在andorid中,我们可以写两个页面,LoginActivity页面和RegisterActivity页面,然后把两个页面关联起来就可以实现登录和注册的切换了,但是这样的页面看起来不是很美观,那么,我们如何可以把页面做的更美观一点呢,在此,我们就可以通过Fragment来实现。
我们随便做一个登录页面来作为例子
由于我们注重于fragment的编写,所以我们的页面ui就写的潦草一点。
在主页面写个登录页面的主程序。
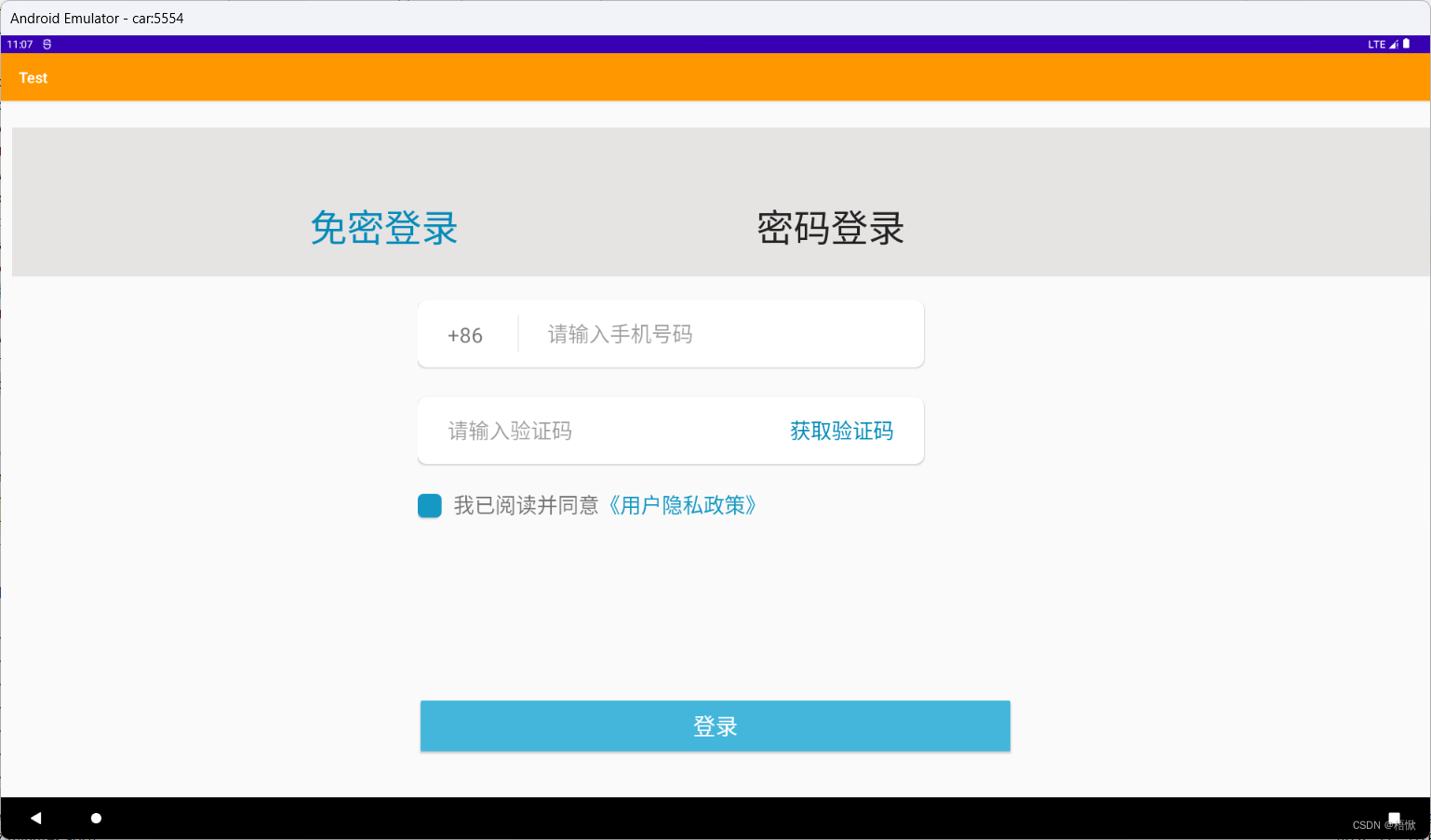
activity_main.xml
XML<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <RelativeLayout android:id="@+id/rl_tab" android:layout_width="match_parent" android:layout_height="200dp" android:layout_marginStart="15dp" android:layout_marginTop="36dp" android:background="#E6E3E3"> <RelativeLayout android:layout_width="match_parent" android:layout_height="100dp" android:layout_marginTop="100dp"> <TextView android:id="@+id/tv_no_pwd" android:layout_width="200dp" android:layout_height="100dp" android:layout_marginLeft="400dp" android:text="免密登录" android:textColor="@drawable/selector_tab" android:textSize="50dp" /> <TextView android:id="@+id/tv_account" android:layout_width="200dp" android:layout_height="100dp" android:layout_marginLeft="400dp" android:layout_toRightOf="@id/tv_no_pwd" android:text="密码登录" android:textColor="@drawable/selector_tab" android:textSize="50dp" /> </RelativeLayout> </RelativeLayout> <!--FrameLayout--> <FrameLayout android:id="@+id/fl_login" android:layout_centerInParent="true" android:layout_width="800dp" android:layout_height="400dp" android:layout_marginLeft="60dp" android:layout_marginTop="350dp" android:layout_marginRight="60dp" /> <Button android:id="@+id/btnlogin" android:layout_width="800dp" android:layout_centerHorizontal="true" android:layout_height="80dp" android:layout_marginLeft="60dp" android:layout_marginTop="800dp" android:layout_marginRight="60dp" android:backgroundTint="#44B6DC" android:gravity="center" android:text="@string/login" android:textColor="#ffffff" android:textSize="30sp" /> </RelativeLayout>
具体的ui设计可以根据自己的要求来写。
在写完界面设计后可以写主页面的点击事件等。
MainActivity.java
javapackage njitt.software.test; import androidx.appcompat.app.AppCompatActivity; import androidx.cardview.widget.CardView; import androidx.fragment.app.FragmentManager; import androidx.fragment.app.FragmentTransaction; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.CheckBox; import android.widget.FrameLayout; import android.widget.RelativeLayout; import android.widget.TextView; import java.util.HashMap; import njitt.software.test.fragment.LoginFragment; import njitt.software.test.fragment.NopwdFragment; public class MainActivity extends AppCompatActivity implements View.OnClickListener{ private HashMap<String, Object> map; private CheckBox cvCheckLogin; private TextView registerUserAgreement; private RelativeLayout rlMain; private RelativeLayout rlBack; private CardView cvAccountLogin; private RelativeLayout rlLogin; private TextView tvPhoneTakeIn; private TextView tvRegister; private TextView tv_no_pwd; private TextView tv_account; private FrameLayout flLogin; private Button btnlogin; private NopwdFragment nopwdFragment; private LoginFragment loginFragment; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initView(); tv_no_pwd.performClick(); } private void initView() { flLogin = (FrameLayout) findViewById(R.id.fl_login); rlMain = (RelativeLayout) findViewById(R.id.rl_main); cvAccountLogin = (CardView) findViewById(R.id.cv_account_login); rlLogin = (RelativeLayout) findViewById(R.id.rl_login); flLogin = (FrameLayout) findViewById(R.id.fl_login); btnlogin = (Button) findViewById(R.id.btnlogin); flLogin = (FrameLayout) findViewById(R.id.fl_login); tv_no_pwd = (TextView) findViewById(R.id.tv_no_pwd); tv_account = (TextView)findViewById(R.id.tv_account); tv_no_pwd.setOnClickListener(this); tv_account.setOnClickListener(this); } private void setSelected() { tv_no_pwd.setSelected(false); tv_account.setSelected(false); } @Override public void onClick(View view) { FragmentManager fragmentManager = getSupportFragmentManager(); FragmentTransaction transaction = fragmentManager .beginTransaction(); hideAllFragment(transaction); switch (view.getId()) { case R.id.tv_no_pwd: setSelected(); tv_no_pwd.setSelected(true); tv_account.setSelected(false); if (nopwdFragment == null) { nopwdFragment = new NopwdFragment(); transaction.add(R.id.fl_login, nopwdFragment); } else { transaction.show(nopwdFragment); } break; case R.id.tv_account: setSelected(); tv_account.setSelected(true); tv_no_pwd.setSelected(false); if (loginFragment == null) { loginFragment = new LoginFragment(); transaction.add(R.id.fl_login, loginFragment); } else { transaction.show(loginFragment); } break; default: break; } transaction.commit(); } private void hideAllFragment(FragmentTransaction transaction) { if (nopwdFragment != null) { transaction.hide(nopwdFragment); } if (loginFragment != null) { transaction.hide(loginFragment); } } }
这是定义文字的默认选中为false。
定义一个fragmentManager管理器

是否隐藏页面。
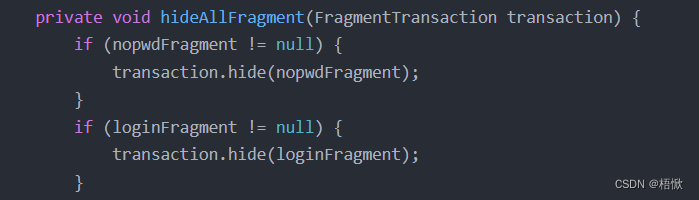
写一个fragment包
在包里写两个fragment页面。
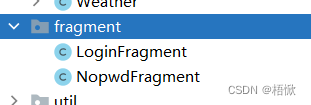
fragment_login.xml
XML<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".fragment.LoginFragment"> <androidx.cardview.widget.CardView android:layout_width="680dp" android:layout_height="90dp" > <TextView android:layout_width="54dp" android:layout_height="37dp" android:text="+86" android:textSize="28dp" android:layout_marginLeft="40dp" android:layout_marginTop="27dp" /> <View android:layout_width="2dp" android:layout_height="50dp" android:background="#ECEFF1" android:layout_marginLeft="134dp" android:layout_marginTop="20dp" /> <EditText android:id="@+id/et_account" android:layout_width="546dp" android:layout_height="match_parent" android:layout_marginLeft="174dp" android:background="@null" android:hint="请输入手机号码" android:textSize="28dp"/> </androidx.cardview.widget.CardView> <androidx.cardview.widget.CardView android:layout_width="680dp" android:layout_height="90dp" android:layout_marginTop="130dp"> <EditText android:id="@+id/et_password" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_marginLeft="40dp" android:background="@null" android:hint="请输入密码" android:textSize="28dp" android:password="true"/> </androidx.cardview.widget.CardView> <androidx.cardview.widget.CardView android:id="@+id/cv_check" android:layout_width="32dp" android:layout_height="32dp" android:layout_marginTop="260dp" > </androidx.cardview.widget.CardView> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:layout_width="wrap_content" android:layout_height="37dp" android:text="我已阅读并同意" android:layout_marginTop="256dp" android:layout_marginLeft="48dp" android:textSize="28dp" /> <TextView android:id="@+id/tv_user_secret" android:layout_width="wrap_content" android:layout_height="37dp" android:text="《用户隐私政策》" android:layout_marginTop="256dp" android:textSize="28dp" android:textColor="#1598C4"/> </LinearLayout> <TextView android:id="@+id/tv_forget" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="540dp" android:text="忘记密码?" android:textColor="#1598C4" android:textSize="28dp" android:onClick="to" android:layout_marginTop="256dp" /> </FrameLayout>
因为我们只写fragment页面,所以我们就不写点击事件了。
fragment_nopwd.xml
XML<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".fragment.NopwdFragment"> <androidx.cardview.widget.CardView android:layout_width="680dp" android:layout_height="90dp" app:cardCornerRadius="12dp"> <TextView android:layout_width="54dp" android:layout_height="37dp" android:text="+86" android:textSize="28dp" android:layout_marginLeft="40dp" android:layout_marginTop="27dp" /> <View android:layout_width="2dp" android:layout_height="50dp" android:background="#ECEFF1" android:layout_marginLeft="134dp" android:layout_marginTop="20dp" /> <EditText android:id="@+id/et_phone_number" android:layout_width="546dp" android:layout_height="match_parent" android:layout_marginLeft="174dp" android:background="@null" android:hint="请输入手机号码" android:textSize="28dp"/> </androidx.cardview.widget.CardView> <androidx.cardview.widget.CardView android:layout_width="680dp" android:layout_height="90dp" app:cardCornerRadius="12dp" android:layout_marginTop="130dp"> <EditText android:id="@+id/et_verify" android:layout_width="500dp" android:layout_height="match_parent" android:layout_marginLeft="40dp" android:background="@null" android:hint="请输入验证码" android:textSize="28dp"/> <TextView android:id="@+id/tv_get_verification_code" android:layout_width="140dp" android:layout_height="37dp" android:layout_marginLeft="500dp" android:layout_marginTop="26dp" android:text="获取验证码" android:textSize="28dp" android:textColor="#038BB9" /> </androidx.cardview.widget.CardView> <androidx.cardview.widget.CardView android:id="@+id/cv_check" android:layout_width="32dp" android:layout_height="32dp" android:layout_marginTop="260dp" app:cardCornerRadius="8dp" app:cardBackgroundColor="#1598C4" > </androidx.cardview.widget.CardView> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:layout_width="wrap_content" android:layout_height="37dp" android:text="我已阅读并同意" android:layout_marginTop="256dp" android:layout_marginLeft="48dp" android:textSize="28dp" /> <TextView android:id="@+id/tv_user_secret" android:layout_width="wrap_content" android:layout_height="37dp" android:text="《用户隐私政策》" android:layout_marginTop="256dp" android:textSize="28dp" android:textColor="#1598C4"/> </LinearLayout> </FrameLayout>
这样,我们就完成了两个fragment页面的ui设计。我们来看一下效果。
1
这样,我们的fragment切换页面就完成了。