在处理大型PDF文件时,将它们分解成更小、更易于管理的块通常是有益的。这个过程称为分区,它可以提高处理效率,并使分析或操作文档变得更容易。在本文中,我们将讨论如何使用Python和为Unstructured.io库将PDF文件划分为更小的部分。
我们将使用两个Python库来完成此任务:
- PyPDF2:一个可以读、写、合并和分割PDF文件的库。
- Unstructured.io:一个可以使用文档图像分析模型分割PDF文档的库。
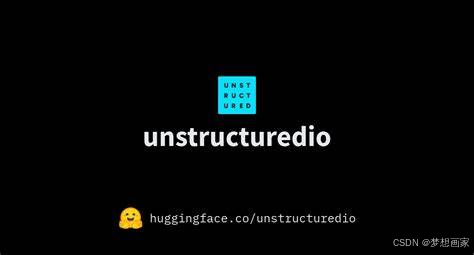
下面是完成这个任务的Python代码:
python
from PyPDF2 import PdfReader, PdfWriter
from unstructured.partition.pdf import partition_pdf
import os
from os import path
# Create the output directory if it doesn't exist
# os.makedirs('./output', exist_ok=True)
path = path.abspath(path.dirname(__file__))
# pdf_file = path + '/sample01.pdf'
filename = path + "/sample02.pdf"
# Read the original PDF
input_pdf = PdfReader(f'{filename}')
batch_size = 2
num_batches = len(input_pdf.pages) // batch_size + 1
filename = path + "/output"
# Extract batches of 100 pages from the PDF
for b in range(num_batches):
writer = PdfWriter()
# Get the start and end page numbers for this batch
start_page = b * batch_size
end_page = min((b+1) * batch_size, len(input_pdf.pages))
# Add pages in this batch to the writer
for i in range(start_page, end_page):
writer.add_page(input_pdf.pages[i])
# Save the batch to a separate PDF file
batch_filename = f'{filename}-batch{b+1}.pdf'
with open(batch_filename, 'wb') as output_file:
writer.write(output_file)
# Now you can use the `partition_pdf` function from Unstructured.io to analyze the batch
elements = partition_pdf(filename=batch_filename)
print(elements)
# Do something with `elements`...
# This will process without issue
# 抽取表格数据
elements = partition_pdf("copy-protected.pdf", strategy="hi_res")
第一步:读PDF文件
首先,我们从PyPDF2库导入必要的类:PdfReader和PdfWriter。PdfReader类用于读取原始PDF文件,该文件存储在名为"exam-prep"的子目录中。
步骤2:分区PDF
我们决定批大小,即PDF的每个块将包含的页数。在本例中,我们选择了100页的批处理大小,但这可以根据您的需要进行调整。
然后通过将PDF中的总页数除以批大小来计算批数量。添加1以确保在页面总数不是批大小的倍数时捕获所有剩余页面。
步骤3:写PDF块
接下来,循环遍历每个批处理,为每个批处理创建一个新的PdfWriter对象。对于每个批处理,我们计算起始页码和结束页码,并使用add_page方法将该范围内的每个页码添加到PdfWriter。
一旦添加了批处理的所有页面,我们将它们写入'output'子目录下的新PDF文件中。每个块的文件名包括原始文件名和批号。
步骤4:分析PDF块
将PDF分成更小的块后,现在可以使用来自非结构化的partition_pdf函数。IO库来分析每个批处理。该函数使用文档图像分析模型对PDF文档进行分段,并返回已解析PDF文档页面中出现的元素列表。
最后总结
将大型PDF文件划分为更小的块可以使它们更容易、容错和消耗更少的内存。