1.Teacher类下定义信号signals:
Student类下定义槽函数:
Teacher.h
#pragma once
#include <QObject>
class Teacher : public QObject
{
Q_OBJECT
public:
Teacher(QObject *parent);
~Teacher();
signals:
void Ask(); //老师向学生提问
void Ask(QString str);
};
Teacher.cpp
#include "Teacher.h"
Teacher::Teacher(QObject *parent)
: QObject(parent)
{}
Teacher::~Teacher()
{}
Student.h
#pragma once
#include <QObject>
#include <QDebug>
#include <QMessageBox>
class Student : public QObject
{
Q_OBJECT
public slots:
void Answer();
void Answer(QString str);
public:
Student(QObject *parent);
~Student();
};
Student.cpp
#include "Student.h"
Student::Student(QObject *parent)
: QObject(parent)
{}
Student::~Student()
{}
void Student::Answer()
{
QMessageBox::information(NULL, "Message", "student answer the question",QMessageBox::Ok|QMessageBox::Cancel);
}
void Student::Answer(QString str)
{
QMessageBox::information(NULL, "Message", str+": Answer Is OK", QMessageBox::Ok | QMessageBox::Cancel);
}
QSingalSlot.h
#include "ui_QSingalSlot.h"
#include "Teacher.h"
#include "Student.h"
class QSingalSlot : public QWidget
{
Q_OBJECT
public:
QSingalSlot(QWidget *parent = nullptr);
~QSingalSlot();
Teacher* tech;
Student* stud;
public slots:
void AskQuestion();
private:
Ui::QSingalSlotClass ui;
};
QSingalSlot.cpp
#include "QSingalSlot.h"
QSingalSlot::QSingalSlot(QWidget *parent)
: QWidget(parent)
{
ui.setupUi(this);
this->tech=new Teacher(nullptr);
this->stud=new Student(nullptr);
//函数指针
void (Teacher:: * teachSignal)(QString) = &Teacher::Ask;
void (Student:: * studentSlot)(QString) = &Student::Answer;
// connect(tech, SIGNAL(Teacher::Ask()), stud, SLOT(Student::Answer())); //写法调用不了
// connect(tech, &Teacher::Ask, stud, &Student::Answer);
connect(tech, teachSignal, stud, studentSlot);
connect(ui.pushButton_Ask, SIGNAL(clicked()), this, SLOT(AskQuestion()));
}
QSingalSlot::~QSingalSlot()
{}
void QSingalSlot::AskQuestion()
{
//emit tech->Ask();
emit tech->Ask("yes or ok");
}
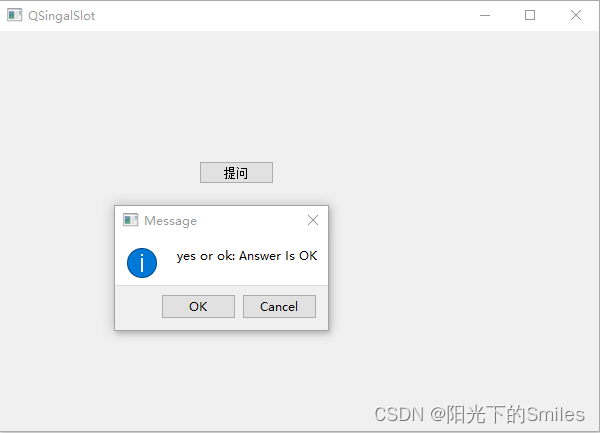