第二天:DOM事件基础,注册事件,tab栏切换
添加事件监听
html
<body>
<button>点击</button>
<script>
const btn = document.querySelector('button')
btn.addEventListener('click', function () {
alert('嗲你')
})
</script>
</body>
addEventListener
分为事件源,事件类型,事件处理程序
关闭广告
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.box {
margin: 100px;
width: 400px;
height: 200px;
background-color: pink;
font-size: 50px;
text-align: center;
}
.box1 {
position: absolute;
width: 20px;
height: 20px;
background-color: #fff;
left: 480px;
top: 120px;
text-align: center;
cursor: pointer;
}
</style>
</head>
<body>
<div class="box">广告</div>
<div class="box1">X</div>
<script>
const box1 = document.querySelector('.box1')
const box = document.querySelector('.box')
box1.addEventListener('click', function () {
box.style.display = 'none'
box1.style.display = 'none'
})
</script>
</body>
</html>
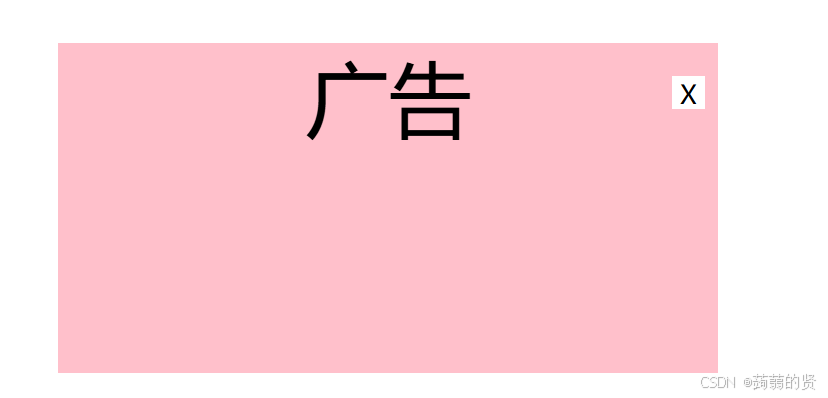
事件监听
随机点名
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
h2 {
text-align: center;
}
.box {
width: 600px;
margin: 50px auto;
display: flex;
font-size: 25px;
line-height: 40px;
}
.qs {
width: 450px;
height: 40px;
color: red;
}
.btns {
text-align: center;
}
.btns button {
width: 120px;
height: 35px;
margin: 0 50px;
}
</style>
</head>
<body>
<h2>随机点名</h2>
<div class="box">
<span>名字是:</span>
<div class="qs">这里显示姓名</div>
</div>
<div class="btns">
<button class="start">开始</button>
<button class="end">结束</button>
</div>
<script>
let timerId = 0
let random = 0
// 数据数组
const arr = ['马超', '黄忠', '赵云', '关羽', '张飞']
const qs = document.querySelector('.qs')
const start = document.querySelector('.start')
start.addEventListener('click', function () {
timerId = setInterval(function () {
random = parseInt(Math.random() * arr.length)
qs.innerHTML = arr[random]
}, 100)
if (arr.length === 1) {
start.disabled = true
end.disabled = true
}
})
const end = document.querySelector('.end')
end.addEventListener('click', function () {
clearInterval(timerId)
arr.splice(random, 1)
})
</script>
</body>
</html>
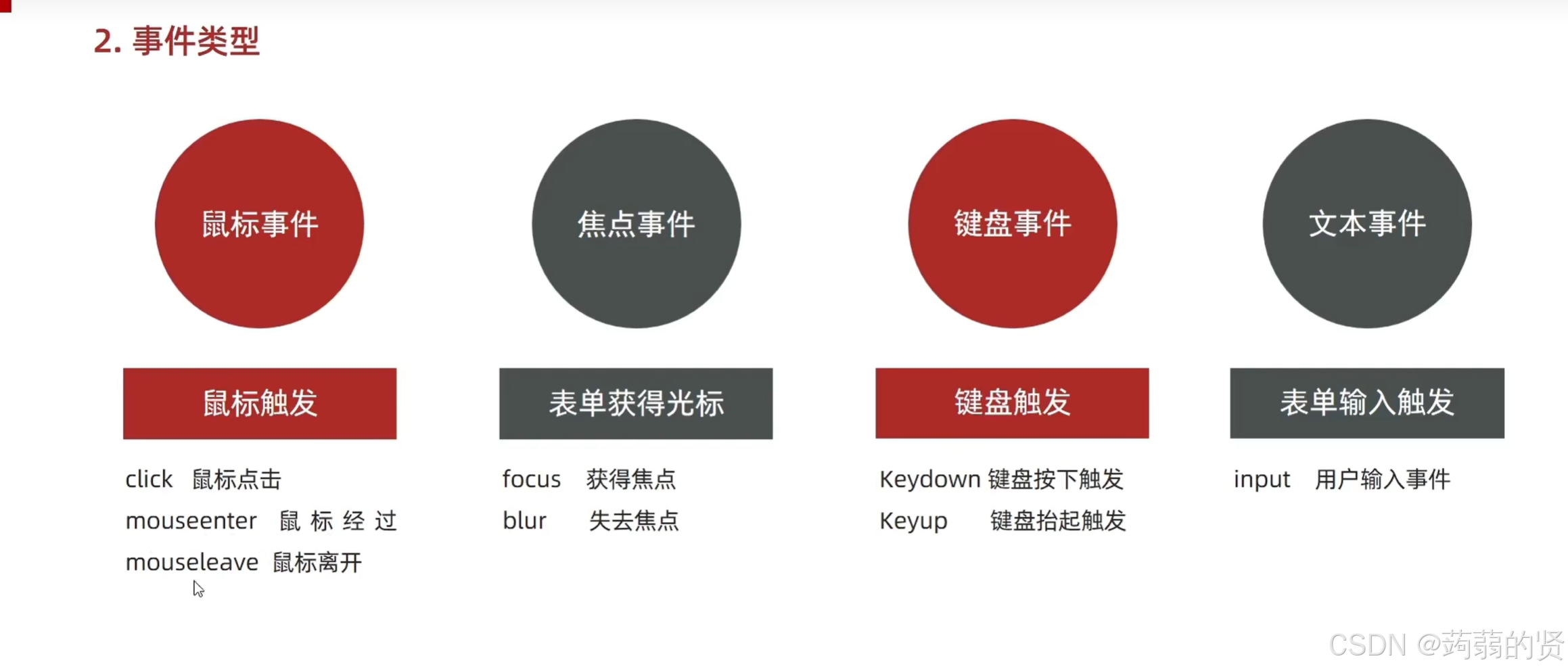
鼠标事件
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 200px;
height: 200px;
background-color: pink;
}
</style>
</head>
<body>
<div></div>
<script>
const div = document.querySelector('div')
div.addEventListener('mouseenter', function () {
console.log('我来了');
})
div.addEventListener('mouseleave', function () {
console.log('我走了');
})
</script>
</body>
</html>
我来了我走了,鼠标经过事件
焦点事件
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="text" name="" id="">
<script>
const input = document.querySelector('input')
input.addEventListener('focus', function () {
console.log('触发焦点');
})
input.addEventListener('blur', function () {
console.log('失去焦点');
})
</script>
</body>
</html>
小米搜索框
html
!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
ul {
list-style: none;
}
.mi {
position: relative;
width: 223px;
margin: 100px auto;
}
.mi input {
width: 223px;
height: 48px;
padding: 0 10px;
font-size: 14px;
line-height: 48px;
border: 1px solid #e0e0e0;
outline: none;
}
.mi .search {
border: 1px solid #ff6700;
}
.result-list {
display: none;
position: absolute;
left: 0;
top: 48px;
width: 223px;
border: 1px solid #ff6700;
border-top: 0;
background: #fff;
}
.result-list a {
display: block;
padding: 6px 15px;
font-size: 12px;
color: #424242;
text-decoration: none;
}
.result-list a:hover {
background-color: #eee;
}
</style>
</head>
<body>
<div class="mi">
<input type="search" placeholder="小米笔记本">
<ul class="result-list">
<li><a href="#">全部商品</a></li>
<li><a href="#">小米11</a></li>
<li><a href="#">小米10S</a></li>
<li><a href="#">小米笔记本</a></li>
<li><a href="#">小米手机</a></li>
<li><a href="#">黑鲨4</a></li>
<li><a href="#">空调</a></li>
</ul>
</div>
<script>
const input = document.querySelector('[type=search]')
const ul = document.querySelector('.result-list')
input.addEventListener('focus', function () {
ul.style.display = 'block'
input.classList.add('search')
})
input.addEventListener('blur', function () {
ul.style.display = 'none'
input.classList.remove('search')
})
</script>
</body>
</html>
键盘事件
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="text" name="" id="">
<script>
const input = document.querySelector('input')
input.addEventListener('keydown', function () {
console.log('键盘按下了');
})
input.addEventListener('keyup', function () {
console.log('键盘弹起了');
})
</script>
</body>
</html>
键盘按下,键盘弹起
html
<body>
<input type="text" name="" id="">
<script>
const input = document.querySelector('input')
input.addEventListener('input', function () {
console.log(input.value);
})
</script>
</body>
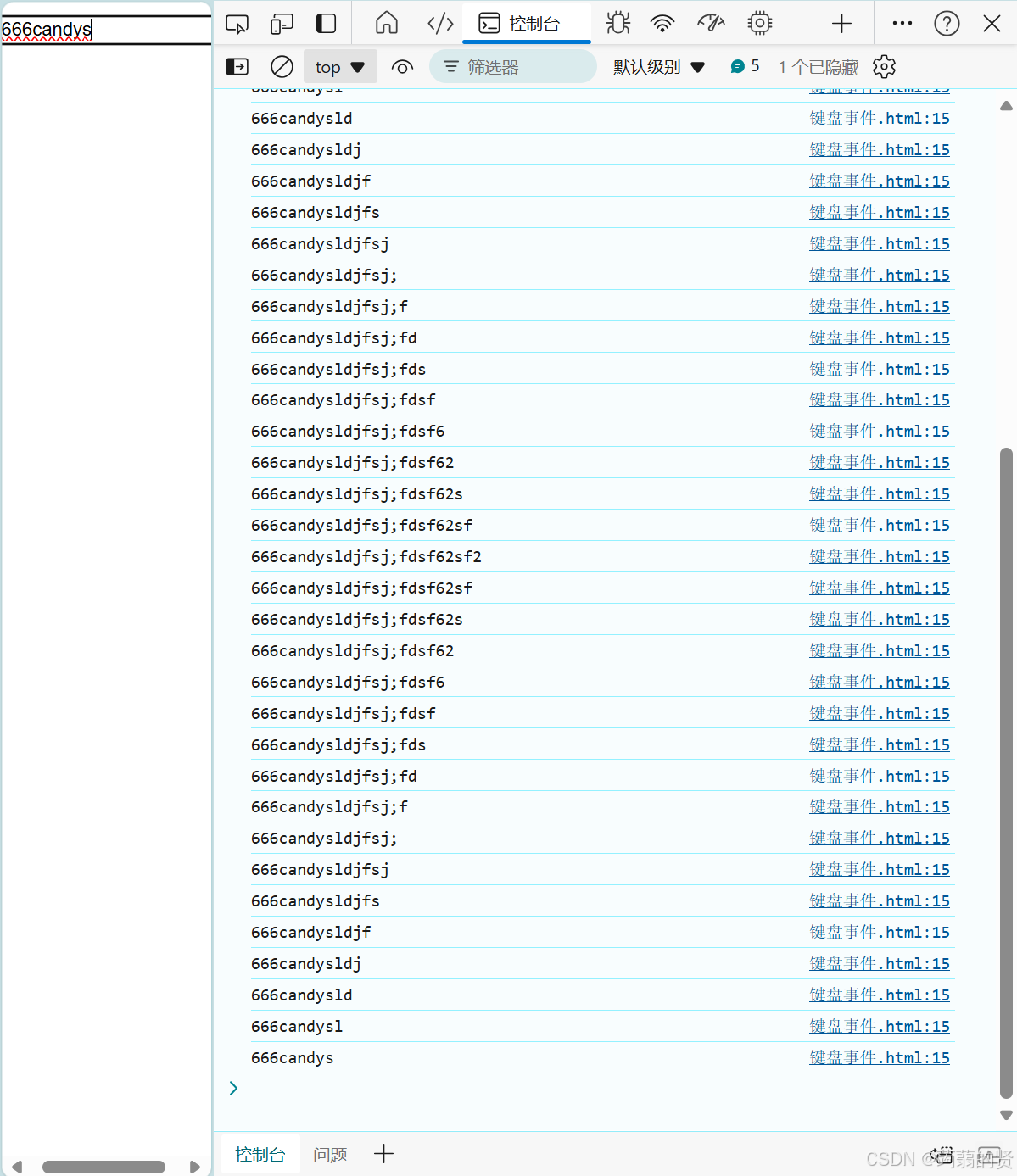
文本事件
复选框
html
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
* {
margin: 0;
padding: 0;
}
table {
border-collapse: collapse;
border-spacing: 0;
border: 1px solid #c0c0c0;
width: 500px;
margin: 100px auto;
text-align: center;
}
th {
background-color: #09c;
font: bold 16px "微软雅黑";
color: #fff;
height: 24px;
}
td {
border: 1px solid #d0d0d0;
color: #404060;
padding: 10px;
}
.allCheck {
width: 80px;
}
</style>
</head>
<body>
<table>
<tr>
<th class="allCheck">
<input type="checkbox" name="" id="checkAll"> <span class="all">全选</span>
</th>
<th>商品</th>
<th>商家</th>
<th>价格</th>
</tr>
<tr>
<td>
<input type="checkbox" name="check" class="ck">
</td>
<td>小米手机</td>
<td>小米</td>
<td>¥1999</td>
</tr>
<tr>
<td>
<input type="checkbox" name="check" class="ck">
</td>
<td>小米净水器</td>
<td>小米</td>
<td>¥4999</td>
</tr>
<tr>
<td>
<input type="checkbox" name="check" class="ck">
</td>
<td>小米电视</td>
<td>小米</td>
<td>¥5999</td>
</tr>
</table>
<script>
const checkAll = document.querySelector('#checkAll')
const cks = document.querySelectorAll('.ck')
checkAll.addEventListener('click', function () {
//遍历小复选框
//让小复选框的checked===大复选框checked
for (let i = 0; i < cks.length; i++) {
cks[i].checked = checkAll.checked
}
})
</script>
</body>
</html>
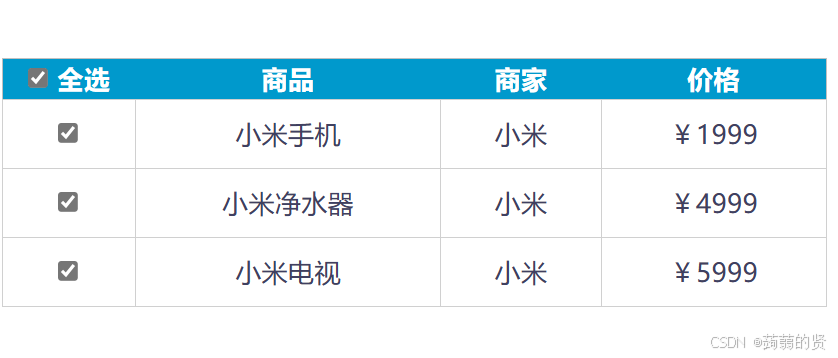
事件对象
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button>点击</button>
<script>
const btn = document.querySelector('button')
btn.addEventListener('click', function (e) {
console.log(e);
})
</script>
</body>
</html>
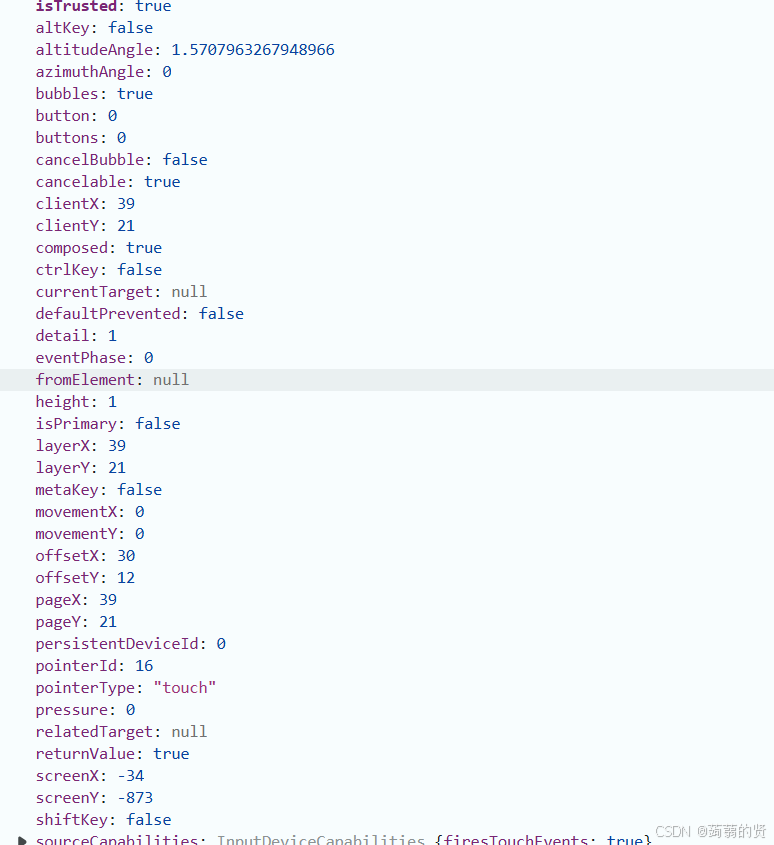