1、概述
需求,表格第一列指标可配置通过后端api传进来,表格显示数据以及鼠标触摸后气泡弹出层提示信息都是从后端传过来,实现动态表格的组件!!实现效果如下:
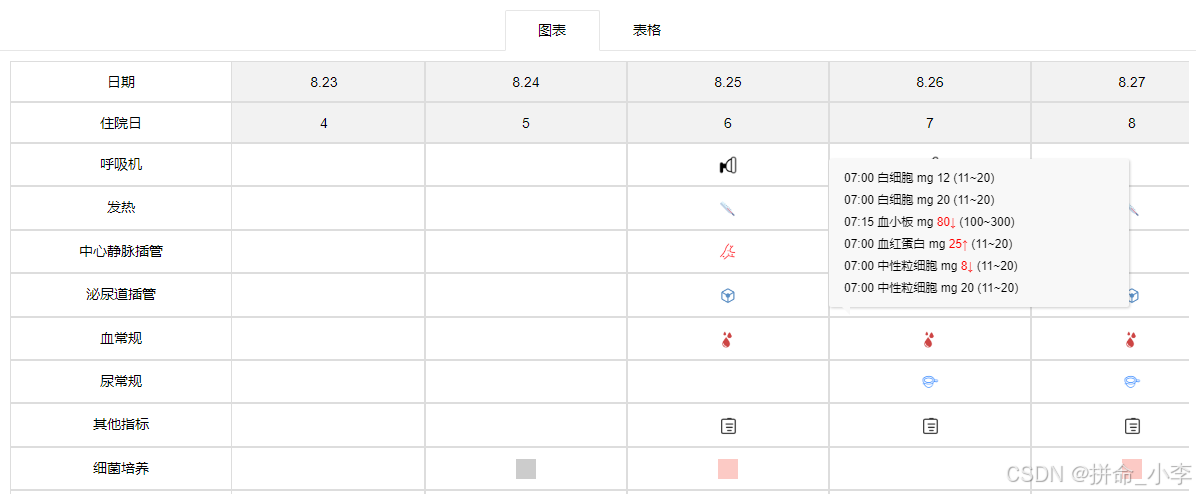
接口标准数据格式如下:
javascript
{
"data": {
"date": [
"8.20",
"8.21",
"8.22",
"8.23",
"8.24",
"8.25",
"8.26",
"8.27",
"8.28",
"8.29",
"8.30",
"8.31"
],
"hosDays": [
"1",
"2",
"3",
"4",
"5",
"6",
"7",
"8",
"9",
"10",
"11",
"12"
],
"maxWidth": "",
"tableData": {
"中心静脉插管": [
{
"id": "25",
"show": "",
"hide": ""
},
{
"id": "26",
"show": "",
"hide": ""
},
{
"id": "27",
"show": "",
"hide": ""
},
{
"id": "28",
"show": "",
"hide": ""
},
{
"id": "29",
"show": "",
"hide": ""
},
{
"id": "30",
"show": "<img style='width:20px;height:20px;' src='/pharmacistws/image/CENTER.jpg'/>",
"hide": ""
},
{
"id": "31",
"show": "<img style='width:20px;height:20px;' src='/pharmacistws/image/CENTER.jpg'/>",
"hide": ""
},
{
"id": "32",
"show": "",
"hide": ""
},
{
"id": "33",
"show": "",
"hide": ""
},
{
"id": "34",
"show": "",
"hide": ""
},
{
"id": "35",
"show": "",
"hide": ""
},
{
"id": "36",
"show": "",
"hide": ""
}
],
"血常规": [
{
"id": "49",
"show": "",
"hide": ""
},
{
"id": "50",
"show": "",
"hide": ""
},
{
"id": "51",
"show": "",
"hide": ""
},
{
"id": "52",
"show": "",
"hide": ""
},
{
"id": "53",
"show": "",
"hide": ""
},
{
"id": "54",
"show": "<img style='width:20px;height:20px;' src='/pharmacistws/image/BLOOD.jpg'/>",
"hide": "<div><span style='color:black'>07:00 白细胞 mg <span style='color:red'>10↓</span> (11~20) </span><br/><span style='color:black'>07:00 血小板 mg 100 (100~300) </span><br/><span style='color:black'>07:00 中性粒细胞 mg <span style='color:red'>10↓</span> (11~20) </span><br/></div>"
},
{
"id": "55",
"show": "<img style='width:20px;height:20px;' src='/pharmacistws/image/BLOOD.jpg'/>",
"hide": "<div><span style='color:black'>07:00 白细胞 mg 12 (11~20) </span><br/><span style='color:black'>07:00 白细胞 mg 20 (11~20) </span><br/><span style='color:black'>07:15 血小板 mg <span style='color:red'>80↓</span> (100~300) </span><br/><span style='color:black'>07:00 血红蛋白 mg <span style='color:red'>25↑</span> (11~20) </span><br/><span style='color:black'>07:00 中性粒细胞 mg <span style='color:red'>8↓</span> (11~20) </span><br/><span style='color:black'>07:00 中性粒细胞 mg 20 (11~20) </span><br/></div>"
},
{
"id": "56",
"show": "<img style='width:20px;height:20px;' src='/pharmacistws/image/BLOOD.jpg'/>",
"hide": "<div><span style='color:green'>未见异常</span></div>"
},
{
"id": "57",
"show": "",
"hide": ""
},
{
"id": "58",
"show": "",
"hide": ""
},
{
"id": "59",
"show": "",
"hide": ""
},
{
"id": "60",
"show": "",
"hide": ""
}
]
}
},
"r_code": 1
}
2、实现代码
js代码
javascript
// 渲染表格数据
function renderTable(eleIdName, eleIdName1, eleIdName2,data) {
var tableHeader = document.querySelector(eleIdName);
var dayNum = document.querySelector(eleIdName1);
var tableHide = document.getElementById(eleIdName2);
// 渲染列头
for (var i = 0; i < data.date.length; i++) {
var headerCell = document.createElement('div');
headerCell.classList.add('header-cell');
headerCell.textContent = data.date[i];
tableHeader.appendChild(headerCell);
var headerCell1 = document.createElement('div');
headerCell1.classList.add('header-cell');
headerCell1.textContent = data.hosDays[i];
dayNum.appendChild(headerCell1);
}
// 渲染数据
var dataHtml = '';
for (var key in data.tableData) {
dataHtml += '<div class="table-row">';
dataHtml += '<div class="cell">';
dataHtml += key;
dataHtml += '</div>';
for (var i = 0; i < data.tableData[key].length; i++) {
dataHtml += '<div class="cell" id="'+data.tableData[key][i].id+'" onmouseover="tableShow('+data.tableData[key][i].id+')" onmouseout="tableCloseTip()">';
dataHtml += data.tableData[key][i].show;
dataHtml += '</div>';
var newElement = document.createElement('div');
newElement.id= "hide_"+data.tableData[key][i].id;
newElement.innerHTML = data.tableData[key][i].hide;
tableHide.appendChild(newElement);
}
dataHtml += '</div>';
}
dayNum.insertAdjacentHTML('afterend', dataHtml);
}
function tableShow(d) {
layui.use(['layer'],function(){
var layer = layui.layer;
var hide = document.getElementById("hide_"+d);
if(hide.innerHTML!=='') {
tips = layer.tips(hide.innerHTML, "#"+d, {
tips: [1, '#F8F8F8'],
area: ['300px','auto'],
time: 60000
});
// $("#layui-layer" + tips).css("position","fixed");
}
});
}
function tableCloseTip () {
layui.use(['layer'],function(){
var layer = layui.layer;
layer.close(tips);
});
}
如何使用
javascript
var tableHtml = ' <div style="overflow-x: auto;">\n' +
' <div class="table">\n' +
' <div class="table-header" id="tableHeader">\n' +
' <div class="header-cell">日期</div>\n' +
' <!-- 更多列头 -->\n' +
' </div>\n' +
' <div class="table-header" id="inHosDay">\n' +
' <div class="header-cell">住院日</div>\n' +
' </div>\n' +
' <!-- 更多单元格 -->\n' +
' </div>\n' +
' <div id="tableHide" style="display:none">\n' +
'\n' +
' </div>\n' +
' </div>'
$("#table1").append(tableHtml);
renderTable("#tableHeader","#inHosDay",'tableHide',ret.data);
注意需要引用layui组件