一、蓝牙连接断开概述
在Android蓝牙开发中,连接稳定性是一个永恒的话题。无论是普通的经典蓝牙还是低功耗蓝牙(BLE),连接断开都是不可避免的常见现象。但是,完善的断连处理机制能让用户体验从"糟糕"变为"无感"。本文将详细探讨Android蓝牙连接断开的各种场景、处理机制以及最佳实践。
蓝牙连接断开是不可避免的常见现象,可分为三类:
- 主动断开:应用程序调用断开API、用户关闭蓝牙
- 超时断开:连接过程超时、数据传输超时、心跳包响应超时
- 异常断开:信号弱、设备超出范围、外围设备关闭、系统休眠
Android系统对蓝牙断连的基本处理流程
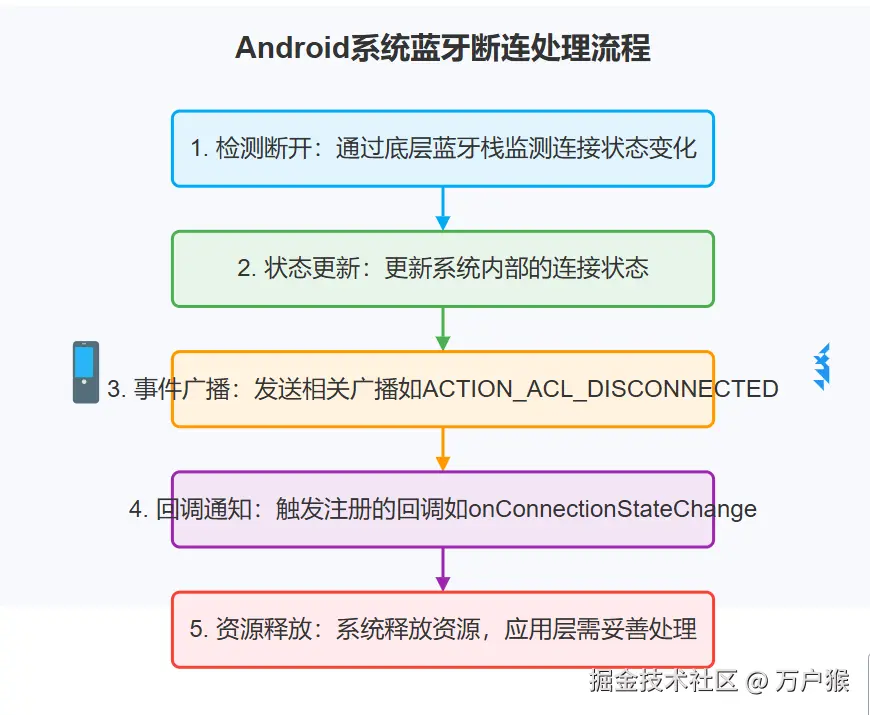
系统提供的这些机制为应用开发者提供了基础,但要构建可靠的蓝牙应用,仍需开发者进行更复杂的处理。
二、主动断开连接的正确实现
disconnect()
与close()
的区别
-
BluetoothGatt.disconnect()
:- 发起GATT断开请求
- 保留GATT客户端实例以便重连
- 会触发
onConnectionStateChange
回调
-
BluetoothGatt.close()
:- 释放所有GATT连接资源
- 调用后不应再使用此实例
- 不会触发额外回调
正确顺序:先disconnect()
,回调确认断开后再close()
。
kotlin
fun disconnectAndClose() {
if (bluetoothGatt != null) {
isDisconnecting = true // 标记为主动断开
bluetoothGatt?.disconnect()
// close()将在onConnectionStateChange回调中调用
}
}
override fun onConnectionStateChange(gatt: BluetoothGatt, status: Int, newState: Int) {
if (newState == BluetoothProfile.STATE_DISCONNECTED) {
if (isDisconnecting) {
// 预期的断开,释放资源
gatt.close()
bluetoothGatt = null
isDisconnecting = false
} else {
// 意外断开,可能需要重连
handleUnexpectedDisconnect(status)
}
}
}
状态管理与线程安全
使用原子变量确保线程安全:
kotlin
private val connectionState = AtomicInteger(STATE_DISCONNECTED)
@Synchronized
private fun updateConnectionState(newState: Int) {
val oldState = connectionState.getAndSet(newState)
if (oldState != newState) {
notifyStateChanged(newState)
}
}
推荐使用状态机模式管理连接状态,明确定义各种状态及其转换条件:
kotlin
object BleConnectionState {
const val DISCONNECTED = 0
const val CONNECTING = 1
const val CONNECTED = 2
const val DISCONNECTING = 3
const val RECONNECTING = 4
fun toString(state: Int): String = when(state) {
DISCONNECTED -> "DISCONNECTED"
CONNECTING -> "CONNECTING"
CONNECTED -> "CONNECTED"
DISCONNECTING -> "DISCONNECTING"
RECONNECTING -> "RECONNECTING"
else -> "UNKNOWN($state)"
}
}
三、超时断开处理策略
自定义超时监控
Android框架未提供直接修改超时参数的API,需实现自定义超时处理:
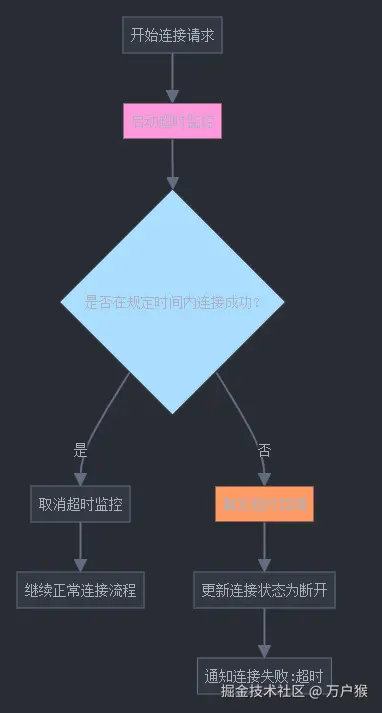
kotlin
class ConnectionTimeoutHandler(private val timeoutMs: Long = 30000) {
private val handler = Handler(Looper.getMainLooper())
private var timeoutRunnable: Runnable? = null
fun startConnectionTimeout(onTimeout: () -> Unit) {
cancelTimeout()
timeoutRunnable = Runnable {
onTimeout()
}
handler.postDelayed(timeoutRunnable!!, timeoutMs)
}
fun cancelTimeout() {
timeoutRunnable?.let { handler.removeCallbacks(it) }
timeoutRunnable = null
}
}
使用示例:
kotlin
connectionTimeoutHandler.startConnectionTimeout {
// 连接超时处理
updateConnectionState(BleConnectionState.DISCONNECTED)
notifyConnectionFailed(ConnectionFailure.TIMEOUT)
}
// 尝试连接
bluetoothDevice?.connectGatt(context, false, gattCallback)
// 连接成功时取消超时
override fun onConnectionStateChange(gatt: BluetoothGatt, status: Int, newState: Int) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
connectionTimeoutHandler.cancelTimeout()
// 处理连接成功...
}
}
四、异常断开连接的检测与处理
信号强度监控
通过定期读取RSSI预测潜在断开:
kotlin
override fun onReadRemoteRssi(gatt: BluetoothGatt, rssi: Int, status: Int) {
if (status == BluetoothGatt.GATT_SUCCESS) {
// 记录RSSI历史用于趋势分析
rssiHistory.add(RssiReading(System.currentTimeMillis(), rssi))
// 信号极弱,可能即将断开
if (rssi < RSSI_CRITICAL_THRESHOLD) {
prepareForPotentialDisconnect()
}
}
}
心跳机制检测连接状态
实现简单的心跳检测机制:
kotlin
private var missedHeartbeats = 0
private fun startHeartbeatMonitor() {
handler.postDelayed(object : Runnable {
override fun run() {
if (isConnected()) {
sendHeartbeat()
if (missedHeartbeats >= 3) {
// 多次心跳无响应,连接可能已断开
handlePotentialConnectionIssue()
}
handler.postDelayed(this, HEARTBEAT_INTERVAL)
}
}
}, HEARTBEAT_INTERVAL)
}
private fun onHeartbeatResponse() {
missedHeartbeats = 0 // 收到响应,重置计数器
}
五、断开连接的广播与回调处理
GATT回调处理
分析断开状态码,区分处理不同情况:
kotlin
override fun onConnectionStateChange(gatt: BluetoothGatt, status: Int, newState: Int) {
if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 分析断开原因
val reason = analyzeDisconnectStatus(status)
when (reason) {
DisconnectReason.NORMAL,
DisconnectReason.LOCAL_HOST_TERMINATED -> {
// 预期断开,清理资源
safeCloseGatt()
}
DisconnectReason.TIMEOUT,
DisconnectReason.REMOTE_DISCONNECTED,
DisconnectReason.GATT_ERROR -> {
// 意外断开,考虑重连
if (autoReconnect) {
scheduleReconnect(gatt.device)
} else {
safeCloseGatt()
}
}
}
}
}
private fun analyzeDisconnectStatus(status: Int): DisconnectReason {
return when (status) {
BluetoothGatt.GATT_SUCCESS -> DisconnectReason.NORMAL
8 -> DisconnectReason.TIMEOUT
19 -> DisconnectReason.REMOTE_DISCONNECTED
22 -> DisconnectReason.LOCAL_HOST_TERMINATED
133 -> DisconnectReason.GATT_ERROR
else -> DisconnectReason.UNKNOWN
}
}
蓝牙状态广播监听
监听系统蓝牙状态变化:
kotlin
private val bluetoothStateReceiver = object : BroadcastReceiver() {
override fun onReceive(context: Context, intent: Intent) {
if (intent.action == BluetoothAdapter.ACTION_STATE_CHANGED) {
val state = intent.getIntExtra(BluetoothAdapter.EXTRA_STATE,
BluetoothAdapter.ERROR)
when (state) {
BluetoothAdapter.STATE_OFF -> {
// 蓝牙关闭,清理资源
handleBluetoothTurnedOff()
}
BluetoothAdapter.STATE_ON -> {
// 蓝牙开启,可能需要重连
if (autoReconnectOnBluetoothEnabled) {
reconnectToLastDevice()
}
}
}
}
}
}
六、重连机制与数据同步
指数退避重连策略
避免频繁重连消耗资源:
kotlin
private fun scheduleReconnect(device: BluetoothDevice) {
reconnectAttempts++
if (reconnectAttempts > MAX_RECONNECT_ATTEMPTS) {
// 超过最大尝试次数,放弃
return
}
// 计算退避时间
val delay = min(
BASE_RETRY_DELAY * 2.0.pow(reconnectAttempts - 1).toLong(),
MAX_RETRY_DELAY
)
handler.postDelayed({ reconnect(device) }, delay)
}
断线数据缓存与恢复
确保重连后数据同步:
kotlin
private fun handleAbruptDisconnect() {
// 保存当前传输进度
saveTransferCheckpoint()
// 缓存未发送的数据
cacheOutgoingData()
}
private fun onReconnected() {
// 恢复会话状态
restoreConnectionContext()
// 继续发送缓存数据
resumeCachedTransfers()
}
小结
高效的蓝牙断开连接处理应涵盖以下要点:
- 资源管理 :正确调用
disconnect()
和close()
释放资源 - 状态监控:通过RSSI监控、心跳检测及时发现潜在断开
- 原因分析:根据断开状态码区分处理不同情况
- 智能重连:使用指数退避策略避免无效重连
- 数据同步:保存断线状态,重连后恢复传输
良好的断连处理机制能极大提升用户体验,是高质量蓝牙应用的关键所在。