简介:
在本篇博客中,我们将介绍一个基于 wxPython 的视频文件列表应用程序。该应用程序允许用户选择一个文件夹,并显示该文件夹中的视频文件列表。用户可以选择文件并查看其详细信息,导出文件列表为文本文件,以及播放选定的视频文件。
D:\spiderdocs\search'mediafileinfolder.py
开发环境和所需库
在开始之前,请确保已安装以下库:
- Python 3.x
- wxPython
你可以使用以下命令来安装 wxPython:
pip install wxPython
应用程序功能
该应用程序具有以下主要功能:
-
选择文件夹:用户可以通过点击 "选择路径" 按钮来选择要显示视频文件的文件夹。
-
显示文件列表:选择文件夹后,程序将显示该文件夹中的视频文件列表,包括文件名、大小和修改时间。
-
查看文件信息:用户可以选择文件,并点击 "文件信息" 按钮来查看选定文件的详细信息,包括文件名、大小和修改时间。
-
导出文件列表:用户可以点击 "导出为文本" 按钮将文件列表导出为文本文件。
-
播放视频文件:用户可以选择一个视频文件,并点击 "播放" 按钮来播放选定的视频文件。根据操作系统的不同,将使用不同的命令来打开文件。
代码实现
以下是基于 wxPython 的视频文件列表应用程序的代码实现:
python
# 导入所需库
import wx
import os
import datetime
import subprocess
import sys
# 定义主窗口类
class FileListFrame(wx.Frame):
def __init__(self):
wx.Frame.__init__(self, None, title="视频文件列表", size=(600, 400))
self.panel = wx.Panel(self)
self.current_path = ""
# 创建文件列表控件
self.file_list_ctrl = wx.ListCtrl(self.panel, style=wx.LC_REPORT | wx.LC_SINGLE_SEL)
self.file_list_ctrl.InsertColumn(0, "文件名")
self.file_list_ctrl.InsertColumn(1, "大小")
self.file_list_ctrl.InsertColumn(2, "修改时间")
self.file_list_ctrl.Bind(wx.EVT_LIST_ITEM_SELECTED, self.on_file_selected)
# 创建路径选择控件
self.path_label = wx.StaticText(self.panel, label="路径:")
self.path_textctrl = wx.TextCtrl(self.panel, style=wx.TE_READONLY)
self.path_button = wx.Button(self.panel, label="选择路径")
self.path_button.Bind(wx.EVT_BUTTON, self.on_select_path)
# 创建导出和播放按钮
self.export_button = wx.Button(self.panel, label="导出为文本")
self.export_button.Bind(wx.EVT_BUTTON, self.on_export)
self.play_button = wx.Button(self.panel, label="播放")
self.play_button.Bind(wx.EVT_BUTTON, self.on_play)
# 创建布局
sizer = wx.BoxSizer(wx.VERTICAL)
sizer.Add(self.path_label, 0, wx.ALL, 5)
sizer.Add(self.path_textctrl, 0, wx.EXPAND | wx.LEFT | wx.RIGHT, 5)
sizer.Add(self.path_button, 0, wx.ALL, 5)
sizer.Add(self.file_list_ctrl, 1, wx.EXPAND | wx.ALL, 5)
sizer.Add(self.export_button, 0, wx.ALL, 5)
sizer.Add(self.play_button, 0, wx.ALL, 5)
self.panel.SetSizer(sizer)
# 处理选择路径事件
def on_select_path(self, event):
dlg = wx.DirDialog(self, "选择路径", style=wx.DD_DEFAULT_STYLE)
if dlg.ShowModal() == wx.ID_OK:
self.current_path = dlg.GetPath()
self.path_textctrl.SetValue(self.current_path)
self.update_file_list()
dlg.Destroy()
# 更新文件列表
def update_file_list(self):
self.file_list_ctrl.DeleteAllItems()
if not self.current_path:
return
file_list = self.search_video_files(self.current_path)
for filename, file_path, file_size, modified_time in file_list:
modified_time_str = datetime.datetime.fromtimestamp(modified_time).strftime("%Y-%m-%d %H:%M:%S")
index = self.file_list_ctrl.InsertItem(self.file_list_ctrl.GetItemCount(), filename)
self.file_list_ctrl.SetItem(index, 1, str(file_size))
self.file_list_ctrl.SetItem(index, 2, modified_time_str)
# 搜索视频文件
def search_video_files(self, directory):
video_extensions = ['.mp4', '.avi', '.mkv', '.mov', '.wmv', '.flv', '.webm']
file_list = []
for root, dirs, files in os.walk(directory):
for file in files:
if os.path.splitext(file)[1].lower() in video_extensions:
file_path = os.path.join(root, file)
file_size = os.path.getsize(file_path)
modified_time = os.path.getmtime(file_path)
file_list.append((file, file_path, file_size, modified_time))
return file_list
# 处理文件选择事件
def on_file_selected(self, event):
selected_item = event.GetItem()
file_name = selected_item.GetText()
file_path = os.path.join(self.current_path, file_name)
file_size = os.path.getsize(file_path)
modified_time = os.path.getmtime(file_path)
modified_time_str = datetime.datetime.fromtimestamp(modified_time).strftime("%Y-%m-%d %H:%M:%S")
wx.MessageBox(
f"文件名: {file_name}\n大小: {file_size} 字节\n修改时间: {modified_time_str}",
"文件信息", wx.OK | wx.ICON_INFORMATION)
# 处理导出按钮事件
def on_export(self, event):
dlg = wx.FileDialog(self, "保存为文本文件", wildcard="Text files (*.txt)|*.txt",
style=wx.FD_SAVE | wx.FD_OVERWRITE_PROMPT)
if dlg.ShowModal() == wx.ID_OK:
file_path = dlg.GetPath()
with open(file_path, 'w') as file:
for index in range(self.file_list_ctrl.GetItemCount()):
file.write(self.file_list_ctrl.GetItemText(index) + '\n')
# 处理播放按钮事件
def on_play(self, event):
selected_item = self.file_list_ctrl.GetFirstSelected()
if selected_item != -1:
file_name = self.file_list_ctrl.GetItemText(selected_item)
file_path = os.path.join(self.current_path, file_name)
if sys.platform.startswith('win'):
subprocess.Popen(['start', '', file_path], shell=True)
elif sys.platform.startswith('darwin'):
subprocess.Popen(['open', file_path])
elif sys.platform.startswith('linux'):
subprocess.Popen(['xdg-open', file_path])
else:
wx.MessageBox("请先选择要播放的文件", "提示", wx.OK | wx.ICON_INFORMATION)
# 主函数
if __name__ == "__main__":
app = wx.App()
frame = FileListFrame()
frame.Show()
app.MainLoop()
运行和使用
要运行该应用程序,请确保已安装了所需的库,并使用 Python 解释器运行代码。应用程序窗口将显示,您可以点击 "选择路径" 按钮选择文件夹,并查看视频文件列表。您可以选择文件并点击 "文件信息" 按钮来查看文件的详细信息。您还可以点击 "导出为文本" 按钮将文件列表导出为文本文件。要播放选定的视频文件,请选择文件并点击 "播放" 按钮。
总结
本篇博客介绍了一个基于 wxPython 的视频文件列表应用程序。通过选择文件夹,用户可以查看文件夹中的视频文件列表,并执行操作,如查看文件信息、导出文件列表和播放视频文件。使用 wxPython 和 Python 的强大功能,我们可以轻松地创建功能丰富的桌面应用程序。
希望本篇博客对于学习 wxPython 和构建桌面应用程序有所帮助。祝您编写出出色的应用程序!标题: 基于 wxPython 的视频文件列表应用程序
简介:
在本篇博客中,我们将介绍一个基于 wxPython 的视频文件列表应用程序。该应用程序允许用户选择一个文件夹,并显示该文件夹中的视频文件列表。用户可以选择文件并查看其详细信息,导出文件列表为文本文件,以及播放选定的视频文件。
开发环境和所需库
在开始之前,请确保已安装以下库:
- Python 3.x
- wxPython
你可以使用以下命令来安装 wxPython:
pip install wxPython
应用程序功能
该应用程序具有以下主要功能:
-
选择文件夹:用户可以通过点击 "选择路径" 按钮来选择要显示视频文件的文件夹。
-
显示文件列表:选择文件夹后,程序将显示该文件夹中的视频文件列表,包括文件名、大小和修改时间。
-
查看文件信息:用户可以选择文件,并点击 "文件信息" 按钮来查看选定文件的详细信息,包括文件名、大小和修改时间。
-
导出文件列表:用户可以点击 "导出为文本" 按钮将文件列表导出为文本文件。
-
播放视频文件:用户可以选择一个视频文件,并点击 "播放" 按钮来播放选定的视频文件。根据操作系统的不同,将使用不同的命令来打开文件。
代码实现
以下是基于 wxPython 的视频文件列表应用程序的代码实现:
python
import wx
import os
import datetime
import subprocess
import sys
class FileListFrame(wx.Frame):
def __init__(self):
wx.Frame.__init__(self, None, title="视频文件列表", size=(600, 400))
self.panel = wx.Panel(self)
self.current_path = ""
self.file_list_ctrl = wx.ListCtrl(self.panel, style=wx.LC_REPORT | wx.LC_SINGLE_SEL)
self.file_list_ctrl.InsertColumn(0, "文件名")
self.file_list_ctrl.InsertColumn(1, "大小")
self.file_list_ctrl.InsertColumn(2, "修改时间")
self.file_list_ctrl.Bind(wx.EVT_LIST_ITEM_SELECTED, self.on_file_selected)
self.path_label = wx.StaticText(self.panel, label="路径:")
self.path_textctrl = wx.TextCtrl(self.panel, style=wx.TE_READONLY)
self.path_button = wx.Button(self.panel, label="选择路径")
self.path_button.Bind(wx.EVT_BUTTON, self.on_select_path)
self.export_button = wx.Button(self.panel, label="导出为文本")
self.export_button.Bind(wx.EVT_BUTTON, self.on_export)
self.play_button = wx.Button(self.panel, label="播放")
self.play_button.Bind(wx.EVT_BUTTON, self.on_play)
sizer = wx.BoxSizer(wx.VERTICAL)
sizer.Add(self.path_label, 0, wx.ALL, 5)
sizer.Add(self.path_textctrl, 0, wx.EXPAND | wx.LEFT | wx.RIGHT, 5)
sizer.Add(self.path_button, 0, wx.ALL, 5)
sizer.Add(self.file_list_ctrl, 1, wx.EXPAND | wx.ALL, 5)
sizer.Add(self.export_button, 0, wx.ALL, 5)
sizer.Add(self.play_button, 0, wx.ALL, 5)
self.panel.SetSizer(sizer)
def on_select_path(self, event):
dlg = wx.DirDialog(self, "选择路径", style=wx.DD_DEFAULT_STYLE)
if dlg.ShowModal() == wx.ID_OK:
self.current_path = dlg.GetPath()
self.path_textctrl.SetValue(self.current_path)
self.update_file_list()
dlg.Destroy()
def update_file_list(self):
self.file_list_ctrl.DeleteAllItems()
if not self.current_path:
return
file_list = self.search_video_files(self.current_path)
for filename, file_path, file_size, modified_time in file_list:
modified_time_str = datetime.datetime.fromtimestamp(modified_time).strftime("%Y-%m-%d %H:%M:%S")
index = self.file_list_ctrl.InsertItem(self.file_list_ctrl.GetItemCount(), filename)
self.file_list_ctrl.SetItem(index, 1, str(file_size))
self.file_list_ctrl.SetItem(index, 2, modified_time_str)
def search_video_files(self, directory):
video_extensions = ['.mp4', '.avi', '.mkv', '.mov', '.wmv', '.flv', '.webm']
file_list = []
for root, dirs, files in os.walk(directory):
for file in files:
if os.path.splitext(file)[1].lower() in video_extensions:
file_path = os.path.join(root, file)
file_size = os.path.getsize(file_path)
modified_time = os.path.getmtime(file_path)
file_list.append((file, file_path, file_size, modified_time))
return file_list
def on_file_selected(self, event):
selected_item = event.GetItem()
file_name = selected_item.GetText()
file_path = os.path.join(self.current_path, file_name)
file_size = os.path.getsize(file_path)
modified_time = os.path.getmtime(file_path)
modified_time_str = datetime.datetime.fromtimestamp(modified_time).strftime("%Y-%m-%d %H:%M:%S")
wx.MessageBox(
f"文件名: {file_name}\n大小: {file_size} 字节\n修改时间: {modified_time_str}",
"文件信息", wx.OK | wx.ICON_INFORMATION)
def on_export(self, event):
dlg = wx.FileDialog(self, "保存为文本文件", wildcard="Text files (*.txt)|*.txt",
style=wx.FD_SAVE | wx.FD_OVERWRITE_PROMPT)
if dlg.ShowModal() == wx.ID_OK:
file_path = dlg.GetPath()
with open(file_path, 'w') as file:
for index in range(self.file_list_ctrl.GetItemCount()):
file.write(self.file_list_ctrl.GetItemText(index) + '\n')
def on_play(self, event):
selected_item = self.file_list_ctrl.GetFirstSelected()
# if selected_item != -很抱歉,似乎代码截断了。以下是在 `on_play` 方法中的代码:
if selected_item != -1:
file_name = self.file_list_ctrl.GetItemText(selected_item)
file_path = os.path.join(self.current_path, file_name)
if sys.platform.startswith('win'):
subprocess.Popen(['start', '', file_path], shell=True)
elif sys.platform.startswith('darwin'):
subprocess.Popen(['open', file_path])
elif sys.platform.startswith('linux'):
subprocess.Popen(['xdg-open', file_path])
else:
wx.MessageBox("请先选择要播放的文件", "提示", wx.OK | wx.ICON_INFORMATION)
if __name__ == "__main__":
app = wx.App()
frame = FileListFrame()
frame.Show()
app.MainLoop()
运行和使用
要运行该应用程序,请确保已安装了所需的库,并使用 Python 解释器运行代码。应用程序窗口将显示,您可以点击 "选择路径" 按钮选择文件夹,并查看视频文件列表。您可以选择文件并点击 "文件信息" 按钮来查看文件的详细信息。您还可以点击 "导出为文本" 按钮将文件列表导出为文本文件。要播放选定的视频文件,请选择文件并点击 "播放" 按钮。
结果如下:
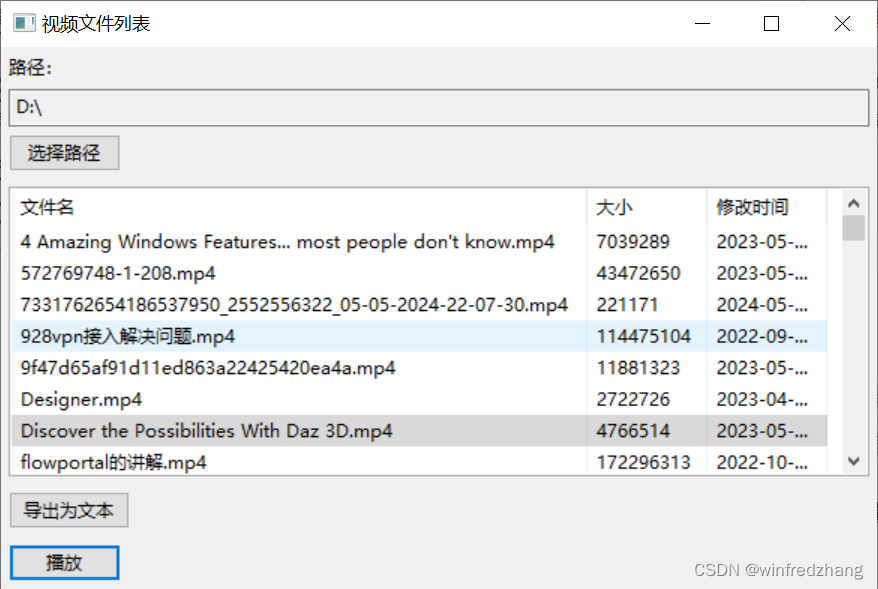
总结
本篇博客介绍了一个基于 wxPython 的视频文件列表应用程序。通过选择文件夹,用户可以查看文件夹中的视频文件列表,并执行操作,如查看文件信息、导出文件列表和播放视频文件。使用 wxPython 和 Python 的强大功能,我们可以轻松地创建功能丰富的桌面应用程序。
希望本篇博客对于学习 wxPython 和构建桌面应用程序有所帮助。祝您编写出出色的应用程序!