问题的发现
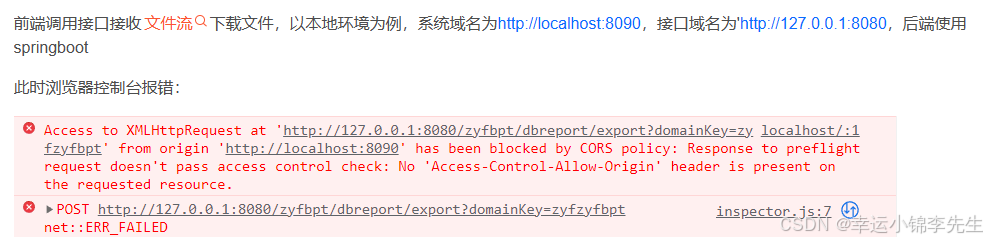
一. 配置vite的vite.config.js
1.首先找到该文件的位置(在最下面)
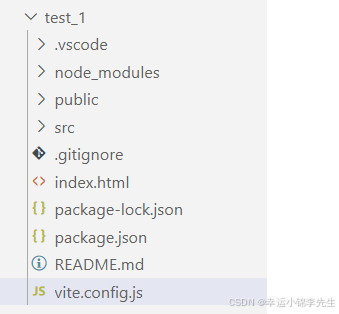
2.填入代码
2.1配置所有路径都可跨域访问
// vite.config.js
import { defineConfig } from 'vite';
export default defineConfig({
server: {
proxy: {
// 匹配所有路径,但请注意,这可能会带来安全风险
// 并且会覆盖所有直接访问的静态资源,除非你有特别的规则来处理它们
'/': {
target: 'http://your-backend-server.com', // 你的后端服务器地址
changeOrigin: true,
rewrite: (path) => path.startsWith('/') ? path : `/${path}` // 根据需要调整重写规则
},
},
},
// 其他配置...
});
2.2只是配置单一路径(只有开头为api才能进行跨域)
// vite.config.js
import { defineConfig } from 'vite';
export default defineConfig({
// ... 其他配置
server: {
// 配置代理
proxy: {
// '/api' 是你希望代理的前缀,你可以根据实际需要修改它
'/api': {
// target 是你的后端 API 服务器的地址
target: 'http://your-backend-server.com',
// changeOrigin 设置为 true,表示在发送请求时会自动改变原始主机头为目标 URL
changeOrigin: true,
// rewrite 是一个函数,用于重写请求的 URL 路径
// 在这个例子中,我们移除了路径中的 '/api' 前缀
rewrite: (path) => path.replace(/^\/api/, ''),
// 你可以根据需要添加更多的配置选项,如 headers、cookieDomainRewrite 等
},
// 你可以添加更多的代理规则,每个规则都映射到一个不同的后端服务
},
},
// ... 其他配置
});
二. 配置idea端(基于springboot'项目)
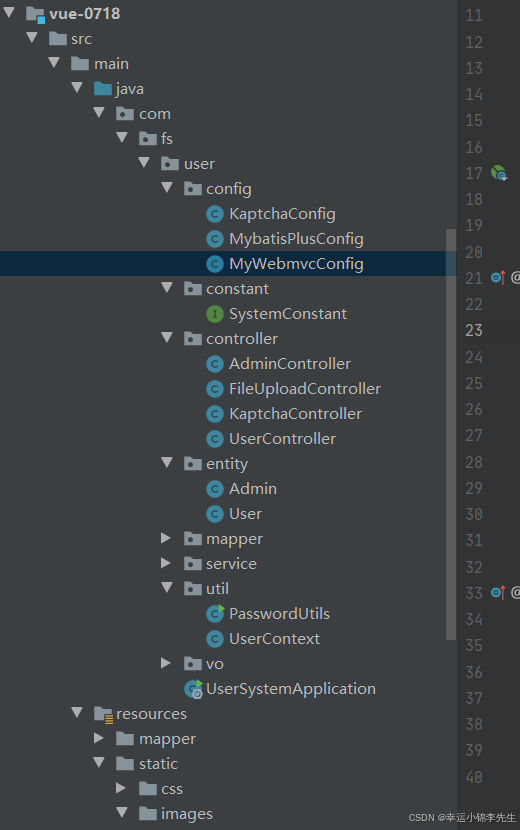
package com.fs.user.config;
import com.fs.user.constant.SystemConstant;
import org.springframework.boot.SpringBootConfiguration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
/**
* @author suke
* @version 1.0
* @title MyWebmvcConfig
* @description
* @create 2024/7/6 16:08
*/
@SpringBootConfiguration
public class MyWebmvcConfig implements WebMvcConfigurer {
//解决跨域
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**") // 允许跨域访问的路径
.allowedOrigins("*") // 允许跨域访问的源
.allowedMethods("POST", "GET", "PUT", "OPTIONS", "DELETE") // 允许请求方法
.maxAge(168000) // 预检间隔时间
.allowedHeaders("*") // 允许头部设置
.allowCredentials(true); // 是否发送cookie
}
//资源处理器
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
//给文件系统某个目录绑定一个url,
//如果不加file: 表示从项目中找 /img/1.jpg --> file: D:/upload/user/1.jpg
//file: 从服务器本地文件系统查找
registry.addResourceHandler("/img/**").addResourceLocations("file:"+ SystemConstant.IMAGE_SAVE_PATH+"/");
}
}
三.封装axios的工具
1.安装qs
axios安装
npm instal axios
# npm安装
npm install qs
2.在src目录下创建一个utils目录,用于存放一些工具的js文件, 在这个目录下我们创建一个request.js用于封装axios
import axios from 'axios'
import qs from 'qs'
/**
* axios的传参方式:
* 1.url 传参 一般用于Get和Delete 实现方式:config.params={JSON}
* 2.body传参 实现方式:config.data = {JSON},且请求头为:headers: { 'Content-Type': 'application/json;charset=UTF-8' }
* 3.表单传参 实现方式:config.data = qs.stringify({JSON}),且请求头为:且请求头为:headers: { 'Content-Type': 'application/x-www-form-urlencoded;charset=UTF-8' }
*/
// axios实例
const $http = axios.create({
baseURL: '',
timeout: 60000,
headers: { 'Content-Type': 'application/json;charset=UTF-8' }
})
// 请求拦截器
$http.interceptors.request.use(
(config) => {
// 追加时间戳,防止GET请求缓存
if (config.method?.toUpperCase() === 'GET') {
config.params = { ...config.params, t: new Date().getTime() }
}
if (Object.values(config.headers).includes('application/x-www-form-urlencoded')) {
config.data = qs.stringify(config.data)
}
return config
},
error => {
return Promise.reject(error)
}
)
// 响应拦截器
$http.interceptors.response.use(
response => {
const res = response.data
return res
},
error => {
return Promise.reject(error)
}
)
// 导出 axios 实例
export default $http
3.在main.js中,把$http绑定到app对象上
// 导入封装好的axios并挂载到Vue全局属性上
import $http from './utils/request'
app.config.globalProperties.$http = $http
使用:
methods: {
sendAjax(){
this.$http.get("https://autumnfish.cn/cloudsearch?keywords=" + this.query)
.then(function(response) {
console.log(response)
}, function(err) {});
}
},
4.axios的配置文件
//axios的配置文件
export default {
method: 'get',
// 基础url前缀
baseUrl: 'http://localhost:8080',
// 请求头信息
headers: {
//默认的请求context-type: application/json
'Content-Type': 'application/json;charset=UTF-8'
},
// 参数
data: {},
// 设置超时时间
timeout: 10000,
// 携带凭证 是否携带cookie
withCredentials: true,
// 返回数据类型
responseType: 'json'
}