1、build.gradle.kts
scss
plugins {
id("com.android.application")
id("org.jetbrains.kotlin.android")
id("org.jetbrains.kotlin.plugin.compose")
}
android {
namespace = "com.codelab.basics"
compileSdk = 36
defaultConfig {
applicationId = "com.codelab.basics"
minSdk = 24
targetSdk = 36
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
vectorDrawables {
useSupportLibrary = true
}
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
kotlinOptions {
jvmTarget = "1.8"
}
buildFeatures {
compose = true
}
packaging {
resources {
excludes += "/META-INF/{AL2.0,LGPL2.1}"
}
}
}
dependencies {
implementation("androidx.core:core-ktx:1.15.0")
implementation("androidx.lifecycle:lifecycle-runtime-ktx:2.8.7")
implementation("androidx.activity:activity-compose:1.10.0")
implementation(platform("androidx.compose:compose-bom:2025.02.00"))
implementation("androidx.compose.ui:ui")
implementation("androidx.compose.ui:ui-graphics")
implementation("androidx.compose.ui:ui-tooling-preview")
implementation("androidx.compose.material3:material3")
implementation("androidx.compose.material:material-icons-extended")
testImplementation("junit:junit:4.13.2")
androidTestImplementation("androidx.test.ext:junit:1.2.1")
androidTestImplementation("androidx.test.espresso:espresso-core:3.6.1")
androidTestImplementation(platform("androidx.compose:compose-bom:2025.02.00"))
androidTestImplementation("androidx.compose.ui:ui-test-junit4")
debugImplementation("androidx.compose.ui:ui-tooling")
debugImplementation("androidx.compose.ui:ui-test-manifest")
}
2、plugins说明
构建脚本中使用的插件:
- 应用
com.android.application
插件,用于配置Android应用项目。 - 应用
org.jetbrains.kotlin.android
插件,启用Kotlin对Android的支持。 - 应用
org.jetbrains.kotlin.plugin.compose
插件,启用Jetpack Compose功能支持。
上面简单的几行,代码了android项目,需要有Kotlin的支持,还启用了Compose支持,如果删除了以后,系统将会报错:
1)删除org.jetbrains.kotlin.android
:
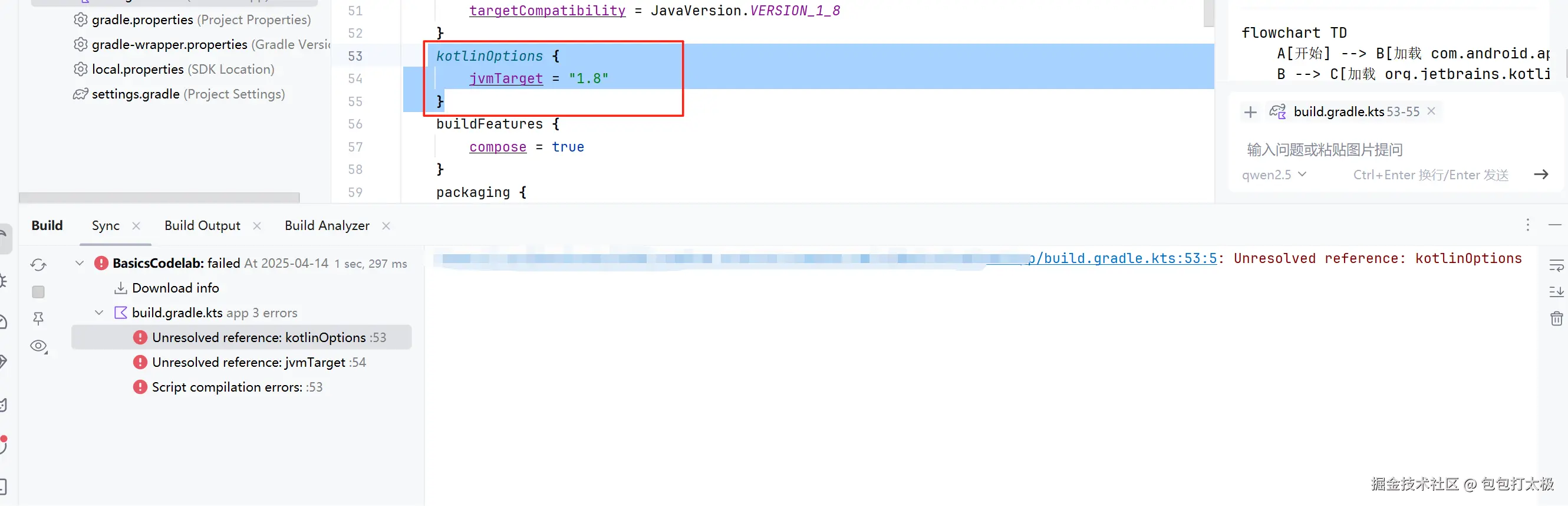
配制文件中用到的kotlin的一些项会直接报错。
2)删除org.jetbrains.kotlin.plugin.compose
: 项目是基于jetpack compose的,所以一定要启用,否则直接就报错。
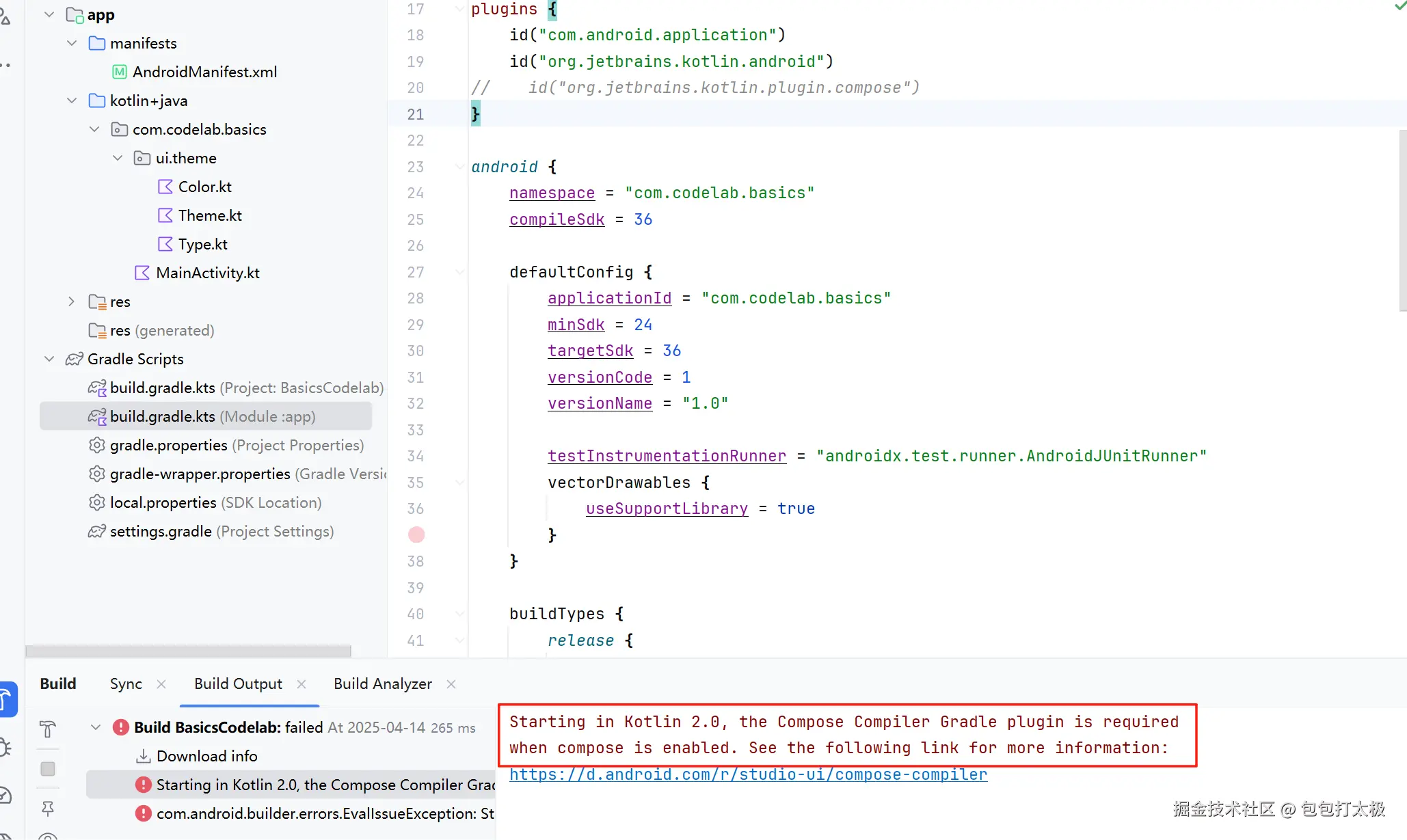
3、android {}
用于配置Android项目的构建参数。
namespace
:命名空间
compileSdk
:SDK版本
applicationId
:应用ID。
minSdk = 24
最低SDK版本
targetSdk = 36
目标SDK版本
versionCode = 1
版本号
versionName = "1.0"
版本号
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
用于在 Android 项目中执行 instrumentation 测试。它指定了测试框架的入口点,使测试能够在设备或模拟器上运行。
ini
vectorDrawables {
useSupportLibrary = true
}
配置 vectorDrawables
,启用支持库以兼容旧版本 Android 系统中的矢量图绘制功能
arduino
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro")
}
}
配置构建类型,定义release版本的混淆设置和ProGuard规则文件
ini
compileOptions {
sourceCompatibility = JavaVersion.VERSION\_1\_8
targetCompatibility = JavaVersion.VERSION\_1\_8
}
kotlinOptions {
jvmTarget = "1.8"
}
设置编译选项,指定Java和Kotlin的兼容性版本为1.8
ini
buildFeatures {
compose = true
}
启用Jetpack Compose
构建特性
arduino
packaging {
resources {
excludes += "/META-INF/{AL2.0,LGPL2.1}"
}
}
配置打包时资源文件的排除规则
4、dependencies
Kotlin代码定义了一个Gradle构建文件中的依赖项配置,主要用于Android项目的依赖管理。
区分了不同环境的依赖,如implementation
用于生产环境,testImplementation
用于测试环境,debugImplementation
用于调试环境。
core-ktx
:用于Android核心库的Kotlin扩展。
lifecycle-runtime-ktx
:用于Android生命周期的Kotlin扩展。
activity-compose
:用于将Jetpack Compose与Activity集成。
compose-bom
:用于管理Jetpack Compose库的版本。引入Compose BOM(Bill of Materials)作为平台依赖,统一管理版本。
ui、ui-graphics、ui-tooling-preview
:用于Jetpack Compose的UI库。
material3
:用于Jetpack Compose的Material Design组件。
material-icons-extended
:用于Jetpack Compose的扩展Material图标。
调试与单元测试
testImplementation("junit:junit:4.13.2")
:添加JUnit 4框架用于单元测试。
androidTestImplementation("androidx.test.ext:junit:1.2.1")
:添加Android测试扩展库,增强JUnit功能。
androidTestImplementation("androidx.test.espresso:espresso-core:3.6.1")
:添加Espresso库,用于UI交互测试。
androidTestImplementation(platform("androidx.compose:compose-bom:2025.02.00"))
和 androidTestImplementation("androidx.compose.ui:ui-test-junit4")
:配置Jetpack Compose测试支持。
debugImplementation("androidx.compose.ui:ui-tooling")
和 debugImplementation("androidx.compose.ui:ui-test-manifest")
:添加调试工具和测试清单文件支持。 控制流图
5、总结
至此一个常用的build.gradle.kts文件,就基本上了解清楚了。