文章目录
- 文章介绍
- 步骤安排及单例讲解
- step1:注册单例类型(main.cpp)
- step2:定义类和私有构造函数(keyboardinputmanager.h)
- step3:(keyboardinputmanager.cpp)
- step4:在qml中调用keyboardinputmanager类(main.qml)
- 重点步骤
文章介绍
给一个单例模式的例子,实现将键盘输入数据打印到界面文本框的操作
步骤安排及单例讲解
step1:注册单例类型(main.cpp)
step2:定义类和私有构造函数(keyboardinputmanager.h)
step3:(keyboardinputmanager.cpp)
step4:在qml中调用keyboardinputmanager类(main.qml)
单例模式的优点:非常适合在 QML 中集成复杂的后端逻辑,使得前端界面可以直接调用后端逻辑的单例实例进行数据处理或业务逻辑运算。
step1:注册单例类型(main.cpp)
main.cpp中只用关注 qmlRegisterSingletonInstance("com.example", 1, 0, "KeyboardInputManager", KeyboardInputManager::instance());;
这行代码将 KeyboardInputManager注册为 QML 中的单例类型
cpp
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QQmlContext>
#include "KeyboardInputManager.h"
int main(int argc, char *argv[])
{
QGuiApplication app(argc, argv);
QQmlApplicationEngine engine;
qmlRegisterSingletonInstance("com.example", 1, 0, "KeyboardInputManager", KeyboardInputManager::instance());
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
if (engine.rootObjects().isEmpty())
return -1;
return app.exec();
}
step2:定义类和私有构造函数(keyboardinputmanager.h)
cpp
#ifndef KEYBOARDINPUTMANAGER_H
#define KEYBOARDINPUTMANAGER_H
#include <QObject>
// KeyboardInputManager 类:管理键盘输入的单例类
class KeyboardInputManager : public QObject
{
Q_OBJECT
public:
// 获取单例实例的方法
static KeyboardInputManager* instance()
{
// 静态局部变量,确保单例
static KeyboardInputManager instance;
return &instance;
}
signals:
// 按键事件信号,传递按键文本
void keyPressed(const QString &key);
protected:
// 事件过滤器方法,用于捕获和处理键盘事件
bool eventFilter(QObject *obj, QEvent *event) override;
private:
// 私有构造函数,确保单例模式
KeyboardInputManager();
// 私有析构函数
~KeyboardInputManager();
// 禁用拷贝构造函数
KeyboardInputManager(const KeyboardInputManager&) = delete;
// 禁用赋值运算符
KeyboardInputManager& operator=(const KeyboardInputManager&) = delete;
};
#endif // KEYBOARDINPUTMANAGER_H
step3:(keyboardinputmanager.cpp)
cpp
#include "KeyboardInputManager.h"
#include <QGuiApplication>
#include <QKeyEvent>
// 构造函数:安装事件过滤器以捕获键盘事件
KeyboardInputManager::KeyboardInputManager()
{
// 安装事件过滤器,将当前实例作为事件过滤器
QGuiApplication::instance()->installEventFilter(this);
}
// 析构函数:默认析构函数
KeyboardInputManager::~KeyboardInputManager() {}
// 事件过滤器:捕获并处理键盘事件
bool KeyboardInputManager::eventFilter(QObject *obj, QEvent *event)
{
// 检查事件类型是否为键盘按下事件
if (event->type() == QEvent::KeyPress) {
// 将事件转换为键盘事件
QKeyEvent *keyEvent = static_cast<QKeyEvent *>(event);
// 发射 keyPressed 信号,将按键文本传递给连接的槽函数
emit keyPressed(keyEvent->text());
// 返回 true 表示事件已处理
return true;
} else {
// 调用父类的事件过滤器处理其他类型的事件
return QObject::eventFilter(obj, event);
}
}
step4:在qml中调用keyboardinputmanager类(main.qml)
cpp
import QtQuick 2.15
import QtQuick.Controls 2.15
import QtQuick.Window 2.15
import com.example 1.0
Window {
visible: true
width: 640
height: 480
title: qsTr("Keyboard Input")
TextArea {
id: textArea
anchors.fill: parent
wrapMode: Text.Wrap
focus: true
Keys.onPressed: {
KeyboardInputManager.keyPressed(event.text)
}
}
Connections {
target: KeyboardInputManager
onKeyPressed: {
textArea.text += key
}
}
}
重点步骤
main.cpp
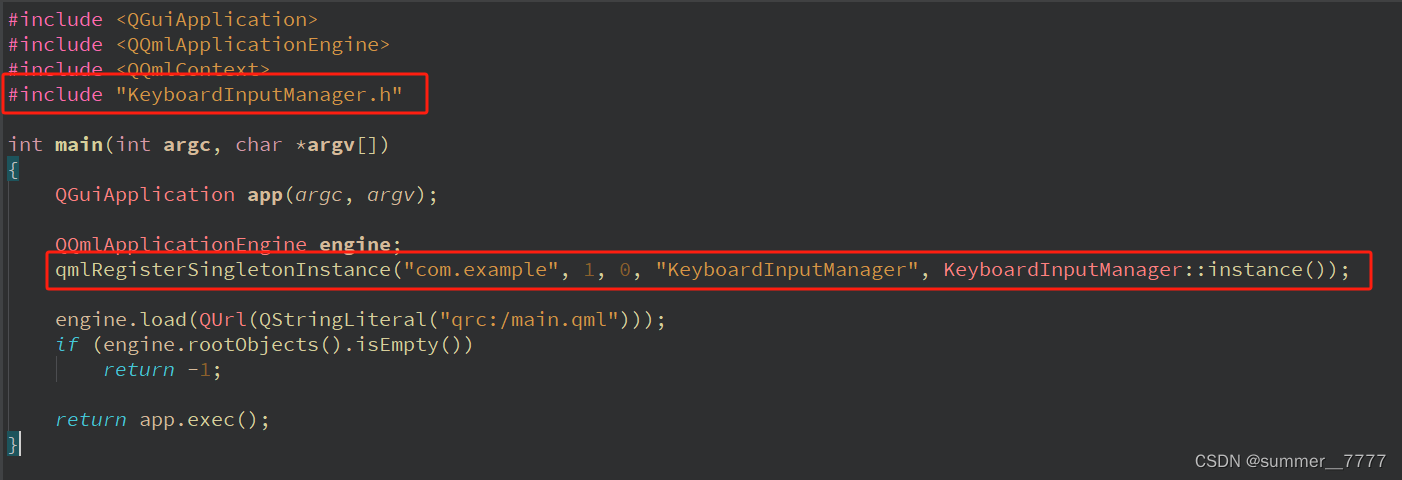
keyboardinputmanager.h
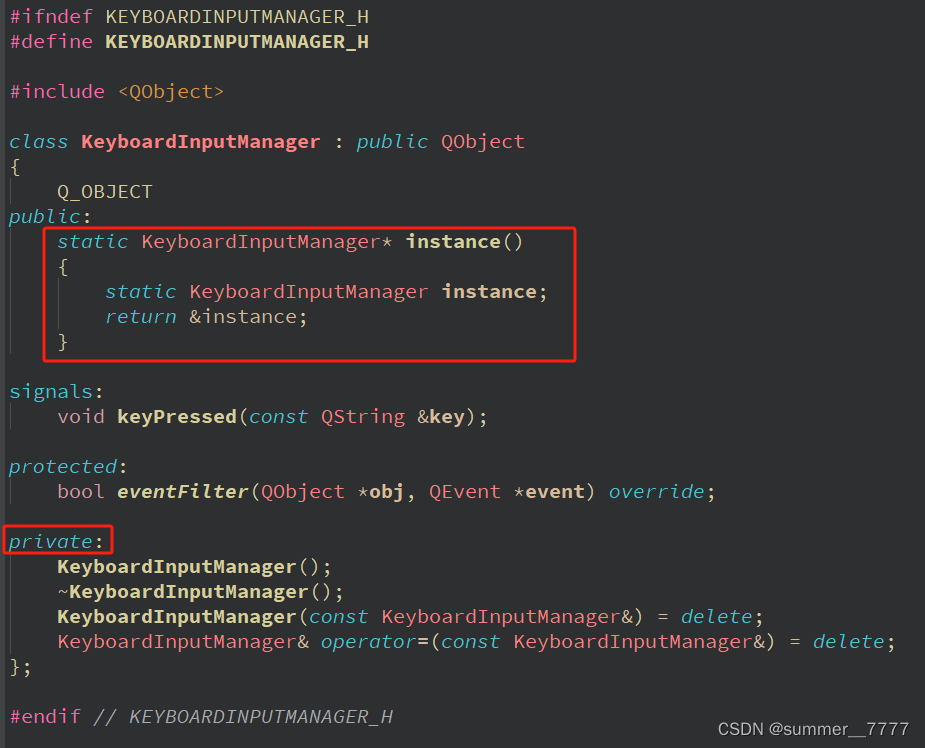
keyboardinputmanager.cpp
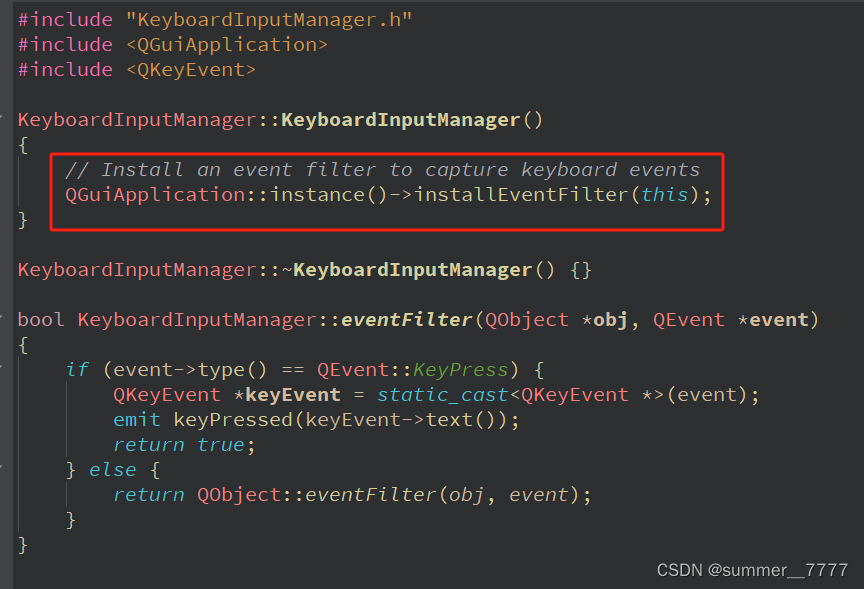
main.qml
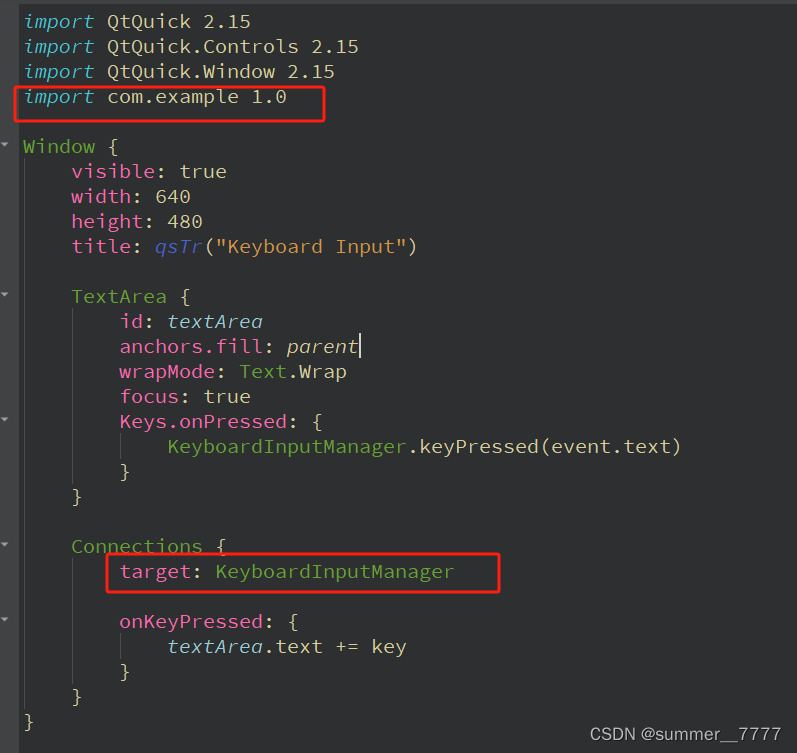