qrc.py
把gpt_qrc.qrc转化成gpt_qrc.py
python
pyrcc5 -o icons_rc.py icons.qrc
python
<RCC>
<qresource prefix="img">
<file>img/53.png</file>
<file>img/ai.png</file>
<file>img/关闭.png</file>
<file>img/最小化.png</file>
</qresource>
</RCC>
UI.py
python
# -*- coding: utf-8 -*-
# Form implementation generated from reading ui file 'gpt_ui.ui'
#
# Created by: PyQt5 UI code generator 5.15.9
#
# WARNING: Any manual changes made to this file will be lost when pyuic5 is
# run again. Do not edit this file unless you know what you are doing.
from PyQt5 import QtCore, QtGui, QtWidgets
class Ui_Form(object):
def setupUi(self, Form):
Form.setObjectName("Form")
Form.resize(828, 874)
self.label = QtWidgets.QLabel(Form)
self.label.setGeometry(QtCore.QRect(80, 180, 651, 501))
self.label.setStyleSheet("\n"
"border-image: url(:/img/img/53.png);\n"
"border-radius:45px;")
self.label.setObjectName("label")
self.frame = QtWidgets.QFrame(Form)
self.frame.setGeometry(QtCore.QRect(510, 180, 217, 52))
self.frame.setStyleSheet("QPushButton{\n"
" border:none;\n"
"}\n"
"QPushButton:hover{\n"
" padding-bottom:5px;\n"
"}")
self.frame.setFrameShape(QtWidgets.QFrame.StyledPanel)
self.frame.setFrameShadow(QtWidgets.QFrame.Raised)
self.frame.setObjectName("frame")
self.horizontalLayout = QtWidgets.QHBoxLayout(self.frame)
self.horizontalLayout.setObjectName("horizontalLayout")
self.pushButton = QtWidgets.QPushButton(self.frame)
self.pushButton.setText("")
icon = QtGui.QIcon()
icon.addPixmap(QtGui.QPixmap(":/img/img/最小化.png"), QtGui.QIcon.Normal, QtGui.QIcon.Off)
self.pushButton.setIcon(icon)
self.pushButton.setObjectName("pushButton")
self.horizontalLayout.addWidget(self.pushButton)
self.pushButton_2 = QtWidgets.QPushButton(self.frame)
self.pushButton_2.setText("")
icon1 = QtGui.QIcon()
icon1.addPixmap(QtGui.QPixmap(":/img/img/关闭.png"), QtGui.QIcon.Normal, QtGui.QIcon.Off)
self.pushButton_2.setIcon(icon1)
self.pushButton_2.setObjectName("pushButton_2")
self.horizontalLayout.addWidget(self.pushButton_2)
self.lineEdit = QtWidgets.QLineEdit(Form)
self.lineEdit.setGeometry(QtCore.QRect(206, 590, 391, 51))
font = QtGui.QFont()
font.setFamily("微软雅黑")
font.setPointSize(10)
self.lineEdit.setFont(font)
self.lineEdit.setStyleSheet("border:none;\n"
"border:2px solid rgb(0,0,0);\n"
"border-radius:5px;\n"
"background:transparent;")
self.lineEdit.setText("")
self.lineEdit.setObjectName("lineEdit")
self.pushButton_3 = QtWidgets.QPushButton(Form)
self.pushButton_3.setGeometry(QtCore.QRect(614, 583, 55, 65))
self.pushButton_3.setStyleSheet("QPushButton{\n"
" border:none;\n"
"}\n"
"QPushButton:hover{\n"
" padding-bottom:5px;\n"
"}")
self.pushButton_3.setText("")
icon2 = QtGui.QIcon()
icon2.addPixmap(QtGui.QPixmap(":/img/img/ai.png"), QtGui.QIcon.Normal, QtGui.QIcon.Off)
self.pushButton_3.setIcon(icon2)
self.pushButton_3.setIconSize(QtCore.QSize(50, 50))
self.pushButton_3.setObjectName("pushButton_3")
self.textBrowser = QtWidgets.QTextBrowser(Form)
self.textBrowser.setGeometry(QtCore.QRect(170, 250, 491, 291))
font = QtGui.QFont()
font.setFamily("幼圆")
font.setPointSize(12)
self.textBrowser.setFont(font)
self.textBrowser.setStyleSheet("background:transparent; border:none; border - style:outset")
self.textBrowser.setObjectName("textBrowser")
self.comboBox = QtWidgets.QComboBox(Form)
self.comboBox.setGeometry(QtCore.QRect(130, 590, 71, 22))
self.comboBox.setStyleSheet("background:transparent;")
self.comboBox.setObjectName("comboBox")
self.comboBox_2 = QtWidgets.QComboBox(Form)
self.comboBox_2.setGeometry(QtCore.QRect(130, 620, 71, 22))
self.comboBox_2.setStyleSheet("background:transparent;")
self.comboBox_2.setObjectName("comboBox_2")
self.retranslateUi(Form)
self.pushButton.clicked.connect(Form.showMinimized) # type: ignore
self.pushButton_2.clicked.connect(Form.close) # type: ignore
QtCore.QMetaObject.connectSlotsByName(Form)
def retranslateUi(self, Form):
_translate = QtCore.QCoreApplication.translate
Form.setWindowTitle(_translate("Form", "顾某"))
self.label.setText(_translate("Form", "、"))
self.lineEdit.setPlaceholderText(_translate("Form", "输入问题:"))
应用
python
#该程序不会保存语音文件,在线播放
import requests,os,io
from gpt_ui import Ui_Form #UI
import sys
from PyQt5 import QtGui
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtCore import Qt, QPoint, QThread,pyqtSignal
import gpt_qrc #qrc生成的py #样式表
from pygame import mixer
import pygame.mixer
#设置任务栏
import ctypes
import hashlib #用于生成sign
import time
ctypes.windll.shell32.SetCurrentProcessExplicitAppUserModelID("com.example.myapp")
pygame.mixer.init() # 语音初始化
#子线程下载
class MyThread(QThread):
name_received = pyqtSignal(str) # 定义信号,传递 str 类型的参数
def __init__(self,ai,s,voice):
super().__init__()
#生成sign
self.timestamp = str(int(time.time() * 1000)) # 将时间戳转换为毫秒
public_secret_key = ""
# 构造签名字符串
signature_string = f"{self.timestamp}:{s}:{public_secret_key}"
hash_object = hashlib.sha256(signature_string.encode('utf-8'))
# 获取十六进制形式的哈希值 hash_hex
self.sign = hash_object.hexdigest()
self.url0 = 'http://api.ztk1.cn/API/Chat3.5.php?msg=' + s + '&id=1&type=1' #gptmini
# self.url0 = 'https://api.lolimi.cn/API/AI/gpt4o.php?sx=&msg=' +s #回答网址参数
self.url1 = 'https://api.zrzh.asia/api/ChatGPT.php?message='+s+'&id=10001'
self.url2 = 'http://api.xyovo.top/API/ChatGPT.php?message='+s+'&id=123&type=text'
self.url3= 'https://claude3.free2gpt.xyz/api/generate'
self.ai = ai
self.data3 ={
"messages": [
{
"role": "user",
"content": s
}
],
"time": self.timestamp,
"sign": self.sign
}
# self.voice_url = 'https://api.lolimi.cn/API/yyhc/'+voice+'.php?msg=' #语音合成网址
# shengyin=https://api.yujn.cn/api/yuyin.php?type=json&from=丁真&msg=我测尼玛
self.voice_url = f'https://api.yujn.cn/api/yuyin.php?type=json&from={voice}&msg=' #语音合成网址
# self.voice = voice
def run(self):
try:
if self.ai==0:
message = requests.post(self.url0).json().get('data').get('content')#dwai 0125
elif self.ai==1:
message = requests.post(self.url1).json().get('data').get('content')#dwai 0125
elif self.ai==2:
message = requests.get(self.url2).text
elif self.ai==3:
message = requests.get(self.url3,json=self.data3).text #api 4.0
self.name_received.emit(message)
print(message)
# #判断 message 的长度是否小于30
# if len(message) < 20:
# try:
# # voice_url = f'https://api.yujn.cn/api/yuyin.php?type=json&from={voice}&msg={message}'
# voice_url = self.voice_url + message
# audio_response = requests.get(voice_url) # 请求音频
# if audio_response.status_code == 200:
# # 将音频数据包装成类文件对象(BytesIO对象)
# audio_data = io.BytesIO(audio_response.content)
# # 加载音频数据并播放
# pygame.mixer.music.load(audio_data)
# pygame.mixer.music.play()
# self.name_received.emit(message)
# # 等待音乐播放完毕
# while pygame.mixer.music.get_busy():
# pygame.time.Clock().tick(10)
# else:
# self.name_received.emit(message)
# print('音频文件请求失败')
# except Exception as e:
# self.name_received.emit(message)
# print("播放失败")
# else:
# self.name_received.emit(message)
except Exception as e:
self.name_received.emit(f'请求错误: {str(e)}')
class guWindow(QWidget):
def __init__(self):
super().__init__()
self.gu = Ui_Form()
self.gu.setupUi(self)
comboBox = self.gu.comboBox
comboBox.addItem("懒羊羊")
comboBox.addItem("科比")
comboBox.addItem("丁真")
comboBox.addItem("陈泽")
comboBox1=self.gu.comboBox_2
# 添加下拉框选项
comboBox1.addItem("1")
comboBox1.addItem("2")
comboBox1.addItem("3")
comboBox1.addItem("4")
an = self.gu.pushButton_3 # 按钮
self.gu.lineEdit.returnPressed.connect(self.gumou) #lineEdit回车运行
an.clicked.connect(self.gumou) # 给按钮绑定函数
self.user_name_qwidget = self.gu.lineEdit
# ✦✦✦✦✦✦✦✦✦✦设置无边框 和可拖动✦✦✦✦✦✦✦✦✦✦✦✦✦固定代码
self.setWindowOpacity(0.90) # 设置窗口透明度
self.setWindowFlag(Qt.FramelessWindowHint) # 去除边框
self.setAttribute(Qt.WA_TranslucentBackground) # 去除白色背景
self.offset = QPoint() # 记录鼠标按下的初始位置
def mousePressEvent(self, event):
self.offset = event.pos()
def mouseMoveEvent(self, event):
if event.buttons() == Qt.LeftButton:
self.move(self.pos() + event.pos() - self.offset) # 移动窗口位置
def gumou(self): # 按钮绑定的函数 功能
s = self.user_name_qwidget.text()
self.user_name_qwidget.clear()
index = self.gu.comboBox.currentIndex() #下拉框选择器
if index == 0:
voice = "懒羊羊"
elif index == 1:
voice = "科比"
elif index == 2:
voice = "丁真"
elif index == 3:
voice = "陈泽"
ai = self.gu.comboBox_2.currentIndex() # 下拉框选择器
if ai == 0:
ai=0
elif ai == 1:
ai=1
elif ai == 2:
ai=2
elif ai == 3:
ai=3
self.my_thread = MyThread(ai,s,voice) # 创建线程
self.my_thread.name_received.connect(self.receive_name) # 连接信号
self.my_thread.start() # 开始线程
self.gu.textBrowser.clear()
self.gu.textBrowser.append("提问:"+s)
def receive_name(self, name): #接收name
self.gu.textBrowser.append('\n'+name)
if __name__ == '__main__':
app = QApplication(sys.argv)
icon = QtGui.QIcon(':/img/img/ai.png')
app.setWindowIcon(icon)
# 创建可拖动窗口实例
ui = guWindow() #函数
# 显示窗口
ui.show()
# 启动应用程序事件循环
sys.exit(app.exec_())
上述所需图片
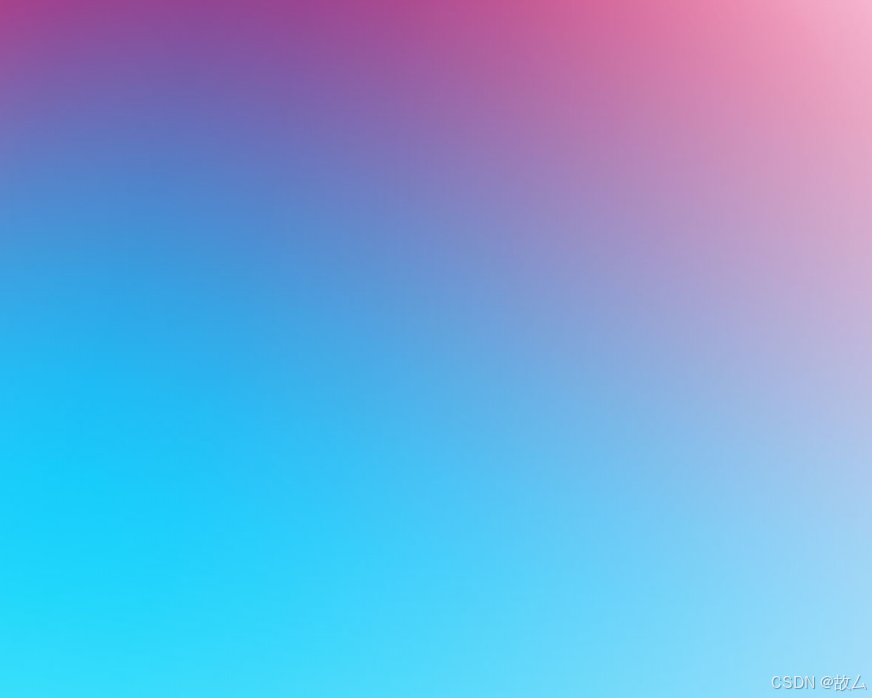
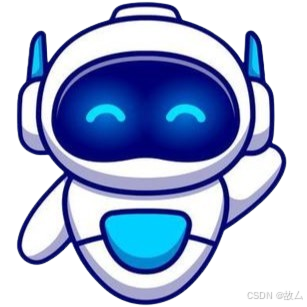