1.函数介绍
1.1 strlen
cs
size_t(就是无符号整形) strlen(const char * str);
字符串已经'\0'作为结束标志,strlen函数返回的是在字符串中'\0'前面出现的字符个数(不包
含'\0')
参数指向的字符串必须要以\0结束。
注意函数的返回值为size_t,是无符号的(易错 ) 不能相减
cs
strlen函数模拟
int my_strlen(char* arr)
{
assert(arr);
int counst = 0;
while (*arr != '\0')
{
counst++;
arr++;
}
return counst;
}
int main()
{
char arr[] = "abcdef";
int len = my_strlen(arr);
printf("%d", len);
return;
}
1.2 strcpy
cs
char * strcpy (char * destination,const char * source)
Copies the C string pointed by source into the array pointed by destination, including the terminating null character (and stopping at that point).
源字符串必须以'\0'结束。
会将源字符串中的'0'拷贝到目标空间。
目标空间必须足够大,以确保能存放源字符串。
目标空间必须可变。
cs
//strcpy函数模拟
void my_strcpy(char*arr2, const char* arr1)
{
assert(arr1);
assert(arr2);
while (*arr1 != '\0')
{
*arr2++ =*arr1++;
}
*arr2 = *arr1;//复制\0;
}
int main()
{
char arr1[] = "abcdef";
char arr2[20] = { 0 };
my_strcpy(arr2, arr1);
printf("%s",arr2);
return 0;
}
1.3 strcat
字符串追加
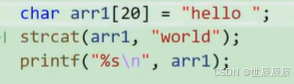
cs
char * strcat (char * destination,const char * source)
Appends a copy of the source string to the destination string. The terminating null characterin destination is overwritten by the first character of source, and a null-character is includedat the end of the new string formed by the concatenation of both in destination.
源字符串必须以'\0'结束。
目标空间必须有足够的大,能容纳下源字符串的内容。
目标空间必须可修改。
字符串自己给自己追加,如何? 不能
cs
//模拟strcat
char* my_strcat(char* dest, const char* src)
{
char* ret = dest;
assert(dest && src);
while (*dest != '\0')
{
dest++;
}
while (*dest++=*src++)
{
;
}
return ret;
}
int main()
{
char arr1[20] = "hello ";
my_strcat(arr1,"world");
printf("%s\n",arr1);
return 0;
}
1.4 strcmp
比内容不是比长度
cs
int strcmp(const char*str1,const char*str2);
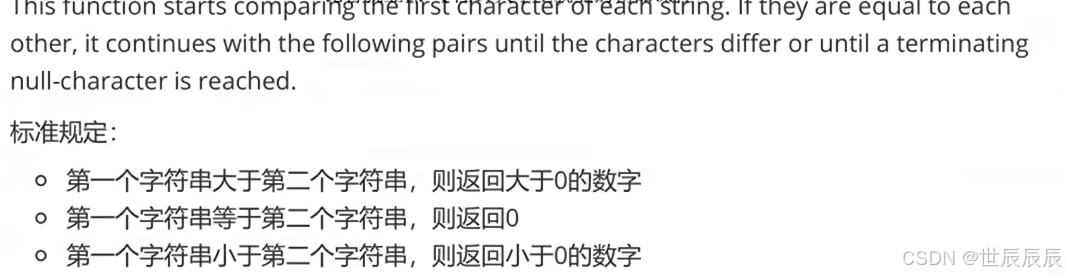
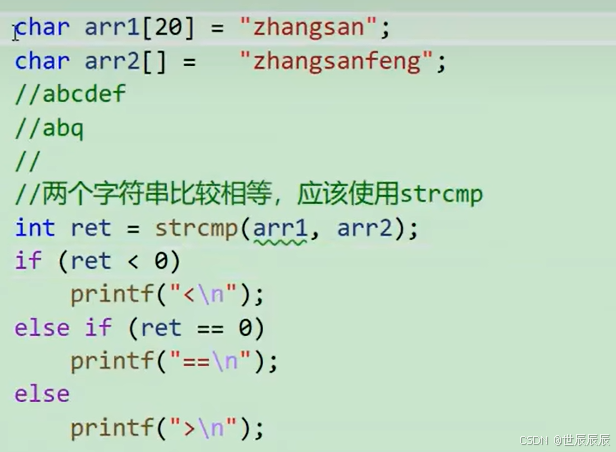
cs
//模拟strcmp
int my_strcmp(const char*str1,const char*str2)
{
assert(*str1&&*str2);
while (*str1==*str2)
{
if (*str1 == '\0')
return 0;
str1++;
str2++;
}
if (*str1 > *str2)
return 1;
else
return -1;
//也可以写成return(*str1-*str2);
}
int main()
{
char arr1[20] = "zhangsan";
char arr2[] = "zhangsanfeng";
int ret = my_strcmp(arr1,arr2);
if (ret < 0)
printf("<\n");
else if (ret == 0)
printf("==\n");
else
printf(">n");
return 0;
}
增加长度限制
strncmp 加了一个参数n
strncat
strncpy
1.5 strstr
查找子串的一个函数
cs
const char *strstr (const char *str1,const char *str2);
char *strstr ( char *str1,const char *str2 )
找到了返回子串的地址
找不到返回空指针
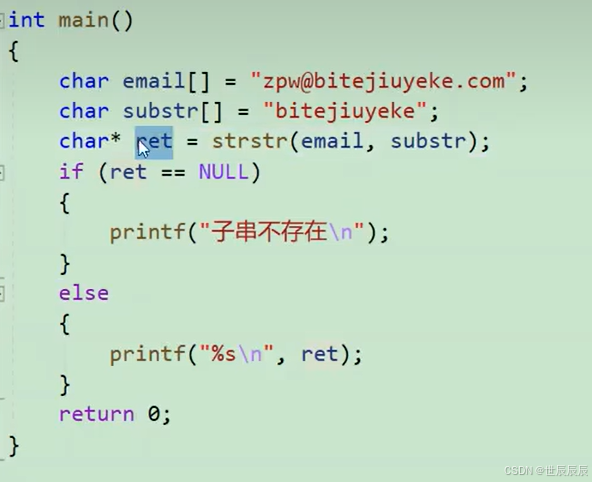
cs
模拟strstr
char * my_strstr(char* str1, const char* str2)
{
assert(str1 && str2);
const char* s1 = str1;
const char* s2 = str2;
const char* p = str1;
while (*p)
{
s1 = p;
s2 = str2;
while (*s1!='\0'&&*s2!='\0'&& * s1 == *s2)
{
s1++;
s2++;
}
if (*s2 == '\0')
{
return (char*)p;
}
p++;
}
return NULL;
}
int main()
{
char email[] = "[email protected]";
char substr[] = "bitejiuyeke";
char* ret = my_strstr(email, substr);
if (ret == NULL)
{
printf("字串不存在\n");
}
else
{
printf("%s",ret);
}
return 0;
}
KMP算法比较高效
1.6 strtok
用来切割字符串
cs
char *strtok(char*str,const char *sep)
sep参数是个字符串!定义了用作分隔符的字符集合
第一个参数指定一个字符串,
它包含了0个或者多个由sep字符串中一个或者多个分隔符分割的标记。
strtok函数找到str中的下一个标记,并将其用\0结尾,返回一个指向这个标记的指针。
(注strtok函数会改变被操作的字符串,所以在使用strtok函数切分的字符串一般都是临时拷贝的内容并且可修改。)
strtok函数的第一个参数不为 NULL,函数将找到str中第一个标记,strtok函数将保存它在字符串中的位置。
strtok函数的第一个参数为NULL,函数将在同一个字符串中被保存的位置开始,查找下一个标记。
如果字符串中不存在更多的标记,,则返回 NULL指针。
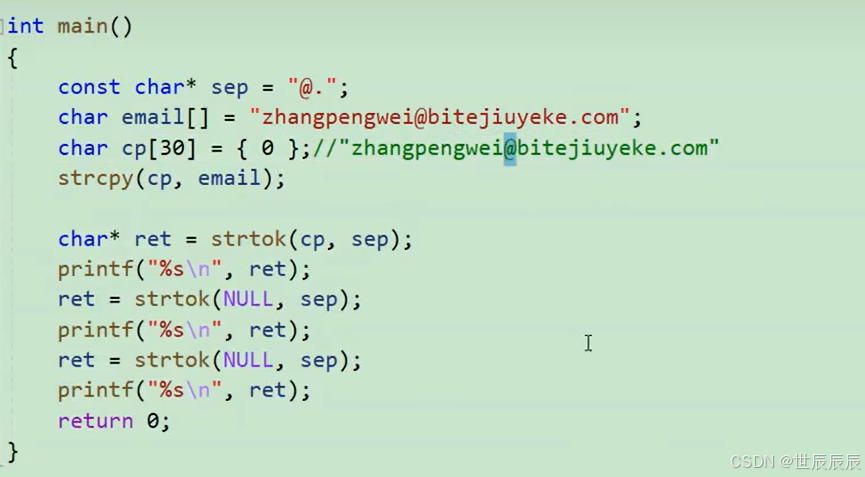
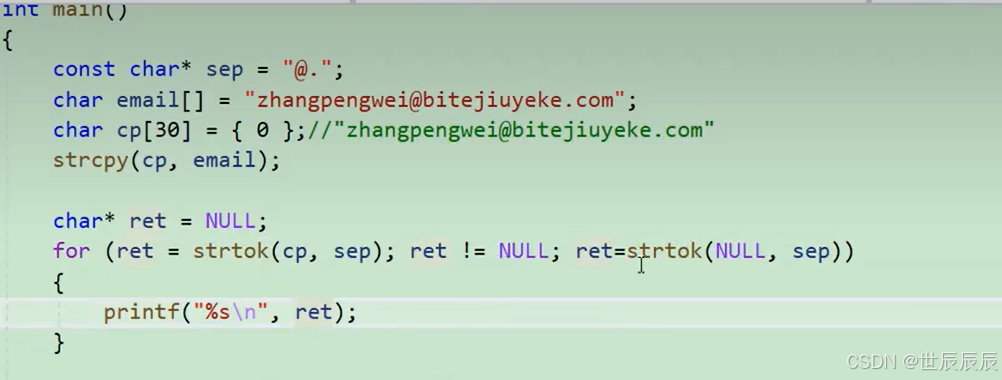
1.7 strerror
返回错误码所对应的信息
cs
char * strerror
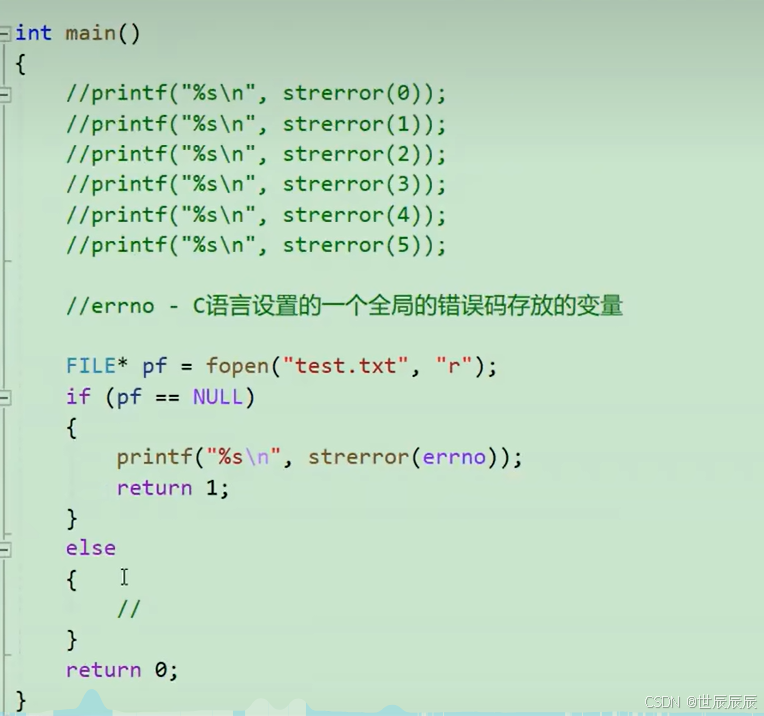
字符分类函数
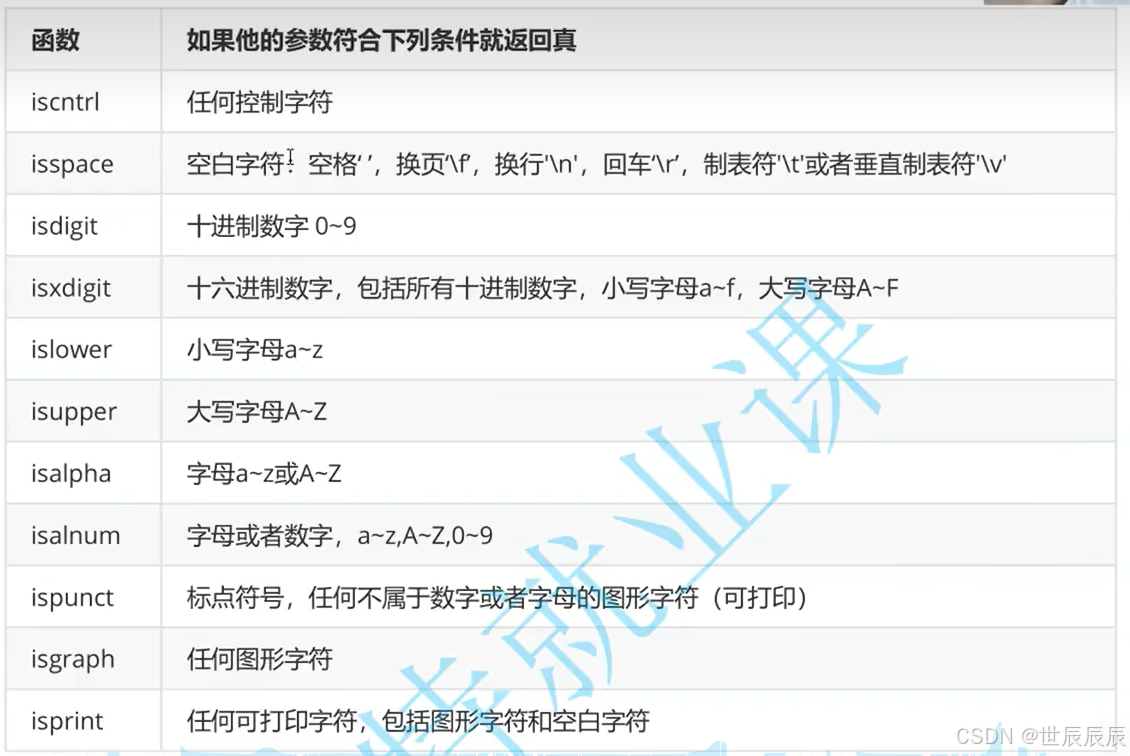
字符转换函数
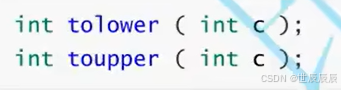
1.8 memcpy
内存拷贝函数,负责两块独立空间中的数据
cs
void*memcpy(void * destination,const void * source ,size_t num);
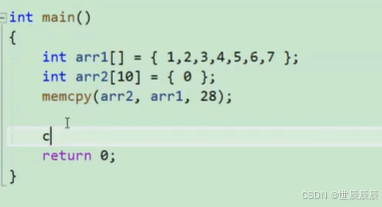
cs
//模拟memcpy
void* my_memcpy(void *dest,const void *src,size_t num)
{
assert(dest && src);
void* ret = dest;
while (num--)
{
*(char*)dest = *(char*)src;
dest = (char*)dest + 1;
src = (char*)src + 1;
}
return ret;
}
int main()
{
int arr1[] = {1,2,3,4,5,6,7};
int arr2[10] = {0};
my_memcpy(arr2,arr1,28);
return 0;
}
1.9 memmove
cs
void*memmove(void * destination,const void * source ,size_t num);
和memcpy的差别就是memmove函数处理的源内存块和目标内存块是可以重叠的
如果源空间和目标空间出现重叠,就得使用memmove函数处理。
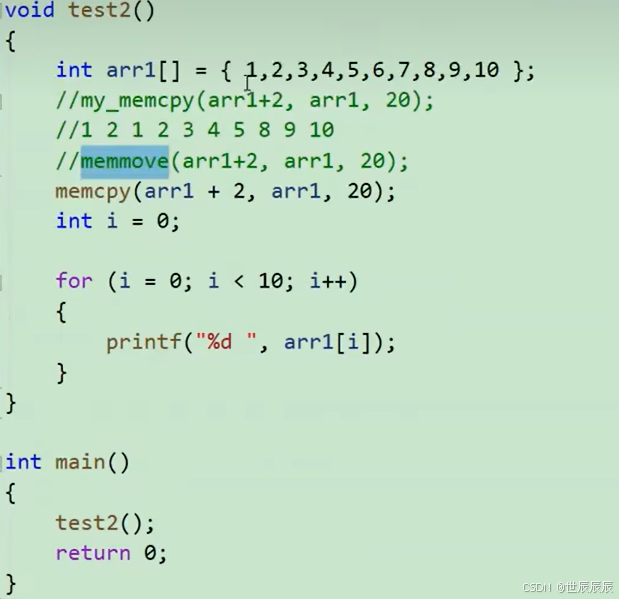
cs
//模拟memmove
void* my_memmove(void *dest,const void *src,size_t num)
{
void* ret = dest;
if (dest <src)
{
//从前向后
while (num--)
{
*(char*)dest = *(char* )src;
dest = (char*)dest + 1;
src = (char*)src + 1;
}
}
else
{
//从后向前
while (num--)
{
*((char*)dest + num) = *((char*)src + num);
}
}
//有时候需要从前往后,有时候从后往前处理数据
return ret;
}
int main()
{
int arr1[] = {1,2,3,4,5,6,7,8,9,10};
my_memmove(arr1+2,arr1,20);
int i = 0;
for (i = 0; i < 10; i++)
{
printf("%d",arr1[i]);
}
return 0;
}
1.10 memcmp
cs
int memcmp(const void * ptr1,const void * ptr2,size_t num);
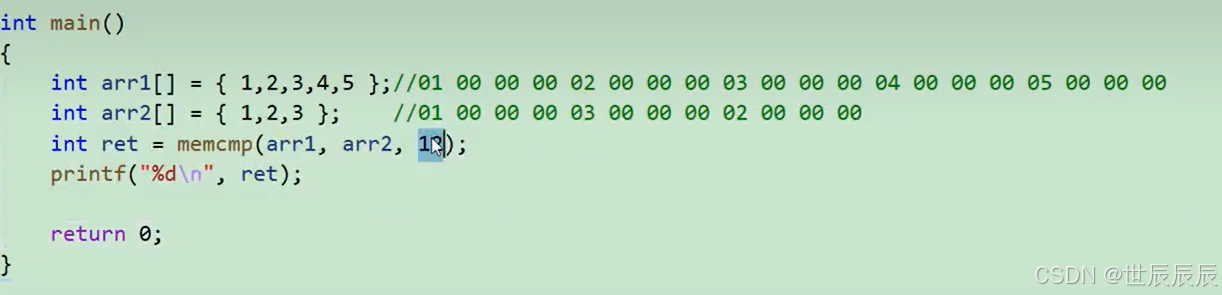
1.11 memset
cs
void * memset(void *ptr,int value,size_t num);
以字节为单位修改,不能初始化数组;
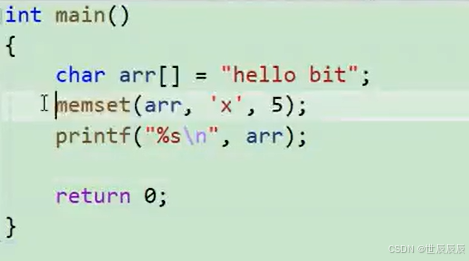