一个复杂的布局案例
实现如下布局:
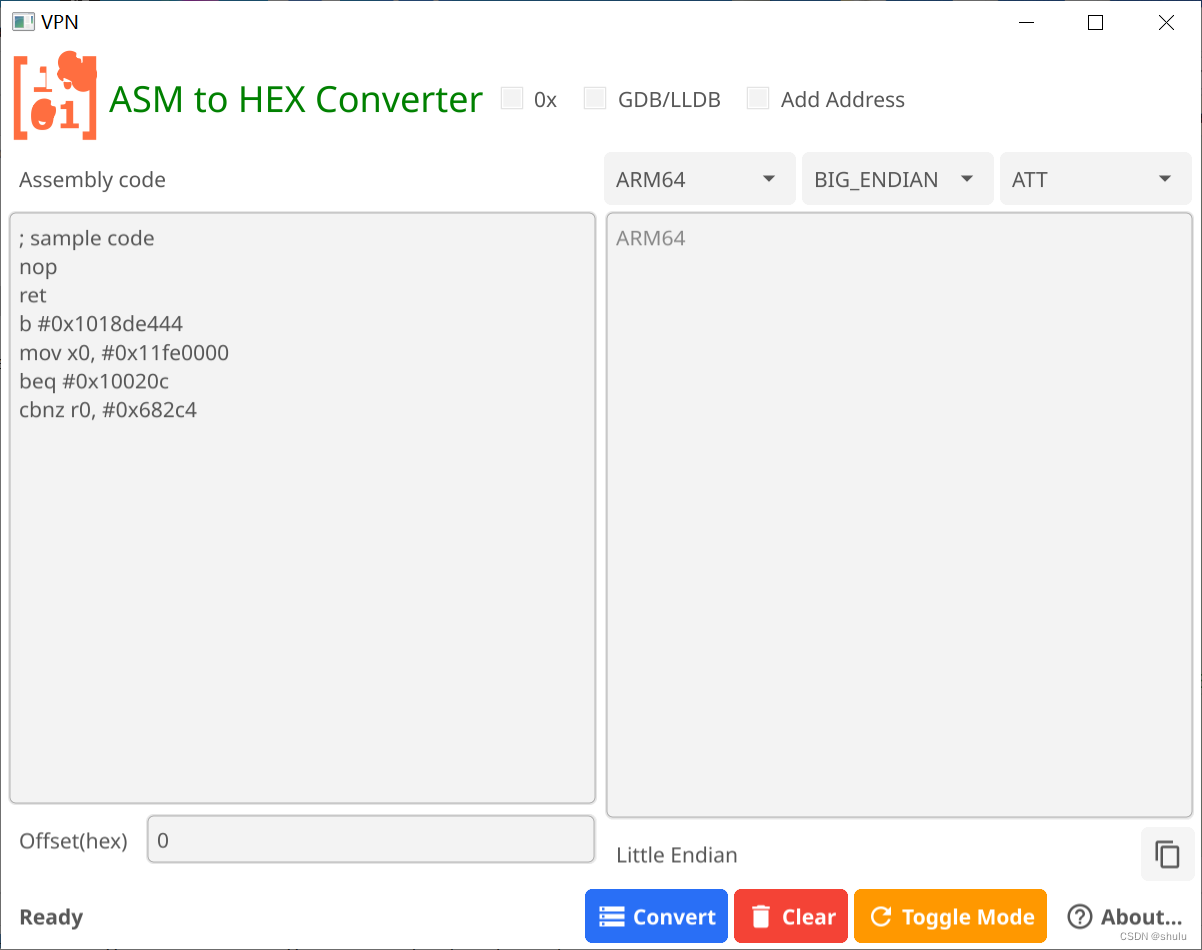
布局分析:
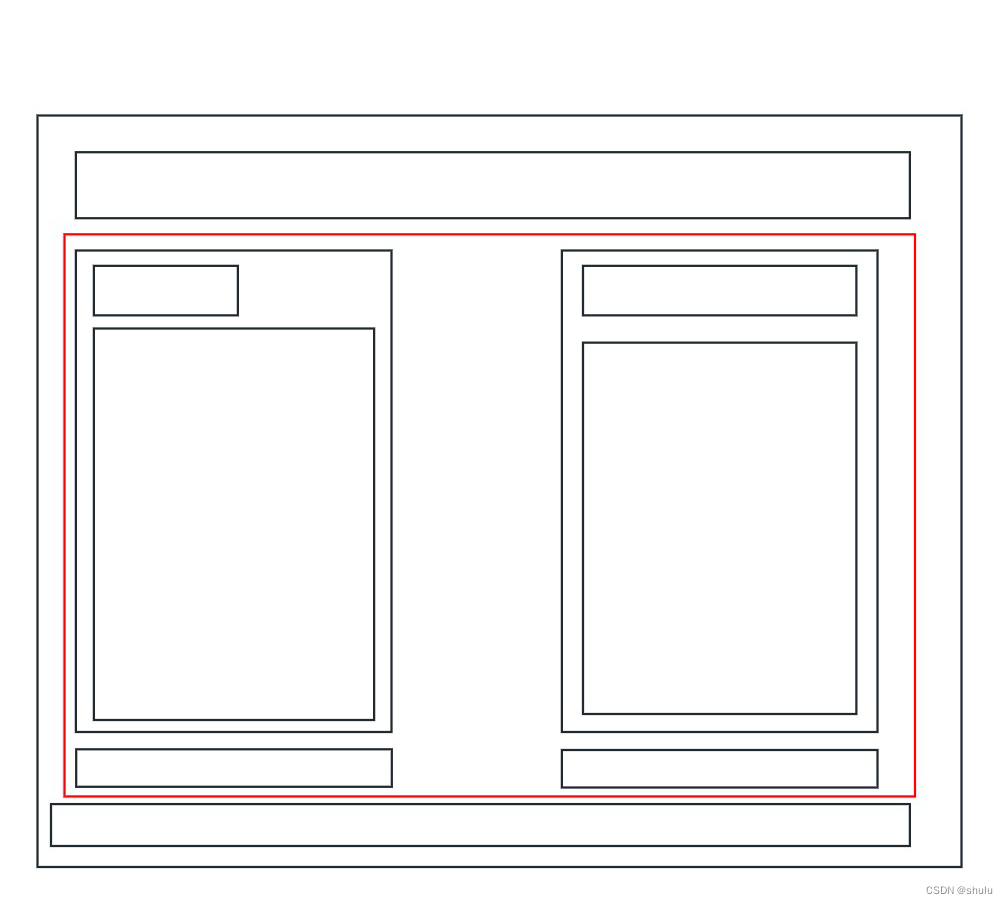
代码如下:
go
package main
import (
"complexlayout/icons"
"fyne.io/fyne/v2"
"fyne.io/fyne/v2/app"
"fyne.io/fyne/v2/canvas"
"fyne.io/fyne/v2/container"
"fyne.io/fyne/v2/layout"
"fyne.io/fyne/v2/theme"
"fyne.io/fyne/v2/widget"
"image/color"
)
const ApplicationTitle string = "ASM to HEX Converter"
var ArchString = []string{"ARM", "ARM64", "MIPS", "X86", "PPC", "SPARC", "SYSTEMZ"}
var ModeString = []string{"LITTLE_ENDIAN", "BIG_ENDIAN"}
var SyntaxString = []string{"INTEL", "ATT"}
func main() {
myApp := app.New()
myWindow := myApp.NewWindow("VPN")
assemblyLabel := widget.NewLabel("Assembly code")
offsetLabel := widget.NewLabel("Offset(hex)")
output1 := widget.NewMultiLineEntry()
output1.SetPlaceHolder("ARM64")
output1.SetMinRowsVisible(24)
output1.TextStyle.Monospace = true
output1_info := widget.NewLabel("Little Endian")
output1_card := container.NewVBox(output1,
container.NewHBox(
output1_info,
layout.NewSpacer(),
widget.NewButtonWithIcon("", theme.ContentCopyIcon(), func() {}),
))
assembly_code := `; sample code
nop
ret
b #0x1018de444
mov x0, #0x11fe0000
beq #0x10020c
cbnz r0, #0x682c4
`
assemblyEditor := widget.NewMultiLineEntry()
assemblyEditor.SetPlaceHolder("Assembly code")
assemblyEditor.SetText(assembly_code)
assemblyEditor.AcceptsTab()
assemblyEditor.SetMinRowsVisible(20)
offsetInput := widget.NewEntry()
offsetInput.SetPlaceHolder("Offset(hex)")
offsetInput.SetText("0")
left_container := container.New(layout.NewVBoxLayout(),
assemblyLabel,
assemblyEditor,
container.New(layout.NewFormLayout(), offsetLabel, offsetInput),
)
right_container := container.New(layout.NewVBoxLayout(),
createDropdowns(),
output1_card,
)
grid := container.New(layout.NewGridLayoutWithColumns(2),
left_container,
right_container,
)
app_title := canvas.NewText(ApplicationTitle, color.NRGBA{0, 0x80, 0, 0xff})
app_title.TextSize = 24
logo_icon := fyne.NewStaticResource("asm2hex.svg", icons.LOGO_ICON_BIN)
background := canvas.NewImageFromResource(logo_icon)
background.SetMinSize(fyne.NewSize(64, 64))
status := widget.NewLabel("Ready")
status.Alignment = fyne.TextAlignTrailing
status.TextStyle = fyne.TextStyle{Bold: true}
convertBtn := widget.NewButtonWithIcon("Convert", theme.StorageIcon(), func() {})
convertBtn.Importance = widget.HighImportance
toggleBtn := widget.NewButtonWithIcon("Toggle Mode", theme.ViewRefreshIcon(), func() {})
toggleBtn.Importance = widget.WarningImportance
clearBtn := widget.NewButtonWithIcon("Clear", theme.DeleteIcon(), func() {})
clearBtn.Importance = widget.DangerImportance
aboutBtn := widget.NewButtonWithIcon("About...", theme.QuestionIcon(), func() {})
aboutBtn.Importance = widget.LowImportance
status_container := container.New(layout.NewHBoxLayout(),
status,
layout.NewSpacer(),
convertBtn,
clearBtn,
toggleBtn,
aboutBtn,
)
main_layout := container.New(layout.NewVBoxLayout(),
container.New(layout.NewHBoxLayout(),
background,
app_title,
widget.NewCheck("0x", func(checked bool) {}),
widget.NewCheck("GDB/LLDB", func(checked bool) {}),
widget.NewCheck("Add Address", func(checked bool) {})),
grid,
status_container,
)
myWindow.SetContent(main_layout)
myWindow.Resize(fyne.NewSize(800, 600))
myWindow.CenterOnScreen()
myWindow.Show()
myApp.Run()
}
func createDropdowns() *fyne.Container {
keystoneArchDropdown := &widget.Select{}
keystoneArchDropdown.ExtendBaseWidget(keystoneArchDropdown)
keystoneArchDropdown.SetOptions(ArchString)
keystoneArchDropdown.SetSelectedIndex(1)
keystoneModeDropdown := &widget.Select{}
keystoneModeDropdown.ExtendBaseWidget(keystoneModeDropdown)
keystoneModeDropdown.SetOptions(ModeString)
keystoneModeDropdown.SetSelectedIndex(1)
keystoneSyntaxDropdown := &widget.Select{}
keystoneSyntaxDropdown.ExtendBaseWidget(keystoneSyntaxDropdown)
keystoneSyntaxDropdown.SetOptions(SyntaxString)
keystoneSyntaxDropdown.SetSelectedIndex(1)
return container.NewHBox(
keystoneArchDropdown,
keystoneModeDropdown,
keystoneSyntaxDropdown,
)
}
总结:
实现这个布局使用到了VBox、HBox、Grid。
使用的组件有:Label、MultiLineEntry、ButtonWithIcon、Entry、Select、ImageFromResource、Check。