思路
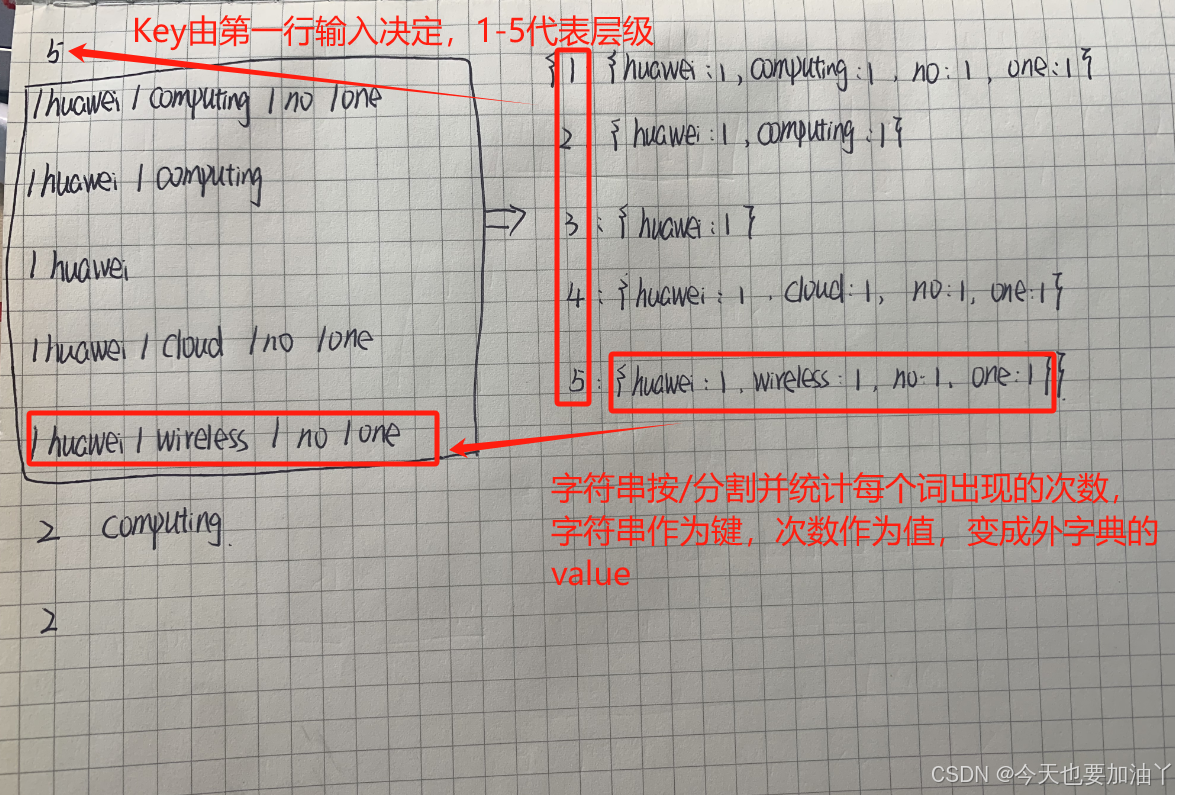
统计第二层级上computing出现的次数的时候,只需要for循环寻找computing是否在字典的键中。
如果找到,计数的时候是加上computing对应的值
python
def main():
import collections
length_char = int(input())
node_info = {}
for i in range(length_char):
char_list = [s for s in input().split('/') if s]
char_dict = collections.Counter(char_list)
node_info[i+1] = char_dict
node = int(input())
target_str = input()
target_count = 0
for i in range(node):
temp = node_info[i+1]
if target_str in temp:
target_count += temp[target_str]
print(target_count)
if __name__ == "__main__":
main()
代码功能概述
这个程序的目的是分析一组输入的字符并计算某个目标字符在特定节点中出现的次数。
逐步解析代码
-
导入库:
pythonimport collections
这行代码导入了
collections
模块,主要用来使用Counter
类,它可以用来计数对象出现的次数。 -
主函数定义:
pythondef main():
这是主函数定义,程序的执行从这里开始。
-
输入字符数量:
pythonlength_char = int(input())
这一行从用户输入获取一个整数,表示接下来将输入的字符组的数量(节点的数量)。
-
初始化字典:
pythonnode_info = {}
创建一个空字典
node_info
,用于存储每个节点的字符计数信息。 -
循环输入并处理字符:
pythonfor i in range(length_char): char_list = [s for s in input().split('/') if s] char_dict = collections.Counter(char_list) node_info[i+1] = char_dict
- 在这个循环中,根据
length_char
的数量,逐行读取输入。 - 使用
input().split('/')
方法把输入的字符串按斜杠(/
)分割成字符列表。 - 过滤掉空字符串。
- 使用
collections.Counter
创建一个字典char_dict
,该字典记录每个字符出现的次数。 node_info[i+1] = char_dict
把每个节点的字符计数存入node_info
字典,键是节点编号(从1开始)。
- 在这个循环中,根据
-
输入节点数量:
pythonnode = int(input())
获取用户输入的一个整数,表示需要检查的节点数量。
-
输入目标字符:
pythontarget_str = input()
获取用户输入的一个字符串,即需要计算出现次数的目标字符。
-
统计目标字符的次数:
pythontarget_count = 0 for i in range(node): temp = node_info[i+1] if target_str in temp: target_count += temp[target_str]
- 初始化计数器
target_count
为 0。 - 遍历每个节点,检查
node_info
中相应节点所存储的字符计数(temp
)。 - 如果
target_str
在当前节点的计数字典中,则将target_count
加 temp[target_str]。
- 初始化计数器
-
输出结果:
pythonprint(target_count)
打印最终统计的目标字符出现的节点数量。
-
程序入口:
pythonif __name__ == "__main__": main()
这一行确保只有在直接运行此文件时才执行主函数
main
。