我使用lombok的@Data注解带来的坑。
代码如下:
公共类:
bash
package com.tyler.oshi.common;
import lombok.Data;
import lombok.NoArgsConstructor;
/**
* @author: TylerZhong
* @description:
*/
@Data
@NoArgsConstructor
public class R {
private int code;
private String msg;
private Object data;
public R(int code, String msg) {
this.code = code;
this.msg = msg;
}
public R(int code, String msg, Object data) {
this.code = code;
this.msg = msg;
this.data = data;
}
public static R ok() {
return new R(200, "success");
}
public static R ok(Object data) {
return new R(200, "success", data);
}
public static R error() {
return new R(500, "error");
}
public static R error(Object data) {
return new R(500, "error", data);
}
public static R error(int code, String msg) {
return new R(code, msg);
}
}
接口:
java
package com.tyler.oshi.controller;
import com.tyler.oshi.common.R;
import com.tyler.oshi.service.CpuLoadMetricsService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* @author: TylerZhong
* @description:
*/
@RequestMapping("/metrics")
@RestController
public class CpuLoadRestController {
@Autowired
private CpuLoadMetricsService cpuLoadMetricsService;
@GetMapping(value = "/cpuload")
public R getCpuLoad() {
double[] cpuLoad = cpuLoadMetricsService.getCpuLoad();
return R.ok(cpuLoad);
}
}
pom.xml
xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.3.10</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.tyler</groupId>
<artifactId>oshi-app</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>oshi-app</name>
<description>oshi-app</description>
<url/>
<licenses>
<license/>
</licenses>
<developers>
<developer/>
</developers>
<scm>
<connection/>
<developerConnection/>
<tag/>
<url/>
</scm>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.github.oshi</groupId>
<artifactId>oshi-core</artifactId>
<version>6.6.5</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<annotationProcessorPaths>
<path>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
postman请求返回如下:
json
{
"timestamp": "2025-04-10T02:26:12.897+00:00",
"status": 406,
"error": "Not Acceptable",
"path": "/metrics/cpuload"
}
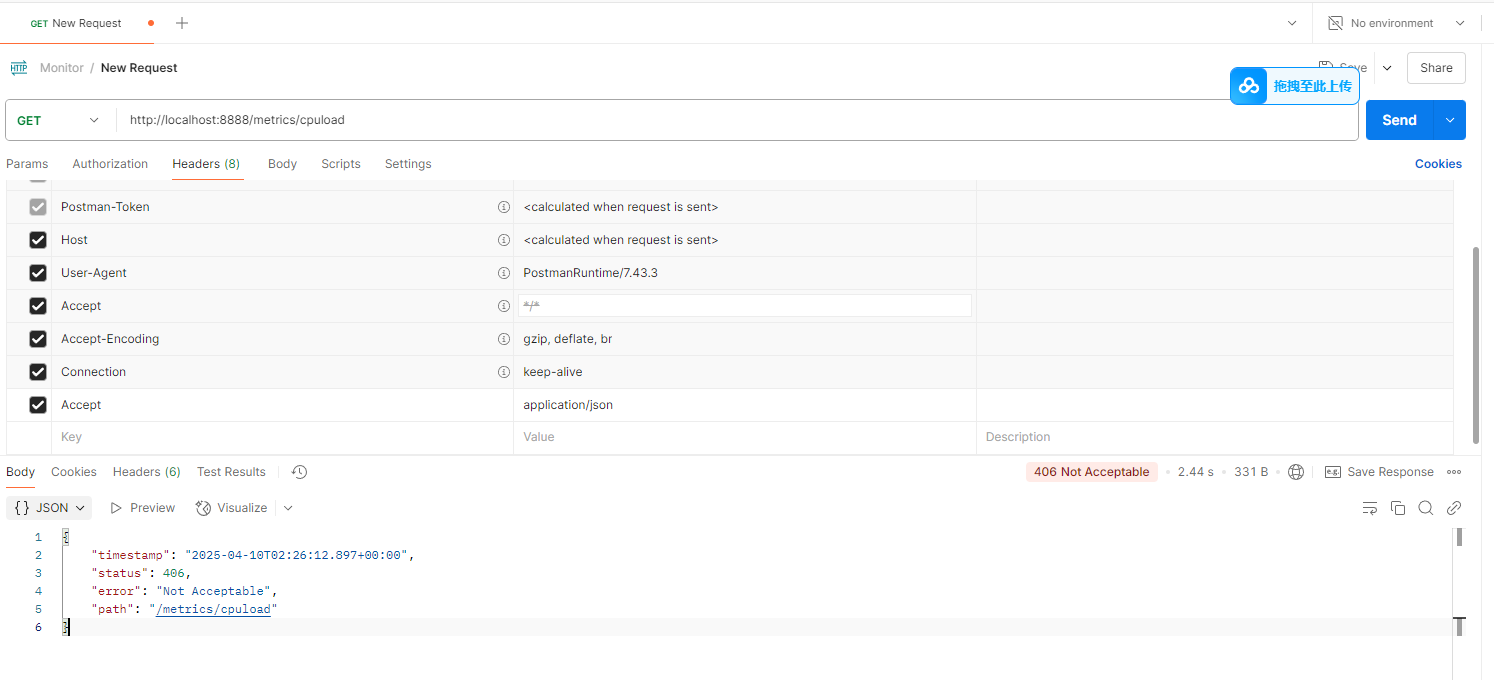
经排查,是lombok注解没有生效。
验证步骤:
检查 Lombok 是否生成 Getter
在 IDE 中编译项目,查看 R 类的编译后的类文件(位于 target/classes 或 build/classes),确认是否存在 getCode()、getMsg()、getData() 方法。
可以看到我的classpath下面的R没有给我生成 getter 方法:
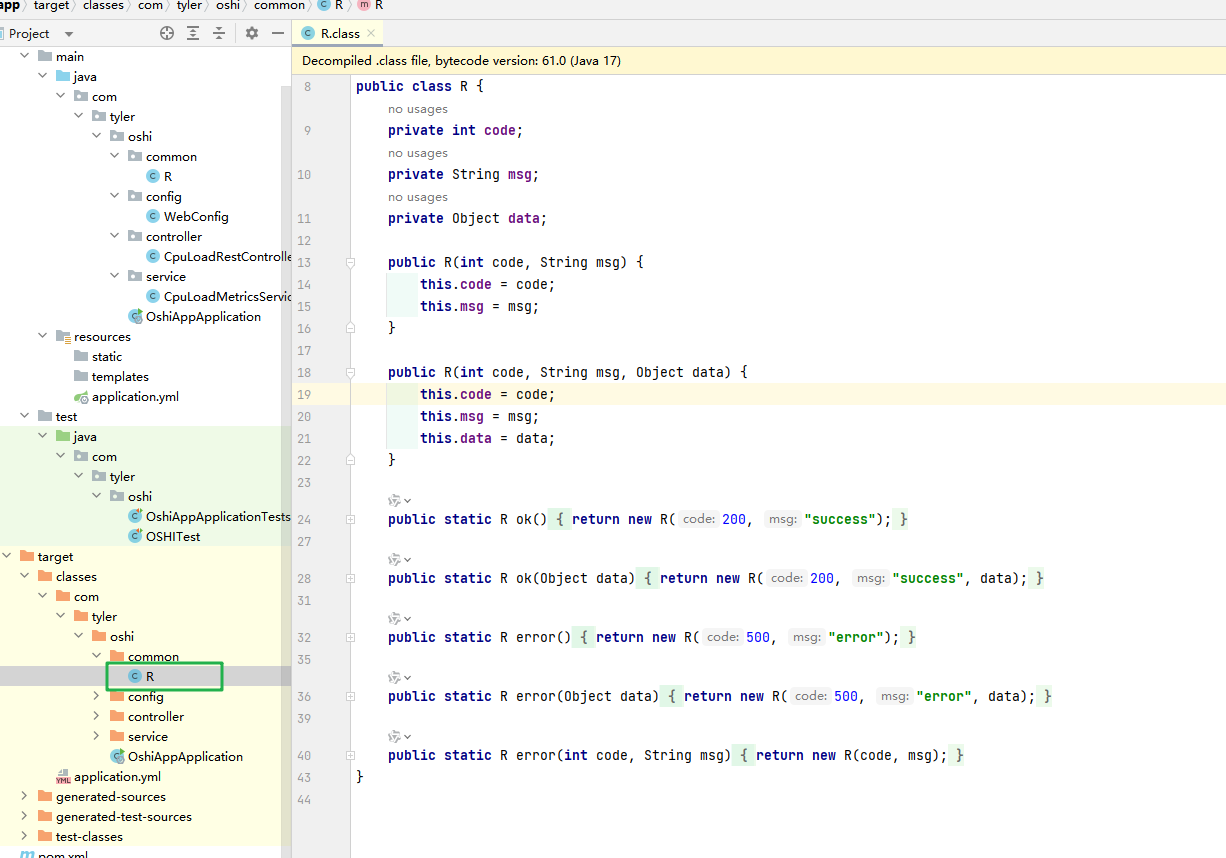
我试了换成 @Getter 注解也没用。
解决方案:手动加上 Getter 和 Setter 方法:
java
package com.tyler.oshi.common;
import lombok.Data;
import lombok.NoArgsConstructor;
/**
* @author: TylerZhong
* @description:
*/
@Data
@NoArgsConstructor
public class R {
private int code;
private String msg;
private Object data;
public R(int code, String msg) {
this.code = code;
this.msg = msg;
}
public R(int code, String msg, Object data) {
this.code = code;
this.msg = msg;
this.data = data;
}
public static R ok() {
return new R(200, "success");
}
public static R ok(Object data) {
return new R(200, "success", data);
}
public static R error() {
return new R(500, "error");
}
public static R error(Object data) {
return new R(500, "error", data);
}
public static R error(int code, String msg) {
return new R(code, msg);
}
// 手动添加Getter和Setter方法
public int getCode() {
return code;
}
public void setCode(int code) {
this.code = code;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
}