//SPDX-License-Identifier:MIT
pragma solidity ^0.8.24;
interface IERC721{
function transFrom(address _from,address _to,uint nftid) external ;
}
contract DutchAuction
{
address payable immutable seller;//卖方
uint immutable startTime;//拍卖开始时间
uint immutable endTime;//拍卖结束时间
uint immutable lastTime;//拍卖持续时间
uint immutable startPrice;//启始价格
uint immutable discount;//每秒减少多少钱
IERC721 public nft;//nft 变量能够存储一个已经部署的符合 IERC721 接口的合约地址
uint public nftId;
constructor(//对合约中的不可变变量进行赋值
address payable _seller,
uint _startTime,
uint _lastTime,
uint _startPrice,
uint _discount,
IERC721 _nft,
uint _nftId
){
seller=_seller;
startTime=_startTime;
lastTime=_lastTime;
endTime=_lastTime+_startTime;
startPrice=_startPrice;
discount=_discount;
require(startPrice>discount * lastTime,"it may become nagetive number");//检查一下防止拍卖价成为负数
nft=_nft;
nftId=_nftId;
}
function getPrice() public view returns(uint ){//获取当前(调用本函数的时刻)的价格
require(startTime+block.timestamp<=endTime,"Sorry,you comes too late");//检查是否流派
return(startPrice-(block.timestamp-startTime)*discount);
}
function buy()external payable returns(bool)
{
uint nowPrice=getPrice();
require(msg.value>= nowPrice,"You have no enough money");
require(startTime+block.timestamp<=endTime,"Sorry,you comes too late");
nft.transFrom(seller,msg.sender,nftId);
uint refund = msg.value- nowPrice;
payable(msg.sender).transfer(refund);
seller.transfer(nowPrice);
destroy();
return true;
}
function destroy()public payable{
seller.transfer(address(this).balance);
}
}
荷兰合约(Dutch Auction Contract)是一种智能合约,通常运行在区块链上,用于实现荷兰式拍卖(Dutch Auction)的拍卖逻辑。荷兰合约的设计旨在确保拍卖过程的透明性、安全性和自动化执行。
荷兰拍卖的基本组成和功能:
-
起始价格设定:
- 合约会设定一个初始的高价作为起始拍卖价格。
-
价格递减设定:
- 根据预定的规则或时间间隔,合约会以固定速度递减拍卖价格。这个递减的速度通常是事先确定好的,可以根据拍卖的需求调整。
-
买家接受价格:
- 参与者可以在拍卖过程中选择接受当前价格。一旦有人接受了当前价格,拍卖即结束。
-
自动执行:
- 荷兰合约通常具备自动执行功能,一旦有人接受了当前价格,合约会立即执行资金的转移和商品或服务的交割。
-
资金管理:
- 合约会确保安全地处理所有资金的转移,确保卖方和买方的权益。
-
拍卖结束条件:
- 拍卖可以在以下条件下结束:
- 有人接受了当前价格;
- 拍卖价格降至预设的最低价;
- 达到预定的拍卖结束时间。
- 拍卖可以在以下条件下结束:
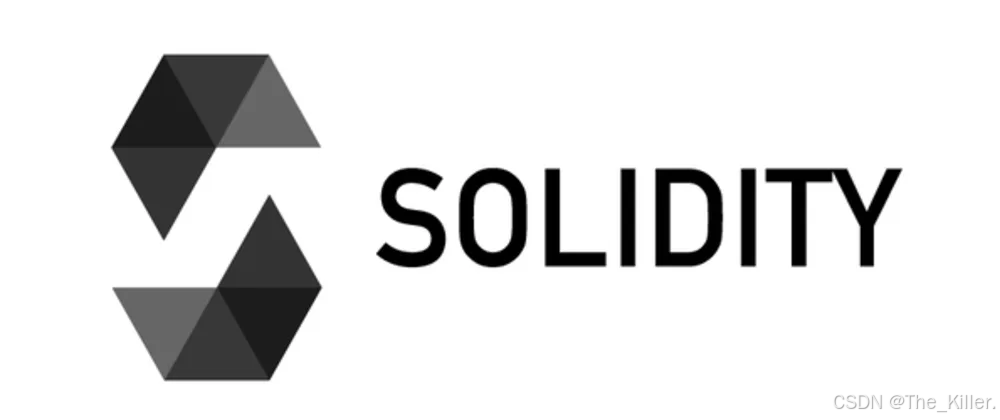