解题思路
由于三种国家都有获胜的可能,所以我们需要分别枚举 X , Y , Z X,Y,Z X,Y,Z 获胜的情况。
设 X X X 获胜,那么对于第 i i i 个事件的贡献为 a [ i ] − ( b [ i ] + c [ i ] ) a[i]-(b[i]+c[i]) a[i]−(b[i]+c[i]),根据贪心的策略,我们可以考虑将所有时间按照贡献进行排序,贡献越大则优先选择。
Y , Z Y,Z Y,Z 获胜同理。
注意
- X X X 的获胜条件为 X > Y + Z X > Y + Z X>Y+Z,非 X ≥ Y + Z X \geq Y + Z X≥Y+Z。
- 无获胜国时,输出
-1
。
AC_Code
cpp
#include <iostream>
#include <cstring>
#include <algorithm>
#include <cstdio>
using namespace std;
typedef long long LL;
const int N = 1e5 + 10;
int n;
int a[N], b[N], c[N], d[N];
int get(int a[], int b[], int c[])
{
for (int i = 0; i < n; ++ i )
d[i] = a[i] - b[i] - c[i];
sort(d, d + n, greater<int>());
LL res = 0;
for (int i = 0; i < n; ++ i )
{
res += d[i];
if (res <= 0)
return i;
}
return n;
}
int main()
{
cin >> n;
for (int i = 0; i < n; ++ i )
cin >> a[i];
for (int i = 0; i < n; ++ i )
cin >> b[i];
for (int i = 0; i < n; ++ i )
cin >> c[i];
int res = max({get(a, b, c), get(b, a, c), get(c, a, b)});
if (res == 0)
res = -1;
cout << res << endl;
return 0;
}
cpp
#include <iostream>
#include <cstring>
#include <algorithm>
using namespace std;
typedef long long LL;
const int N = 1e5 + 10;
int n;
struct Node
{
int x, y, z;
}q[N];
bool cmp_x(Node a, Node b)
{
return a.x - a.y - a.z > b.x - b.y - b.z;
}
bool cmp_y(Node a, Node b)
{
return a.y - a.x - a.z > b.y - b.x - b.z;
}
bool cmp_z(Node a, Node b)
{
return a.z - a.x - a.y > b.z - b.x - b.y;
}
int main()
{
cin >> n;
for (int i = 0; i < n; ++ i )
cin >> q[i].x;
for (int i = 0; i < n; ++ i )
cin >> q[i].y;
for (int i = 0; i < n; ++ i )
cin >> q[i].z;
LL res = -1, sum = 0, t = 0;
sort(q, q + n, cmp_x);
for (int i = 0; i < n; ++ i )
{
sum += q[i].x - q[i].y - q[i].z;
if (sum <= 0)
break;
t ++;
}
res = max(res, t);
sum = 0, t = 0;
sort(q, q + n, cmp_y);
for (int i = 0; i < n; ++ i )
{
sum += q[i].y - q[i].x - q[i].z;
if (sum <= 0)
break;
t ++;
}
res = max(res, t);
sum = 0, t = 0;
sort(q, q + n, cmp_z);
for (int i = 0; i < n; ++ i )
{
sum += q[i].z - q[i].x - q[i].y;
if (sum <= 0)
break;
t ++;
}
res = max(res, t);
cout << (!res? -1: res) << endl;
return 0;
}
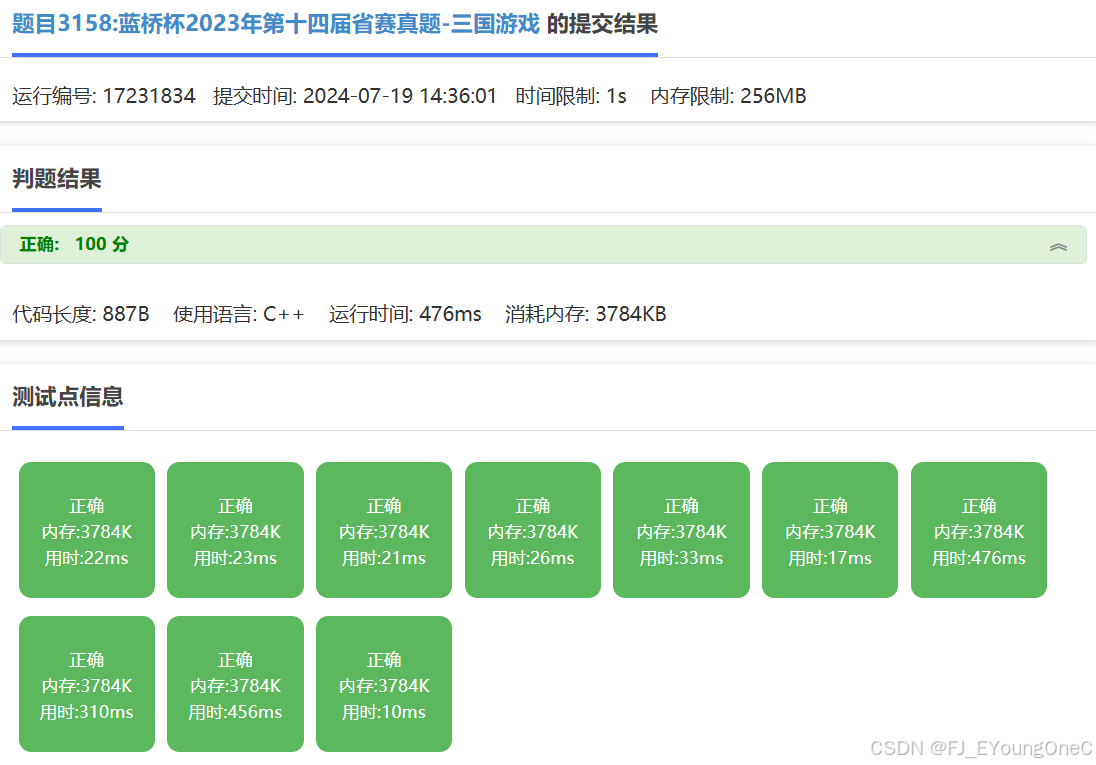