图片
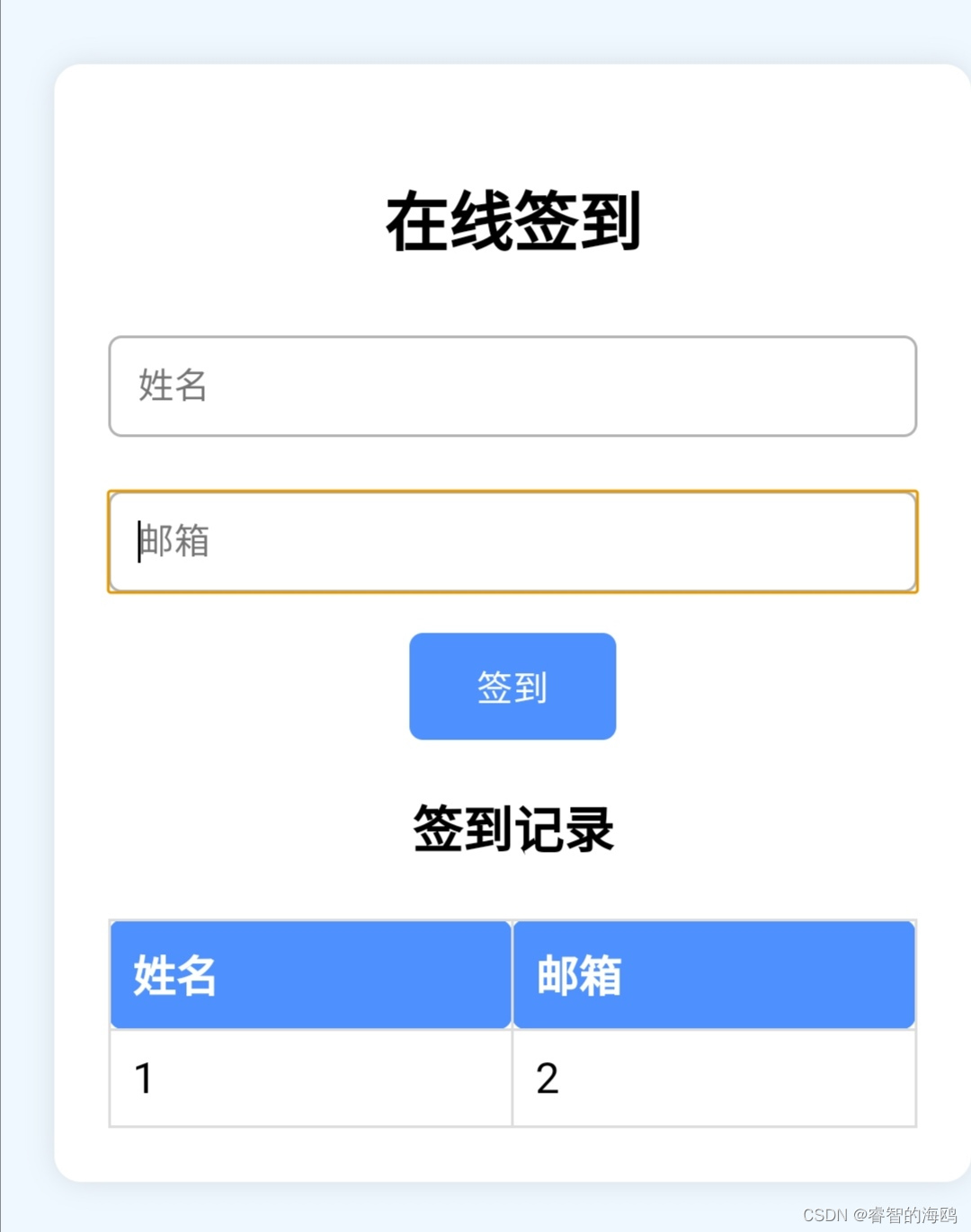
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>在线签到系统</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f0f8ff;
padding: 20px;
}
.container {
background-color: white;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0,0,0,0.1);
padding: 20px;
width: 300px;
margin: auto;
text-align: center; /* 居中内容 */
}
input[type=text], input[type=email] {
width: 100%;
padding: 10px;
margin: 5px 0 15px 0;
border: 1px solid #b3b3b3;
border-radius: 5px;
box-sizing: border-box;
}
button {
background-color: #4d90fe; /* 蓝色背景 */
color: white;
padding: 10px 25px; /* 调整宽度 */
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #4787ed; /* 鼠标悬停时的蓝色 */
}
.alert {
color: red;
display: none;
}
table {
width: 100%;
margin-top: 20px;
border-collapse: collapse;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
border-radius: 5px;
}
th {
background-color: #4d90fe; /* 蓝色背景 */
color: white;
}
tr:nth-child(even) {
background-color: #f2f2f2;
}
</style>
<script>
function checkIn() {
var name = document.getElementById("name").value.trim(); // 去除前后空格
var email = document.getElementById("email").value.trim();
var alert = document.getElementById("alert");
var checkInList = document.getElementById("checkInList");
if (name === "" || email === "") {
alert.textContent = "姓名和邮箱不能为空!";
alert.style.display = "block";
return;
} else if (localStorage.getItem(email + "_checked")) { // 使用_email_checked避免冲突
alert.textContent = "您已签到过!";
alert.style.display = "block";
return;
} else {
localStorage.setItem(email + "_checked", "true");
alert.textContent = "签到成功!";
alert.style.display = "block";
// 添加签到记录到表格
var newRow = checkInList.insertRow(-1);
var nameCell = newRow.insertCell(0);
var emailCell = newRow.insertCell(1);
nameCell.textContent = name;
emailCell.textContent = email;
// 清空输入框
document.getElementById("name").value = "";
document.getElementById("email").value = "";
setTimeout(function() {
alert.style.display = "none";
}, 2000);
}
}
</script>
</head>
<body>
<div class="container">
<h2>在线签到</h2>
<input type="text" id="name" placeholder="姓名" required>
<input type="email" id="email" placeholder="邮箱" required>
<button οnclick="checkIn()">签到</button>
<p id="alert" class="alert"></p>
<h3>签到记录</h3>
<table>
<thead>
<tr>
<th>姓名</th>
<th>邮箱</th>
</tr>
</thead>
<tbody id="checkInList">
<!-- 签到记录将动态插入此处 -->
</tbody>
</table>
</div>
</body>
</html>