一、概述
1、定义:组合多个对象形成树形结构 以表示具有部分-整体关系的层次结构
2、组合模式让客户端统一对待单个对象和组合对象
3、组合模式又称为部分-整体模式
4、将对象组织到树形结构中,可以用来描述整体与部分的关系
二、组合模式的结构
组合模式包含以下3个角色:
1、Component(抽象构件)
2、Leaf(叶子构件)
3、Composite(容器对象)
三、UML图
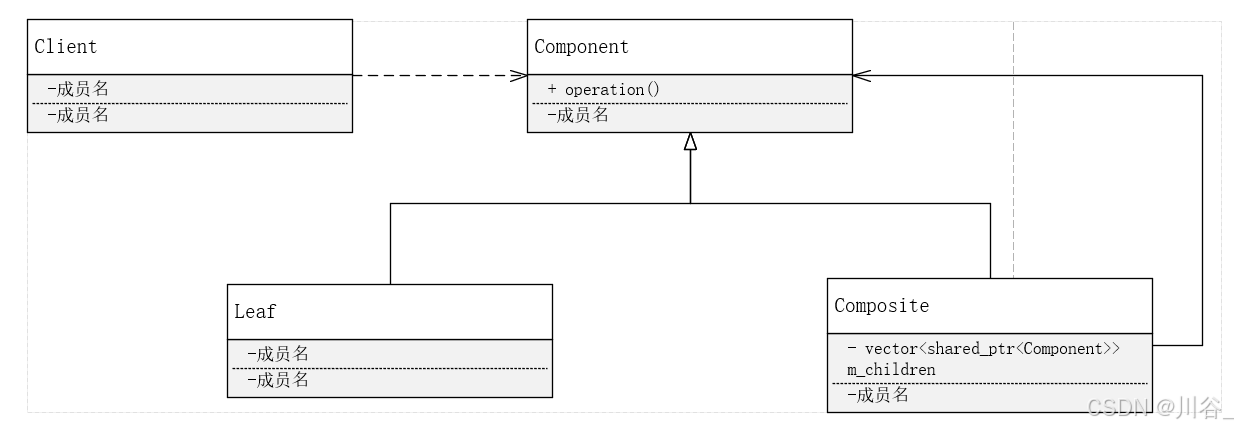
四、模式优点
1、可以清楚地定义分层次的复杂对象,表示对象的全部或部分层次,让客户端忽略了层次的差异,方便对整个层次结构进行控制
2、增加新的容器构件和叶子构件都很方便,符合开闭原则
3、为树形结构的面向对象实现提供了一种灵活的解决方案
五、模式缺点
1、在增加新构件时,很难对容器中的构件类型进行限制
六、示例代码
cpp
#include <iostream>
using namespace std;
#include <memory>
#include <vector>
//组件部件
class Component
{
public:
virtual void operation() const = 0;
virtual ~Component() = default;
};
//叶子节点
class Leaf : public Component
{
public:
void operation() const override
{
cout << "Leaf operation" << endl;
}
};
//组合节点
class Composite : public Component
{
public:
void add(const std::shared_ptr<Component>& component)
{
m_childen.push_back(component);
}
void operation() const override
{
cout << "Composite operation" << endl;
for (const auto& child : m_childen)
child->operation();
}
private:
vector<std::shared_ptr<Component>> m_childen;
};
int main()
{
//创建叶子节点
auto leaf1 = std::make_shared<Leaf>();
auto leaf2 = std::make_shared<Leaf>();
//创建组合节点
auto composite = std::make_shared<Composite>();
composite->add(leaf1);
composite->add(leaf2);
//执行操作
composite->operation();
return 0;
}