目录
941、pandas.CategoricalIndex.set_categories方法
942、pandas.CategoricalIndex.as_ordered方法
943、pandas.CategoricalIndex.as_unordered方法
944、pandas.CategoricalIndex.map方法
945、pandas.CategoricalIndex.equals方法

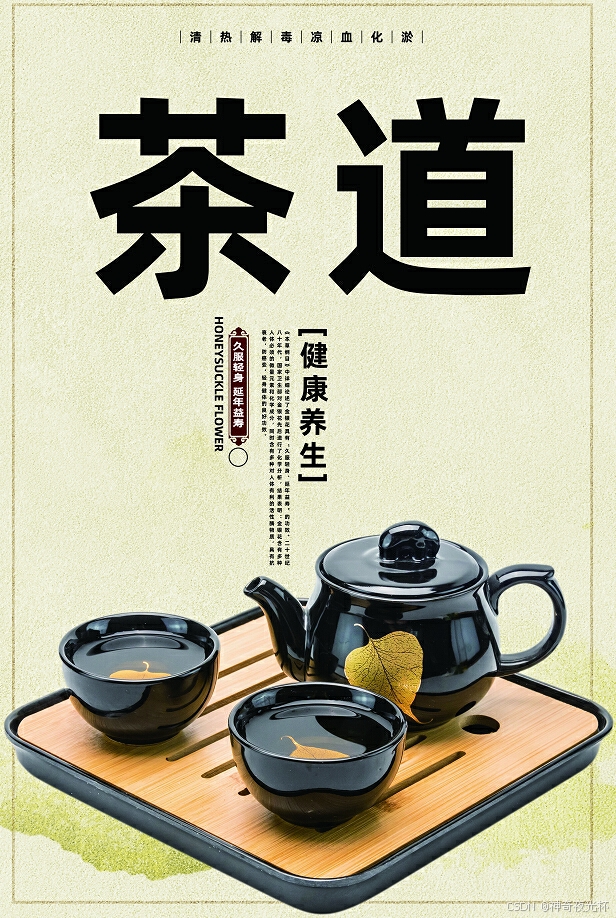

一、用法精讲
941、pandas.CategoricalIndex.set_categories方法
941-1、语法
python
# 941、pandas.CategoricalIndex.set_categories方法
pandas.CategoricalIndex.set_categories(*args, **kwargs)
Set the categories to the specified new categories.
new_categories can include new categories (which will result in unused categories) or remove old categories (which results in values set to NaN). If rename=True, the categories will simply be renamed (less or more items than in old categories will result in values set to NaN or in unused categories respectively).
This method can be used to perform more than one action of adding, removing, and reordering simultaneously and is therefore faster than performing the individual steps via the more specialised methods.
On the other hand this methods does not do checks (e.g., whether the old categories are included in the new categories on a reorder), which can result in surprising changes, for example when using special string dtypes, which does not considers a S1 string equal to a single char python string.
Parameters:
new_categories
Index-like
The categories in new order.
ordered
bool, default False
Whether or not the categorical is treated as a ordered categorical. If not given, do not change the ordered information.
rename
bool, default False
Whether or not the new_categories should be considered as a rename of the old categories or as reordered categories.
Returns:
Categorical with reordered categories.
Raises:
ValueError
If new_categories does not validate as categories
941-2、参数
941-2-1、*args**(可选)****:**其他位置参数,为后续扩展功能做预留。
941-2-2、**kwargs**(可选)****:**其他关键字参数,为后续扩展功能做预留。
941-3、功能
用于更新CategoricalIndex对象的类别(categories),可以通过指定新的类别来重新定义分类顺序,该方法主要用于修改分类索引中的类别,而不会改变数据的实际值。
941-4、返回值
返回一个新的CategoricalIndex对象,其中类别被设置为new_categories,原CategoricalIndex对象保持不变(即set_categories是不可变操作)。
941-5、说明
无
941-6、用法
941-6-1、数据准备
python
无
941-6-2、代码示例
python
# 941、pandas.CategoricalIndex.set_categories方法
import pandas as pd
# 创建一个CategoricalIndex对象
index = pd.CategoricalIndex(["low", "medium", "high", "medium"], categories=["low", "medium", "high"], ordered=True)
# 使用set_categories修改类别顺序
new_index = index.set_categories(["high", "medium", "low"])
print(new_index)
941-6-3、结果输出
python
# 941、pandas.CategoricalIndex.set_categories方法
# CategoricalIndex(['low', 'medium', 'high', 'medium'], categories=['high', 'medium', 'low'], ordered=True, dtype='category')
942、pandas.CategoricalIndex.as_ordered方法
942-1、语法
python
# 942、pandas.CategoricalIndex.as_ordered方法
pandas.CategoricalIndex.as_ordered(*args, **kwargs)
Set the Categorical to be ordered.
Returns:
Categorical
Ordered Categorical.
942-2、参数
942-2-1、*args**(可选)****:**其他位置参数,为后续扩展功能做预留。
942-2-2、**kwargs**(可选)****:**其他关键字参数,为后续扩展功能做预留。
942-3、功能
用于将CategoricalIndex对象的类别变为有序类别,对于有序类别,可以执行排序、比较、以及其他相关操作,该方法不会改变类别本身的内容,只会修改其顺序属性。
942-4、返回值
- 如果inplace=False,该方法返回一个新的CategoricalIndex对象,且该对象的类别被标记为有序。
- 如果inplace=True,则原CategoricalIndex对象会被直接修改为有序类别,且该方法没有返回值。
942-5、说明
无
942-6、用法
942-6-1、数据准备
python
无
942-6-2、代码示例
python
# 942、pandas.CategoricalIndex.as_ordered方法
import pandas as pd
# 创建一个无序的CategoricalIndex对象
index = pd.CategoricalIndex(["apple", "banana", "cherry", "banana"], categories=["apple", "banana", "cherry"], ordered=False)
# 使用as_ordered将其转换为有序的CategoricalIndex
ordered_index = index.as_ordered()
print(ordered_index)
942-6-3、结果输出
python
# 942、pandas.CategoricalIndex.as_ordered方法
# CategoricalIndex(['apple', 'banana', 'cherry', 'banana'], categories=['apple', 'banana', 'cherry'], ordered=True, dtype='category')
943、pandas.CategoricalIndex.as_unordered方法
943-1、语法
python
# 943、pandas.CategoricalIndex.as_unordered方法
pandas.CategoricalIndex.as_unordered(*args, **kwargs)
Set the Categorical to be unordered.
Returns:
Categorical
Unordered Categorical.
943-2、参数
943-2-1、*args**(可选)****:**其他位置参数,为后续扩展功能做预留。
943-2-2、**kwargs**(可选)****:**其他关键字参数,为后续扩展功能做预留。
943-3、功能
用于将分类索引转换为无序索引。
943-4、返回值
返回一个新的Index对象,该对象是原始分类索引的无序版本,新索引中的值与原始索引中的值相同,但不再具有任何分类关系或顺序。
943-5、说明
无
943-6、用法
943-6-1、数据准备
python
无
943-6-2、代码示例
python
# 943、pandas.CategoricalIndex.as_unordered方法
import pandas as pd
# 创建一个分类索引
cat_index = pd.CategoricalIndex(['A', 'B', 'C', 'A', 'B', 'C'], categories=['A', 'B', 'C'], ordered=True)
print(cat_index)
# 将分类索引转换为无序索引
unordered_index = cat_index.as_unordered()
print(unordered_index)
943-6-3、结果输出
python
# 943、pandas.CategoricalIndex.as_unordered方法
# CategoricalIndex(['A', 'B', 'C', 'A', 'B', 'C'], categories=['A', 'B', 'C'], ordered=True, dtype='category')
# CategoricalIndex(['A', 'B', 'C', 'A', 'B', 'C'], categories=['A', 'B', 'C'], ordered=False, dtype='category')
944、pandas.CategoricalIndex.map方法
944-1、语法
python
# 944、pandas.CategoricalIndex.map方法
pandas.CategoricalIndex.map(mapper, na_action=None)
Map values using input an input mapping or function.
Maps the values (their categories, not the codes) of the index to new categories. If the mapping correspondence is one-to-one the result is a CategoricalIndex which has the same order property as the original, otherwise an Index is returned.
If a dict or Series is used any unmapped category is mapped to NaN. Note that if this happens an Index will be returned.
Parameters:
mapper
function, dict, or Series
Mapping correspondence.
Returns:
pandas.CategoricalIndex or pandas.Index
Mapped index.
944-2、参数
944-2-1、mapper**(必需)****:**一个函数或字典,用于指定映射的规则,mapper会应用到每个元素上,对CategoricalIndex中的元素逐一转换。如果mapper是一个函数,那么该函数将对每个类别值进行操作;如果是字典,类别将根据字典中定义的键值对进行替换。
944-2-2、na_action**(可选,默认值为None)****:**用于指定是否跳过缺失值(NaN)的映射操作。
- **na_action=None:**对缺失值进行映射操作(即应用mapper)。
- **na_action='ignore':**忽略缺失值,不对其应用mapper。
944-3、功能
将给定的mapper应用于CategoricalIndex中的每个元素,从而生成一个新的Index,每个元素的值都经过了mapper的转换。
944-4、返回值
返回一个新的Index(不是CategoricalIndex),该Index包含了映射后的结果,返回的类型将是Index或其子类,具体取决于映射结果的类型。
944-5、说明
无
944-6、用法
944-6-1、数据准备
python
无
944-6-2、代码示例
python
# 944、pandas.CategoricalIndex.map方法
import pandas as pd
# 创建一个CategoricalIndex
cat_index = pd.CategoricalIndex(["apple", "banana", "cherry"])
# 使用map方法,传入一个函数进行映射
mapped_index = cat_index.map(lambda x: x.upper())
print(mapped_index)
944-6-3、结果输出
python
# 944、pandas.CategoricalIndex.map方法
# CategoricalIndex(['APPLE', 'BANANA', 'CHERRY'], categories=['APPLE', 'BANANA', 'CHERRY'], ordered=False, dtype='category')
945、pandas.CategoricalIndex.equals方法
945-1、语法
python
# 945、pandas.CategoricalIndex.equals方法
pandas.CategoricalIndex.equals(other)
Determine if two CategoricalIndex objects contain the same elements.
Returns:
bool
True if two pandas.CategoricalIndex objects have equal elements, False otherwise.
945-2、参数
945-2-1、other**(必需)****:**另一个Index或CategoricalIndex对象,与当前的CategoricalIndex对象进行比较。
945-3、功能
检查两个CategoricalIndex是否在类别、顺序和对应的值上完全相同,如果两个CategoricalIndex对象的类别顺序、分类标签的类别,以及是否有相同的缺失值等都一致,则返回True;否则返回False。
945-4、返回值
返回一个布尔值,如果两个CategoricalIndex相等,返回True;否则返回False。
945-5、说明
无
945-6、用法
945-6-1、数据准备
python
无
945-6-2、代码示例
python
# 945、pandas.CategoricalIndex.equals方法
import pandas as pd
# 创建三个CategoricalIndex
cat_index1 = pd.CategoricalIndex(["apple", "banana", "cherry"], categories=["apple", "banana", "cherry"], ordered=True)
cat_index2 = pd.CategoricalIndex(["apple", "banana", "cherry"], categories=["apple", "banana", "cherry"], ordered=True)
cat_index3 = pd.CategoricalIndex(["apple", "banana", "cherry"], categories=["cherry", "banana", "apple"], ordered=True)
# 比较两个相同的CategoricalIndex
print(cat_index1.equals(cat_index2))
# 比较两个不同的CategoricalIndex
print(cat_index1.equals(cat_index3))
945-6-3、结果输出
python
# 945、pandas.CategoricalIndex.equals方法
# True
# False