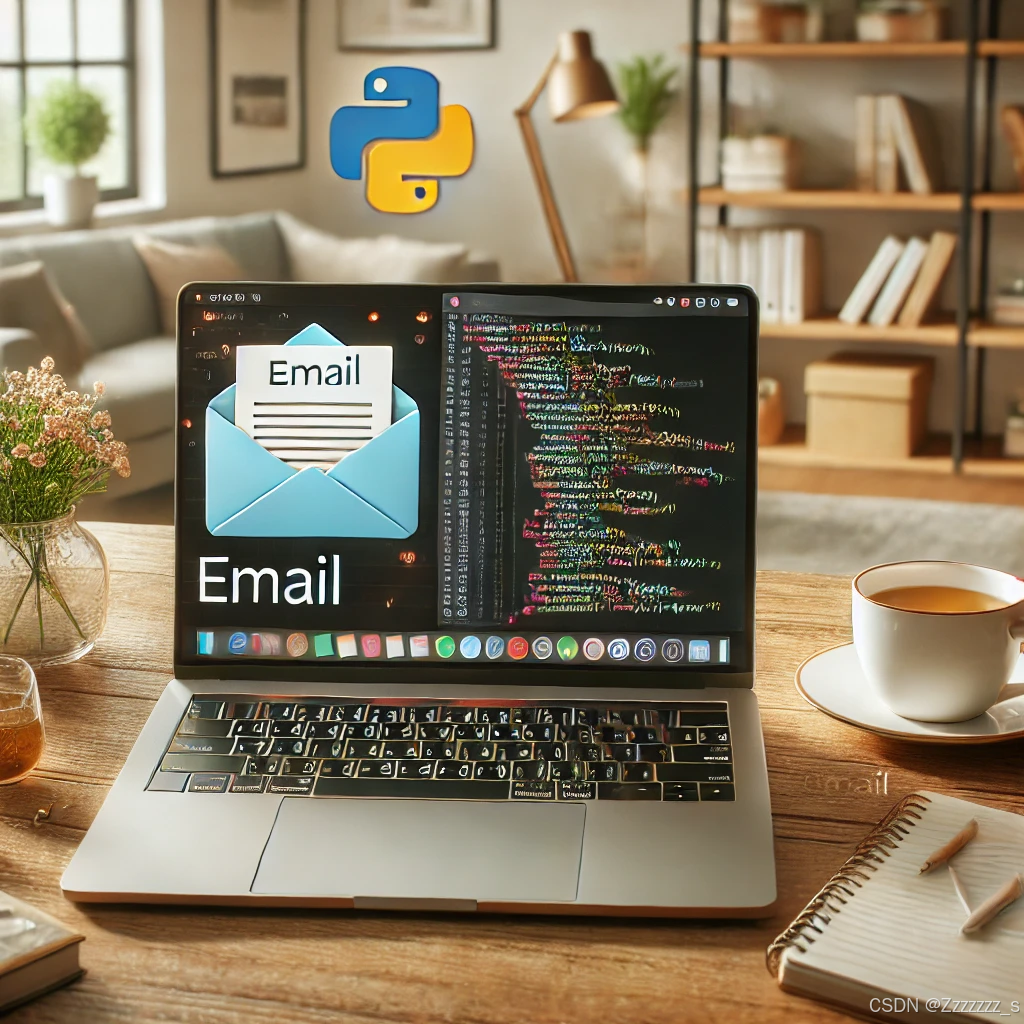
用的是qq邮箱,具体获取smtp的password可以看这个文章
获取密码
Python中发送邮件的艺术:普通邮件、PDF附件与Markdown附件
在今天的博客中,我们将探讨如何使用Python的smtplib
库来发送电子邮件,包括发送普通文本邮件、携带PDF文件的邮件和附带Markdown文件的邮件。这些功能在自动化报告发送、通知更新等多种场景中非常有用。
基础邮件发送
首先,我们从最基本的邮件发送功能开始。下面的Python脚本展示了如何使用smtplib
发送一个简单的文本邮件:
python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email(subject, body, to_email):
smtp_server = 'smtp.qq.com'
from_email = 'your_email@qq.com'
password = 'your_password'
message = MIMEMultipart()
message['From'] = from_email
message['To'] = to_email
message['Subject'] = subject
message.attach(MIMEText(body, 'plain'))
with smtplib.SMTP_SSL(smtp_server) as server:
server.login(from_email, password)
server.sendmail(from_email, to_email, message.as_string())
send_email('Hello World', 'This is the body of the email.', 'receiver@example.com')
这个脚本使用了SMTP_SSL
来确保邮件发送过程的安全性。记得替换上面代码中的邮箱地址和密码。
发送PDF附件
接下来,让我们看看如何发送一个包含PDF附件的邮件。这在发送报告或文档时特别有用:
python
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
import os
def send_email(subject, body, to_email, file_path):
smtp_server = 'smtp.qq.com'
from_email = 'your_email@qq.com'
password = 'your_password'
message = MIMEMultipart()
message['From'] = from_email
message['To'] = to_email
message['Subject'] = subject
message.attach(MIMEText(body, 'plain'))
with open(file_path, "rb") as attachment:
part = MIMEBase('application', 'pdf')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header("Content-Disposition", f"attachment; filename= {os.path.basename(file_path)}")
message.attach(part)
with smtplib.SMTP_SSL(smtp_server) as server:
server.login(from_email, password)
server.sendmail(from_email, to_email, message.as_string())
send_email('Monthly Report', 'Please find attached the monthly report.', 'receiver@example.com', 'path/to/your/report.pdf')
注意这里我们设置了附件的MIME类型为'application/pdf'
来确保正确的文件格式识别。
发送Markdown附件
最后,我们来看看如何发送一个Markdown文件作为附件的邮件。这对于发送文档或笔记非常方便:
python
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base = MIMEBase
from email import encoders
import os
def send_email(subject, body, to_email, file_path):
smtp_server = 'smtp.qq.com'
from_email = 'your_email@qq.com'
password = 'your_password'
message = MIMEMultipart()
message['From'] = from_email
message['To'] = to_email
message['Subject'] = subject
message.attach(MIMEText(body, 'plain'))
with open(file_path, "rb") as attachment:
part = MIMEBase('text', 'plain')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header("Content-Disposition", f"attachment; filename= {os.path.basename(file_path)}")
message.attach(part)
with smtplib.SMTP_SSL(smtp_server) as server:
server.login(from_email, password)
server.sendmail(from_email, to_email, message.as_string())
send_email('Documentation Update', 'Please review the attached documentation.', 'receiver@example.com', 'path/to/your/document.md')
这里,我们将MIME类型设置为'text/plain'
来匹配Markdown文件的纯文本性质。
以上就是如何在Python中使用smtplib
发送不同类型的邮件。希望这些脚本对你有所帮助!你可以根据需要进行调整以适应不同的发送需求。