
if (x >= 0 && x <= 18)
c++;
if (x > 18 && x <= 35)
t++;
if (x > 35 && x <= 60)
a++;
if (x > 60)
o++;
}
printf("%.2f%%\n", c * 100.0 / n);
printf("%.2f%%\n", t * 100.0 / n);
printf("%.2f%%\n", a * 100.0 / n);
printf("%.2f%%\n", o * 100.0 / n);
return 0;
}
这组数据不存储下来也是可以的,因为只有⼀组数据,所以⼀边读取,⼀边统计也是可以的,这样省略了数组空间的开销
c++
#include <iostream>
using namespace std;
int n;
int num;
int p1, p2, p3, p4;
int main()
{
cin >> n;
int i = 0;
//输⼊⼀个处理⼀个
for (i = 0; i < n; i++)
{
cin >> num;
if (num >= 0 && num <= 18)
p1++;
else if (num >= 19 && num <= 35)
p2++;
else if (num >= 36 && num <= 60)
p3++;
else
p4++;
}
printf("%.2f%%\n", p1 * 1.0 / n * 100);
printf("%.2f%%\n", p2 * 1.0 / n * 100);
printf("%.2f%%\n", p3 * 1.0 / n * 100);
printf("%.2f%%\n", p4 * 1.0 / n * 100);
return 0;
}
题⽬要求输出的是百分⽐,是带%的,这个要特殊处理⼀下
B2092 开关灯 - 洛谷
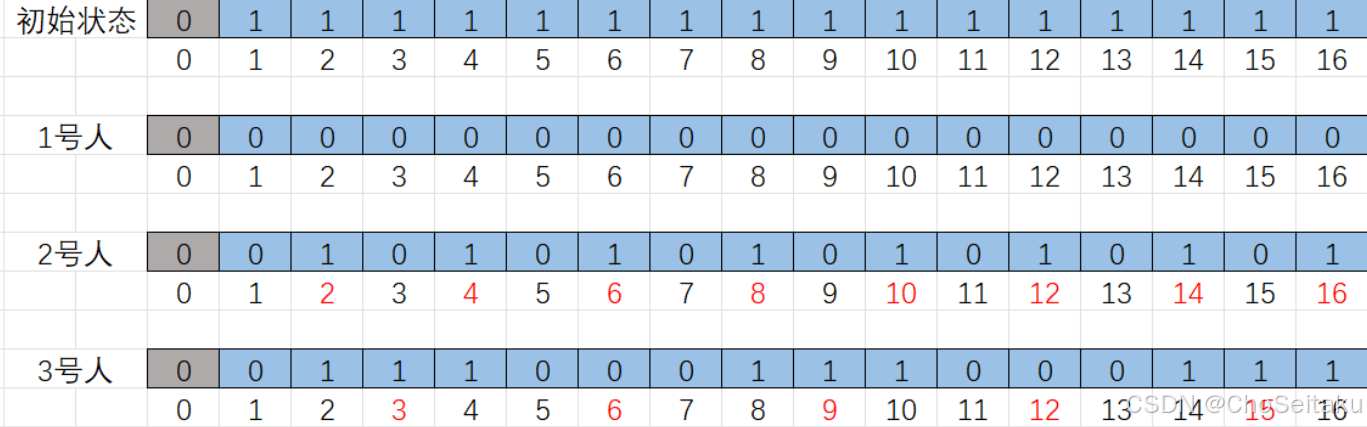
c++
#include <bits/stdc++.h>
using namespace std;
const int N = 5010;
int a[N] = {0};
int main()
{
ios::sync_with_stdio(false);
cin.tie(nullptr);
int n;
cin >> n;
for(int i = 2; i <= n; i++)
{
for (int j = i; j <= n; j++)
{
if (j % i == 0)
{
//arr[j] = !arr[j];
if(a[j] == 1)
a[j] = 0;
else
a[j] = 1;
}
}
}
for (int i = 1; i <= n; i++)
{
if (a[i] == 0)
cout << i << ' ';
}
cout << endl;
return 0;
}
P1428 小鱼比可爱 - 洛谷
c++
#include <bits/stdc++.h>
using namespace std;
int main()
{
ios::sync_with_stdio(false);
cin.tie(nullptr);
int n;
cin >> n;
vector<int> a(n);
for (auto &x : a)
{
cin >> x;
}
for (int i = 0; i < n; i++)
{
int cnt = 0;
for (int j = 0; j < i; j++)
{
if (a[j] < a[i])
{
cnt++;
}
}
cout << cnt << ' ';
}
return 0;
}
冒泡排序
冒泡排序的原理:通过重复地遍历待排序的数列,依次⽐较相邻元素并交换,使得每⼀轮遍历都将未排序部分的最⼤或最⼩值"冒泡"到数列的⼀端
c++
#include <bits/stdc++.h>
using namespace std;
int main()
{
ios::sync_with_stdio(false);
cin.tie(nullptr);
int n;
cin >> n;
vector<int> a(n);
for (auto &x : a)
{
cin >> x;
}
for (int i = 0; i < n - 1; i++)
{
for (int j = 0; j < n - 1 - i; j++)
{
if (a[j] < a[j + 1])
{
int tmp = a[j];
a[j] = a[j+1];
a[j+1] = tmp;
}
}
}
for (auto x : a)
{
cout << x << endl;
}
return 0;
}