在Python中,配置管理是软件开发中的一个重要环节,它允许开发者在不修改代码的情况下调整应用程序的行为。configparser
模块是Python标准库的一部分,用于处理配置文件。配置文件通常以简单的键值对格式存在,类似于Windows的.ini
文件。通过configparser
,开发者可以轻松读取、修改、创建和管理这些配置文件。
2. 配置文件的基本结构
在使用configparser
之前,我们先了解一下配置文件的结构。配置文件通常由多个部分(sections)组成,每个部分包含多个键值对(key-value pairs)。
一个典型的配置文件可能如下所示:
bash
[DEFAULT]
ServerAliveInterval = 45
Compression = yes
CompressionLevel = 9
ForwardX11 = yes
[bitbucket.org]
User = hg
[topsecret.server.com]
Port = 50022
ForwardX11 = no
在上面的例子中:
[DEFAULT]
是一个特殊的部分,用来定义默认值。[bitbucket.org]
和[topsecret.server.com]
是普通部分,每个部分都包含一些配置项。
3. configparser
模块的基本操作
3.1 读取配置文件
要读取配置文件,我们首先需要创建一个ConfigParser
对象,并使用read
方法加载配置文件。
python
import configparser
config = configparser.ConfigParser()
config.read('example.ini')
读取配置文件后,可以通过部分名称和键名称来访问具体的配置值。
python
# 访问默认部分中的配置项
server_alive_interval = config['DEFAULT']['ServerAliveInterval']
compression = config['DEFAULT']['Compression']
# 访问特定部分中的配置项
user = config['bitbucket.org']['User']
port = config['topsecret.server.com']['Port']
3.2 检查部分和键的存在性
在读取配置之前,最好检查部分和键是否存在,以避免出现异常。
python
if 'bitbucket.org' in config:
print("bitbucket.org section exists")
if config.has_option('topsecret.server.com', 'Port'):
print("Port option exists in topsecret.server.com section")
3.3 修改配置文件
configparser
还允许我们修改现有的配置文件。我们可以通过set
方法来更改某个键的值:
python
config.set('bitbucket.org', 'User', 'new_user')
此外,还可以使用add_section
方法来添加新的部分:
python
config.add_section('new_section')
config.set('new_section', 'new_key', 'new_value')
修改完成后,可以通过write
方法将更改保存到文件:
python
with open('example.ini', 'w') as configfile:
config.write(configfile)
3.4 删除部分或键
如果需要删除部分或键,可以使用remove_section
和remove_option
方法。
python
config.remove_section('new_section')
config.remove_option('bitbucket.org', 'User')
4. 高级用法
4.1 处理默认值
在configparser
中,DEFAULT
部分的值可以作为所有其他部分的默认值。例如,如果某个部分中没有明确设置ServerAliveInterval
,则会使用DEFAULT
部分中的值。
python
# 访问topsecret.server.com部分中的ServerAliveInterval
# 如果topsecret.server.com中没有明确设置,则会使用DEFAULT中的值
server_alive_interval = config['topsecret.server.com'].get('ServerAliveInterval')
4.2 数据类型转换
从配置文件中读取的值默认都是字符串类型,如果需要其他数据类型,如整数、布尔值等,可以使用相应的转换方法。
python
# 转换为整数
port = config.getint('topsecret.server.com', 'Port')
# 转换为布尔值
forward_x11 = config.getboolean('DEFAULT', 'ForwardX11')
# 转换为浮点数
compression_level = config.getfloat('DEFAULT', 'CompressionLevel')
4.3 处理多种配置文件格式
configparser
可以解析多种格式的配置文件,包括简单的键值对、带有层级结构的键值对等。通过设置delimiters
参数,可以自定义键和值之间的分隔符。
python
config = configparser.ConfigParser(delimiters=('=', ':'))
config.read('example.ini')
此外,还可以通过设置comment_prefixes
参数自定义注释符号,默认情况下,#
和;
都是有效的注释符号。
python
config = configparser.ConfigParser(comment_prefixes=(';', '#'))
config.read('example.ini')
4.4 处理多行值
configparser
允许在配置文件中定义多行值,这些值可以通过缩进或使用反斜杠(\)
来表示。
bash
[multiline]
long_value = This is a long value that \
spans multiple lines
在读取时,configparser
会自动将这些多行内容合并为一个字符串。
python
long_value = config.get('multiline', 'long_value')
print(long_value)
# 输出: This is a long value that spans multiple lines
4.5 使用字典接口
ConfigParser
类也可以像字典一样使用,使得访问部分和键更加直观。此外,configparser
还支持与Python的dict
进行交互。
python
config_dict = dict(config['bitbucket.org'])
# 输出所有键值对
for key, value in config_dict.items():
print(f'{key} = {value}')
5. 实际应用示例
在实际开发中,configparser
广泛用于各种场景,如配置数据库连接、设置日志选项、定义应用程序的行为等。下面我们来看几个实际应用的示例。
5.1 数据库配置
假设我们有一个应用程序需要连接到多个数据库,可以使用configparser
来管理这些配置。
bash
[mysql]
host = localhost
user = root
password = secret
database = myapp
[postgresql]
host = localhost
user = postgres
password = secret
database = myapp
在程序中,可以使用以下代码来读取这些配置:
python
config = configparser.ConfigParser()
config.read('database.ini')
mysql_config = {
'host': config['mysql']['host'],
'user': config['mysql']['user'],
'password': config['mysql']['password'],
'database': config['mysql']['database'],
}
postgres_config = {
'host': config['postgresql']['host'],
'user': config['postgresql']['user'],
'password': config['postgresql']['password'],
'database': config['postgresql']['database'],
}
# 用于连接数据库的代码
5.2 日志配置
在复杂的应用程序中,日志是非常重要的部分。我们可以使用configparser
来配置日志选项。
bash
[loggers]
keys=root,simpleExample
[handlers]
keys=consoleHandler
[formatters]
keys=simpleFormatter
[logger_root]
level=DEBUG
handlers=consoleHandler
[logger_simpleExample]
level=DEBUG
handlers=consoleHandler
qualname=simpleExample
propagate=0
[handler_consoleHandler]
class=StreamHandler
level=DEBUG
formatter=simpleFormatter
args=(sys.stdout,)
[formatter_simpleFormatter]
format=%(asctime)s - %(name)s - %(levelname)s - %(message)s
在程序中,我们可以读取这些配置并设置日志记录器:
python
import logging
import logging.config
import configparser
config = configparser.ConfigParser()
config.read('logging.ini')
logging.config.fileConfig(config)
logger = logging.getLogger('simpleExample')
logger.debug('This is a debug message')
5.3 环境配置
configparser
也可以用于管理不同环境下的配置,例如开发、测试和生产环境的不同配置。
bash
[development]
debug = true
database = dev_db
[testing]
debug = false
database = test_db
[production]
debug = false
database = prod_db
根据当前环境,可以选择加载不同的部分:
python
import configparser
import os
config = configparser.ConfigParser()
config.read('environment.ini')
env = os.getenv('ENV', 'development')
env_config = config[env]
debug_mode = env_config.getboolean('debug')
database_name = env_config['database']
6. 常见问题及最佳实践
6.1 文件不存在时的处理
当配置文件不存在时,configparser
会引发FileNotFoundError
。可以通过捕获异常进行处理,或者提前检查文件是否存在。
python
import os
config_file = 'example.ini'
if not os.path.exists(config_file):
print(f"Configuration file {config_file} does not exist.")
else:
config.read(config_file)
6.2 配置文件的备份和恢复
在修改配置文件之前,建议先进行备份,以便在需要时恢复。
python
import shutil
shutil.copy('example.ini', 'example_backup.ini')
# 修改配置文件的代码
configparser
模块为Python开发者提供了一个简单且强大的工具,用于管理配置文件。它支持多种格式的配置文件,允许设置默认值、处理多行值,并且与字典有很好的兼容性。在实际应用中,configparser
可以帮助开发者更好地组织和管理应用程序的配置,确保代码的可维护性和灵活性。
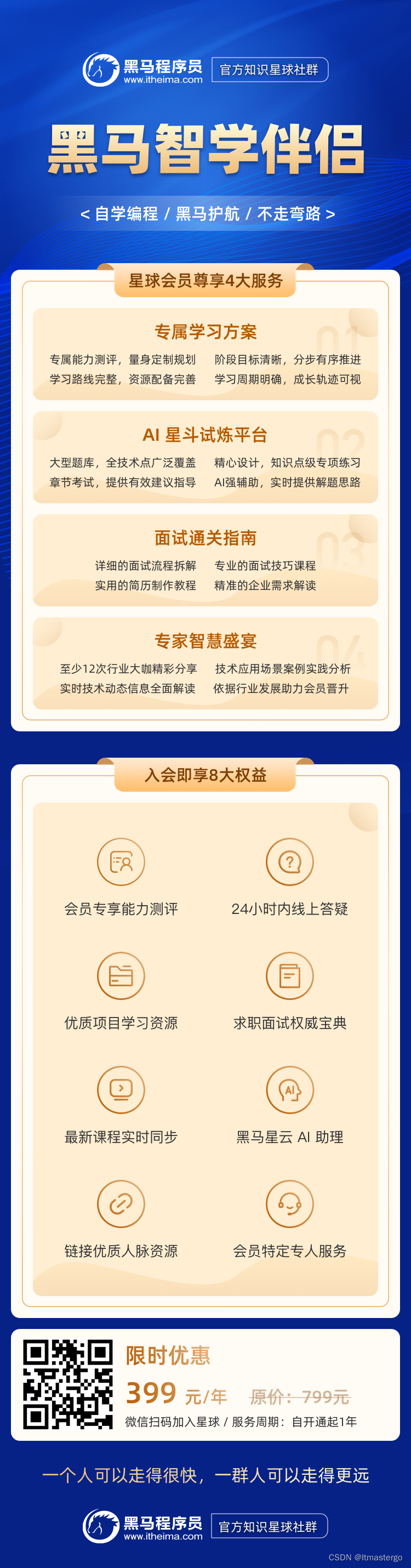