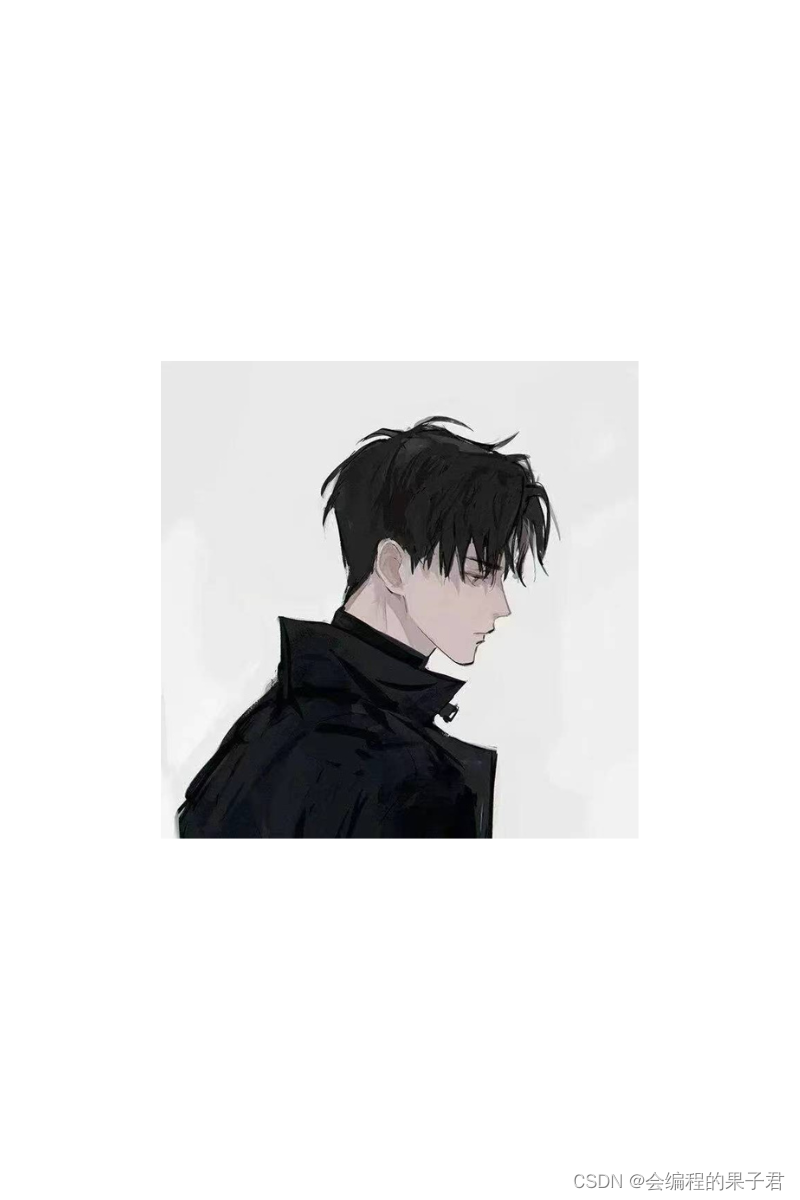
🌈个人主页:羽晨同学
💫个人格言:"成为自己未来的主人~"

string是用于字符串,可以增删改查
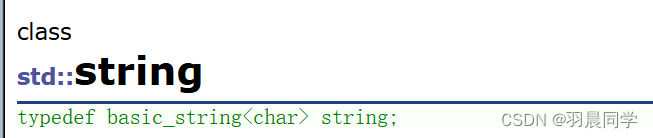
首先,我们来看一下string的底层

接下来,我们来看一下string的常用接口有哪些:
cpp
#define _CRT_SECURE_NO_WARNINGS
#include<iostream>
#include<string>
#include<list>
#include<algorithm>
using namespace std;
void test_string1()
{
//常用
string s1;
string s2("hello world");
string s3(s2);
cout << s3;
}
int main()
{
test_string1();
return 0;
}

我们可以看到的是,string起到了拷贝字符串的作用。
接下来是一些不常用的接口功能:
cpp
string s4(s3, 3, 5);
cout << s4 << endl;
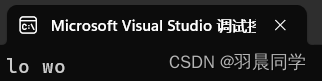
我们可以看到这个接口的功能是从下标为3开始,向后拷贝五个字节
cpp
string s5(s2, 3);
cout << s5 << endl;
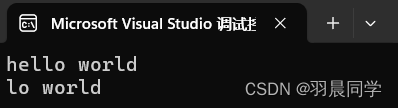
我们可以看到,这个接口的目的是从下标为3开始,一直拷贝到结尾
cpp
string s6(s2, 3, 30);
string s7("hello world", 5);
string s8(10, 'x');
cout << s6 << endl;
cout << s7 << endl;
cout << s8 << endl;
在接下来的接口6,7,8,这三个接口当中,想要实现的功能分别为
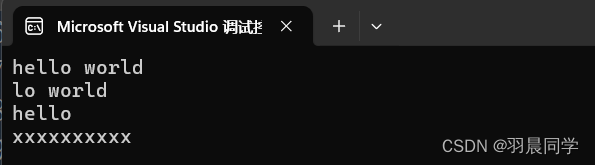
接口6是下标为3开始到30,由于30大于最大长度,所以到字符串的结尾
接口7是拷贝前5个
接口8是拷贝10个x
接下来,让我们看一下下面的这段代码:
cpp
void test_string2()
{
//隐式类型转换
string s2 = "hello world";
string& s3 = "hello world";
}
在这段代码中,涉及到的知识点为隐式类型转换,我们都知道,临时变量具有常性,所以这段代码会出现报错,报错原因是由于权限的放大。
所以,我们需要再前面加上一个const
cpp
void test_string2()
{
//隐式类型转换
string s2 = "hello world";
const string& s3 = "hello world";
}
这样子,我们就避免了权限放大的这个问题。
cpp
void push_back(const string &s)
{
}
void test_string2()
{
//隐式类型转换
string s2 = "hello world";
const string& s3 = "hello world";
//构造
string s1("hello world");
push_back(s1);
push_back("hello world");
}
在这段代码中我们可以看到,当传参的时候,我们既可以传临时变量,也可以传字符串
接下来让我们简单实现一下string中的operator[]的粗略逻辑:
cpp
class string
{
public:
//引用返回
//1.减少拷贝
//2.修改返回对象
char& operator[](size_t i)
{
assert(i < _size);
return _str[i];
}
private:
char* _str;
size_t _size;
size_t _capacity;
};
这个告诉我们一件什么事情呢?那就是在[]中是存在暴力检查的。
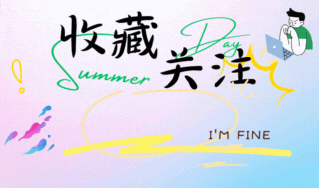
