JavaEE16
一、文件系统操作
在java标准库中,实现了File类,其中提供了文件操作的方法!
1、构造方法:
|------------------------------------|-------------------------------------|
| 方法名 | 说明 |
| File(File parent , String child) | 根据父目录+孩子文件路径,创建一个新的File 实例 |
| File( String pathname) | 根据文件路径创建一个新的File的实例,路径可以是绝对路径或者相对路径 |
| File( String parent, String child) | 根据父目录+孩子文件路径,创建一个新的File实例,父目录用路径表示 |
2、与文件路径有关的方法:
|--------|---------------------|--------------------|
| 返回类型 | 方法名 | 说明 |
| String | getParent( ) | 返回File对象的父目录文件路径 |
| String | getName( ) | 返回File对象的纯文件名称 |
| String | getPath( ) | 返回File对象的文件路径 |
| String | getAbsolutePath( ) | 返回File对象的绝对路径 |
| String | getCanonicalPath( ) | 返回File对象的修饰过程的绝对路径 |
代码案例一:绝对路径创建法
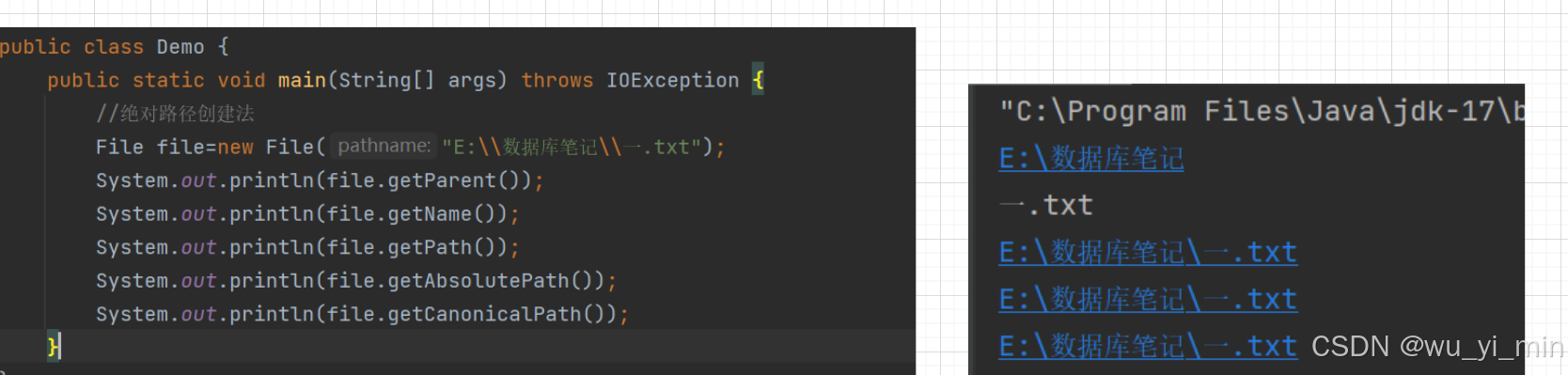
代码案例二:相对路径创建法(基准目录是当前IDEA所打开文件的所在目录)
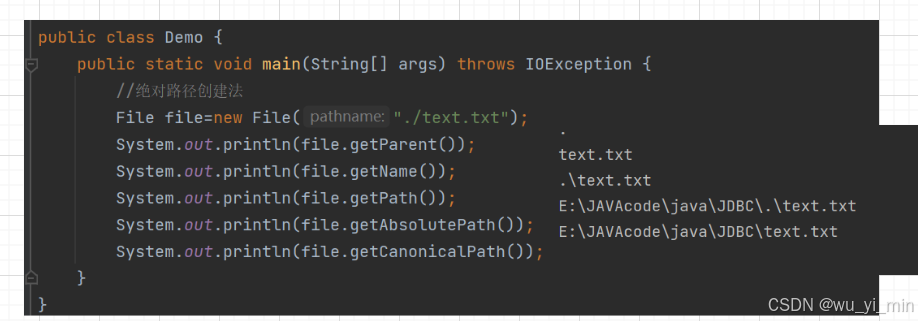
3、文件判断方法
|---------|------------------|-------------------------------|
| 返回值 | 方法名 | 说明 |
| bollean | exists( ) | 判断File对象所描述的文件是否真实存在 |
| boolean | isDirectory( ) | 判断File对象代表的文件是否是一个目录 |
| boolean | isFile() | 判断File对象所代表的文件是否是一个普通文件 |
| boolean | createNewFile( ) | 根据File对象,自动创建一个空文件,创建成功返回true |
| boolean | delete( ) | 删除File对象所描述的文件,删除成功返回true |
代码案例一:文件不存在的情况
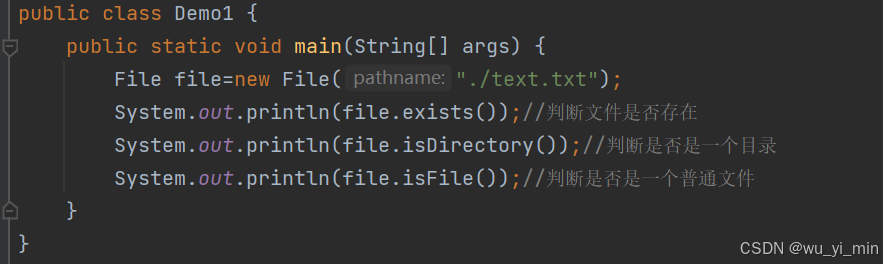

代码案例二: 文件存在的情况
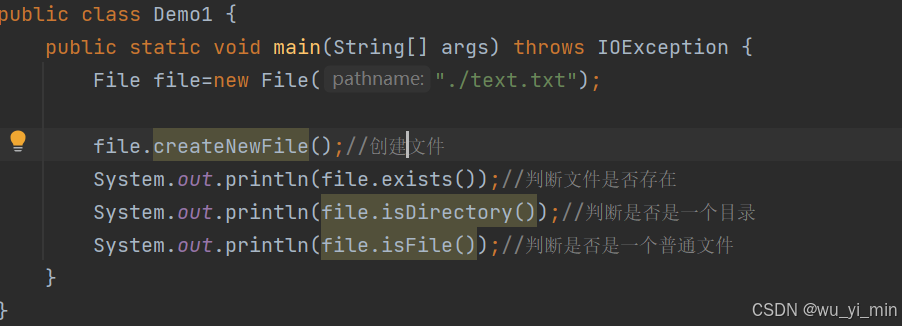

同时,在该文件目录下,也会创建对应的txt文件
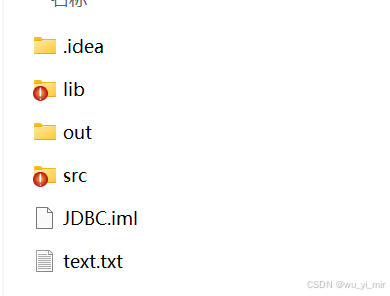
删除文件
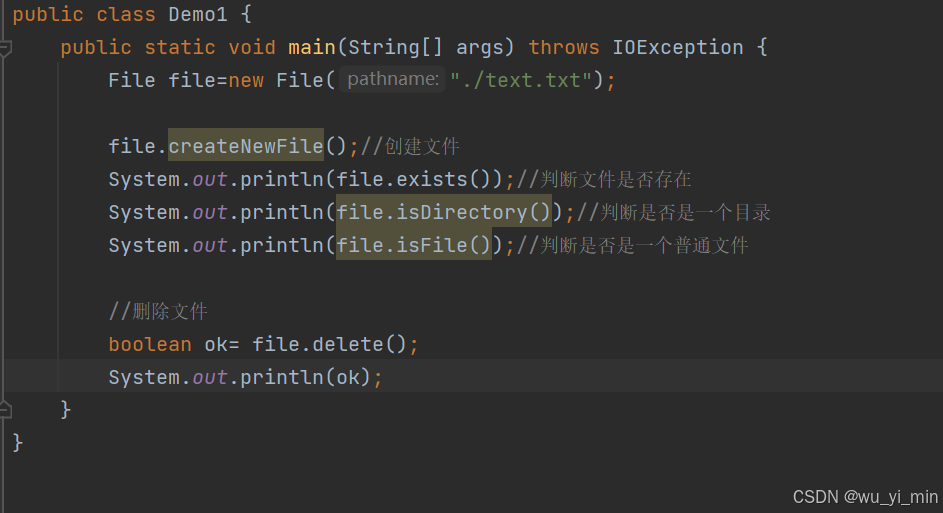
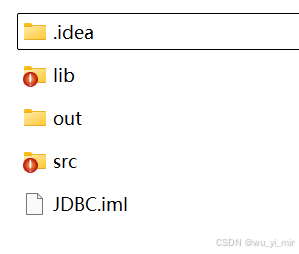
其实,还有另外一种删除操作,deleteOnExit(),当进程结束时,才真的执行删除操作!应用场景是可以创建一些临时文件!
4、与文件目录有关的方法
|-------------|--------------|-------------------------------|
| 返回值 | 方法名 | 说明 |
| String[ ] | list( ) | 返回File对象代表的目录下的所有文件名 |
| File[ ] | listFiles( ) | 返回File对象代表的目录下的所有文件,以File对象表示 |
代码案例1:(打印目录中第一层的文件路径)
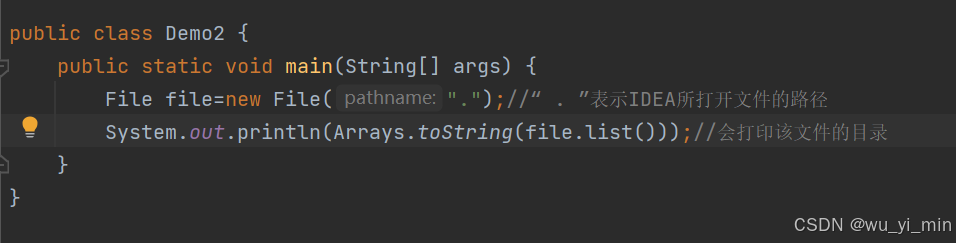

代码案例2:递归打印目录下所有文件路径
//递归打印所有文件路径
public class Demo5 {
public static void scan(File current){
//判断current是否为目录
if(!current.isDirectory()){
return;
}
//此时表示是current是目录
File[] files=current.listFiles();
if(files==null||files.length==0){
return;
}
//此时表示该目录存在
for(File f:files){
if(f.isFile()){
System.out.println(f.getAbsolutePath());
}else{
scan(f);
}
}
}
public static void main(String[] args) {
File f=new File(".");
scan(f);
}
}
5、创建目录
代码案例一:创建普通目录
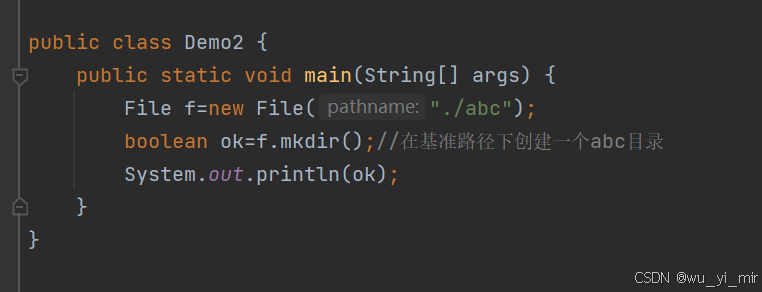
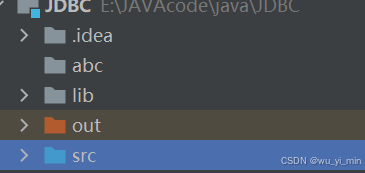
2、代码案例二:创建多层目录
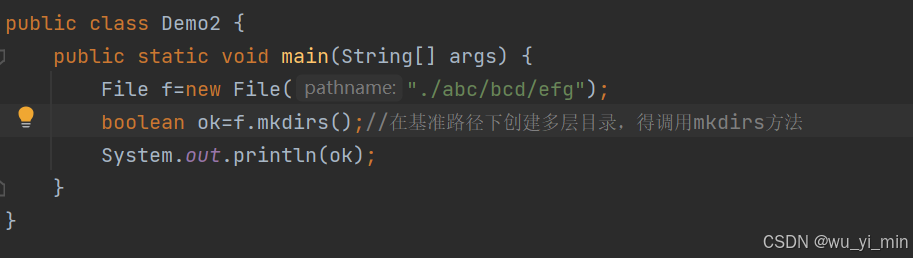
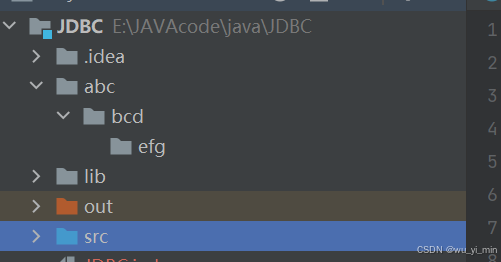
6、重命名文件(移动文件)
代码案例一:修改文件名
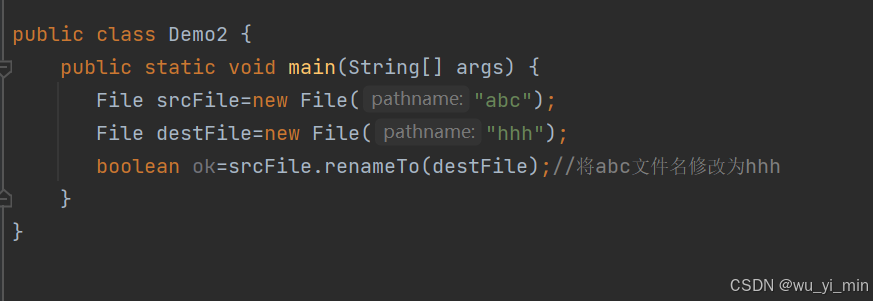
代码案例二:修改文件级别
任务:将efg文件的级别设置为和hhh文件同级
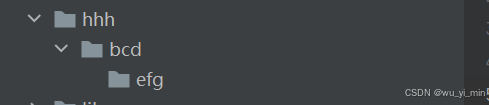
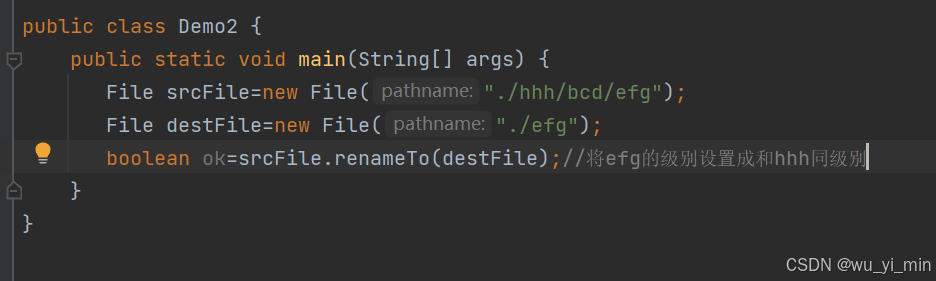
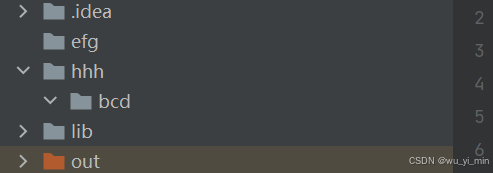
二、文件内容操作
1、IO流
文件操作内容包括:读文件 和 写文件,都是操作系统提供的api,java也进行了封装!要学习文件内容的操作,离不开对"文件流"的了解!
文件流就像水流一样,不过它流动的是数据,它也叫IO流。
IO流主要分为两个大类:
1)字节流(二进制): 读写数据的基本单位,就是字节。(InputStream、OutputStream)
2)字符流(文本):读写数据的基本单位,就是字符.(Reader、Writer)
2、文件的打开和关闭
在对文件内容进行操作的时候,首先需要打开文件。打开文件,其实是在该进程的文件描述符表 中创建了一个新的表项!
文件描述符表,可以理解为一个数组,数组的每个元素就是一个struct file对象,每个结构体就描述了对应操作的文件信息。数组的下标,就称为"文件描述符"。
每次打开一个文件,就相当于在数组上占用了一个位置,而在系统内核中,文件描述符表数组,是固定长度,不可扩容的!除非主动close文件,才能释放空间!如果代码里一直打开文件,不去关闭,就会使这里的空间越来越小,等数组搞满了,后续打开文件就会失败!
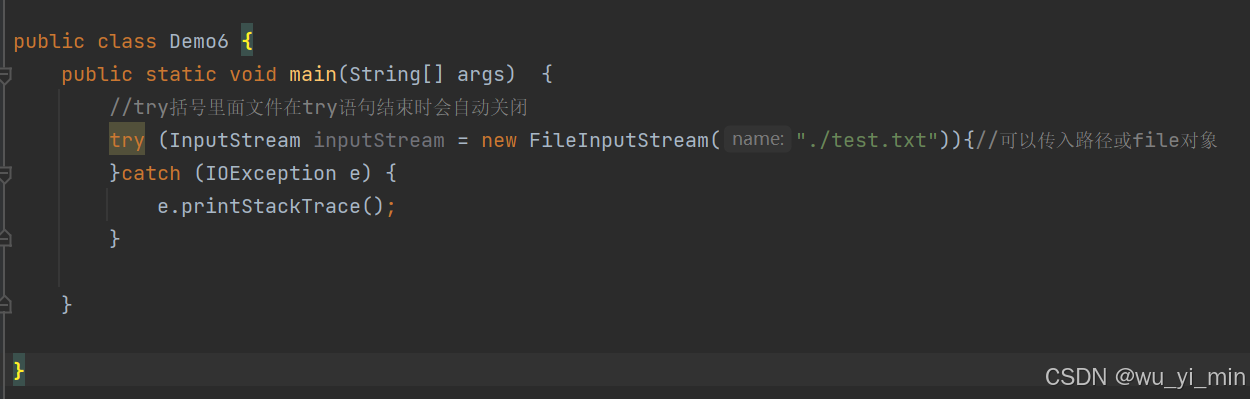
JavaEE17
3、读文件
方法一:
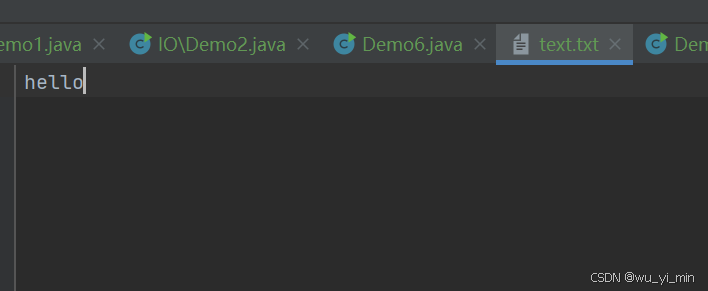
public static void main(String[] args) throws IOException {
//try括号里面文件在try语句结束时会自动关闭
try (InputStream inputStream = new FileInputStream("./text.txt")){//可以传入路径或file对象
while(true){
//当read返回值为-1,表示文件读取结束
int b=inputStream.read();
if(b==-1){
break;
}
System.out.printf("0x%x\n",b);
}
}catch (IOException e) {
e.printStackTrace();
}
}
缺点:频繁进行IO操作,开销大!
方法二:
public class Demo6 {
public static void main(String[] args) throws IOException {
//try括号里面文件在try语句结束时会自动关闭
try (InputStream inputStream = new FileInputStream("./text.txt")){//可以传入路径或file对象
//一次IO操作,该操作把在硬盘上读取的数据,填充到buffer内存中
byte[] buffer=new byte[1024];
int n= inputStream.read(buffer);//返回成读取的字节数
System.out.println(n);
}catch (IOException e) {
e.printStackTrace();
}
}
}
4、写文件
代码案例一:
注意:一旦使用outputStream打开文件,会清空原文件的内容!
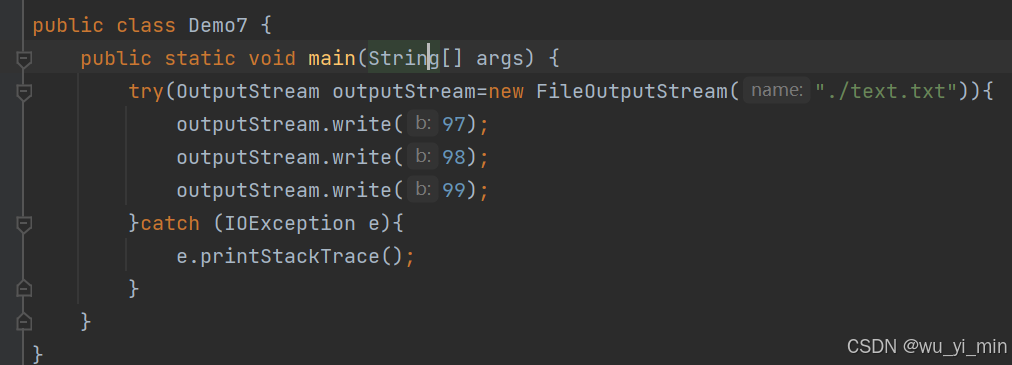
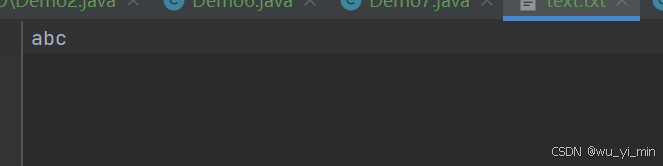
代码案例二:追加模式
如果不想清空原文件的内容,可以开启outputStream的追加的模式
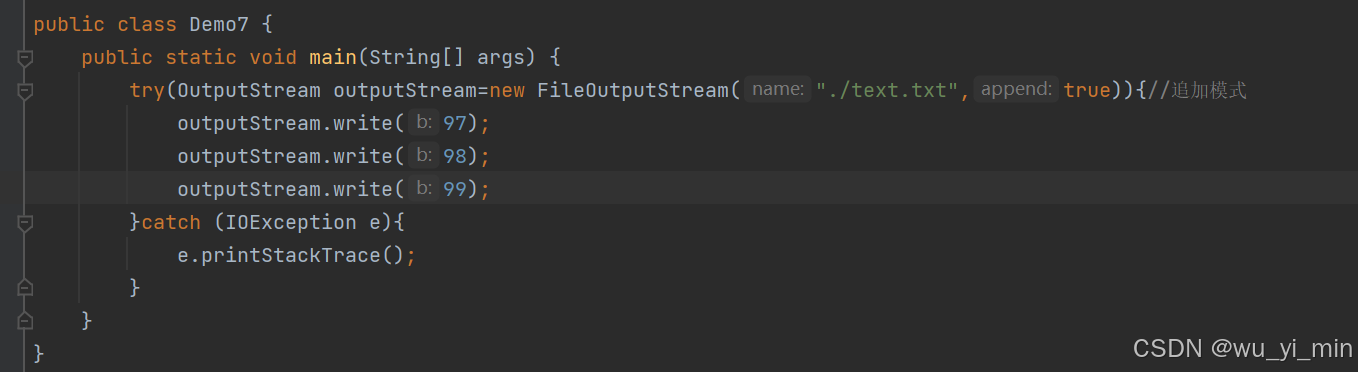
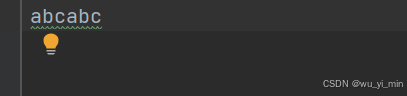
代码案例三:一次写入多个字节
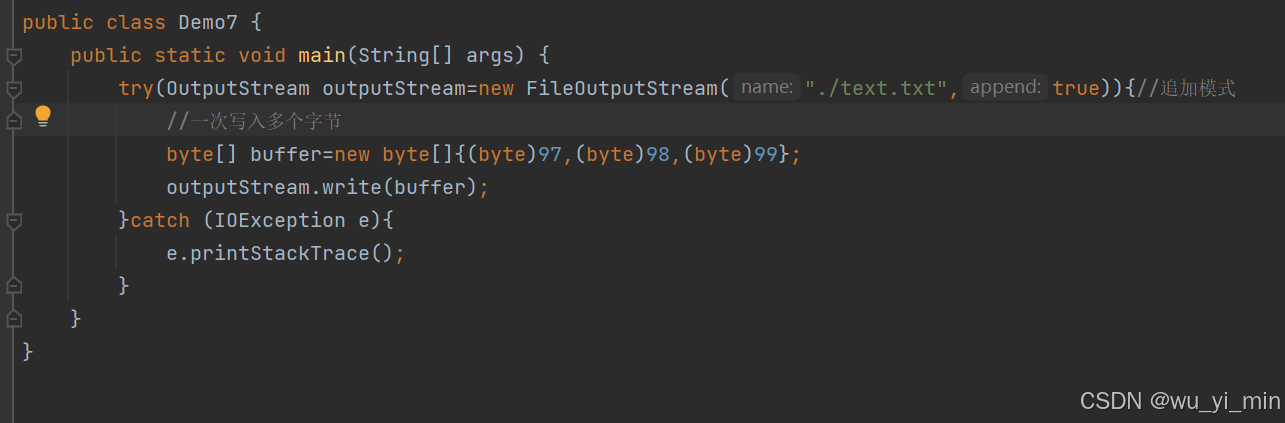
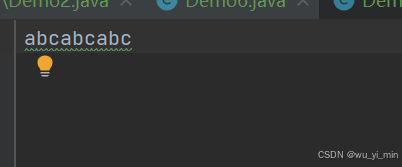
5、字符流读文件
在utf8编码,每个汉字是3个字节,当使用字符流读取数据的时候,就会自动切换编码方式为unicode编码,此时每个汉字是两个字节!
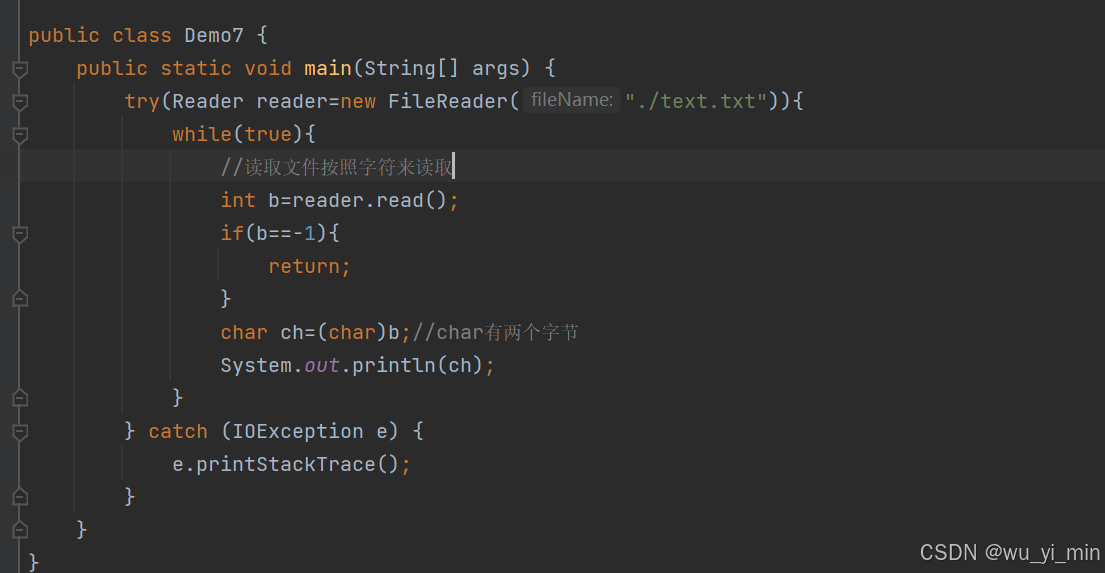
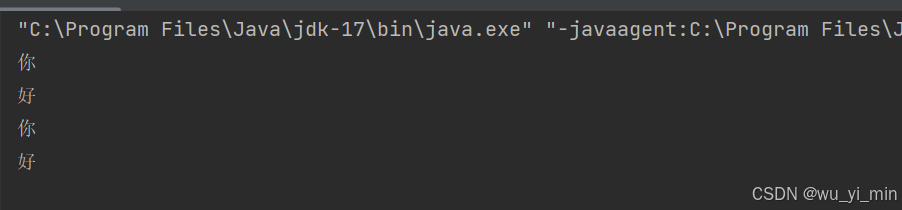
6、字符流写文件
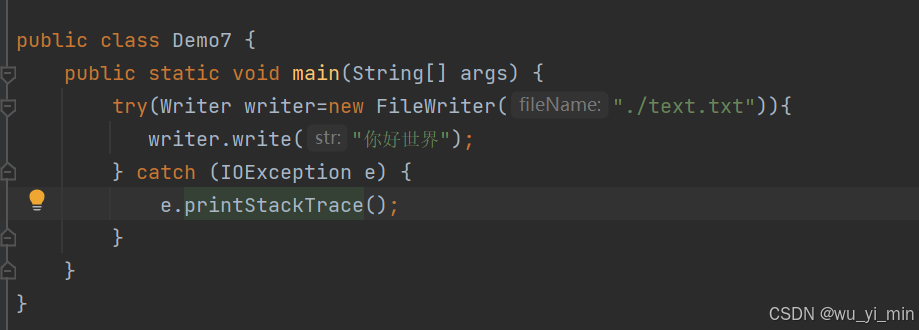
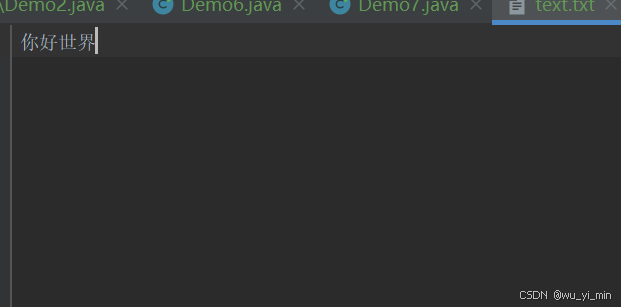
一、文件操作实践
案例一:删除文件名包含关键字的文件
public class Demo8 {
private static void doSomething(File f,String key) {
//如果包含关键字,让用户决定要不要删除
if (f.getName().contains(key)) {
System.out.println("是否要删除文件"+f.getName()+"删除Y,不删除N");
Scanner scanner = new Scanner(System.in);
String choice = scanner.next();
if(choice.equals("Y")||choice.equals("y")){
f.delete();
System.out.println("删除文件成功");
}else if(choice.equals("N")||choice.equals("n")){
System.out.println("不删除该文件");
}else{
System.out.println("输入有误,重新输入");
doSomething(f,key);
}
}
}
private static void scan(File file,String key){
//如果不是目录,结束该方法
if(!file.isDirectory()){
return;
}
//如果目录下没有文件,结束该方法
File[] list=file.listFiles();
if(list==null||list.length==0){
return;
}
for(File f:list){
if(f.isFile()){
doSomething(f,key);
}else{
scan(f,key);
}
}
}
//删除文件名含有关键字的文件
public static void main(String[] args) {
//1、输入文件路径
System.out.println("请输入文件路径");
Scanner scanner=new Scanner(System.in);
String rootPath=scanner.next();
//2、打开文件并开始递归遍历文件
File file=new File(rootPath);
//输入关键字
System.out.println("请输入关键字");
String key=scanner.next();
scan(file,key);
}
}
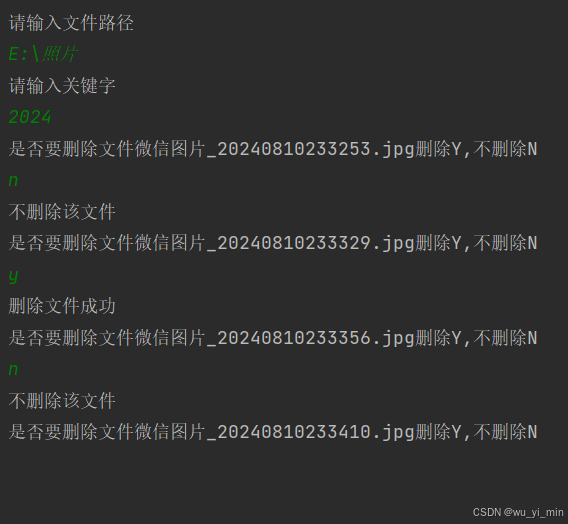
案例二:复制文件
**注意:**复制的时候,如果目录中文件不存在,会自动创建文件,但是如果目录不存在,那么无法自动创建目录!
public class Demo8 {
//复制文件
public static void main(String[] args) {
Scanner scanner=new Scanner(System.in);
System.out.println("请输入源文件的文件路径");
String src=scanner.next();
File srcFile=new File(src);
if(!srcFile.isFile()){
System.out.println("源文件路径有误!");
return;
}
System.out.println("请输入目标文件的路径");
String dest=scanner.next();
File destFile=new File(dest);
if(!destFile.getParentFile().isDirectory()){
System.out.println("目标父目录有误!");
return;
}
try(InputStream inputStream=new FileInputStream(srcFile);
OutputStream outputStream=new FileOutputStream(destFile)) {
while (true) {
byte[] buffer = new byte[1024];
int n =inputStream.read(buffer);//读完放buffer里面
if(n==-1){
break;
}
outputStream.write(buffer,0,n);
}
}catch (IOException e){
e.printStackTrace();
}
}
}
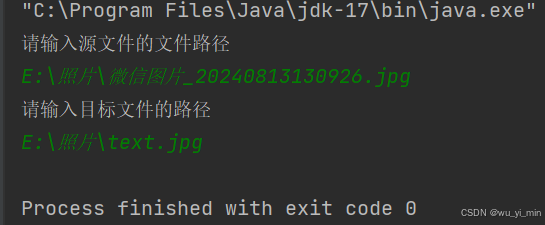
案例三:按照内容查找文件
public class Demo9 {
private static void doSearch(File file,String key) {
StringBuilder stringBuilder=new StringBuilder();
try(Reader reader=new FileReader(file)){
while(true) {
char[] buffer = new char[1024];
int n=reader.read(buffer);
if(n==-1){
break;
}
String s=new String(buffer,0,n);
stringBuilder.append(s);
}
if(stringBuilder.indexOf(key)==-1){
System.out.println("该文件不是目标文件");
}else{
System.out.println("找到目标文件"+file.getAbsolutePath());
}
}catch (IOException e){
e.printStackTrace();
}
}
private static void scan(File file,String key){
if(!file.isDirectory()){
return;
}
File[] list=file.listFiles();
if(list==null||list.length==0){
return;
}
for(File f:list){
if(f.isFile()){
doSearch(f,key);
}else{
scan(f,key);
}
}
}
//查找某个目录底下所有内容包含关键字的文件
public static void main(String[] args) {
//1、输入文件路径
System.out.println("请输入文件路径");
Scanner scanner=new Scanner(System.in);
String rootPath=scanner.next();
//2、创建文件对象并且判断是否为文件目录
File file=new File(rootPath);
if(!file.isDirectory()){
//该路径不是目录
System.out.println("文件路径有误!");
return;
}
//3、该文件是路径,开始查找
System.out.println("输入关键字");
String key=scanner.next();
scan(file,key);
}
}
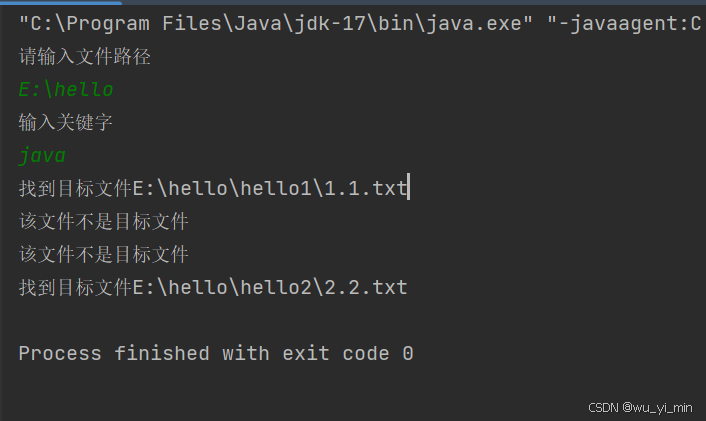