1. 网页端
1.1 框架
Vue + Element+UI + axios
1.2 框架搭建步骤
1.3 配置文件 main.js
javascript
import {createApp} from 'vue'
import ElementUi from 'element-plus'
import 'element-plus/dist/index.css';
import axios from "axios";
import router from './router'
import App from './App'
const app = createApp(App)
app.use(ElementUi)
app.use(router)
app.config.globalProperties.$http = axios
app.config.globalProperties.$axios = axios
app.mount('#app');
1.4 配置路由
1.4.1 添加 依赖
npm install vue-router@4
javascript
import { createRouter, createWebHistory } from 'vue-router';
import Home from '@/views/page/page';
const routes = [
{ path: '/page', component: Home }
];
const router = createRouter({
history: createWebHistory(),
routes
});
export default router;
1.4.2 注意Vue 的钩子函数触发事件
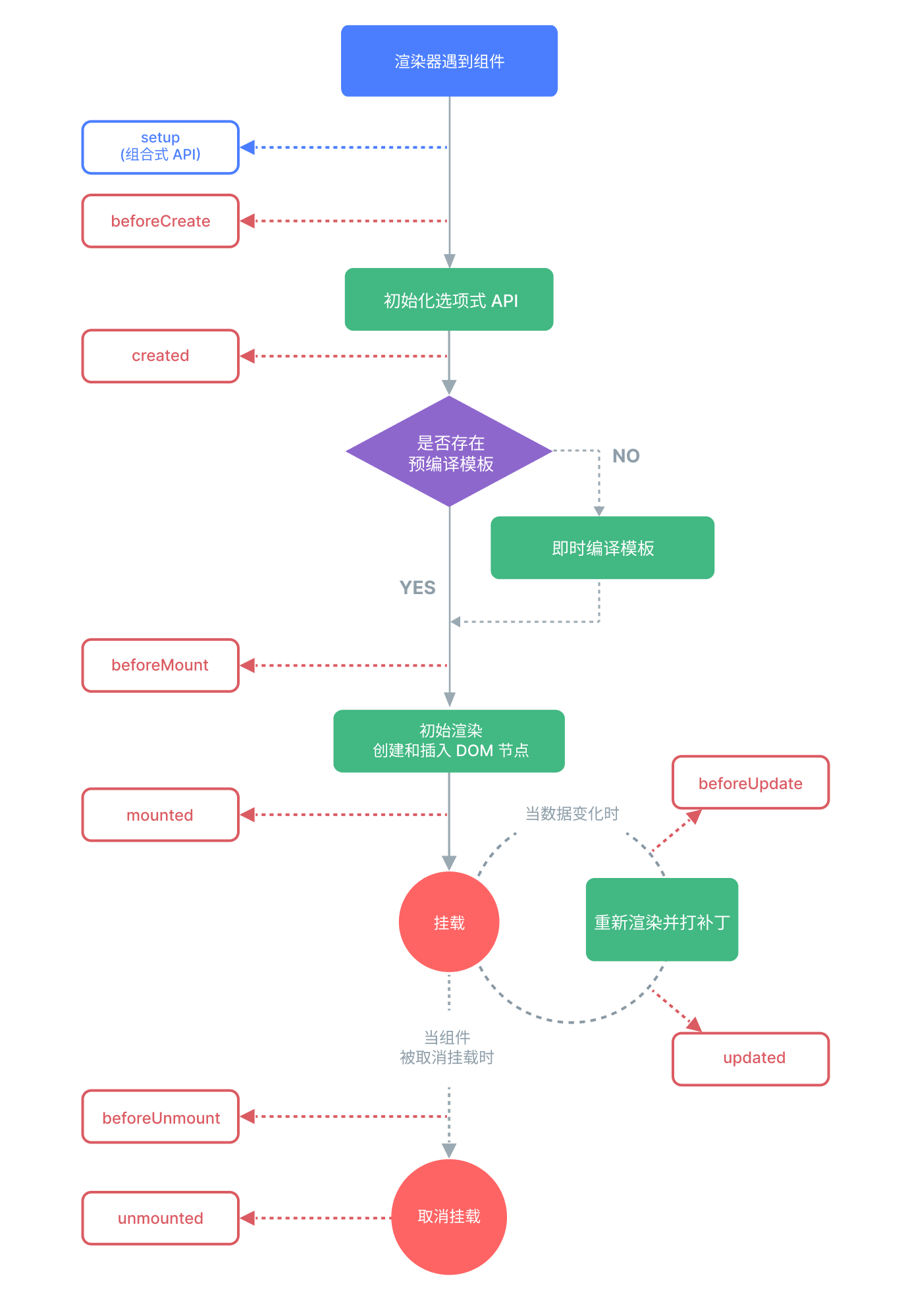
1.4.3 核心钩子
created : 首次进入刷新、一般进行首次的页面数据加载
mounted : 数据发生变更之后、重新加载
2. 后端
2.1 框架
2.1.1 语言: JAVA
2.2.2 开发框架 :
SpringBoot 2.0 、Spring cloud Finchley.SR1
2.2 微服务
2.2.1 结构
2.2.2 框架搭建步骤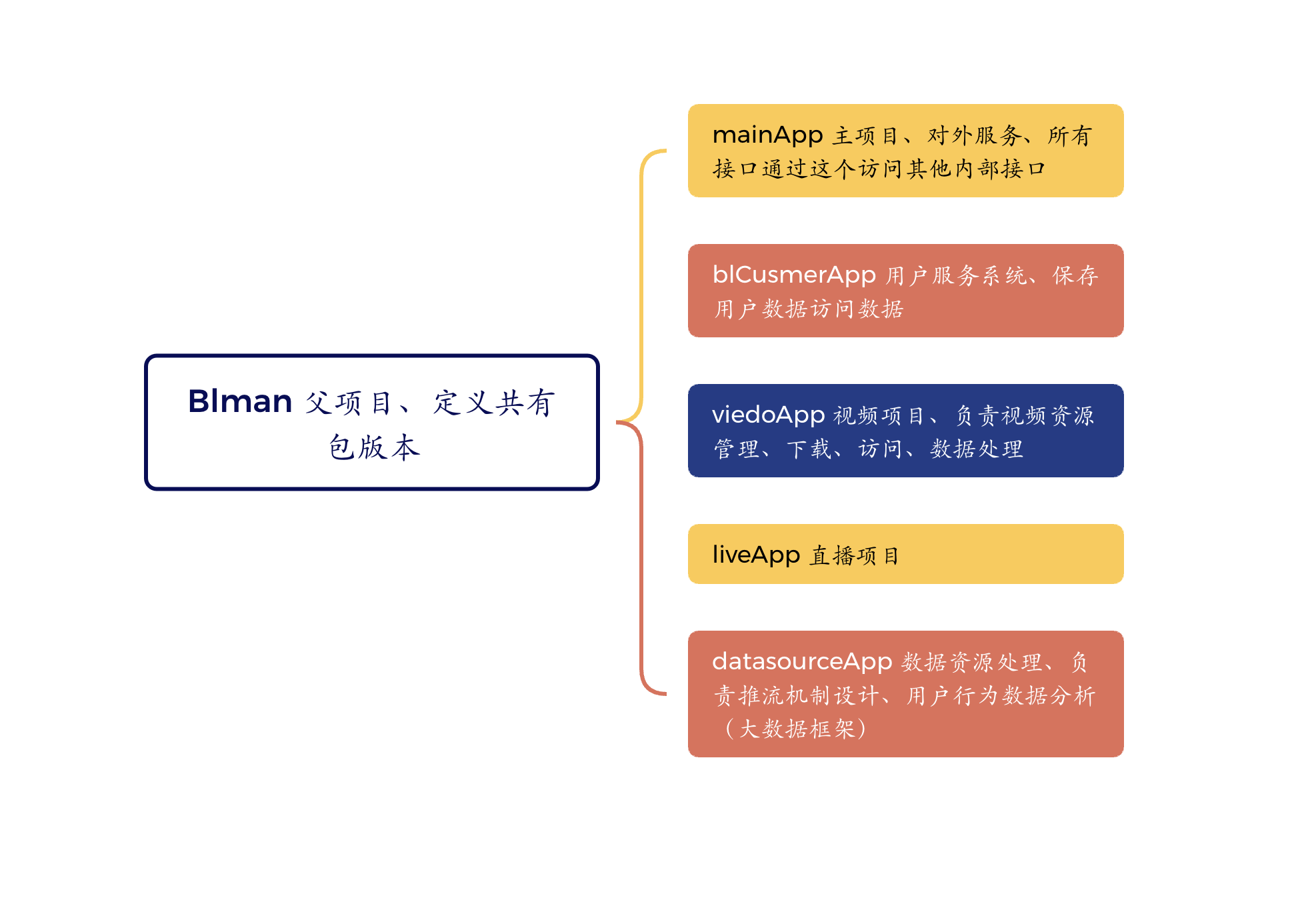
2.3 微服务节点配置
2.3.1 BlMan (父节点)
2.3.1.1 blMan parent 父节点 POM 配置
XML
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.bu</groupId>
<artifactId>blMan</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>pom</packaging>
<name>blMan</name>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<modules>
<module>mainApp</module>
<module>blUserApp</module>
<module>deploy</module>
</modules>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<!-- spring boot 启动配置 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<!-- 注册中心 nacos -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-alibaba-nacos-discovery</artifactId>
<version>2.2.0.RELEASE</version>
</dependency>
<!-- 远程调用 feign -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-feign</artifactId>
<version>1.4.7.RELEASE</version>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.2.2.RELEASE</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>Hoxton.SR1</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>2.2.0.RELEASE</version>
<executions>
<execution>
<id>repackage</id>
<goals>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
2.3.2 mainApp 所有服务网关、权限管理平台
2.3.2.1 mainApp POM 配置
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<artifactId>mainApp</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<parent>
<artifactId>blMan</artifactId>
<groupId>com.bu</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<dependencies>
<!-- mybatis 配置 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.24</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<!-- redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
<version>2.2.0.RELEASE</version>
</dependency>
<!-- 网关 -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
<!-- 负载均衡 -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-ribbon</artifactId>
</dependency>
</dependencies>
</project>
2.3.2.2 mainApp 启动类配置
java
package com.bu;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@SpringBootApplication
// 注册中心
@EnableDiscoveryClient
public class MainApp {
public static void main(String[] args) {
SpringApplication.run(MainApp.class, args);
}
}
2.3.2.2 mainApp yml 配置文件
php
# 启动端口
server:
port: 8991
# 注册中心名
spring:
application:
name: main
# redis 配置、无用户密码无需配置
redis:
host: localhost
port: 6379
# 数据源
datasource:
url: jdbc:mysql://localhost:3306/数据库?serveTimezone=GMT+8
password: 用户密码
username: 用户名
driver-class-name: com.mysql.cj.jdbc.Driver
cloud:
cloud:
# 注册中心地址
nacos:
discovery:
server-addr: http://localhost:8848
# 网关
gateway:
discovery:
locator:
enabled: true
# 路由匹配规则
routes:
- id: deploy
uri: lb://deployApp
order: 1
predicates:
- Path= /deploy/**
filters:
- StripPrefix=1
2.3.3 deployApp 配置平台信息(页面配置)
2.3.3.1 deployApp POM 配置
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<artifactId>deploy</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.24</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.18</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<parent>
<artifactId>blMan</artifactId>
<groupId>com.bu</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
</project>
2.3.3.2 deployApp 启动类 配置
java
package com.bu;
import org.mybatis.spring.annotation.MapperScan;
import org.mybatis.spring.annotation.MapperScans;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@SpringBootApplication
@EnableDiscoveryClient
@MapperScan(basePackages = "com.bu.**.**.dao")
public class DeployMain {
public static void main(String[] args) {
SpringApplication.run(DeployMain.class, args);
}
}
2.3.3.3 deployApp yml 配置
java
spring:
application:
# 注册中心 微服务名称
name: deployApp
datasource:
url: jdbc:mysql://localhost:3306/数据库?serveTimezone=GMT+8
password: 用户密码
username: 用户
driver-class-name: com.mysql.cj.jdbc.Driver
cloud:
nacos:
discovery:
server-addr: http://localhost:8848
mybatis:
mapper-locations: classpath:/mapper/**.xml
server:
port: 8992
3. 小程序端
3.1 框架
3.1.1 微信小程序、vantWeb
3.1.2 创建小程序项目 (appId 在 微信小程序官网申请,不使用云服务开发)
3.1.3 默认页面
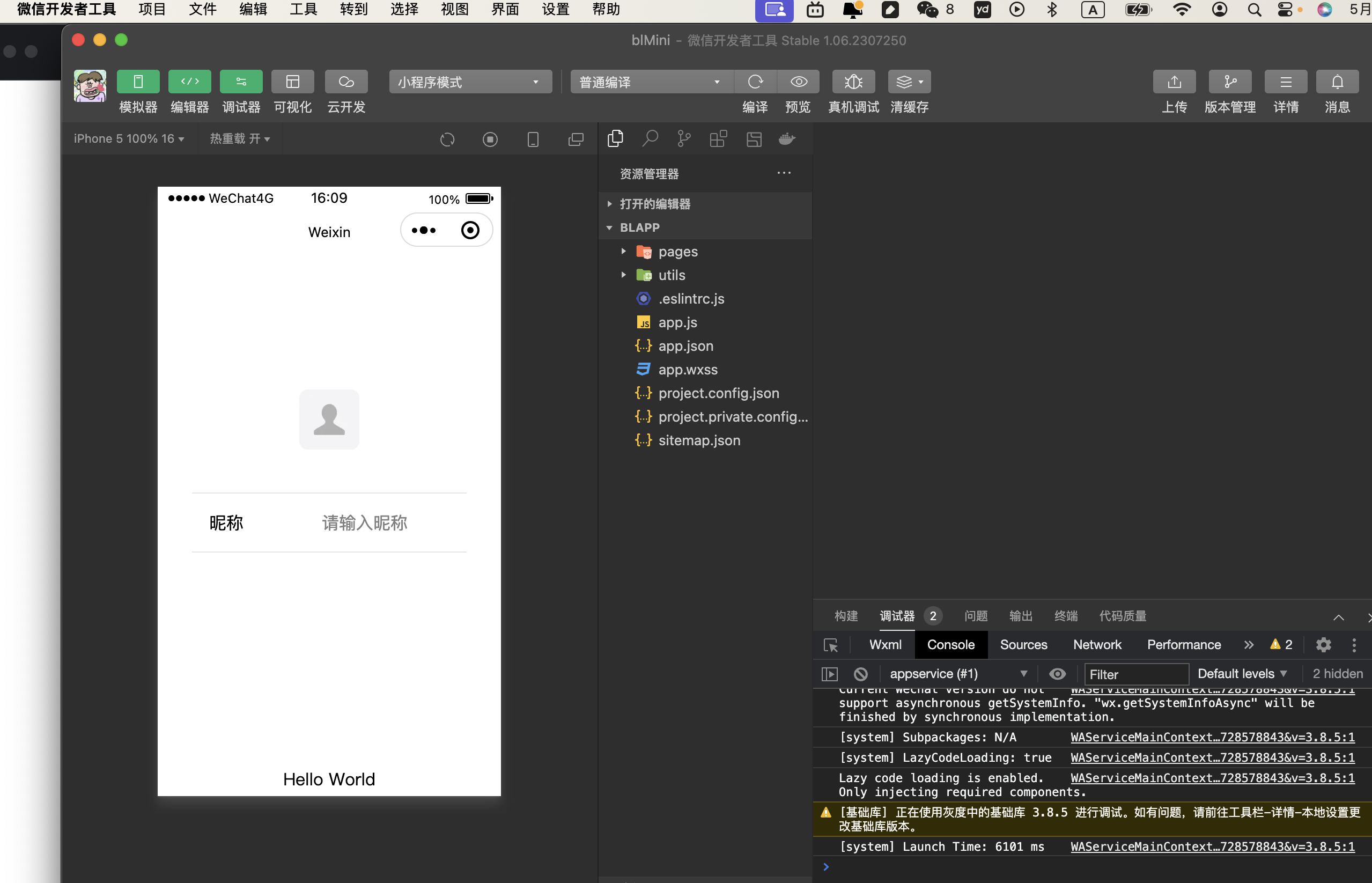
3.1.4 引入 vant-webapp ui 包
npm i @vant/weapp
上诉前端、手机端、后端框架搭建完成、开始页面编写。。。
4. vue 前端页面 布局、手机端页面布局
4.1 页面设计开发
4.1.1 首页
4.1.1.1 整体布局
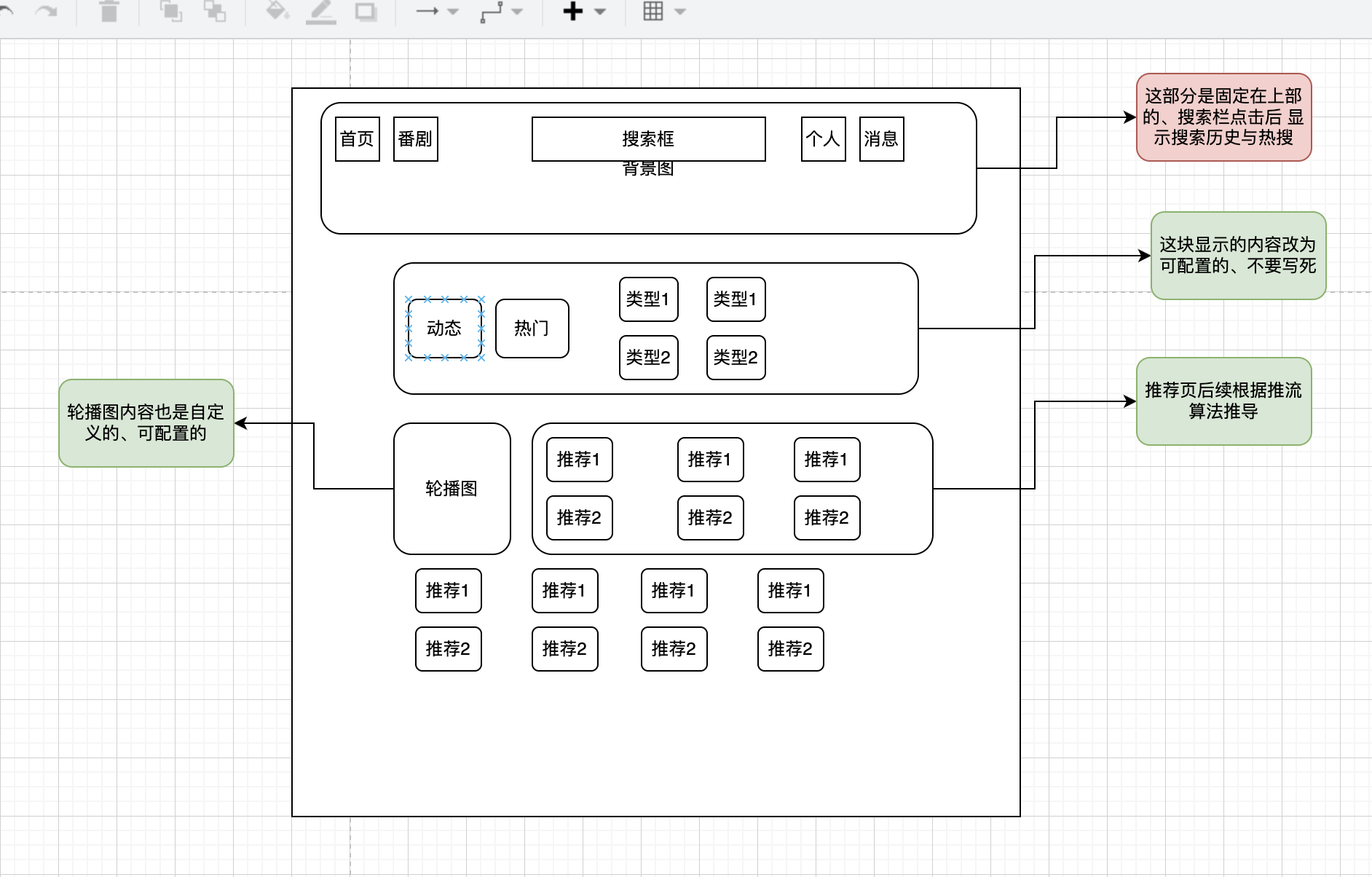
4.1.1.2 采用 element-ui 重写
4.1.1.2.1 宏观布局
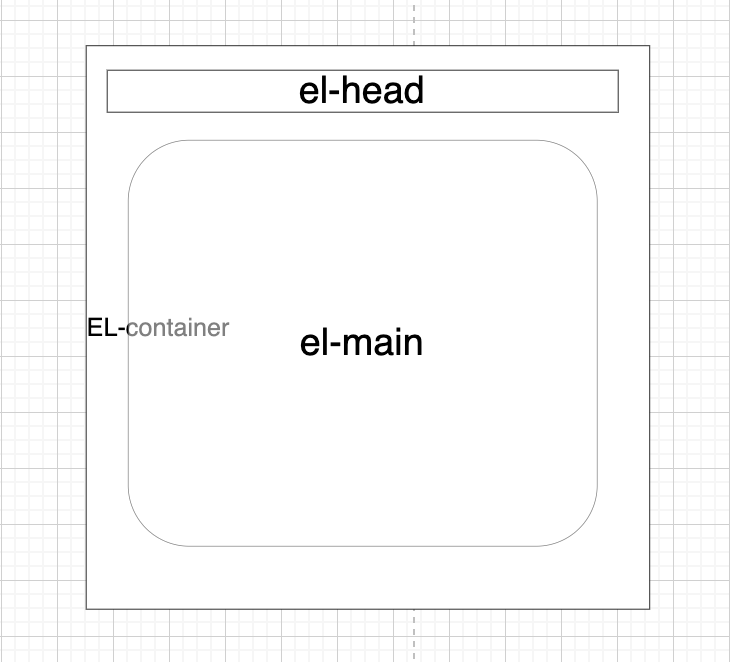
4.1.1.2.2 导航栏设计
设计思路:这块内容处于整体页面正上方、width为100%、铺满上方
UI代码要点:
javascript
el-container 整体布局、el-header 表示当前元素处于上方
导航栏 使用 el-menu 设置
子元素 el-menu-item (单菜单、可依据router跳转) 、el-submenu (二级下拉菜单)
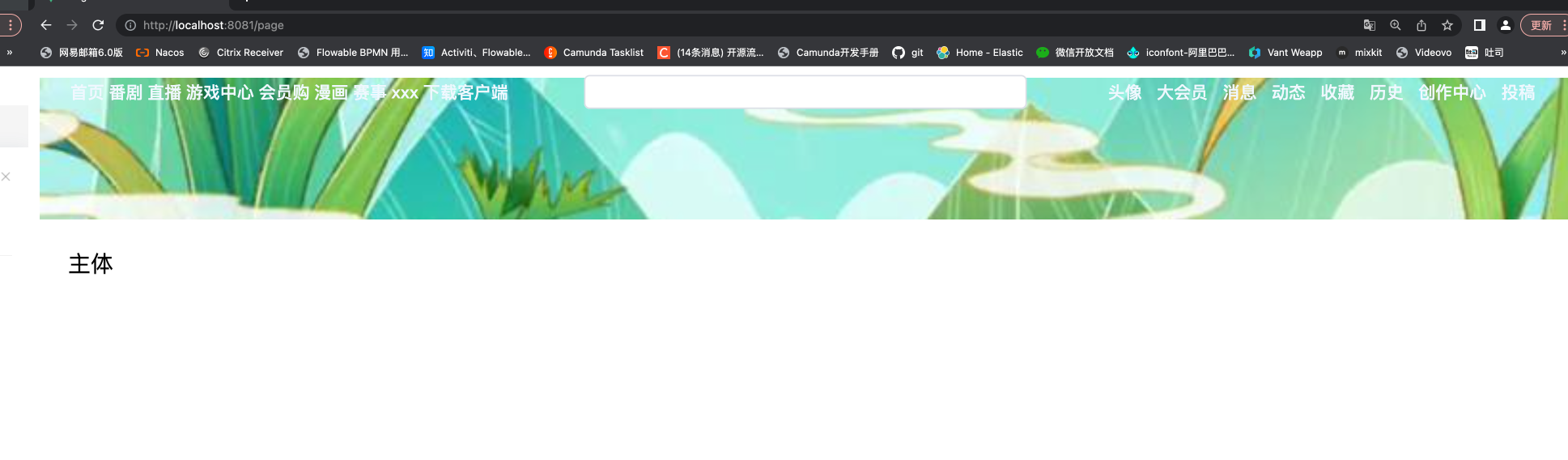
4.1.1.3 小程序首页大致布局设计
最终效果:
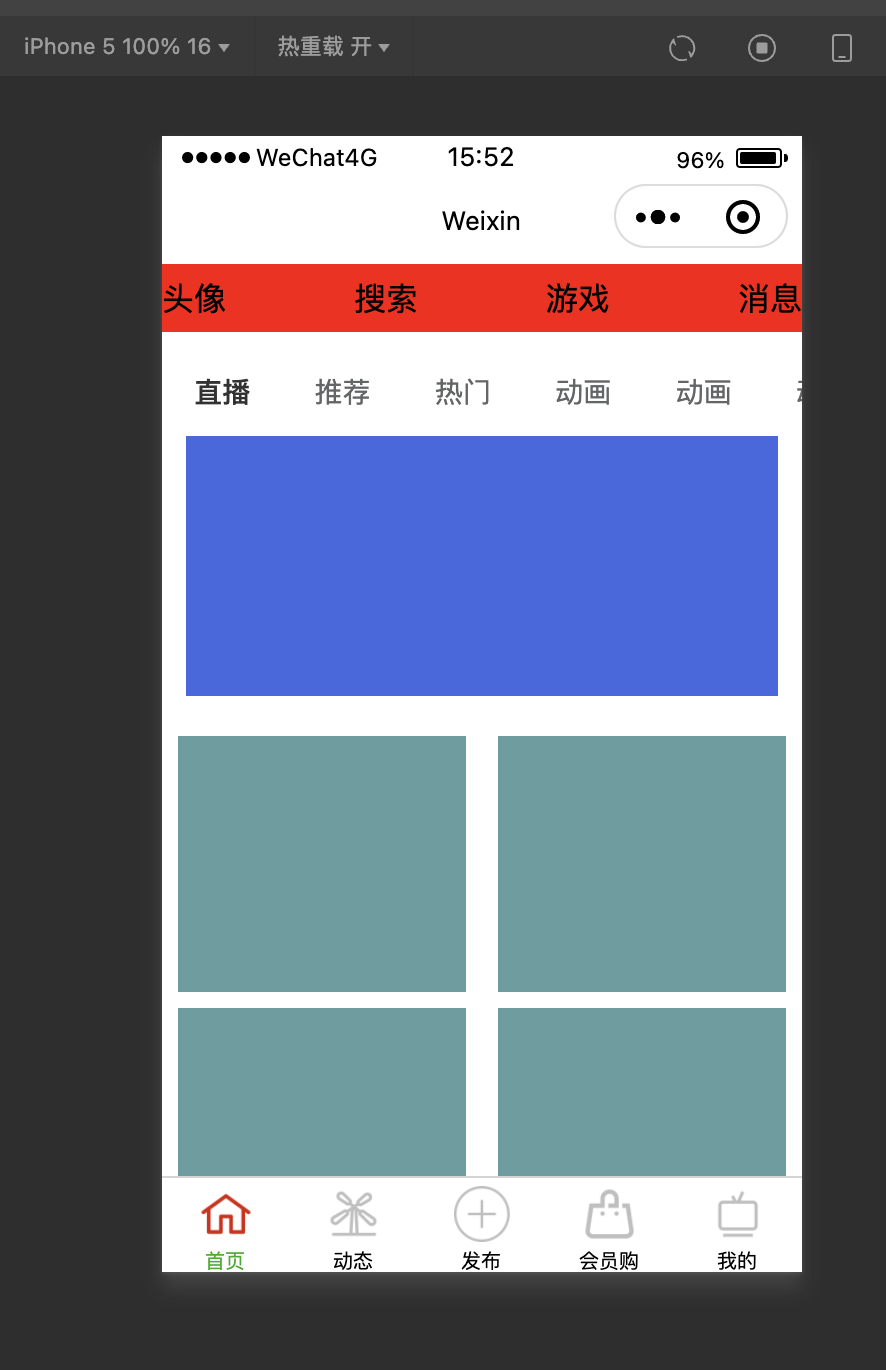
index.wxml
XML
<scroll-view scroll-x="true" class="main_tb">
<view class="head_title">
<view>头像</view>
<view>搜索</view>
<view>游戏</view>
<view>消息</view>
</view>
<view class="head_nav" style="top: {{top}};">
<view class="head_nav_title">
<van-tabs active="{{ active }}" line-width="0" ellipsis>
<van-tab wx:for="{{title_menu}}" wx:for-index="index"
wx:for-item="title_" title="{{title_.title_name}}"></van-tab>
</van-tabs>
</view>
<view class="head_nav_mark"></view>
<view class="head_nav_fixed">
固定
</view>
</view>
<view class="recommend">3</view>
<view class="vedio_main">
<view class="vedio_main_one"></view>
<view class="vedio_main_one"></view>
<view class="vedio_main_one"></view>
<view class="vedio_main_one"></view>
</view>
</scroll-view>
index.wxss
css
.main_tb {
display: flex;
justify-content: space-between;
/* 竖向排列 */
flex-direction: column;
}
.head_title {
height: 80rpx;
background-color: red;
display: flex;
justify-content: space-between;
align-items: center;
}
.head_nav {
height: 80rpx;
background-color: rosybrown;
display: flex;
justify-content: start;
flex-direction: row;
align-items: center;
position:fixed;
}
.head_nav_title {
width: 90%;
height: 80rpx;
}
.head_nav_fixed {
width: 10%;
height: 80rpx;
position: fixed;
}
.head_nav_mark {
width: 10%;
height: 80rpx;
}
.recommend {
height: 400rpx;
background-color: royalblue;
margin: 30rpx;
}
.vedio_main {
display: flex;
justify-content:space-around;
flex-direction: row;
flex-wrap: wrap;
}
.vedio_main .vedio_main_one {
background-color: cadetblue;
height: 300rpx;
width: 45%;
margin-top: 20rpx;
}
index.json
bash
{
"usingComponents": {
"van-tab": "@vant/weapp/tab/index",
"van-tabs": "@vant/weapp/tabs/index"
},
"enablePullDownRefresh": true,
"onReachBottomDistance": 0
}
index.js
javascript
Page({
data: {
title_menu : [
{
title_code : 1,
title_name : "直播"
},
{
title_code : 2,
title_name : "推荐"
},
{
title_code : 3,
title_name : "热门"
},{
title_code : 4,
title_name : "动画"
},{
title_code : 5,
title_name : "动画"
},{
title_code : 6,
title_name : "动画"
},{
title_code : 7,
title_name : "动画"
},{
title_code : 9,
title_name : "动画"
},{
title_code : 10,
title_name : "动画"
},{
title_code : 11,
title_name : "动画"
}
],
active : 2,
top: "100rpx",
},
onPullDownRefresh() {
console.log('触发了上拉刷新事件')
this.setData({
top:"100rpx"
})
},
onReachBottom() {
if(this.data.top !== 0) {
this.setData({
top:0
})
console.log("触底事件触发")
}
},
})
app.json 主配置文、包含一些tabbar(最下面的标签)、新增的一些页面
标签获取网站 https://www.iconfont.cn/
bash
{
"pages": [
"pages/index/index",
"pages/dynamic/dynamic",
"pages/publish/publish",
"pages/vip_shop/vip_shop",
"pages/mine/mine",
"pages/logs/logs"
],
"window": {
"navigationBarTextStyle": "black",
"navigationBarTitleText": "Weixin",
"navigationBarBackgroundColor": "#ffffff"
},
"componentFramework": "glass-easel",
"sitemapLocation": "sitemap.json",
"lazyCodeLoading": "requiredComponents",
"usingComponents": {
},"tabBar": {
"list": [{
"pagePath": "pages/index/index",
"text": "首页",
"iconPath": "./image/HOME.png",
"selectedIconPath": "./image/home_seleced.png"
},{
"pagePath": "pages/dynamic/dynamic",
"text": "动态",
"iconPath": "./image/miss_page_icon/fengche.png",
"selectedIconPath": "./image/selected_page_icon/fengche.png"
},{
"pagePath": "pages/publish/publish",
"text": "发布",
"iconPath": "./image/miss_page_icon/publish.png",
"selectedIconPath": "./image/selected_page_icon/publish.png"
},{
"pagePath": "pages/vip_shop/vip_shop",
"text": "会员购",
"iconPath": "./image/miss_page_icon/gouwubao.png",
"selectedIconPath": "./image/selected_page_icon/gouwubao.png"
},{
"pagePath": "pages/mine/mine",
"text": "我的",
"iconPath": "./image/miss_page_icon/dianshi.png",
"selectedIconPath": "./image/selected_page_icon/dianshi.png"
}]
}
}
4.1.1.4 后端服务搭建
4.1.1.4.1 deploy 服务 部署配置信息
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<artifactId>deploy</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.24</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.18</version>
</dependency>
</dependencies>
<parent>
<artifactId>blMan</artifactId>
<groupId>com.bu</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
</project>
XML
spring:
application:
# 注册中心 微服务名称
name: deployApp
datasource:
url: jdbc:mysql://localhost:3306/自己新建的库?serveTimezone=GMT+8
password: 密码
username: 用户名
driver-class-name: com.mysql.cj.jdbc.Driver
cloud:
nacos:
discovery:
server-addr: http://localhost:8848
mybatis:
mapper-locations: classpath:/mapper/**.xml
server:
port: 8992
项目地址: 地址