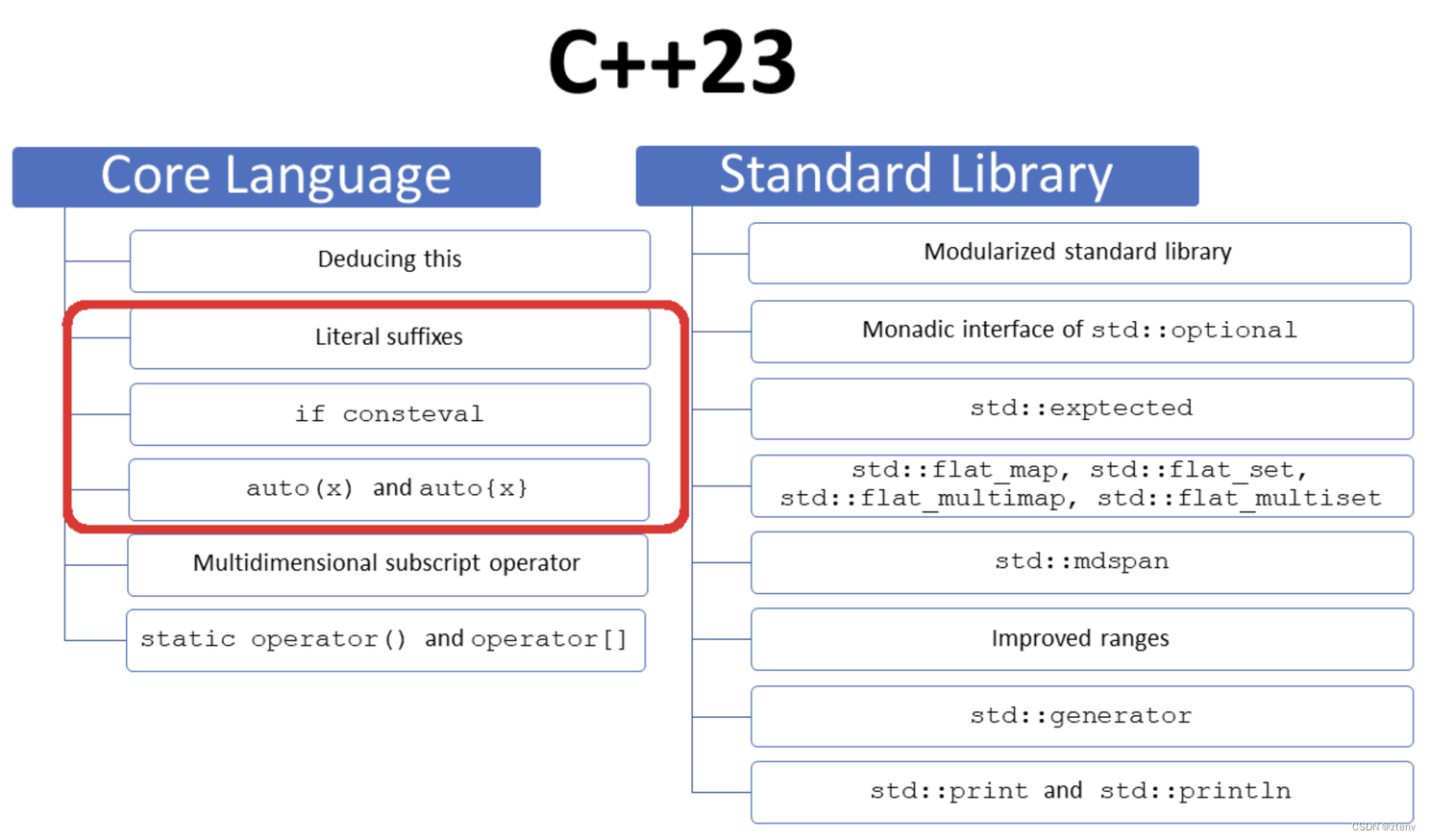
New language features
New language feature testing macros
Explicit object parameters, explicit object member functions (deducing this)
if consteval / if not consteval
Multidimensional subscript operator (e.g. v[1, 3, 7] = 42;)
static operator()
static operator[]
auto(x): decay-copy in the language
Attributes on lambda-expressions
Optional extended floating-point types: std::float{16|32|64|128}_t, and std::bfloat16_t.
#elifdef, #elifndef, and #warning
Literal suffix 'Z'/'z' for (signed) std::size_t literals
Assumptions via new attribute [[assume(expression)]]
Named universal character escapes
A portable source file encoding is UTF-8
White-spaces trimming before line splicing.
New library features
New modules
std and std.compat
New headers
C compatibility headers:
Library features
New library feature testing macros
New ranges fold algorithms
String formatting improvements
"flat" container adaptors: std::flat_map, std::flat_multimap, std::flat_set, std::flat_multiset
std::mdspan
std::generator
std::basic_string::contains, std::basic_string_view::contains
Construction of std::string_view from nullptr is disallowed
std::basic_string::resize_and_overwrite
Monadic operations for std::optional: or_else, and_then, transform
Stacktrace library
New ranges algorithms:
New range adaptors (views):
Changes to ranges library
Changes to views library
Marking unreachable code: std::unreachable
New vocabulary type std::expected
std::move_only_function
New I/O stream std::spanstream with program-provided fixed size buffer
std::byteswap
std::to_underlying
Associative containers heterogeneous erasure.
C++23, the latest iteration of the C++ programming language standard, follows its predecessor C++20 with several enhancements aimed at improving usability, performance, and code clarity. As a refinement of the language, C++23 doesn't introduce as many large features as C++20, which included major additions like concepts, ranges, and coroutines. However, it does provide important updates and new features that developers should be aware of. This article delves into some of the most significant changes and improvements in C++23 compared to previous versions.
- Simplified Syntax for using Declarations
C++23 has introduced a simplified syntax for using declarations, which allows a base class's constructor or assignment operator to be inherited more concisely. Previously, inheriting constructors from a base class required specifying each constructor individually, which could be verbose and error-prone. The new feature reduces boilerplate code and enhances readability.
C++
struct Base {
Base(int x) {}
Base(double x, int y) {}
};
struct Derived : Base {
using Base::Base; // Inherit all constructors from Base
};
- Standardized std::print
One of the most anticipated features in C++23 is the introduction of std::print. This feature aims to standardize a common operation---printing to the console---which previously required using std::cout or other more complex I/O functions. std::print simplifies this task by providing a straightforward way to print formatted data, reducing the learning curve for new C++ programmers and increasing code clarity.
C++
#include <iostream>
int main() {
std::print("Hello, World!\n");
std::print("The answer is: {}\n", 42);
}
- Enhancements to std::expected
std::expected is a template class that handles expected errors as an alternative to exceptions. It was proposed to provide a way to return and check for error conditions in a manner that is more visible in the function signature than exceptions. C++23 aims to include this feature to simplify error handling and improve performance by avoiding the overhead associated with exceptions.
C++
#include <expected>
#include <iostream>
std::expected<int, const char*> compute(int x) {
if (x < 0) return "Negative input not allowed";
return x * x;
}
int main() {
auto result = compute(10);
if (result) {
std::cout << "Result: " << *result << std::endl;
} else {
std::cout << "Error: " << result.error() << std::endl;
}
}
- std::mdspan
Another notable addition is std::mdspan a multidimensional array view that provides an interface for accessing elements in a multidimensional data layout without owning the underlying data. This feature is crucial for scientific and numerical applications where performance and flexibility in data access patterns are important.
C++
#include <mdspan>
#include <vector>
#include <iostream>
int main() {
std::vector<int> data = {1, 2, 3, 4, 5, 6, 7, 8, 9};
std::mdspan<int, std::extents<3, 3>> matrix(data.data());
for (int i = 0; i < 3; ++i) {
for (int j = 0; j < 3; ++j) {
std::cout << matrix(i, j) << " ";
}
std::cout << "\n";
}
}
- Improved Lambda Expressions
C++23 continues to enhance lambda expressions by introducing new features such as simplified syntax for not capturing any variables and improved constexpr capabilities. These improvements make lambdas more powerful and easier to use in a wider range of contexts, including compile-time programming.
C++
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto is_even = [](int x) constexpr { return x % 2 == 0; };
auto pos = std::find_if(numbers.begin(), numbers.end(), is_even);
if (pos != numbers.end()) {
std::cout << "First even number: " << *pos << std::endl;
}
}
- constexpr Enhancements
The constexpr specifier has been significantly expanded in C++23, allowing more complex computations to be performed at compile time. This includes the ability to use dynamic memory allocation, try and catch blocks, and virtual calls within constexpr functions, which were previously not possible.
C++
#include <iostream>
constexpr int* create_array(int size) {
return new int[size]{1, 2, 3}; // Dynamic allocation in constexpr
}
int main() {
constexpr auto* array = create_array(3);
std::cout << array[0] << ", " << array[1] << ", " << array[2] << std::endl;
delete[] array;
}
- Networking TS (Technical Specification)
While not fully integrated into the standard, progress has been made on the Networking TS, which is expected to eventually be part of the standard library. C++23 has made strides towards incorporating more networking capabilities directly into the standard library, facilitating easier and more standardized network programming.
Conclusion
C++23 offers several practical enhancements that refine the capabilities introduced in C++20 and earlier versions. As with any new standard, the adoption rate will depend on compiler support and the specific needs of projects. Nonetheless, personally I find these improvements in C++23 an attractive upgrade for C++ developers seeking to leverage the latest advancements in the language.