自定义过滤工厂类
DemoGatewayFilterFactory
java
package com.learning.springcloud.custom;
import org.apache.commons.lang.StringUtils;
import org.springframework.cloud.gateway.filter.GatewayFilter;
import org.springframework.cloud.gateway.filter.GatewayFilterChain;
import org.springframework.cloud.gateway.filter.factory.AbstractGatewayFilterFactory;
import org.springframework.http.HttpStatus;
import org.springframework.stereotype.Component;
import org.springframework.web.server.ServerWebExchange;
import reactor.core.publisher.Mono;
import java.util.Arrays;
import java.util.List;
@Component
public class DemoGatewayFilterFactory extends AbstractGatewayFilterFactory<DemoGatewayFilterFactory.Config> {
public DemoGatewayFilterFactory() {
super(Config.class);
}
public List<String> shortcutFieldOrder() {
return Arrays.asList("name", "value");
}
@Override
public GatewayFilter apply(DemoGatewayFilterFactory.Config config) {
return new GatewayFilter() {
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
String value = exchange.getRequest().getQueryParams().getFirst("name");
if (StringUtils.isBlank(value)) {
// 为空 则证明是正常的请求 需要放行
return chain.filter(exchange);
}
if ("YES".equals(value)) {
return chain.filter(exchange);
}
exchange.getResponse().setStatusCode(HttpStatus.NOT_FOUND);
return exchange.getResponse().setComplete();
}
};
}
public static class Config {
private String name;
private String value;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getValue() {
return value;
}
public void setValue(String name) {
this.value = name;
}
}
}
过滤器配置说明
java
server:
port: 8088
spring:
application:
name: api-gateway
cloud:
nacos:
discovery:
server-addr: 127.0.0.1:8847
username: nacos
password: nacos
gateway:
routes:
- id: order_route # 路由唯一标识
#uri: http://localhost:8020 # 需要转发的地址
uri: lb://order-service # 需要转发的地址
# 断言规则 用于路由规则的匹配
predicates:
# - Path=/order-serv/**
# - After=2024-01-01T23:00:11.518+08:00[Asia/Shanghai]
# - Before=2025-01-01T23:00:11.518+08:00[Asia/Shanghai]
- Between=2024-01-01T23:00:11.518+08:00[Asia/Shanghai],2025-01-01T23:00:11.518+08:00[Asia/Shanghai]
# - Header=X-Request-Id,\d+
# - Host=127.0.0.1
# - Query=name,lq
# - Method=GET,POST
# http://localhost:8088/order-serv/order/add => http://localhost:8020/order-serv/order/add
- Demo=YES
#配置过滤器工厂
filters:
- StripPrefix=1 # 转发去掉第一层路径
- AddRequestHeader=X-Request-name,tom #添加请求头
- AddRequestParameter=color, blue # 添加请求参数
- PrefixPath=/demo
# - RedirectTo=302, https://www.baidu.com/ #重定向到百度
- Demo=name,YES # 自定义过滤工厂配置
# http://localhost:8020/order-serv/order/add => http://localhost:8020/order/add
访问效果
- 不带name参数的请求放行
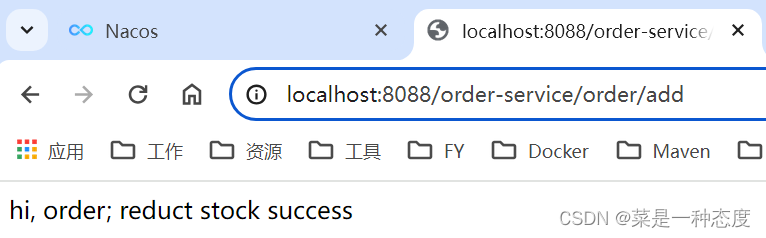
- 带name参数值不是YES的拦截,返回 not found
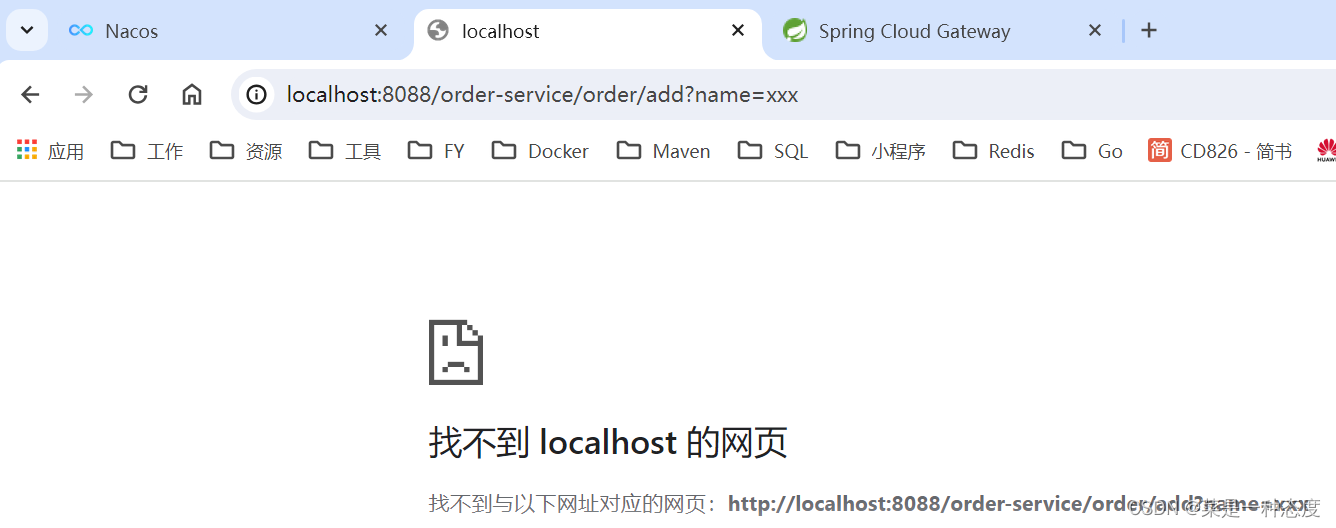
- 带name参数值是YES的放行
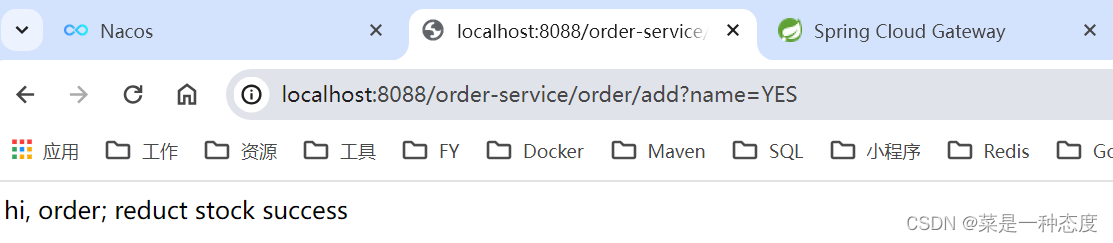
实现说明
- 命名必须需要以 FilterFactory 结尾(约定规范)
- 继承 AbstractGatewayFilterFactory 类
- 必须为spring的组件bean(@Component)
- 必须要有内部类 Config 以及 对应的 shortcutFieldOrder 方法
- 重写 apply 方法的逻辑 (apply(DemoGatewayFilterFactory.Config config))
- 可通过 exchange.getRequest() 获取到 ServerHttpRequest 对象
- 从而获取到请求的参数、请求方式、请求头等信息