一、低难度(刷新a标签)
1、需求
给a标签每15s刷新一次,显示最新的时间(时间必须由后端获取) 应该如何操作呢
2、代码
后端
cs
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using Microsoft.AspNetCore.Mvc.Rendering;
using WebApplication1.Models;
namespace WebApplication1.Controllers
{
public class MainController : Controller
{
public IActionResult Index()
{
var currentTime = DateTime.Now.ToString("HH:mm:ss");
ViewBag.CurrentTime = currentTime;
return View(); // 当前时间传给对应的视图(/Views/Main/Index)
}
// Action 用于获取最新时间的方法
public IActionResult GetCurrentTime()
{
var currentTime = DateTime.Now.ToString("HH:mm:ss");
return Content(currentTime); // 直接返回字符串
}
}
}
前端
html
@{
var now = ViewBag.CurrentTime; // 初始化获取时间
}
<h1>测试页面</h1>
<a id="showtime">当前的时间是:@now</a> <!-- Razor 语法中输出变量值的正确方式 -->
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
// 定义一个函数来更新时间显示
function updateTime() {
// 使用 Ajax 请求获取最新时间
$.ajax({
url: '@Url.Action("GetCurrentTime", "Main")', // ajax发送get请求到Main控制器下的GetCurrentTime方法中
type: 'GET',
success: function (data) {
$('#showtime').text('当前的时间是:' + data);
},
error: function () {
console.log('无法获取最新时间');
}
});
}
// 页面加载完成后立即执行一次,并且每隔15秒执行一次
$(document).ready(function () {
updateTime(); // 初始化页面加载时获取一次最新时间
setInterval(updateTime, 15000); // 每15秒调用一次该方法
});
</script>
二、中难度(刷新表格table)
1.需求
每隔十秒更新一下table里面的数据
2.代码
后端:
cs
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using Microsoft.AspNetCore.Mvc.Rendering;
using WebApplication1.Models;
namespace WebApplication1.Controllers
{
public class MainController : Controller
{
public class Student
{
public int Id { get; set; }
public string Name { get; set; }
}
public IActionResult Index()
{
List<Student> list = new List<Student>();
list.Add(new Student { Id=1,Name="小虹"});
list.Add(new Student { Id = 2, Name = "小美" });
list.Add(new Student { Id = 3, Name = "小刚" });
ViewData["Student"] = list;
return View();
}
public IActionResult GetStudentList()
{
Random rnd = new Random();
int randomNumber = rnd.Next(1, 101); // 生成一个1到100之间的随机整数
int randomNumber2 = rnd.Next(1, 101); // 生成一个1到100之间的随机整数
int randomNumber3 = rnd.Next(1, 101); // 生成一个1到100之间的随机整数
List<Student> list = new List<Student>
{
new Student { Id = 1, Name = $"小虹{randomNumber}" },
new Student { Id = 2, Name = $"小美{randomNumber2}" },
new Student { Id = 3, Name = $"小刚{randomNumber3}" }
};
return Json(list);
}
}
}
前端:
html
@using static WebApplication1.Controllers.MainController
@{
var list = ViewData["Student"] as List<Student>;
}
<h1>学生列表</h1>
<table class="table" id="studentTable">
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
</tr>
</thead>
<tbody>
@foreach (var student in list)
{
<tr>
<td>@student.Id</td>
<td>@student.Name</td>
</tr>
}
</tbody>
</table>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
function updateStudentTable() {
// 使用 AJAX 请求更新数据
$.ajax({
url: '@Url.Action("GetStudentList", "Main")', // 调用Get请求,访问后端的Main控制器的GetStudentList方法
type: 'GET',
success: function (data) {
// 清空表格内容
$('#studentTable tbody').empty();
// 遍历新数据并添加到表格中
data.forEach(function (student) {
$('#studentTable tbody').append(`
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
</tr>
`);
});
}
});
}
// 初始化页面加载数据
//updateStudentTable();
// 定时器,每隔10秒调用一次方法
setInterval(updateStudentTable, 10000); // 10秒间隔
</script>
三、注意事项
不要忘记引用Jquery库
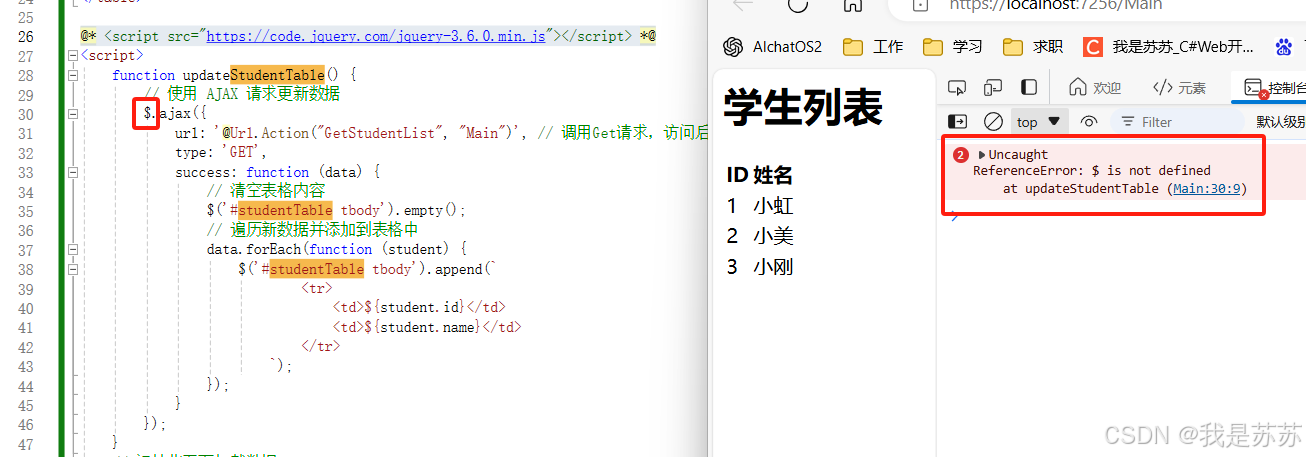
html
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>