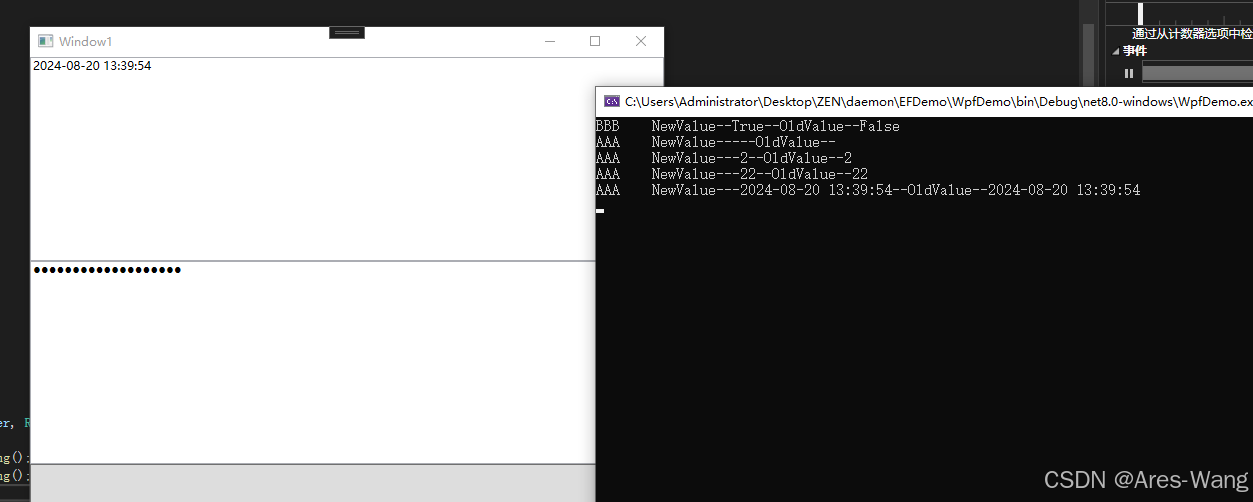
csharp
using System;
using System.Collections.Generic;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WpfDemo
{
public class ZenViewModel:INotifyPropertyChanged
{
private string userName;
public string UserName
{
get { return userName; }
set {
userName = value;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs("UserName"));
}
}
private string password;
public string Password
{
get { return password; }
set {
password = value;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs("Password"));
}
}
public event PropertyChangedEventHandler? PropertyChanged;
}
}
csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
namespace WpfDemo
{
public class ZenPasswordBoxHelper
{
public static string GetZenPassword(DependencyObject obj)
{
return (string)obj.GetValue(ZenPasswordProperty);
}
public static void SetZenPassword(DependencyObject obj, string value)
{
obj.SetValue(ZenPasswordProperty, value);
}
// Using a DependencyProperty as the backing store for ZenPassword. This enables animation, styling, binding, etc...
public static readonly DependencyProperty ZenPasswordProperty =
DependencyProperty.RegisterAttached("ZenPassword", typeof(string), typeof(ZenPasswordBoxHelper), new PropertyMetadata("",OnZenPropertyChangedCallback));
private static void OnZenPropertyChangedCallback(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
if (d is PasswordBox)
{
PasswordBox pwdBox = (PasswordBox)d;
pwdBox.Password = (string)e.NewValue;
Console.WriteLine("AAA NewValue---" + (string)e.NewValue+ "--OldValue--" + (string)e.NewValue);
}
else
return;
}
public static bool GetIsEnableBind(DependencyObject obj)
{
return (bool)obj.GetValue(IsEnableBindProperty);
}
public static void SetIsEnableBind(DependencyObject obj, bool value)
{
obj.SetValue(IsEnableBindProperty, value);
}
// Using a DependencyProperty as the backing store for IsEnableBind. This enables animation, styling, binding, etc...
public static readonly DependencyProperty IsEnableBindProperty =
DependencyProperty.RegisterAttached("IsEnableBind", typeof(bool), typeof(ZenPasswordBoxHelper), new PropertyMetadata(false, OnPropertyChangedCallback));
private static void OnPropertyChangedCallback(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
if (d is PasswordBox)
{
PasswordBox pwdBox = (PasswordBox)d;
if ((bool)e.NewValue)
{
pwdBox.PasswordChanged += PwdBox_PasswordChanged;
}
else
{
pwdBox.PasswordChanged -= PwdBox_PasswordChanged;
}
Console.WriteLine("BBB NewValue--"+e.NewValue.ToString()+ "--OldValue--" + e.OldValue.ToString());
}
}
private static void PwdBox_PasswordChanged(object sender, RoutedEventArgs e)
{
PasswordBox pwdBox = (PasswordBox)sender;
SetZenPassword(pwdBox, pwdBox.Password);
}
}
}
csharp
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Windows.Threading;
namespace WpfDemo
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
ZenViewModel vm;
public MainWindow()
{
InitializeComponent();
vm = new ZenViewModel();
this.DataContext = vm;
}
private void Button_Click(object sender, RoutedEventArgs e)
{
vm.Password = DateTime.Now.ToString();
vm.UserName = DateTime.Now.ToString();
}
}
}
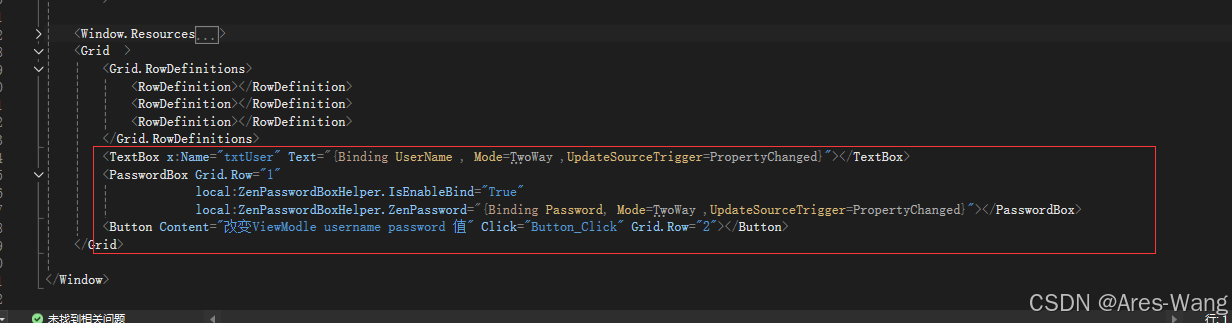