思维导图:
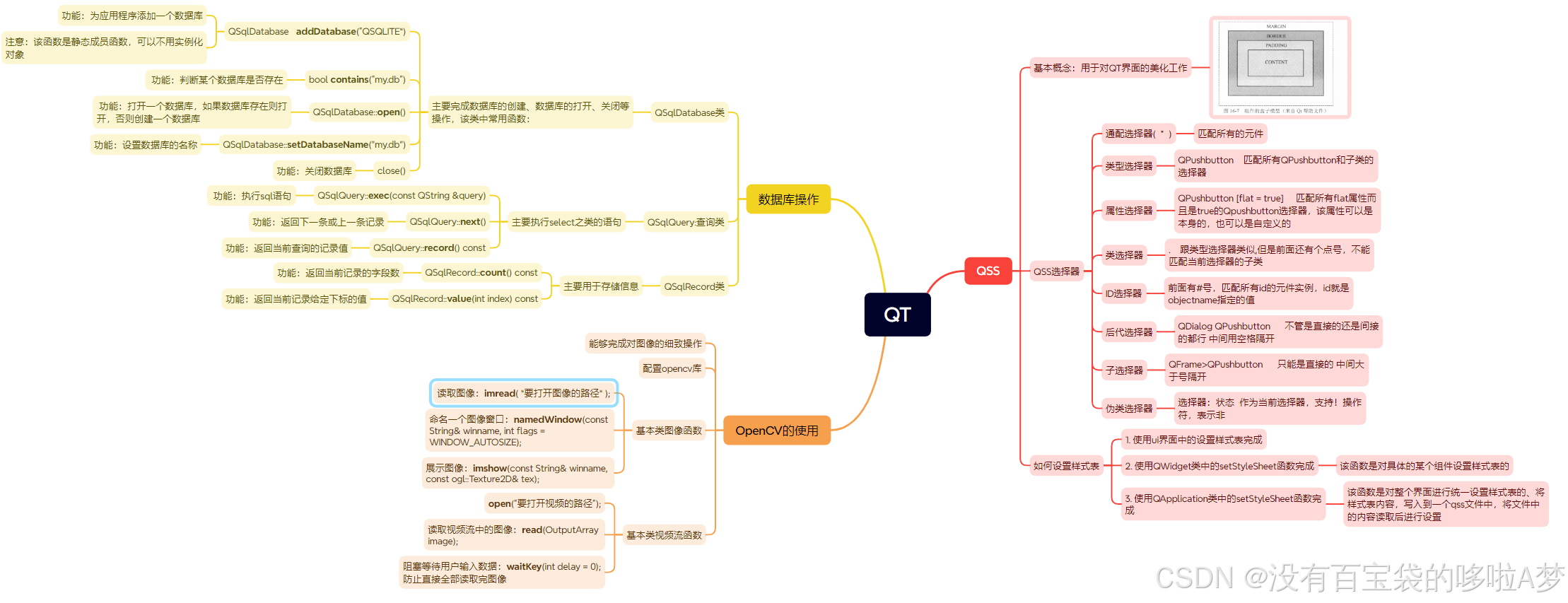
学习内容:
1. QSqlDatabase:数据库管理类
主要完成数据库的创建、数据库的打开、关闭等操作
该类中常用函数:
1、 QSqlDatabase addDatabase(const QString &type, const QString &connectionName = QLatin1String(defaultConnection))
功能:为应用程序添加一个数据库,如果数据库不存在则添加,如果数据库存在,则直接构造
参数:数据库驱动类型
2、 bool contains(const QString &connectionName = QLatin1String(defaultConnection))
功能:判断某个数据库是否存在
参数:数据库的名称 返回值:存在返回真,否则返回假
3、bool QSqlDatabase::open()
功能:打开一个数据库,如果数据库存在则打开,否则创建一个数据库
参数:无 返回值:bool类型,成功打开返回true失败返回false
4、void QSqlDatabase::setDatabaseName(const QString &name)
功能:设置数据库的名称
参数:数据库名称 返回值:无
2. QSqlQuerry:查询类
能完成对select语句的执行
该类常用的函数
1、bool QSqlQuery::exec(const QString &query)
功能:执行sql语句,并将结果放入到该类的对象中以便于后期的使用
参数:要执行的sql语句
返回值:成功执行返回true,失败返回false
2、bool QSqlQuery::next() bool QSqlQuery::previous()
功能:返回下一条或上一条记录
参数:无
返回值:bool类型
3、QSqlRecord QSqlQuery::record() const
功能:返回当前查询的记录值
参数:无
返回值:QSqlRecord类对象
3. QSqlRecord类
1> 该类用于存储某一条记录的信息
2> 常用函数
1、int QSqlRecord::count() const
功能:返回当前记录的字段的个数
参数:无
返回值:字段的个数
2、QVariant QSqlRecord::value(int index) const
功能:返回给定下标的字段的值
参数:字段的下标
返回值:给定下标的字段值
头文件
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include<QSqlDatabase> //数据库管理类
#include<QSqlQuery> //数据库查询类
#include<QSqlError> //数据库错误信息类
#include<QSqlRecord> //数据库记录类
QT_BEGIN_NAMESPACE
namespace Ui { class Widget; }
QT_END_NAMESPACE
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
private slots:
void on_addBtn_clicked();
void on_showBtn_clicked();
private:
Ui::Widget *ui;
QSqlDatabase db; //使用无参构造,实例化一个数据库管理类对象
};
#endif // WIDGET_H
源文件
#include "widget.h"
#include "ui_widget.h"
#include<QMessageBox>
#include<QDebug>
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
//当界面被关闭时,断开数据库的连接
connect(this, &Widget::destroyed, [&](){
db.close();
});
//1、添加数据库
db = QSqlDatabase::addDatabase("QSQLITE"); //添加的是sqlite3的数据库驱动
//2、设置数据库的名称
db.setDatabaseName("my.db");
//4、打开数据库
if(!db.open())
{
QMessageBox::information(this,"提示","数据库打开失败");
return;
}
//完成对数据表的创建,需要一个sql语句的执行者
QSqlQuery querry; //实例化对象
//准备sql语句
QString sql = "create table if not exists Stu(id int, name char, score double);";
//执行sql语句
if(!querry.exec(sql))
{
QMessageBox::information(this,"提示","数据表创建失败");
return;
}
}
Widget::~Widget()
{
delete ui;
}
//添加信息对应的槽函数
void Widget::on_addBtn_clicked()
{
//1、获取ui界面上的信息
int ui_id = ui->idEdit->text().toInt(); //id号
QString ui_name = ui->nameEdit->text(); //name
double ui_score = ui->scoreEdit->text().toDouble(); //score
//判断是否某个数据没有填写
if(ui_id==0 || ui_name.isEmpty() || ui_score==0)
{
QMessageBox::information(this,"提示","请将信息填写完整");
return;
}
//2、准备sql语句
QString sql =tr("insert into Stu(id, name, score) values(%1, '%2', %3);").arg(ui_id).arg(ui_name).arg(ui_score);
//qDebug()<<sql;
//3、准备语句执行者
QSqlQuery querry;
if(!querry.exec(sql))
{
QMessageBox::information(this,"提示","数据添加失败");
return;
}
QMessageBox::information(this,"提示","数据添加成功");
}
//展示信息按钮对应的槽函数
void Widget::on_showBtn_clicked()
{
ui->tableWidget->clearContents(); //清空表格内容
//准备sql语句
QString sql;
if(ui->nameEdit->text().isEmpty())
{
sql = "select * from Stu;"; //表示查询所有表中信息
}else
{
sql = tr("select * from Stu where name='%1';").arg(ui->nameEdit->text());
}
qDebug()<<sql;
//准备sql执行者
QSqlQuery querry;
if(!querry.exec(sql))
{
QMessageBox::information(this,"提示","查询失败");
qDebug() << "失败原因:"<< querry.lastError().text();
return;
}
//程序执行至此,查询结果保存在querry类对象中了
//可以调用该类的成员函数next进行遍历该结果集
int i = 0;
while(querry.next())
{
//循环中表示,querry指向的任意一条记录
//可以调用成员函数record函数获取当前指向的记录数据:querry.record
//querry.record().count():返回当前记录的字段个数
//querry.record().value(i):表示的是当前记录的下标为i的字段的值
//qDebug()<<querry.record().value(1).toString();
//将查询的数据展示到ui界面
//准备数据容器
for(int j=0; j<querry.record().count(); j++)
{
QTableWidgetItem *item = new QTableWidgetItem(querry.record().value(j).toString());
//将当前项目设置到 ui界面的(i,j)下标处
ui->tableWidget->setItem(i,j, item);
}
i++; //表示遍历下一行
}
}