目的:
设计一个简单的汽车租赁系统,包含以下功能:
- 添加车辆:用户可以添加新的车辆到系统中,包括车辆的品牌、型号、车牌号、日租金等信息。
- 查找车辆:用户可以通过车牌号或者品牌来查找车辆,并显示该车辆的租赁信息。
- 租车:用户可以根据车辆的品牌和租赁天数来租车,系统会计算租车的费用。
- 归还车辆:用户可以归还已租赁的车辆,系统会更新车辆的租赁状态并计算租金。
分析:
- 使用面向对象的方式实现,包括至少两个类(例如 Car 和 CarRentalSystem)。
- 使用合适的数据结构(例如列表)来存储车辆信息和租赁记录。
- 提供命令行界面,不需要图形用户界面。
实现:
car类:
using System;
public class Car
{
public string Brand { get; set; }
public string Model { get; set; }
public string LicensePlate { get; set; }
public decimal DailyRent { get; set; }
public bool IsRented { get; set; }
public Car(string brand, string model, string licensePlate, decimal dailyRent)
{
Brand = brand;
Model = model;
LicensePlate = licensePlate;
DailyRent = dailyRent;
IsRented = false;
}
public override string ToString()
{
return $"Brand: {Brand}, Model: {Model}, License Plate: {LicensePlate}, Daily Rent: {DailyRent:C}, Status: {(IsRented ? "Rented" : "Available")}";
}
}
CarRentalSystem 类:
using System;
using System.Collections.Generic;
using System.Linq;
public class CarRentalSystem
{
private List<Car> cars;
public CarRentalSystem()
{
cars = new List<Car>();
}
public void AddCar(string brand, string model, string licensePlate, decimal dailyRent)
{
cars.Add(new Car(brand, model, licensePlate, dailyRent));
Console.WriteLine("Car added successfully.");
}
public void FindCar(string searchCriteria)
{
var foundCars = cars.Where(car => car.LicensePlate.Contains(searchCriteria) || car.Brand.Contains(searchCriteria)).ToList();
if (foundCars.Count > 0)
{
foreach (var car in foundCars)
{
Console.WriteLine(car);
}
}
else
{
Console.WriteLine("No cars found.");
}
}
public void RentCar(string brand, int days)
{
var availableCar = cars.FirstOrDefault(car => car.Brand == brand && !car.IsRented);
if (availableCar != null)
{
availableCar.IsRented = true;
decimal totalCost = availableCar.DailyRent * days;
Console.WriteLine($"Car rented successfully. Total cost: {totalCost:C}");
}
else
{
Console.WriteLine("No available cars found for the specified brand.");
}
}
public void ReturnCar(string licensePlate)
{
var rentedCar = cars.FirstOrDefault(car => car.LicensePlate == licensePlate && car.IsRented);
if (rentedCar != null)
{
rentedCar.IsRented = false;
Console.WriteLine("Car returned successfully.");
}
else
{
Console.WriteLine("Car not found or already returned.");
}
}
}
主程序:
using System;
public class Program
{
public static void Main()
{
CarRentalSystem carRentalSystem = new CarRentalSystem();
while (true)
{
Console.WriteLine("\nCar Rental System");
Console.WriteLine("1. Add Car");
Console.WriteLine("2. Find Car");
Console.WriteLine("3. Rent Car");
Console.WriteLine("4. Return Car");
Console.WriteLine("5. Exit");
Console.Write("Enter your choice: ");
string choice = Console.ReadLine();
switch (choice)
{
case "1":
Console.Write("Enter brand: ");
string brand = Console.ReadLine();
Console.Write("Enter model: ");
string model = Console.ReadLine();
Console.Write("Enter license plate: ");
string licensePlate = Console.ReadLine();
Console.Write("Enter daily rent: ");
decimal dailyRent = decimal.Parse(Console.ReadLine());
carRentalSystem.AddCar(brand, model, licensePlate, dailyRent);
break;
case "2":
Console.Write("Enter license plate or brand to search: ");
string searchCriteria = Console.ReadLine();
carRentalSystem.FindCar(searchCriteria);
break;
case "3":
Console.Write("Enter brand to rent: ");
string rentBrand = Console.ReadLine();
Console.Write("Enter number of days: ");
int days = int.Parse(Console.ReadLine());
carRentalSystem.RentCar(rentBrand, days);
break;
case "4":
Console.Write("Enter license plate to return: ");
string returnLicensePlate = Console.ReadLine();
carRentalSystem.ReturnCar(returnLicensePlate);
break;
case "5":
return;
default:
Console.WriteLine("Invalid choice. Please try again.");
break;
}
}
}
}
完整代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace windows期末text1
{
public class Car
{
public string Brand { get; set; }
public string Model { get; set; }
public string LicensePlate { get; set; }
public decimal DailyRent { get; set; }
public bool IsRented { get; set; }
public Car(string brand, string model, string licensePlate, decimal dailyRent)
{
Brand = brand;
Model = model;
LicensePlate = licensePlate;
DailyRent = dailyRent;
IsRented = false;
}
public override string ToString()
{
return $"Brand: {Brand}, Model: {Model}, License Plate: {LicensePlate}, Daily Rent: {DailyRent:C}, Status: {(IsRented ? "Rented" : "Available")}";
}
}
public class CarRentalSystem
{
private List<Car> cars;
public CarRentalSystem()
{
cars = new List<Car>();
}
public void AddCar(string brand, string model, string licensePlate, decimal dailyRent)
{
cars.Add(new Car(brand, model, licensePlate, dailyRent));
Console.WriteLine("Car added successfully.");
}
public void FindCar(string searchCriteria)
{
var foundCars = cars.Where(car => car.LicensePlate.Contains(searchCriteria) || car.Brand.Contains(searchCriteria)).ToList();
if (foundCars.Count > 0)
{
foreach (var car in foundCars)
{
Console.WriteLine(car);
}
}
else
{
Console.WriteLine("No cars found.");
}
}
public void RentCar(string brand, int days)
{
var availableCar = cars.FirstOrDefault(car => car.Brand == brand && !car.IsRented);
if (availableCar != null)
{
availableCar.IsRented = true;
decimal totalCost = availableCar.DailyRent * days;
Console.WriteLine($"Car rented successfully. Total cost: {totalCost:C}");
}
else
{
Console.WriteLine("No available cars found for the specified brand.");
}
}
public void ReturnCar(string licensePlate)
{
var rentedCar = cars.FirstOrDefault(car => car.LicensePlate == licensePlate && car.IsRented);
if (rentedCar != null)
{
rentedCar.IsRented = false;
Console.WriteLine("Car returned successfully.");
}
else
{
Console.WriteLine("Car not found or already returned.");
}
}
}
internal class Program
{
static void Main(string[] args)
{
CarRentalSystem carRentalSystem = new CarRentalSystem();
while (true)
{
Console.WriteLine("\nCar Rental System");
Console.WriteLine("1. Add Car");
Console.WriteLine("2. Find Car");
Console.WriteLine("3. Rent Car");
Console.WriteLine("4. Return Car");
Console.WriteLine("5. Exit");
Console.Write("Enter your choice: ");
string choice = Console.ReadLine();
switch (choice)
{
case "1":
Console.Write("Enter brand: ");
string brand = Console.ReadLine();
Console.Write("Enter model: ");
string model = Console.ReadLine();
Console.Write("Enter license plate: ");
string licensePlate = Console.ReadLine();
Console.Write("Enter daily rent: ");
decimal dailyRent = decimal.Parse(Console.ReadLine());
carRentalSystem.AddCar(brand, model, licensePlate, dailyRent);
break;
case "2":
Console.Write("Enter license plate or brand to search: ");
string searchCriteria = Console.ReadLine();
carRentalSystem.FindCar(searchCriteria);
break;
case "3":
Console.Write("Enter brand to rent: ");
string rentBrand = Console.ReadLine();
Console.Write("Enter number of days: ");
int days = int.Parse(Console.ReadLine());
carRentalSystem.RentCar(rentBrand, days);
break;
case "4":
Console.Write("Enter license plate to return: ");
string returnLicensePlate = Console.ReadLine();
carRentalSystem.ReturnCar(returnLicensePlate);
break;
case "5":
return;
default:
Console.WriteLine("Invalid choice. Please try again.");
break;
}
}
}
}
}
实现结果:
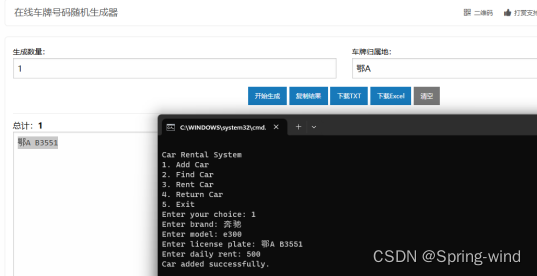
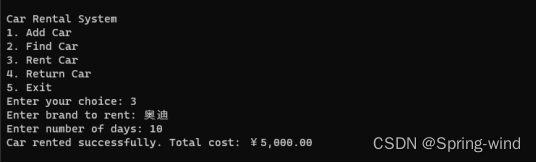
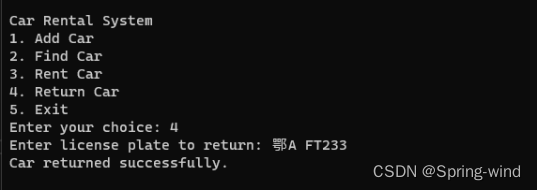
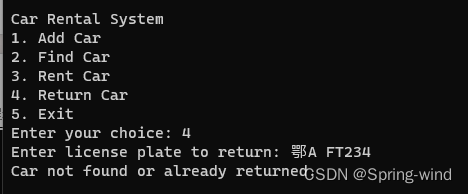
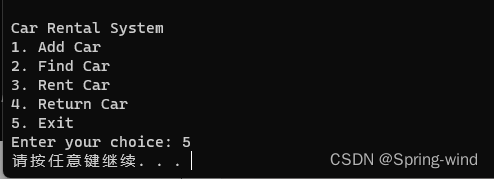
以上就是完整的代码及实现。
实验小结
通过此次实验,掌握了以下知识和技能:
- 面向对象编程的基本概念,包括类和对象的使用。
- 使用列表存储和管理数据。
- 实现类的方法以完成特定功能。
- 设计和实现简单的命令行用户界面。
- 编写和调试C#程序。
重难点分析
重点
-
面向对象编程概念
- 理解类和对象的概念,以及如何定义类的属性和方法。
- 掌握构造函数的使用,初始化对象时设置初始值。
-
列表数据结构
- 使用列表存储多个对象。
- 熟练掌握列表的添加、查找和遍历操作。
-
方法的实现
- 编写方法实现特定功能,例如添加车辆、查找车辆、租车和归还车辆。
- 在方法中处理数据并进行必要的计算,例如租车费用的计算。
难点
-
查找和筛选
- 如何高效地在列表中查找符合特定条件的对象。
- 使用
LINQ
提高查找和筛选操作的效率和代码可读性。
-
状态管理
- 正确管理车辆的租赁状态,避免出现状态不一致的情况。
- 归还车辆时,确保车辆状态更新正确。
-
用户输入处理
- 处理用户输入的有效性,例如租赁天数应为正整数,日租金应为正数。
- 提供适当的错误提示和输入提示,提高用户体验。
实验报告
实验名称
汽车租赁系统设计与实现
实验目的
通过设计和实现一个简单的汽车租赁系统,掌握C#面向对象编程的基本知识,熟悉类和对象的使用、列表数据结构的操作,以及简单的命令行界面设计。
实验内容
- 设计并实现
Car
类,用于表示车辆的基本信息。 - 设计并实现
CarRentalSystem
类,用于管理车辆的添加、查找、租赁和归还功能。 - 实现一个简单的命令行界面,让用户可以与系统进行交互。
实验环境
- 操作系统:Windows 11
- 编程语言:C#
- 开发工具:Visual Studio 2022
实验步骤
-
设计
Car
类- 创建一个新类
Car
,包含品牌、型号、车牌号、日租金和租赁状态等属性。 - 实现
Car
类的构造函数,用于初始化车辆信息。 - 重写
ToString
方法,用于输出车辆信息。
- 创建一个新类
-
设计
CarRentalSystem
类- 创建一个新类
CarRentalSystem
,使用列表存储车辆信息。 - 实现添加车辆、查找车辆、租车和归还车辆的方法。
- 在添加车辆方法中,将新车辆添加到列表中。
- 在查找车辆方法中,根据车牌号或品牌查找并输出车辆信息。
- 在租车方法中,根据品牌查找可用车辆并计算租赁费用。
- 在归还车辆方法中,根据车牌号查找已租赁的车辆并更新其租赁状态。
- 创建一个新类
-
实现命令行界面
- 编写主程序,通过命令行界面与用户进行交互。
- 提供菜单选项,让用户可以选择不同的操作。
- 根据用户的选择调用相应的方法,完成相应的功能。