2 设置响应数据
在 Express 中,可以通过 res
对象设置 HTTP 响应。常见的响应方法包括 res.send
、res.json
、res.status
、res.sendFile
等。
2.1 发送文本和 JSON 响应
发送文本响应:
javascript
app.get('/text', (req, res) => {
res.send('Hello, world!');
});
发送 JSON 响应:
javascript
app.get('/json', (req, res) => {
const data = { message: 'Hello, world!' };
res.json(data);
});
代码详解:
res.send('Text')
:发送文本响应。res.json({ key: 'value' })
:发送 JSON 响应。
2.2 设置响应状态码
可以通过 res.status
方法设置 HTTP 响应状态码。
示例:
javascript
app.get('/not-found', (req, res) => {
res.status(404).send('Resource not found');
});
代码详解:
res.status(404).send('Message')
:设置响应状态码为 404,并发送响应消息。
2.3 发送文件
可以通过 res.sendFile
方法发送文件作为响应。
示例:
javascript
const path = require('path');
app.get('/file', (req, res) => {
const filePath = path.join(__dirname, 'public', 'example.txt');
res.sendFile(filePath);
});
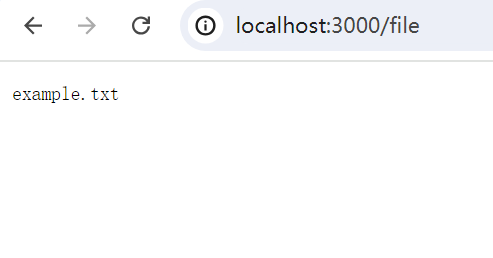
代码详解:
res.sendFile(filePath)
:发送文件作为响应。
3 处理表单数据
处理表单数据是 Web 应用程序的常见需求。通常使用 POST 请求发送表单数据,并使用中间件解析请求体。
示例:
html
<!DOCTYPE html>
<html>
<head>
<title>Form</title>
</head>
<body>
<form action="/submit-form" method="POST">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<button type="submit">Submit</button>
</form>
</body>
</html>
服务器端代码:
javascript
app.use(express.urlencoded({ extended: true }));
app.post('/submit-form', (req, res) => {
// 获取表单数据
const name = req.body.name;
res.send(`Form submitted! Name: ${name}`);
});
代码详解:
express.urlencoded({ extended: true })
:解析 URL 编码的请求体,将结果存储在req.body
。req.body.name
:访问表单数据中的name
字段。
4 使用查询参数
查询参数是 URL 中的键值对,通常用于传递额外的数据。可以通过 req.query
访问查询参数。
示例:
javascript
app.get('/search', (req, res) => {
const term = req.query.term;
const sort = req.query.sort;
res.send(`Search term: ${term}, Sort order: ${sort}`);
});
当用户访问 /search?term=express&sort=desc
时,响应内容将为 "Search term: express, Sort order: desc"。
代码详解:
req.query
:获取查询参数,返回一个对象。
5 处理文件上传
处理文件上传是许多 Web 应用程序的重要功能。可以使用 multer
中间件处理文件上传。
安装 multer
:
bash
npm install multer --save
配置 multer
:
javascript
const multer = require('multer');
const upload = multer({ dest: 'uploads/' });
app.post('/upload', upload.single('file'), (req, res) => {
const file = req.file;
res.send(`File uploaded: ${file.originalname}`);
});
代码详解:
multer({ dest: 'uploads/' })
:配置multer
,将上传的文件保存到uploads
目录。upload.single('file')
:处理单个文件上传,file
是表单字段的名称。req.file
:访问上传的文件信息。
HTML 表单示例:
html
<!DOCTYPE html>
<html>
<head>
<title>File Upload</title>
</head>
<body>
<form action="/upload" method="POST" enctype="multipart/form-data">
<label for="file">Choose file:</label>
<input type="file" id="file" name="file">
<button type="submit">Upload</button>
</form>
</body>
</html>
通过本章内容,读者应该能够理解并掌握如何在 Express 中处理 HTTP 请求和响应,包括解析请求数据、设置响应数据、处理表单数据、使用查询参数和处理文件上传。这些知识是开发功能丰富的 Web 应用程序的基础。