一、0udpClient
控件:
button(打开,关闭,发送),textbox,richTextBox
打开UDP:
UdpClient udp:
cs
namespace _01udpClient
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//打开
UdpClient udp;
private void button1_Click(object sender, EventArgs e)
{
//1创建udp对象 指定ip地址和端口号
udp = new UdpClient(new IPEndPoint(IPAddress.Any, 8080));
//2 接收数据
startReceive();
}
void startReceive()
{
new Thread(() =>
{
try
{
while (true)
{
//创建ip接受客户端的ip地址
IPEndPoint ip = null;
//接收数据 返回字节数组
byte[] body = udp.Receive(ref ip);
string s = Encoding.UTF8.GetString(body);
BeginInvoke((Action)(() =>
{
richTextBox1.AppendText(ip.ToString() + ":" + s + "\t\n");
}));
}
}
catch
{
}
}).Start();
}
//关闭
private void button2_Click(object sender, EventArgs e)
{
udp.Close();//关闭
udp = null;
}
private void button3_Click(object sender, EventArgs e)
{
byte[] bs = Encoding.UTF8.GetBytes(this.textBox1.Text);
//发数据
//参数1 字节数组
//参数2 字节长度
//参数3 目标主机地址
//参数4 端口号
udp.Send(bs, bs.Length, "192.168.107.71", 8080);
}
}
}
二、udpClient组播
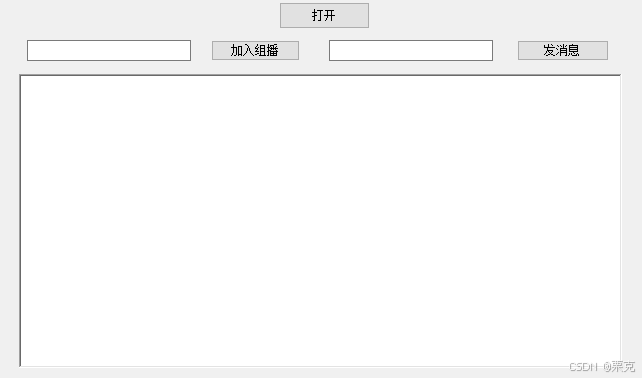
cs
namespace _02udpClinet组播
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
//打开服务器
private void button3_Click(object sender, EventArgs e)
{
udp = new UdpClient(new IPEndPoint(IPAddress.Any, 8080));
strartReceive();
}
UdpClient udp;
//异步的方式
//1 new Thread() 分线程
//2 Task.Run() 异步任务
//3 async(异步)和await (等待)
async void strartReceive()
{
while (true)
{
//await 跟一个异步的任务
// 等待异步结束之后 再去执行
//receiveAsync() 异步接收数据
UdpReceiveResult body = await udp.ReceiveAsync();
// body.RemoteEndPoint 远程终端
//body.Buffer 数据字节数组
BeginInvoke((Action)(() =>
{
richTextBox1.AppendText(body.RemoteEndPoint.ToString() + ":" + Encoding.UTF8.GetString(body.Buffer)+"\t\n");
}));
}
}
// 加入组播
private void button1_Click(object sender, EventArgs e)
{
//Join 加入
udp.JoinMulticastGroup(IPAddress.Parse(this.textBox1.Text));//加入组播地址
}
private void button2_Click(object sender, EventArgs e)
{
//发送消息
byte[] bs = Encoding.UTF8.GetBytes(this.textBox2.Text);
udp.Send(bs, bs.Length, this.textBox1.Text, 8080);
}
}
}