请求网络获取数据
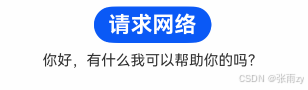
- 点击按钮发送一个post请求,发送一条string
- 由于此处的返回result.data本身就是一个string,因此不需要转换类型
ts
Button('请求网络')
.margin({ top: 10 })
.fontSize(24)
.fontWeight(FontWeight.Bold)
.onClick(() => {
httpRequestPost('http://test.xxx', "你好")
.then((result: ResponseResult) => {
console.log("msg=" + result.msg)
console.log("data=" + result.data)
this.message = result.data.toString()
})
})
- 如果需要类型转换,可以使用as转换
- result.data as ResponseToken
ts
httpRequestGet('http://test.xxx')
.then((result: ResponseResult) => {
let data = result.data as ResponseToken
console.log("msg=" + result.msg)
console.log("token=" + data.access_token)
})
ts
export class ResponseToken {
access_token: string = "";
expires_in: string = "";
expires_date: string = "";
}
封装一个HttpUtil
- 对外开放一个httpRequestGet和httpRequestPost,用于get和post请求
ts
import { http } from '@kit.NetworkKit';
import ResponseResult from './ResponseResult';
/**
* Initiate an HTTP GET request to the specified URL.
*/
export function httpRequestGet(url: string) {
return httpRequest(url, http.RequestMethod.GET);
}
/**
* Initiate an HTTP POST request to the specified URL.
*/
export function httpRequestPost(url: string, newsData: string) {
return httpRequest(url, http.RequestMethod.POST, newsData);
}
/**
* Initiates an HTTP request to a given URL.
*
* @param url URL for initiating an HTTP request
* @param method Request method.
* @param extraData Additional data of the request.
* @returns Returns {@link ResponseResult}.
*/
function httpRequest(url: string, method: http.RequestMethod, params?: string): Promise<ResponseResult> {
let httpRequest = http.createHttp();
let responseResult = httpRequest.request(url, {
method: method,
header: {
'Content-Type': 'application/json'
},
extraData: params
});
let serverData = new ResponseResult();
// Processes the data and returns.
return responseResult.then((value: http.HttpResponse) => {
if (value.responseCode === 200) {
// Obtains the returned data.
let result = `${value.result}`;
let resultJson: ResponseResult = JSON.parse(result);
if (resultJson.code === '000000') {
serverData.data = resultJson.data;
}
serverData.code = resultJson.code;
serverData.msg = resultJson.msg;
} else {
serverData.msg = `Network request failed, please try later!&${value.responseCode}`;
}
return serverData;
}).catch(() => {
serverData.msg = 'Network request failed, please try later!';
return serverData;
});
}
- ResponseResult为服务端返回的基本结构
ts
export default class ResponseResult {
/**
* Code returned by the network request: success, fail.
*/
code: string;
/**
* Message returned by the network request.
*/
msg: string | Resource;
/**
* Data returned by the network request.
*/
data: string | Object | ArrayBuffer;
constructor() {
this.code = '';
this.msg = '';
this.data = '';
}
}