JSON数据交换
规则
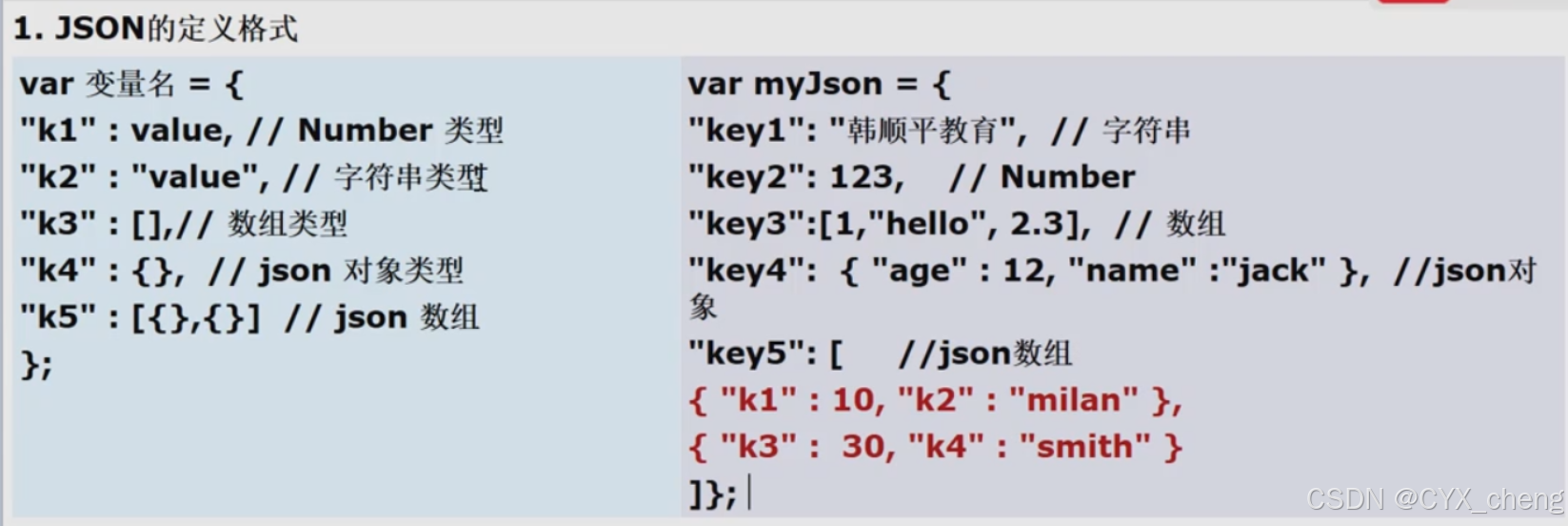
JSON对象和字符串转换

javascript
<script type="text/javascript">
var jsonPerson = {
"name": "jack",
"age": 20
}
console.log(jsonPerson);
var strPerson = JSON.stringify(jsonPerson);//对jsonPerson没有影响
console.log(strPerson);
//格式必须满足JSON格式
var strDog = "{\"name\":\"John\",\"age\":30}";
var jsonDog = JSON.parse(strDog);
console.log(jsonDog)
</script>
注意与细节
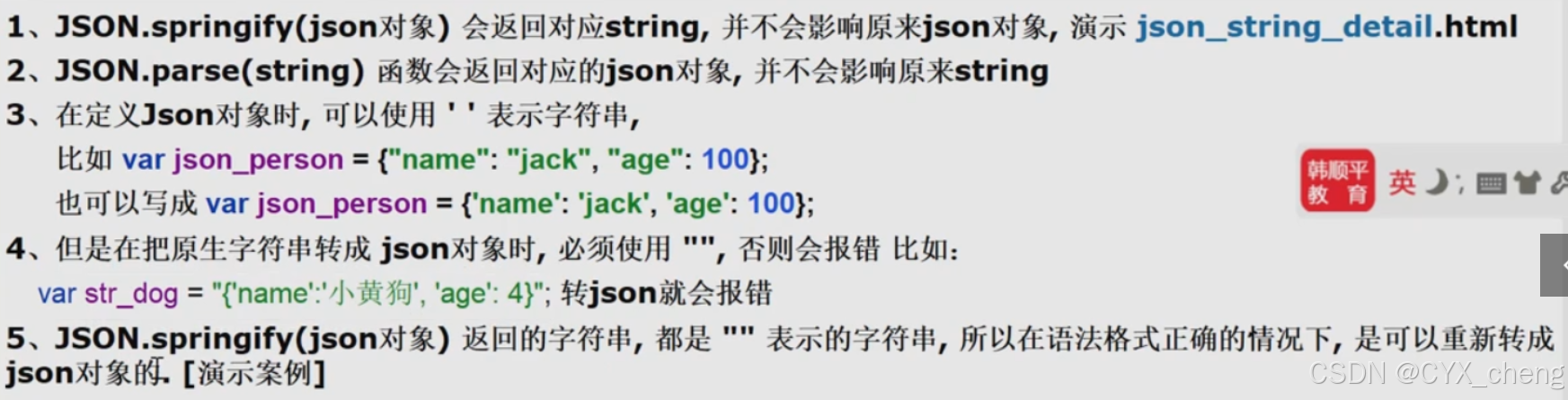
在定义JSON对象时,key可以不加引号,在浏览器查看的时候都会加上双引号,所以可以直接相互转换
在java中使用

应用场景
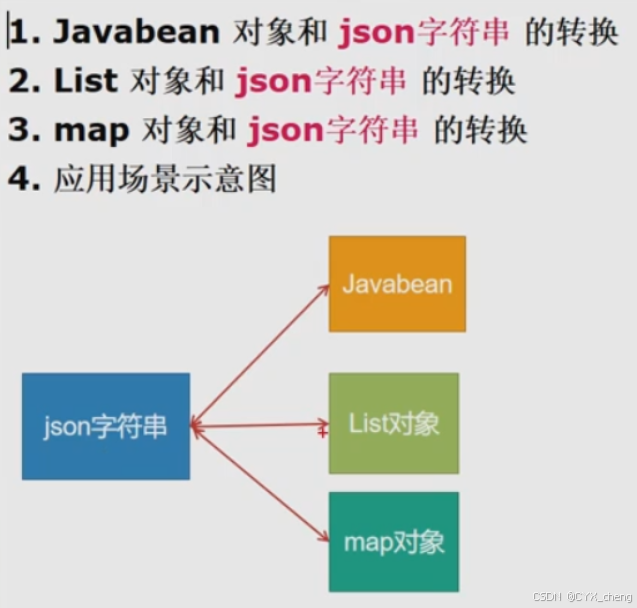
json字符串与javabean转换
java
public class JavaJson {
public static void main(String[] args) {
Gson gson = new Gson();
Book book = new Book(100, "学java");
//javabean->json字符串
String strBook = gson.toJson(book);
System.out.println(strBook);
//json字符串->javabean
//strBook就是json字符串,Book.class底层是反射
Book book1 = gson.fromJson(strBook, Book.class);
System.out.println(book1);
}
}
json字符串与list对象转换
java
public class JavaJson {
public static void main(String[] args) {
Gson gson = new Gson();
ArrayList<Book> list = new ArrayList<>();
list.add(new Book(100, "学java1"));
list.add(new Book(200, "学java2"));
//javabean->json字符串
String strBookList = gson.toJson(list);
System.out.println(strBookList);
//json字符串->集合,需要gson提供的一个类:TypeToken
//返回类型的完整路径,然后进行底层反射
//TypeToken<List<Book>>()会调用无参构造器(protected不同包不能使用)
//加了{}就是匿名内部类,是Type的子类,执行子类的无参构造器时,默认super,不受protected限制
Type type = new TypeToken<List<Book>>() {}.getType();
List<Book> bookList = gson.fromJson(strBookList, type);
System.out.println(bookList);
}
}
json字符串与map对象转换
java
public class JavaJson {
public static void main(String[] args) {
Gson gson = new Gson();
Map<String,Book> map = new HashMap<>();
map.put("k1",new Book(100, "学java1"));
map.put("k2",new Book(200, "学java2"));
//javabean->json字符串
String strBookList = gson.toJson(map);
System.out.println(strBookList);
//json字符串->集合,需要gson提供的一个类:TypeToken
Map<String,Book> bookMap = gson.fromJson(strBookList, new TypeToken<Map<String,Book>>(){}.getType());
System.out.println(bookMap);
}
}
AJAX异步请求
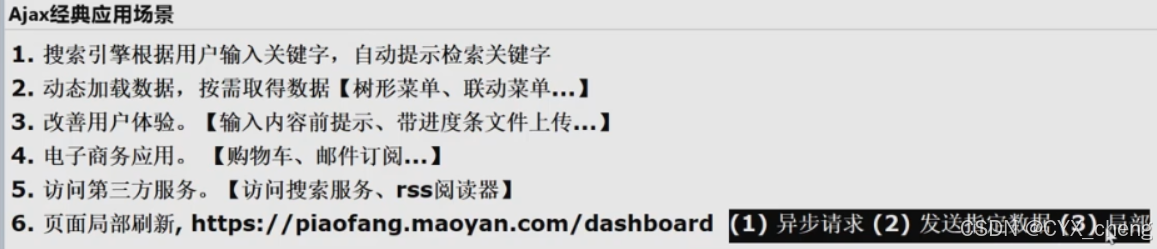
传统web数据通信方式
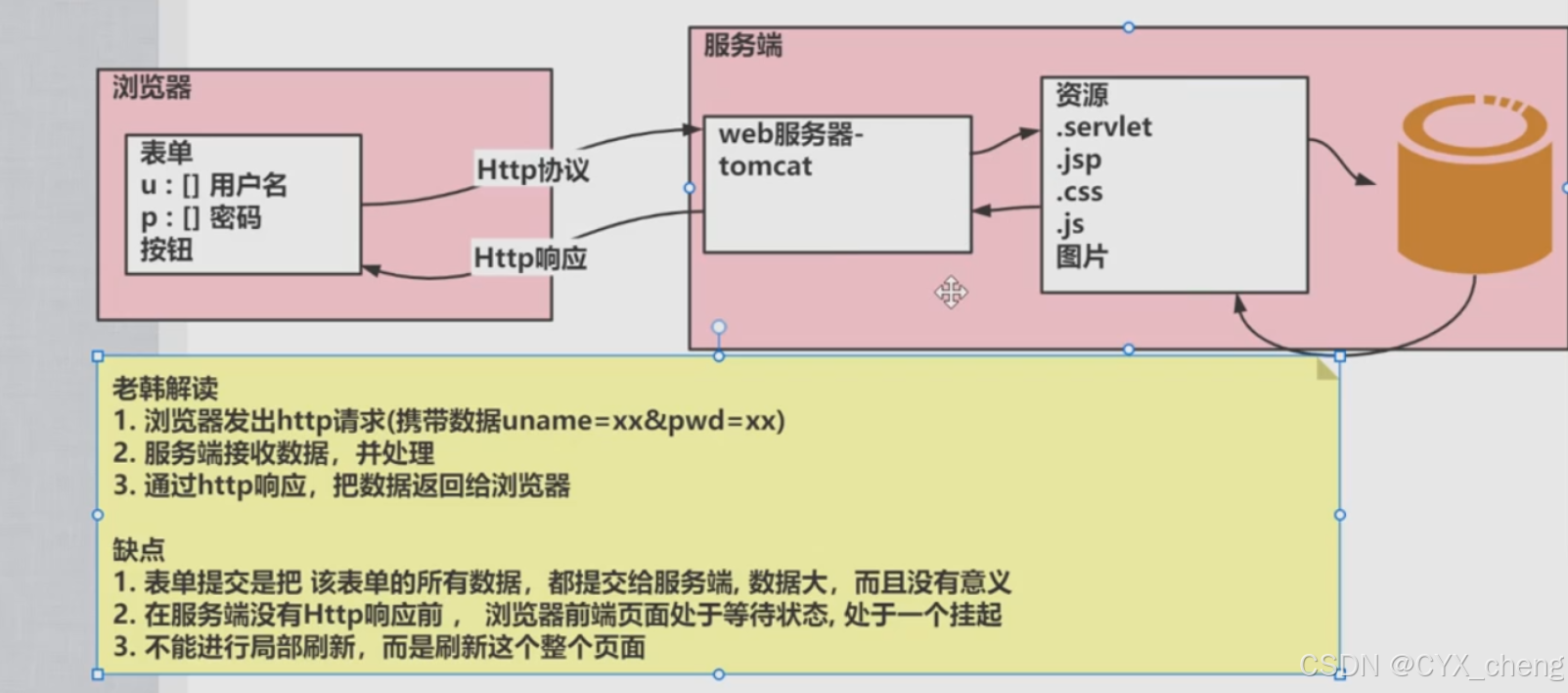
AJAX数据通信方式
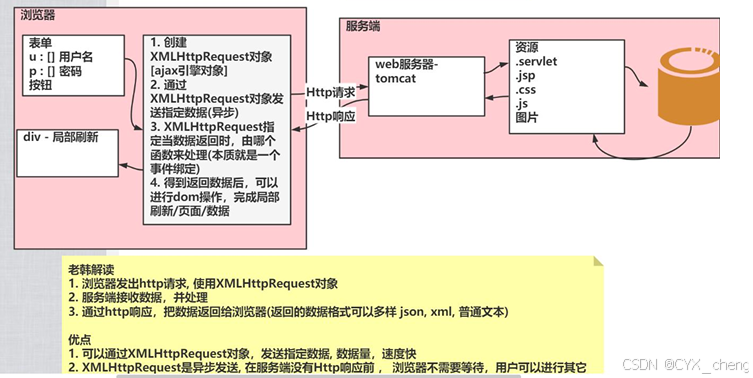
可以进行局部刷新
应用
验证用户名是否存在
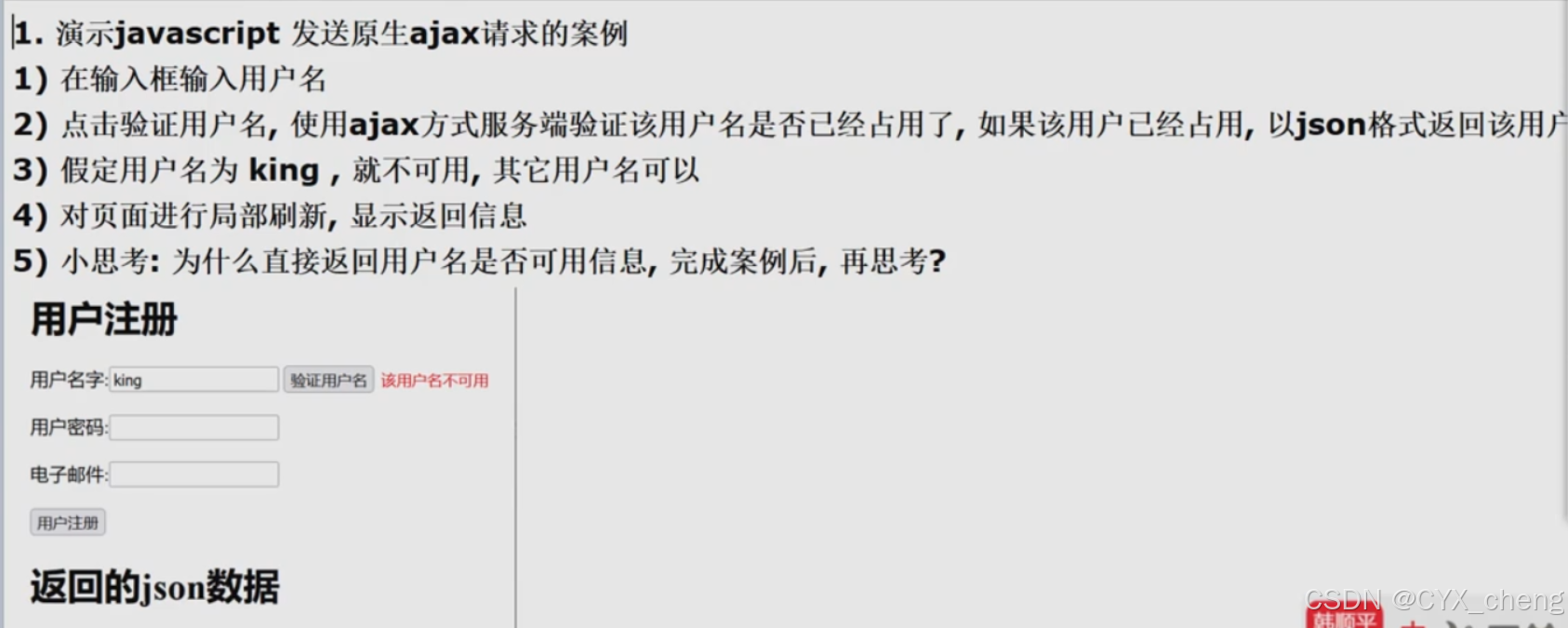
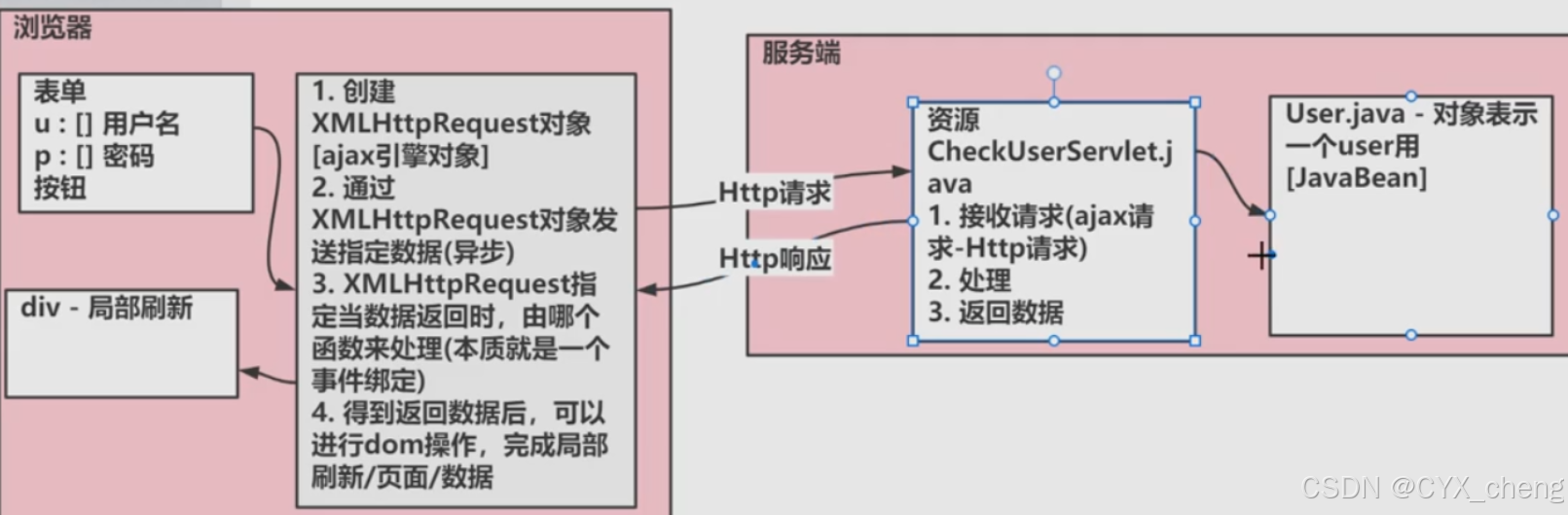
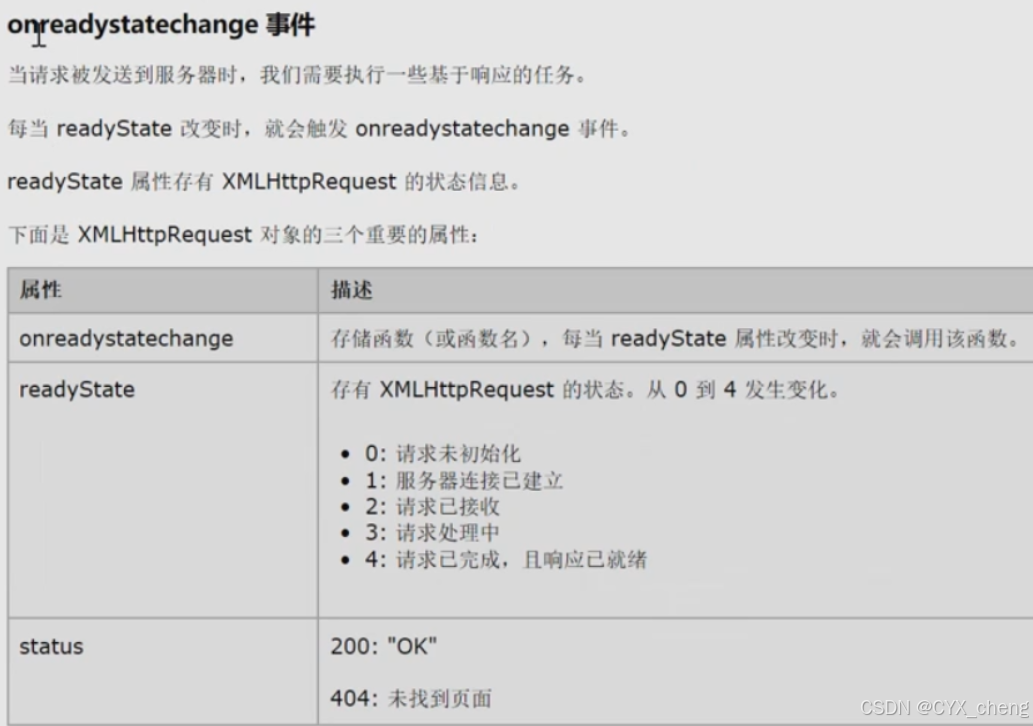
java
public class CheckUserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request,response);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("u");
System.out.println(username);
response.setContentType("text/html;charset=utf-8");
if("king".equals(username)) {
User king = new User(100, "king", "666", "king@sohu.com");
String strKing = new Gson().toJson(king);
response.getWriter().write(strKing);
} else {
response.getWriter().write("");
}
}
}
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>用户注册</title>
<script>
window.onload = function () {
var checkButton = document.getElementById("checkButton");
checkButton.onclick = function () {
var xhr = new XMLHttpRequest();
var uname = document.getElementById("uname").value;
xhr.open("GET","/ajax/check?u=" + uname, true);
//在send函数调用前,给XMLHttpRequest绑定一个事件,当数据变化,会触发该事件
xhr.onreadystatechange = function () {
// console.log(xhr);
if(xhr.readyState == 4 && xhr.status == 200) {
document.getElementById("div1").innerText = xhr.responseText;
var responseText = xhr.responseText;
if(responseText != "") {
document.getElementById("myres").value = "用户名不可用";
} else {
document.getElementById("myres").value = "用户名可用";
}
}
}
xhr.send();
}
}
</script>
</head>
<body>
<h1>用户注册~</h1>
<form action="/ajax/check" method="POST">
用户名字:<input type="text" name="username" id="uname">
<input type="button" id="checkButton" value="验证用户名">
<input style="border-width: 0;color: red" type="text" id="myres"><br/><br/>
用户密码:<input type="password" name="password"><br/><br/>
电子邮件:<input type="text" name="email"><br/><br/>
<input type="submit" value="用户注册">
</form>
<h1>返回的json数据</h1>
<div id="div1"></div>
</body>
</html>
JQuery操作AJAX
$.ajax方法
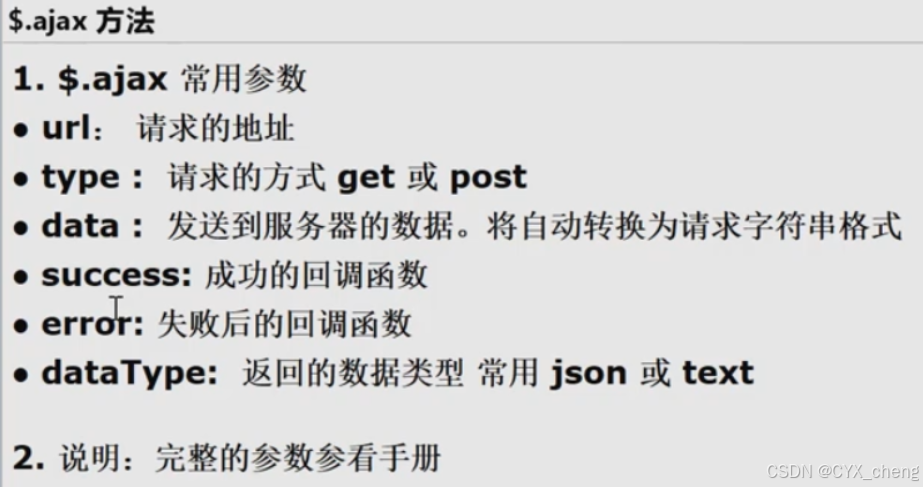
.get请求和.post请求
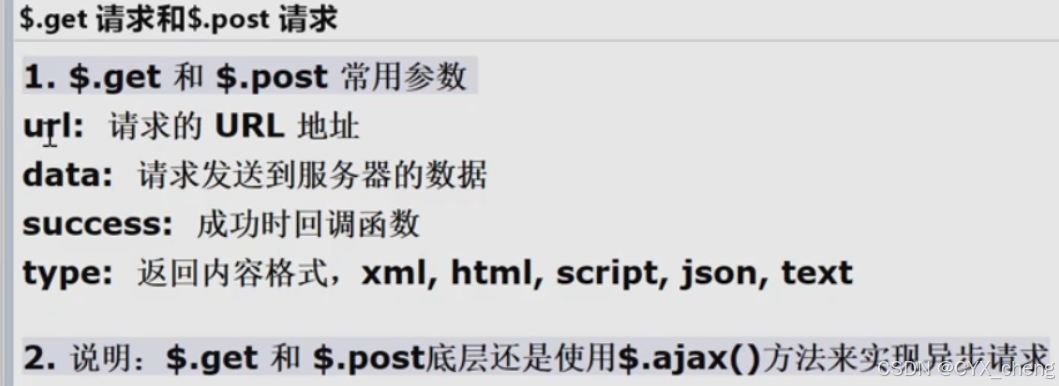
$.getJSON
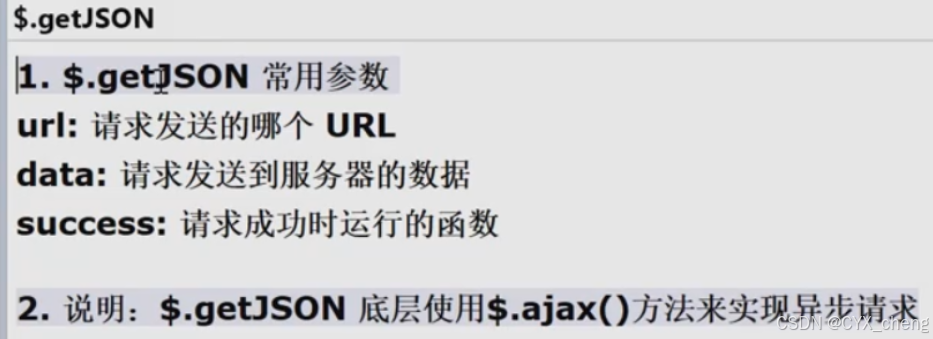
应用
java
public class CheckUserServlet extends HttpServlet {
private UserService userService = new UserService();
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doPost(request,response);
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//取决于JQuery中data定义的名字
String username = request.getParameter("username");
System.out.println(username);
response.setContentType("text/json;charset=utf-8");
if("king".equals(username)) {
User user = new User(100, "king", "abc", "king@sohu.com");
response.getWriter().write(new Gson().toJson(user));
} else {
User user = new User(1, "", "", "");
response.getWriter().write(new Gson().toJson(user));
}
}
}
3种情况
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>用户注册</title>
<script src="script/jquery-3.7.1.js"></script>
<script>
$(function () {
$("#checkButton").click(function () {
$.ajax({
url: "/ajax/check",
type: "POST",
data: {
username: $("#uname").val(),
//传日期,为了浏览器缓存,浏览器一直变化,会发送POST请求
date: new Date()
},
error: function () {//失败后的回调函数
console.log("失败")
},
success: function (data, status, xhr) {
console.log(data);
console.log(status);
console.log(xhr);
$("#div1").html(JSON.stringify(data));
if("" == data.username) {
$("#myres").val("该用户名可用");
} else {
$("#myres").val("该用户名不可用");
}
},
dataType: "json"
})
//讲解.get()使用,必须按顺序url,data,success回调函数,返回的数据格式
$.get(
"/ajax/check",
{
username: $("#uname").val(),
date: new Date()
},
function (data, status, xhr) {
//可以根据状态码处理失败的情况
console.log(data);
console.log(status);
console.log(xhr);
$("#div1").html(JSON.stringify(data));
if ("" == data.username) {
$("#myres").val("该用户名可用");
} else {
$("#myres").val("该用户名不可用");
}
},
"json"
)
//如果通过jquery发出的ajax请求是get,并且返回的数据格式是json,可以直接使用getJson()函数
$.getJSON(
"/ajax/check",
{
username: $("#uname").val(),
date: new Date()
},
function (data, status, xhr) {
//可以根据状态码处理失败的情况
console.log(data);
console.log(status);
console.log(xhr);
$("#div1").html(JSON.stringify(data));
if ("" == data.username) {
$("#myres").val("该用户名可用");
} else {
$("#myres").val("该用户名不可用");
}
}
)
})
})
</script>
</head>
<body>
<h1>用户注册~</h1>
<form action="/ajax/check" method="POST">
用户名字:<input type="text" name="username" id="uname">
<input type="button" id="checkButton" value="验证用户名">
<input style="border-width: 0;color: red" type="text" id="myres"><br/><br/>
用户密码:<input type="password" name="password"><br/><br/>
电子邮件:<input type="text" name="email"><br/><br/>
<input type="submit" value="用户注册">
</form>
<h1>返回的json数据</h1>
<div id="div1"></div>
</body>
</html>
ThreadLocal线程数据共享和安全
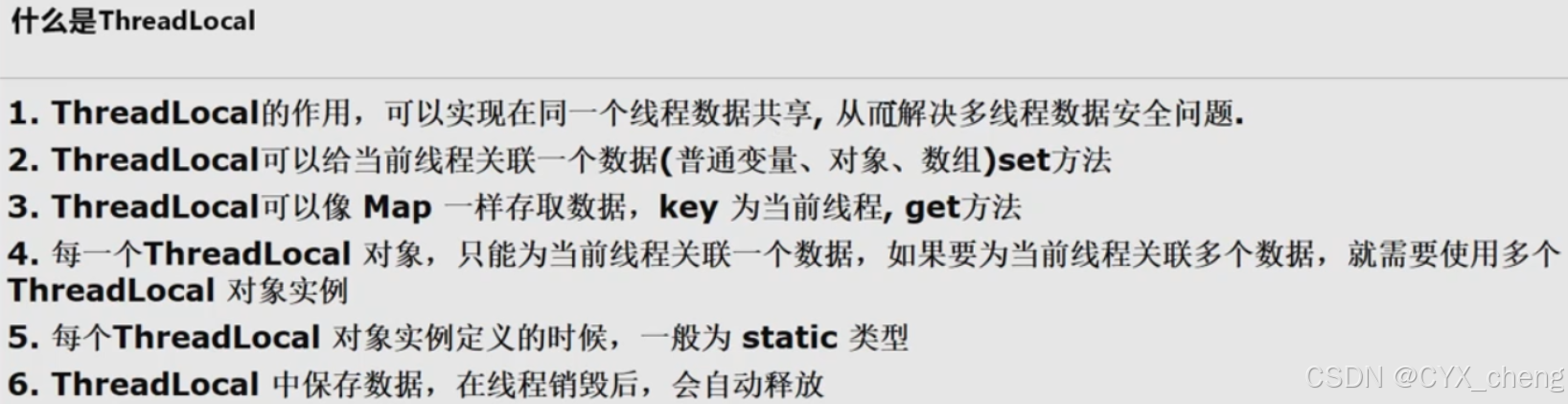
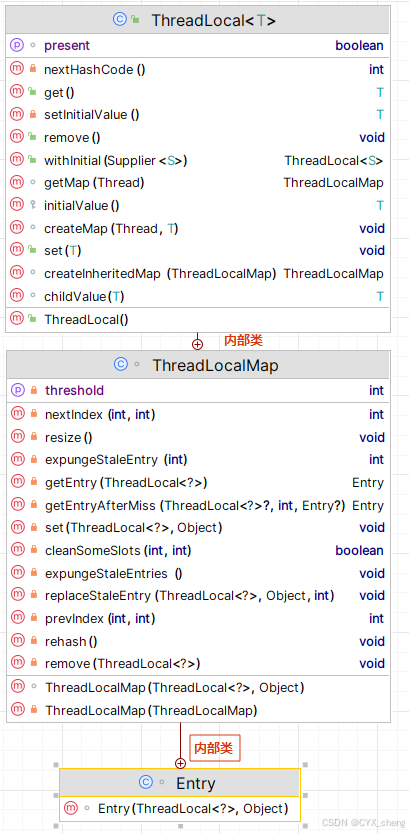
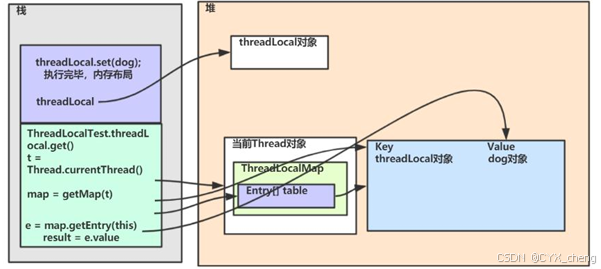
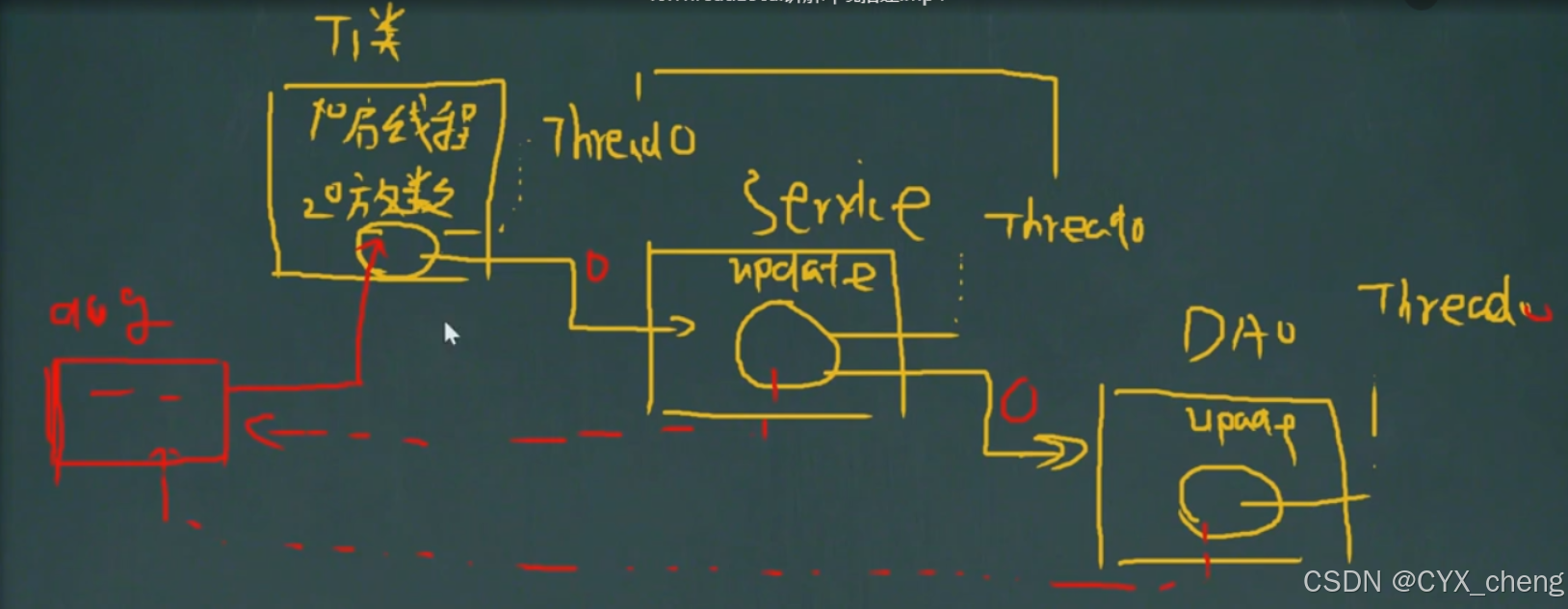
set分析
演示:在一个线程中,共享数据(线程安全)
java
public class T1 {
//创建ThreadLocal对象
public static ThreadLocal<Object> threadLocal1 = new ThreadLocal<>();
public static ThreadLocal<Object> threadLocal2 = new ThreadLocal<>();
public static class Task implements Runnable {
@Override
public void run() {
Dog dog = new Dog();
Pig pig = new Pig();
/*
public void set(T value) {
1、获取当前线程,关联到当前线程
Thread t = Thread.currentThread();
2、通过线程对象,获取ThreadLocalMap
是ThreadLocal的静态内部类
ThreadLocalMap map = getMap(t);
3、如果map不为空,就将数据(Dog...)放入map
key:threadLocal,value:存放的数据
一个threadLocal只能关联一个数据,set会替换掉
4、如果map为null,就创建一个和当前关联的ThreadLocal,并且将该数据放入
if (map != null) {
map.set(this, value);
} else {
createMap(t, value);
}
}
* */
threadLocal1.set(dog);
// threadLocal1.set(pig);替换
threadLocal2.set(pig);//跟threadLocal2关联,并且都被当前Thread管理
System.out.println("在run方法中的线程= " + Thread.currentThread().getName());
System.out.println("在run方法中的dog= " + dog);
new T1service().update();
}
}
public static void main(String[] args) {
new Thread(new Task()).start();
}
}
java
public class T1service {
public void update() {
/*
public T get() {
1、先得到当前的线程对象
Thread t = Thread.currentThread();
2、获取到对应的ThreadLocalMap
ThreadLocalMap map = getMap(t);
if (map != null) {
3、如果map不为空,根据当前的ThreadLocal对象,得到对应的Entry
ThreadLocalMap.Entry e = map.getEntry(this);
if (e != null) {
4、如果Entry不为空,返回当前ThreadLocal关联的数据value
@SuppressWarnings("unchecked")
T result = (T)e.value;
return result;
}
}
return setInitialValue();
}
* */
Object o = T1.threadLocal1.get();
String name = Thread.currentThread().getName();
System.out.println("在T1service的update()线程是= " + name);
System.out.println("在T1service的dog是= " + o);
new T2DAO().update();
}
}
java
public class T2DAO {
public void update() {
Object o = T1.threadLocal1.get();
String name = Thread.currentThread().getName();
System.out.println("在T2DAO的update()线程是= " + name);
System.out.println("在T2DAO的dog是= " + o);
}
}