swtich语句:
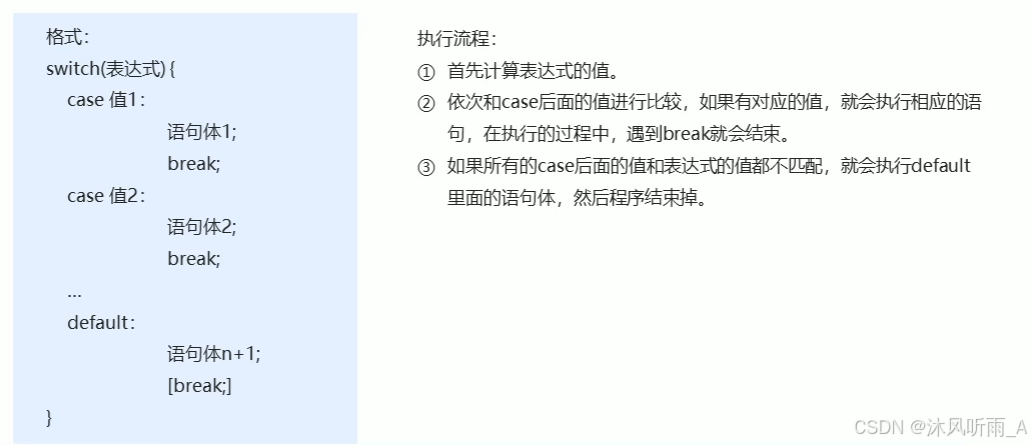
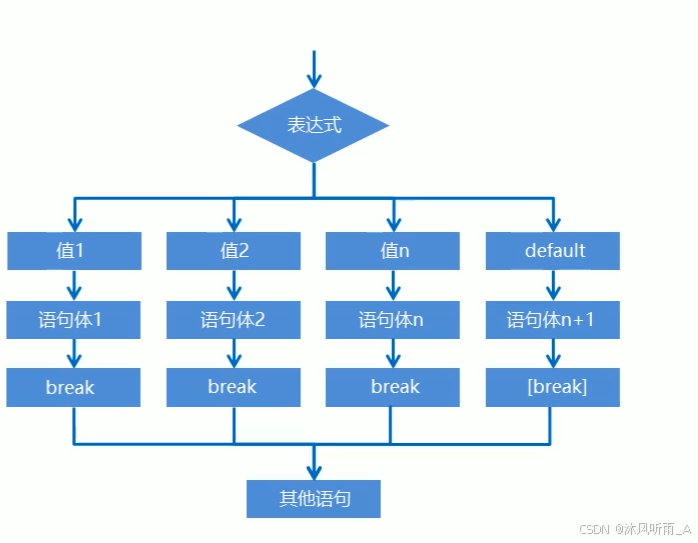
测试代码1:
java
package testswitch.com;
//根据月份和年份,当月份是 2 时,检查年份是否为闰年,然后继续执行下一个 case,打印出"三月",然后终止switch 语句。
public class MainSwitch {
public static void main(String[] args) {
int month = 2;
int year = 2024;
switch (month) {
case 1:
System.out.println("一月");
break;
case 2:
System.out.println("二月");
if (year % 4 == 0) {
System.out.println("这是闰年的二月");
}
// 注意:没有 break,将继续执行下一个 case
case 3:
System.out.println("三月");
break;
case 4:
System.out.println("四月");
break;
default:
System.out.println("其他月份");
break;
}
}
}
运行结果如下:
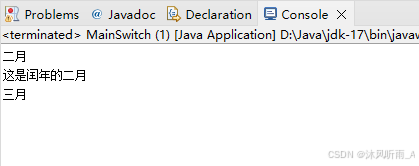
测试代码2:
java
package testswitch.com;
import java.util.Scanner;
public class LeapYearExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入年份:");
int year = scanner.nextInt();
System.out.print("请输入月份:");
int month = scanner.nextInt();
if (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) {
System.out.println(year + "年是闰年");
} else {
System.out.println(year + "年不是闰年");
}
switch (month) {
case 1:
System.out.println("一月有31天");
break;
case 2:
if (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) {
System.out.println("二月有29天");
} else {
System.out.println("二月有28天");
}
break;
case 3:
System.out.println("三月有31天");
break;
case 4:
System.out.println("四月有30天");
break;
case 5:
System.out.println("五月有31天");
break;
case 6:
System.out.println("六月有30天");
break;
case 7:
System.out.println("七月有31天");
break;
case 8:
System.out.println("八月有31天");
break;
case 9:
System.out.println("九月有30天");
break;
case 10:
System.out.println("十月有31天");
break;
case 11:
System.out.println("十一月有30天");
break;
case 12:
System.out.println("十二月有31天");
break;
default:
System.out.println("无效的月份");
break;
}
}
}
运行结果如下:
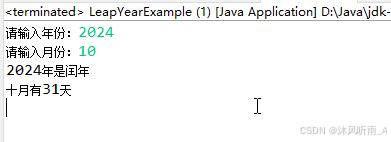
测试代码3:
java
package testswitch.com;
import java.util.Scanner;
//使用一个while循环实现选择操作。
//输入数字选择不同的操作,包括计算两个数字的和、判断一个数的奇偶性以及退出程序。
//根据选择,程序会进行相应的处理并输出结果,直到退出为止。
public class InPutSwitch {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
boolean running = true;
while (running) {
System.out.println("请选择操作:");
System.out.println("1. 计算两个数字的和");
System.out.println("2. 判断一个数的奇偶性");
System.out.println("3. 退出");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("请输入第一个数字:");
int num1 = scanner.nextInt();
System.out.println("请输入第二个数字:");
int num2 = scanner.nextInt();
int sum = num1 + num2;
System.out.println("两个数字的和为:" + sum);
break;
case 2:
System.out.println("请输入一个数字:");
int number = scanner.nextInt();
if (number % 2 == 0) {
System.out.println(number + " 是偶数");
} else {
System.out.println(number + " 是奇数");
}
break;
case 3:
running = false;
System.out.println("谢谢使用,再见!");
break;
default:
System.out.println("无效的选择,请重新输入");
}
}
}
}
运行结果如下:
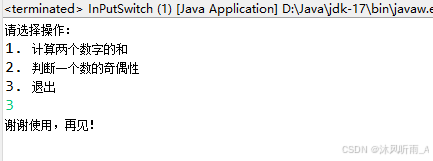
测试代码4:case穿透
java
package testswitch.com;
//case 穿透执行多个case 分支的代码块。
//当变量 day 的值为1、2或3时,它将执行与这三个 case 相关联的代码块,因为它们没有被 break 所终止。
public class SwitchTest {
public static void main(String[] args) {
int day = 3;
switch (day) {
case 1:
case 2:
case 3:
System.out.println("星期一、二或三");
break;
case 4:
case 5:
System.out.println("星期四或五");
break;
default:
System.out.println("其他");
break;
}
}
}
测试代码5:case穿透
java
package testswitch.com;
import java.util.Scanner;
//switch语句的case穿透。
public class CasePenetration{
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入月份:");
int month = scanner.nextInt();
scanner = new Scanner(System.in);
System.out.print("请输入年份:");
int year = scanner.nextInt();
switch (month) {
case 2:
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) {
System.out.println(year + "年是闰年");
} else {
System.out.println(year + "年不是闰年");
}
case 3:
System.out.println("执行月份为3的代码块");
break;
// 继续添加其它月份的case...
default:
System.out.println("输入的月份无效");
break;
}
}
}
运行结果如下:
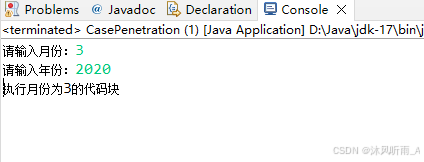