前言
HTML中的<template>标记是 Web 开发中一个功能强大但经常未得到充分利用的元素。它允许你定义可重复使用的内容,这些内容可以克隆并插入 DOM 中而无需最初渲染。
此功能对于创建动态、交互式 Web 应用程序特别有用。
在本文中,我们将探讨有效使用 <template> 标记的 10 个基本技巧,帮助你在项目中充分发挥其潜力。
1. 了解 <template> 标记的基础知识
<template> 标记是一个 HTML 元素,它包含页面加载时不会渲染的客户端内容。相反,可以使用 JavaScript 在稍后克隆内容并将其插入文档中。
html
<template id="my-template">
<div class="content">
<h2>Template Content</h2>
<p>This content is inside a template.</p>
</div>
</template>
为什么它很有用
通过使用 <template>,我们可以创建可重复使用的内容块,这些内容块存储在文档中,但在需要之前不会显示。这对于创建 UI 组件的多个实例或动态管理大型数据集等场景特别有用。
2. 利用 <template> 标记获取动态内容
<template> 标记最常见的用途之一是动态生成动态内容。例如,你可以克隆模板的内容并将其附加到 DOM 以显示从 API 获取的数据。
javascript
const template = document.getElementById('my-template');
const clone = template.content.cloneNode(true);
document.body.appendChild(clone);
最佳实践
-
确保唯一 ID:克隆内容时,请确保更新应保持唯一的 ID 或其他属性。
-
避免过度克隆:仅克隆您需要的内容以避免不必要的内存使用。
3. 使用 <template> 标记获取可重复使用的 UI 组件
<template> 标记非常适合创建可重复使用的 UI 组件,例如,模式窗口、工具提示或卡片。通过将组件结构存储在模板中,你可以轻松实例化多个实例而无需重复代码。
html
<template id="card-template">
<div class="card">
<h3>Card Title</h3>
<p>Card content goes here.</p>
</div>
</template>
<script>
const template = document.getElementById('card-template');
for (let i = 0; i < 3; i++) {
const clone = template.content.cloneNode(true);
document.body.appendChild(clone);
}
</script>
提示
-
模块化代码:将模板定义与 JavaScript 逻辑分开,以保持代码整洁有序。
-
克隆后自定义:修改克隆的内容(例如文本或属性)以创建组件的唯一实例。
4. 将 <template> 标记与 JavaScript 框架集成
许多现代 JavaScript 框架和库(如 React、Vue 和 Angular)都使用其模板系统。但是,原生 <template> 标记在某些情况下仍然有用,例如,当需要管理框架生命周期之外的内容时。
使用 Vanilla JavaScript 的示例
javascript
const template = document.getElementById('my-template');
const list = document.getElementById('item-list');
fetch('/api/items')
.then(response => response.json())
.then(items => {
items.forEach(item => {
const clone = template.content.cloneNode(true);
clone.querySelector('h2').textContent = item.title;
clone.querySelector('p').textContent = item.description;
list.appendChild(clone);
});
});
提示
将 <template> 标记用于边缘情况:在特定于框架的解决方案可能不够灵活的场景中使用 <template> 标记。
与 Web 组件结合:在自定义元素中使用 <template> 标记来定义影子 DOM 内容。
5. 使用 <template> 优化性能
<template> 标记对于性能优化很有用。由于 <template> 中的内容在克隆之前不会呈现,因此,可以通过延迟呈现非必要内容来减少初始页面加载时间。
html
<template id="deferred-content">
<div class="hidden-content">
<p>This content is loaded later.</p>
</div>
</template>
<script>
window.addEventListener('load', () => {
const template = document.getElementById('deferred-content');
const clone = template.content.cloneNode(true);
document.body.appendChild(clone);
});
</script>
最佳实践
-
延迟非关键内容:使用 <template> 加载用户不立即需要的内容。
-
延迟加载:将 <template> 与延迟加载技术相结合以进一步提高性能。
6. 使用 <template> 增强可访问性
使用 <template> 标签时,请注意可访问性。由于 <template> 中的内容在插入 DOM 之前不会呈现,因此,确保所有用户都可以访问克隆的内容非常重要。
提示
克隆后添加 ARIA 属性:确保在将模板内容插入 DOM 后添加任何必要的 ARIA 属性。
屏幕阅读器测试:使用屏幕阅读器测试你的应用程序以确保可访问克隆的内容。
javascript
const template = document.getElementById('accessible-template');
const clone = template.content.cloneNode(true);
clone.querySelector('.content').setAttribute('aria-live', 'polite');
document.body.appendChild(clone);
7. 使用 <template> 管理大型数据集
对于需要显示大型数据集的应用程序,<template> 标记可以帮助高效地管理 DOM。通过克隆模板而不是手动创建元素,可以保持代码整洁并优化 DOM 交互。
html
<template id="row-template">
<tr>
<td class="name"></td>
<td class="age"></td>
<td class="email"></td>
</tr>
</template>
<script>
const template = document.getElementById('row-template');
const tableBody = document.getElementById('table-body');
fetch('/api/users')
.then(response => response.json())
.then(users => {
users.forEach(user => {
const clone = template.content.cloneNode(true);
clone.querySelector('.name').textContent = user.name;
clone.querySelector('.age').textContent = user.age;
clone.querySelector('.email').textContent = user.email;
tableBody.appendChild(clone);
});
});
</script>
提示
-
高效渲染:使用 <template> 标记高效地渲染大型表格或列表。
-
批量更新:批量 DOM 更新以最大限度地减少重排和重绘。
8. 使用 <template> 来显示条件内容
<template> 标记非常适合需要根据用户交互或数据有条件地显示内容的场景。这允许你提前准备内容并在必要时显示它。
html
<template id="login-template">
<div class="login-form">
<input type="text" placeholder="Username">
<input type="password" placeholder="Password">
<button>Login</button>
</div>
</template>
<script>
const template = document.getElementById('login-template');
const loginContainer = document.getElementById('login-container');
document.getElementById('show-login').addEventListener('click', () => {
const clone = template.content.cloneNode(true);
loginContainer.appendChild(clone);
});
</script>
提示
-
预加载模板:预加载可能根据用户操作显示的内容以确保流畅的体验。
-
切换可见性:将 <template> 与可见性切换相结合以实现交互式元素。
9. 使用 <template> 简化表单处理
表单通常需要动态元素,例如,添加或删除输入字段。<template> 标签可让你在需要时克隆预定义的表单字段,从而简化此过程。
html
<template id="field-template">
<div class="form-field">
<input type="text" placeholder="Enter value">
<button class="remove-field">Remove</button>
</div>
</template>
<script>
const template = document.getElementById('field-template');
const form = document.getElementById('dynamic-form');
document.getElementById('add-field').addEventListener('click', () => {
const clone = template.content.cloneNode(true);
form.appendChild(clone);
});
form.addEventListener('click', event => {
if (event.target.classList.contains('remove-field')) {
event.target.closest('.form-field').remove();
}
});
</script>
提示
-
动态表单:使用 <template> 根据用户输入动态添加或删除表单字段。
-
表单验证:确保克隆的表单字段包含任何必要的验证逻辑。
10. 将 <template> 与 Web 组件结合使用
<template> 标签是创建 Web 组件不可或缺的一部分。通过将 <template> 与自定义元素结合使用,你可以在应用程序中封装和重用复杂的 UI 组件。
html
<template id="tooltip-template">
<style>
.tooltip {
position: absolute;
background: #333;
color: #fff;
padding: 5px;
border-radius: 4px;
font-size: 12px;
visibility: hidden;
transition: visibility 0.2s;
}
</style>
<div class="tooltip">
<slot></slot>
</div>
</template>
<script>
class TooltipElement extends HTMLElement {
constructor() {
super();
const template = document.getElementById('tooltip-template').content;
const shadowRoot = this.attachShadow({ mode: 'open' });
shadowRoot.appendChild(template.cloneNode(true));
}
connectedCallback() {
this.addEventListener('mouseover', () => {
this.shadowRoot.querySelector('.tooltip').style.visibility = 'visible';
});
this.addEventListener('mouseout', () => {
this.shadowRoot.querySelector('.tooltip').style.visibility = 'hidden';
});
}
}
customElements.define('my-tooltip', TooltipElement);
</script>
<!-- Usage of the custom tooltip element -->
<my-tooltip>
Hover over me
<span slot="tooltip-text">This is a tooltip</span>
</my-tooltip>
将 <template> 与 Web 组件结合使用的好处。
-
封装:将组件的样式和逻辑与应用程序的其余部分隔离开来。
-
可重用性:轻松在应用程序的不同部分重用复杂组件。
-
一致性:确保整个应用程序中 UI 元素的行为和外观一致。
<template> HTML 标签是一种多功能工具,可以显著改善你在 Web 应用程序中管理和操作 DOM 内容的方式。无论你是在创建可重复使用的组件、管理大型数据集还是优化性能,<template> 标记都提供了简洁高效的解决方案。
通过掌握本文讨论的技巧和技术,你可以充分利用 <template> 标记的潜力来构建更动态、更易于维护且性能更佳的 Web 应用程序。
在继续探索 <template> 标记的功能时,请考虑将其与 Web 组件和 JavaScript 框架等现代 Web 开发实践相结合,以创建更强大、更灵活的解决方案。
关于优联前端
武汉优联前端科技有限公司由一批从事前端10余年的专业人才创办,是一家致力于H5前端技术研究的科技创新型公司,为合作伙伴提供专业高效的前端解决方案,合作伙伴遍布中国及东南亚地区,行业涵盖广告,教育, 医疗,餐饮等。有效的解决了合作伙伴的前端技术难题,节约了成本,实现合作共赢。可进行Web前端,微信小程序、小游戏,2D/3D游戏,动画交互与UI广告设计等各种技术研发。
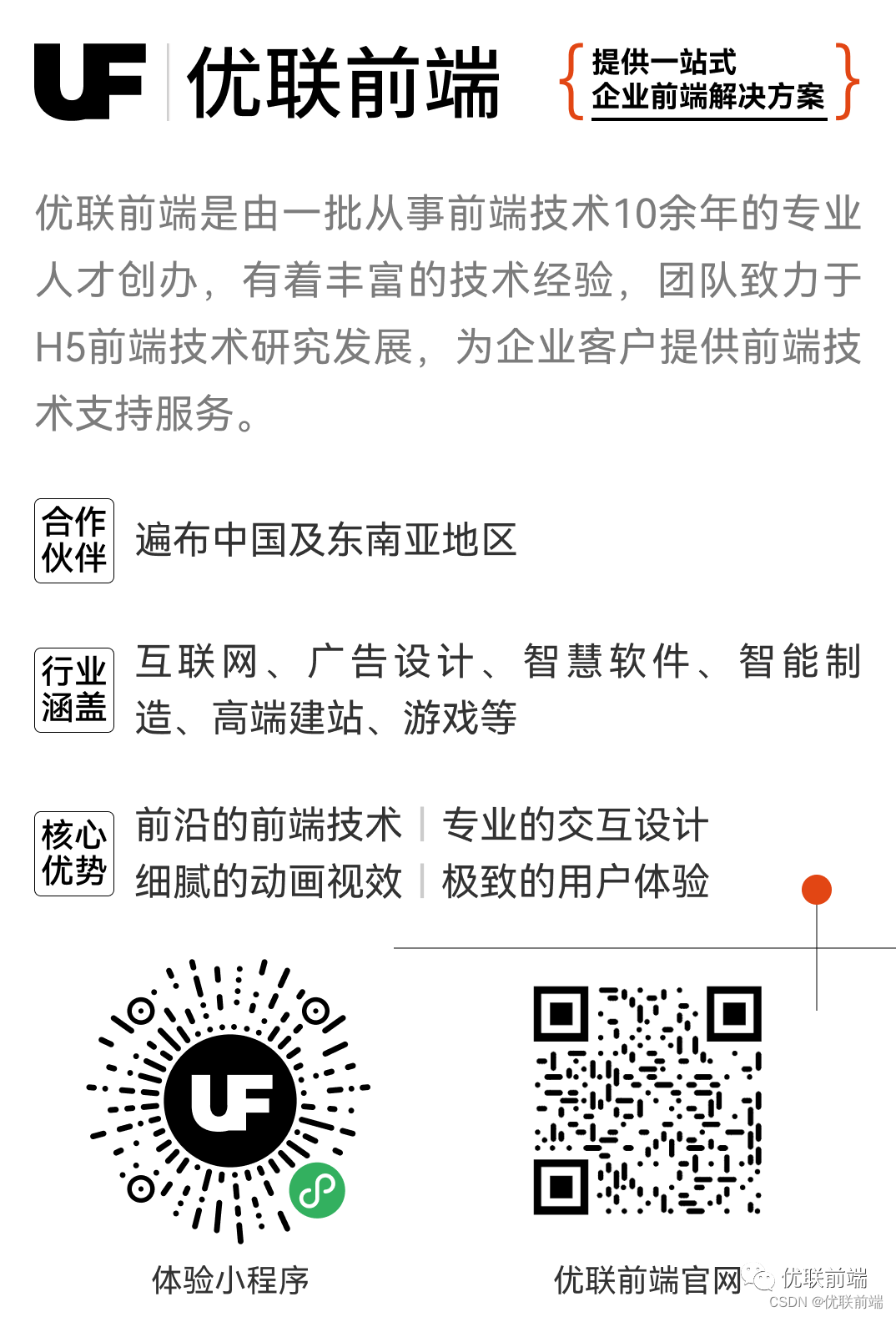