清明假期在家闲来无事,突发奇想能不能自己用Java写一个控制台进度条打印工具,然后马上开干。
1. 效果图

图1 默认打印

图2 带状态和任务名称打印
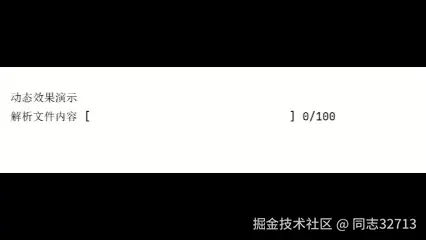
图3 动态打印效果展示
2. 代码
java
/**
* 控制台进度条打印工具
*/
public class ProgressBarPrintingUtils {
/**
* 使用示例
*/
public static void main(String[] args) throws InterruptedException {
// 1.进度条打印(直接传参)
System.out.println("进度条打印1");
printProgressBar(100, 50, 30, '=', null, null, null);
System.out.println();
// 2.进度条打印(通过进度条对象传参)
System.out.println("\n进度条打印2");
printProgressBar(new ProgressBarBuilder()
.total(100)
.completed(80)
.length(30)
.character('#')
.state(State.ERROR)
.name("任务一")
.description("")
.build());
System.out.println();
// 3.动态效果演示
System.out.println("\n动态效果演示");
Random r = new Random();
ProgressBar progressBar = createProgressBarBuilder()
.total(100)
.completed(0)
.length(30)
.character('█')
.name("解析文件内容")
.build();
while (true) {
printProgressBar(progressBar);
if (progressBar.completed >= progressBar.total) {
break;
}
Thread.sleep(500);
progressBar.completed += r.nextInt(10);
if (progressBar.getCompleted() >= progressBar.getTotal()) {
progressBar.setCompleted(progressBar.getTotal());
progressBar.setState(State.SUCCESS);
progressBar.setDescription("解析完成");
}
}
System.out.println();
}
/**
* 打印进度条
*
* @param total 任务总数
* @param completed 已完成任务数量
* @param length 进度条的长度,单位为字符
* @param character 填充进度进度条字符
* @param state 进度条的状态,用于在控制台显示不同的文字颜色
*
*/
public static void printProgressBar(int total, int completed, int length, char character,
State state, String name, String description) {
System.out.print("\r");
System.out.print(getColorStartTagByState(state));
if (name != null) {
System.out.print(name);
System.out.print(" ");
}
System.out.print("[");
int ratio = completed >= total ? length : (int) Math.floor(completed / (double)total * length);
for (int i = 1; i <= ratio; i++) {
System.out.print(character);
}
for (int i = 1; i <= length - ratio; i++) {
System.out.print(" ");
}
System.out.print("] ");
System.out.print(completed);
System.out.print("/");
System.out.print(total);
System.out.print(" ");
if (description != null) {
System.out.print(description);
}
if (state != null) {
System.out.print("\033[0m");
}
}
/**
* 打印进度条
*
* @param progressBar 进度条配置信息
*/
public static void printProgressBar(ProgressBar progressBar) {
if (progressBar == null) {
return;
}
printProgressBar(progressBar.total, progressBar.completed, progressBar.length, progressBar.character,
progressBar.state, progressBar.name, progressBar.description);
}
/**
* 创建进度条构造器
*/
public static ProgressBarBuilder createProgressBarBuilder() {
return new ProgressBarBuilder();
}
/**
* 通过状态枚举获取颜色开始标签
*
* @param state 状态
*/
private static String getColorStartTagByState(State state) {
if (state == null) {
return "";
}
switch (state) {
case ERROR:
return "\033[31m";
case SUCCESS:
return "\033[32m";
case WARNING:
return "\033[33m";
default:
return "";
}
}
/**
* 进度条状态枚举类
*/
public static enum State {
// 正常或默认
NORMAL,
// 警告
WARNING,
// 错误
ERROR,
// 成功
SUCCESS
}
/**
* 进度条类
*/
public static class ProgressBar {
private int total;
private int completed;
private int length;
private char character;
private State state;
private String name;
private String description;
private ProgressBar(ProgressBarBuilder builder) {
this.total = builder.total;
this.completed = builder.completed;
this.length = builder.length;
this.character = builder.character;
this.state = builder.state;
this.name = builder.name;
this.description = builder.description;
}
public int getTotal() {
return total;
}
public void setTotal(int total) {
this.total = total;
}
public int getCompleted() {
return completed;
}
public void setCompleted(int completed) {
this.completed = completed;
}
public int getLength() {
return length;
}
public void setLength(int length) {
this.length = length;
}
public char getCharacter() {
return character;
}
public void setCharacter(char character) {
this.character = character;
}
public State getState() {
return state;
}
public void setState(State state) {
if (state == null) {
this.state = State.NORMAL;
return;
}
this.state = state;
}
public String getName() {
return name;
}
public void setName(String name) {
if (name == null) {
this.name = "";
}
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
if (description == null) {
this.description = "";
}
this.description = description;
}
}
/**
* 进度条构造类
*/
public static class ProgressBarBuilder {
private int total;
private int completed;
private int length;
private char character;
private State state;
private String name;
private String description;
public ProgressBarBuilder() {
this.total = 100;
this.completed = 0;
this.length = 20;
this.character = '=';
this.state = State.NORMAL;
this.name = "";
this.description = "";
}
public ProgressBarBuilder total(int total) {
this.total = total;
return this;
}
public ProgressBarBuilder completed(int completed) {
this.completed = completed;
return this;
}
public ProgressBarBuilder length(int length) {
this.length = length;
return this;
}
public ProgressBarBuilder character(char character) {
this.character = character;
return this;
}
public ProgressBarBuilder state(State state) {
this.state = state;
return this;
}
public ProgressBarBuilder name(String name) {
if (name == null) {
name = "";
}
this.name = name;
return this;
}
public ProgressBarBuilder description(String description) {
if (description == null) {
description = "";
}
this.description = description;
return this;
}
public ProgressBar build() {
return new ProgressBar(this);
}
}
}
以上代码基于个人爱好编写只用作分享,肯定有不足的地方,欢迎大家提出宝贵的意见和看法。