目录
[2. 字符转换函数1.字符分类函数](#2. 字符转换函数1.字符分类函数)
[2. 字符转换函数](#2. 字符转换函数)
接下来介绍部分字符函数测试
2. 字符转换函数
1.字符分类函数
1.1iscntrl
注:任何控制字符 检查是否有控制字符 符合为真
cs
int main()
{
int i = 0;
char str[] = "first line \n second line \n";
//判断是否遇到控制字符
while (!iscntrl(str[i]))
{
putchar(str[i]);//putchar输出一个字符
i++;
}
return 0;
}
结果
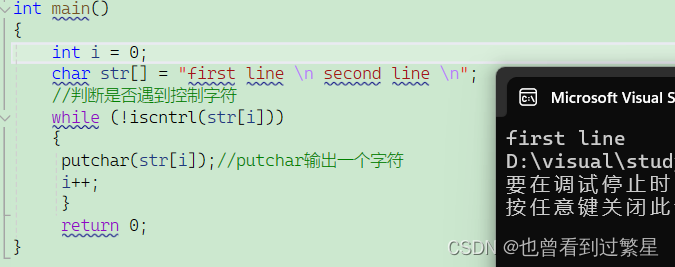
1.2isspace
cs
isspace 检查是否为 空白字符 其中空白字符包含 ' ' , '\t' , '\n' , '\v', '\f', '\r'
// 空格 制表符 换行 垂直制表符 换页 回车
cs
int main()
{
char c;
int i = 0;
char str[] = "Example sentence to test isspace\n";
while (str[i])
{
c = str[i];
if (isspace(c))//
{
c = '\n';
}
putchar(c);//putchar输出一个字符
i++;
}
return 0;
}
结果:
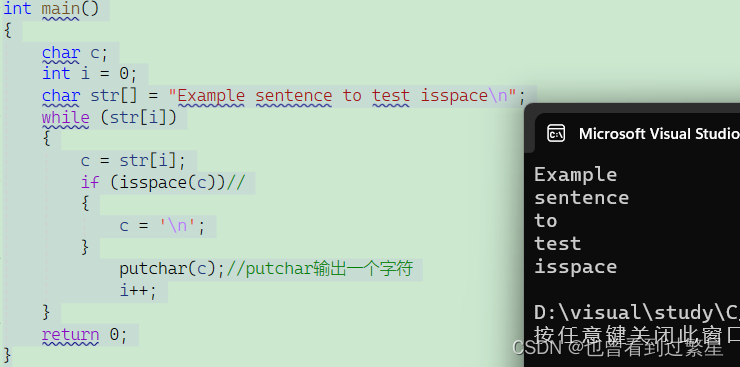
1.3isdigit
检查是否为十进制的数(整数) 0~9
cs
#include <ctype.h>
int main()
{
char str[] = "1776ad";
int year;
if (isdigit(str[0]))
{
year = atoi(str); //atoi 将字符串转为数字 赋值的类型为整型
printf("The year that followed %d was %d.\n", year, year + 1);
}
return 0;
}
结果:
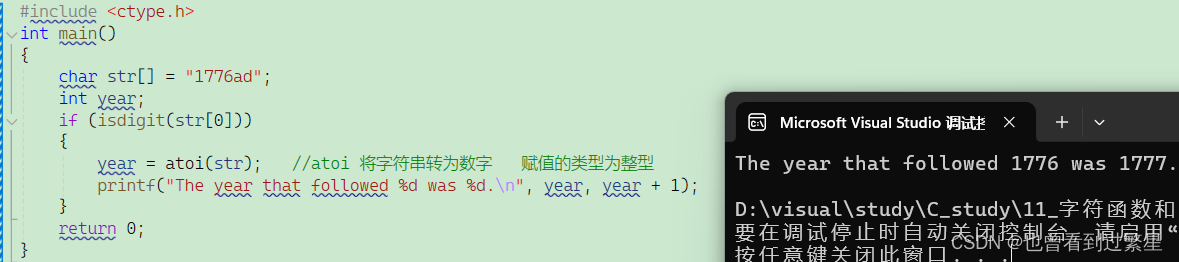
1.4isxdigit
检查是否为十六进制数(int) :0 1 2 3 4 5 6 7 8 9 a b c d e f A B C D E F
cs
int main()
{
char str[] = "ffff";
long int number;
if (isxdigit(str[0]))
{
number = strtol(str, NULL, 16); //strtol 字符串转换为长整型
printf("The hexadecimal number %lx is %ld.\n", number, number);
}
return 0;
}
结果:
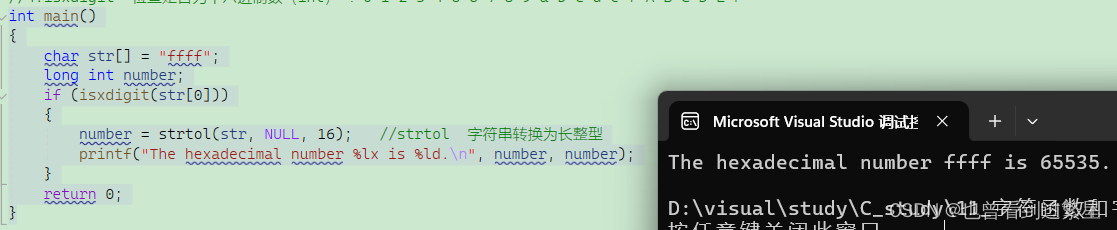
1.5islower
检查是否为小写字母(int):a b c d e f g h i j k l m n o p q r s t u v w x y z.
将字符串中的小写字母转大写,其他字符不变。
cs
int main()
{
int i = 0;
char str[] = "test String\n";
char c;
while (str[i])
{
c = str[i];
if (islower(c))
{
c -= 32;
}
putchar(c);
i++;
}
return 0;
}
结果:
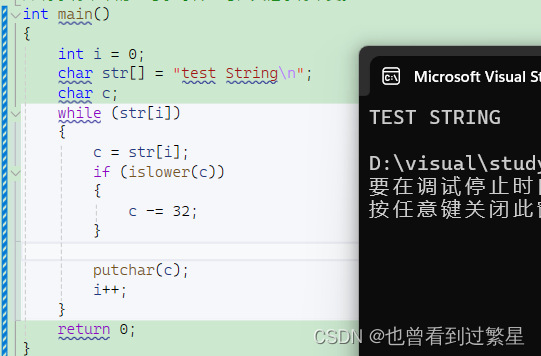
1.6isupper
检查是否为大写字母(int)A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
将字符串中的大写字⺟转小写,其他字符不变。
cs
int main()
{
int i = 0;
char str[] = "Test String.\n";
char c;
while (str[i])
{
c = str[i];
if (isupper(c)) c = tolower(c);
putchar(c);
i++;
}
return 0;
}
结果:
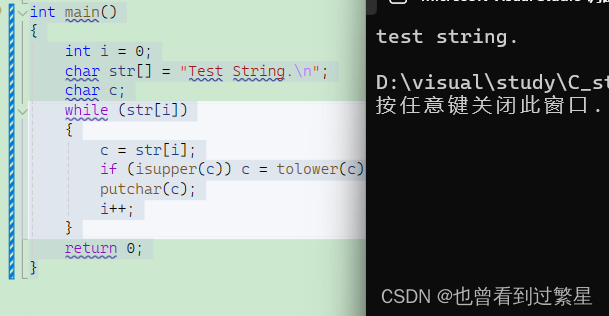
1.7isalnum
检查是否为字母数字
cs
int main()
{
int i;
char str[] = "c3po...";
i = 0;
while (isalnum(str[i]))
{
i++;
}
printf("The first %d characters are alphanumeric\n", i);
return 0;
}
结果:
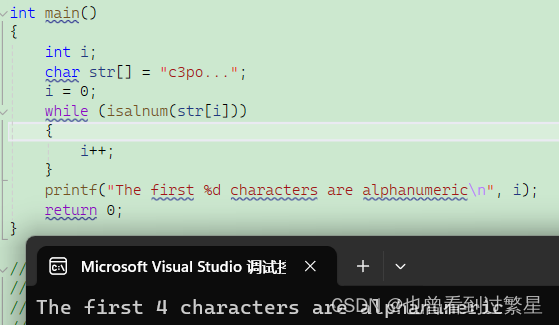
1.8ispunct
检查是否为标点符号 标点符号,任何不属于数字或者字母的图形字符(可打印)
cs
int main()
{
int i = 0;
int cx = 0;
char str[] = "Hello, welcome!";
while (str[i])
{
if (ispunct(str[i])) cx++;
i++;
}
printf("Sentence contains %d punctuation characters.\n", cx);
return 0;
}
结果:
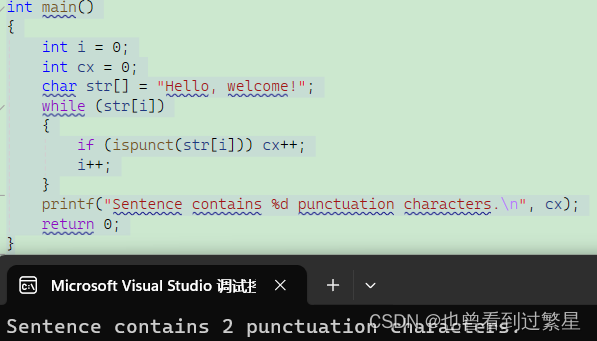
1.9isprint
检查字符是否可打印
cs
int main()
{
int i = 0;
char str[] = "first line \n second line \n";
while (isprint(str[i]))
{
putchar(str[i]);
i++;
}
return 0;
}
结果:
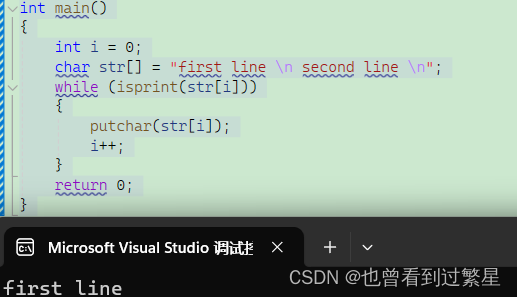
2. 字符转换函数
cs
int tolower ( int c ); //将参数传进去的⼤写字⺟转⼩写
int toupper ( int c ); //将参数传进去的⼩写字⺟转⼤写
cs
#include <stdio.h>
#include <ctype.h>
int main ()
{
int i = 0;
char str[] = "Test String.\n";
char c;
while (str[i])
{
c = str[i];
if (islower(c))
c = toupper(c);
putchar(c);
i++;
}
return 0;
}
结果:
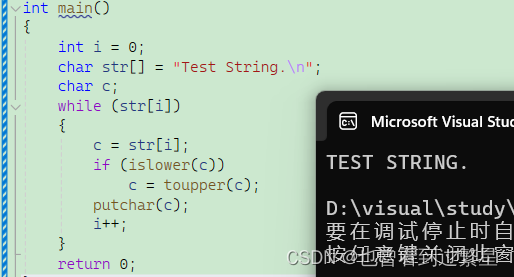