1、环境配置
系统:windows10系统
QT:版本5.15.2 编译器:MSVC2019_64bit 编辑器:QT Creator
OSG版本:3.7.0 64位 为MSVC环境下编译
osgQt:为第三方编译的库,OSG因为版本不同已经不提供osgQt的封装。
osg-earth版本: 3.6.0
2、如何导入相关的库
衔接:QT+OSG+osg-earth显示一个球-CSDN博客
QT的pro文件:
cpp
QT += core gui
QT +=opengl
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
CONFIG += c++17
# You can make your code fail to compile if it uses deprecated APIs.
# In order to do so, uncomment the following line.
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0
SOURCES += \
main.cpp \
osgshowwidget.cpp
HEADERS += \
osgshowwidget.h
FORMS += \
osgshowwidget.ui
# Default rules for deployment.
qnx: target.path = /tmp/$${TARGET}/bin
else: unix:!android: target.path = /opt/$${TARGET}/bin
!isEmpty(target.path): INSTALLS += target
win32:CONFIG(release, debug|release): LIBS += -L$$PWD/osg370MSVC2019_64bit/OSG/lib/ -losg -losgDB -losgUtil -losgViewer -losgGA -losgWidget -lOpenThreads
else:win32:CONFIG(debug, debug|release): LIBS += -L$$PWD/osg370MSVC2019_64bit/OSG/lib/ -losgd -losgDBd -losgUtild -losgViewerd -losgGAd -losgWidgetd -lOpenThreadsd
else:unix: LIBS += -L$$PWD/osg370MSVC2019_64bit/OSG/lib/ -losg -losgDB -losgUtil -losgViewer -losgGA -losgWidget -lOpenThreads
INCLUDEPATH += $$PWD/osg370MSVC2019_64bit/OSG/include
DEPENDPATH += $$PWD/osg370MSVC2019_64bit/OSG/include
win32:CONFIG(release, debug|release): LIBS += -L$$PWD/osgQtVC2019_64bit/vs2019_x64_osgQt/osgQt/lib/ -losgQOpenGL
else:win32:CONFIG(debug, debug|release): LIBS += -L$$PWD/osgQtVC2019_64bit/vs2019_x64_osgQt/osgQt/lib/ -losgQOpenGLd
else:unix: LIBS += -L$$PWD/osgQtVC2019_64bit/vs2019_x64_osgQt/osgQt/lib/ -losgQOpenGL
INCLUDEPATH += $$PWD/osgQtVC2019_64bit/vs2019_x64_osgQt/osgQt/include
DEPENDPATH += $$PWD/osgQtVC2019_64bit/vs2019_x64_osgQt/osgQt/include
win32:CONFIG(release, debug|release): LIBS += -L$$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/lib/ -losgEarth
else:win32:CONFIG(debug, debug|release): LIBS += -L$$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/lib/ -losgEarthd
else:unix: LIBS += -L$$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/lib/ -losgEarth
INCLUDEPATH += $$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/include
DEPENDPATH += $$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/include
win32:CONFIG(release, debug|release): LIBS += -L$$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/lib/ -losgEarth
else:win32:CONFIG(debug, debug|release): LIBS += -L$$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/lib/ -losgEarthd
else:unix: LIBS += -L$$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/lib/ -losgEarth
INCLUDEPATH += $$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/include
DEPENDPATH += $$PWD/vs2019_x64_osgearth_3.6.0/OSGEARTH/include
3、代码部分
.h
cpp
#ifndef OSGSHOWWIDGET_H
#define OSGSHOWWIDGET_H
#include <QWidget>
#include <osgViewer/Viewer>
#include <osg/Node>
#include <osgDB/ReadFile>
#include <osgGA/TrackballManipulator>
#include<osgGA/FlightManipulator>
#include <osgViewer/View>
#include <osg/ArgumentParser>
#include <osgGA/CameraManipulator>
#include <osgEarth/EarthManipulator>
#include <osgEarth/Map>
#include <osgEarth/MapNode>
#include <osgEarth/TMS>
#include <osgEarth/EarthManipulator>
#include <osg/ArgumentParser>
#include <osgDB/ReadFile>
#include <osgGA/TrackballManipulator>
#include<osgGA/FlightManipulator>
#include <osgViewer/View>
#include <osgQOpenGL/osgQOpenGLWidget>
#include <osg/Timer>
#include <osg/ArgumentParser>
#include <osgGA/CameraManipulator>
namespace Ui {
class osgShowWidget;
}
class osgShowWidget : public QWidget
{
Q_OBJECT
public:
explicit osgShowWidget(QWidget *parent = nullptr);
~osgShowWidget();
protected slots:
void initWindow();
protected:
osgQOpenGLWidget* _pOsgQOpenGLWidget;
osg::ref_ptr<osgViewer::Viewer> pViewer;
private:
Ui::osgShowWidget *ui;
};
#endif // OSGSHOWWIDGET_H
.cpp
cpp
#include "osgshowwidget.h"
#include "ui_osgshowwidget.h"
#include <QCoreApplication>
#include <QApplication>
#include <QDir>
#include <QString>
#include <QDebug>
osgShowWidget::osgShowWidget(QWidget *parent) :
QWidget(parent),
ui(new Ui::osgShowWidget)
{
ui->setupUi(this);
this->move(0,0);
ui->widget->move(0,0);
_pOsgQOpenGLWidget = new osgQOpenGLWidget(ui->widget);
_pOsgQOpenGLWidget->setGeometry(ui->widget->geometry());
connect(_pOsgQOpenGLWidget, SIGNAL(initialized()), this, SLOT(initWindow()));
}
osgShowWidget::~osgShowWidget()
{
delete ui;
if(_pOsgQOpenGLWidget != NULL)
delete _pOsgQOpenGLWidget;
}
void osgShowWidget::initWindow()
{
// osgViewer::Viewer* pViewer = _pOsgQOpenGLWidget->getOsgViewer();
// pViewer->setCameraManipulator(new osgGA::TrackballManipulator);
// osg::Node* node = osgDB::readNodeFile("model/F-22.ive");
// pViewer->setSceneData(node);
QString exeDir = QCoreApplication::applicationDirPath();
QDir::setCurrent(exeDir);
osgEarth::initialize();
osgViewer::Viewer* pViewer = _pOsgQOpenGLWidget->getOsgViewer();
//初始化View
//pViewer = getOsgViewer();
//设置相机
pViewer->setCameraManipulator(new osgEarth::Util::EarthManipulator);
osg::ref_ptr<osg::Group> root; //根节点
osg::ref_ptr<osg::Node> earth; //地球节点
root = new osg::Group;
QString relativeEarthFilePath = "Maps/ocean.earth"; // 请替换为你实际的.earth文件相对路径
QString earthFilePath = exeDir + "/" + relativeEarthFilePath;
earth = osgDB::readNodeFile(earthFilePath.toStdString());
if (!earth.valid())
qDebug() << "File not found";
root->addChild(earth.get());
pViewer->setSceneData(root.get());
pViewer->realize();
}
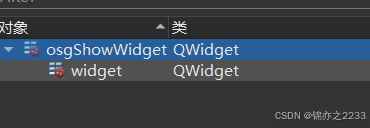
main.cpp
cpp
#include <QCoreApplication>
#include <QApplication>
#include <QDir>
#include "osgshowwidget.h"
#include <osgEarth/Map>
#include <osgEarth/MapNode>
#include <osgEarth/EarthManipulator>
#include <osgEarth/MapNode>
#include <osgEarth/TMS>
#include <osgEarth/EarthManipulator>
#include <osg/ArgumentParser>
#include <osgViewer/Viewer>
#include <QWidget>
#include <osgViewer/Viewer>
#include <osg/Node>
#include <osgDB/ReadFile>
#include <osgGA/TrackballManipulator>
#include<osgGA/FlightManipulator>
#include <osgViewer/View>
#include <osgQOpenGL/osgQOpenGLWidget>
#include <osg/Timer>
#include <osg/ArgumentParser>
#include <osgGA/CameraManipulator>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// // 获取可执行文件的目录
osgShowWidget w;
w.show();
// 初始化osgViewer
// 初始化osgEarth
// osgEarth::initialize();
// osg::ArgumentParser args(&argc, argv);
// // 创建Viewer实例
// osgViewer::Viewer viewer(args);
// // 使用相对于exe的相对路径来构建.earth文件的完整路径
// QString relativeEarthFilePath = "Maps/ocean.earth"; // 请替换为你实际的.earth文件相对路径
// QString earthFilePath = exeDir + "/" + relativeEarthFilePath;
// // 请替换为你实际的.earth文件路径
// osg::ref_ptr<osg::Node> earthNode = osgDB::readNodeFile(earthFilePath.toStdString());
// if (!earthNode) {
// std::cerr << "Failed to load .earth file: " << earthFilePath.toStdString() << std::endl;
// return -1; // 如果加载失败,返回错误码
// }
// // 设置场景数据为加载的.earth文件
// viewer.setSceneData(earthNode.get());
// // 设置地球操控器
// viewer.setCameraManipulator(new osgEarth::Util::EarthManipulator(args));
// // 运行Viewer
// return viewer.run();
return a.exec();
}
4、运行结果
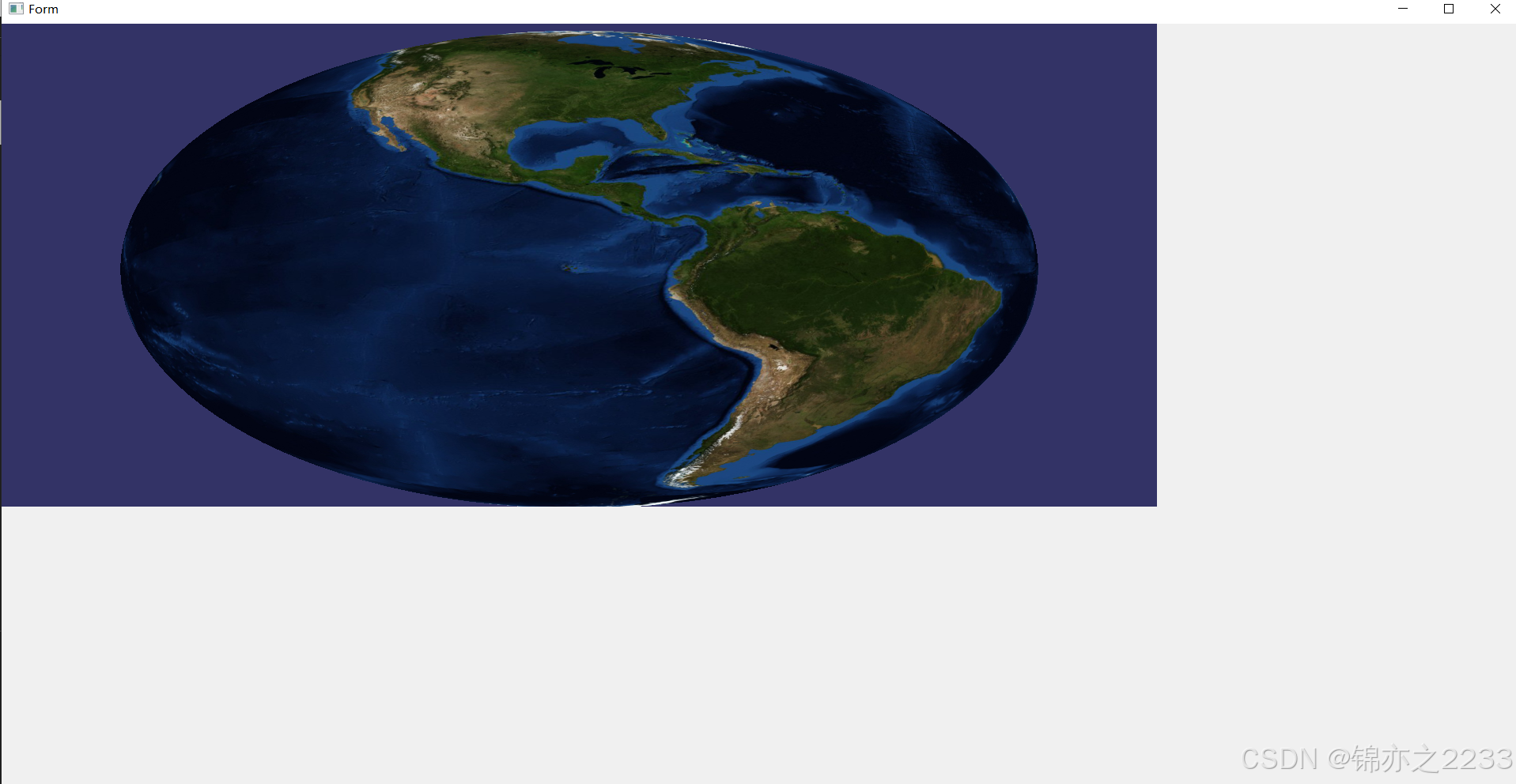
5、出现的问题
不知道还qt的bug还是什么问题,我的窗口直接show出来会发生偏移,窗口出现的位置默认就不是在0.0处,必须写代码,move移动到0.0才行,这应该是qt兼容性问题,不是我代码的问题,所以我的代码有一部分对于正常显示qt界面也就不需要。