1.继承的概念
继承是一个类继承另外一个类,称继承的类为子类/派生类,被继承的类称为父类/基类。
比如下面两个类,Student和Person,Student称为子类,Person称为父类。
#include<iostream>
using namespace std;
class Person
{
public:
void identity()
{
cout << "void identity()" << _name << endl;
}
public:
string _name = "李四";
string _tel;
string _address;
int _age;
};
class Student : public Person
{
public:
void study()
{
//...
}
public:
int ID;
};
2.继承的定义
1.2.1定义格式
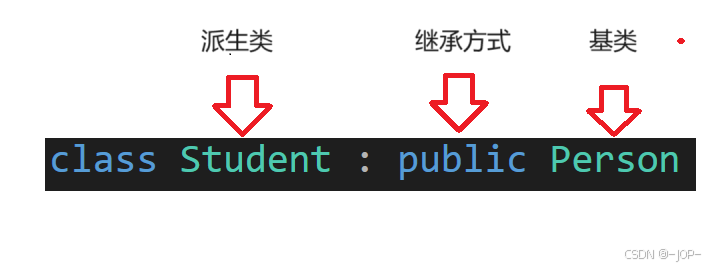
1.2.2继承基类成员访问方式的变化
|--------------------|-----------------|-----------------|-----------------|
| 类成员/继承方式 | public继承 | protected继承 | public继承 |
| 基类的public成员 | 派生类的public成员 | 派生类的public成员 | 派生类的public成员 |
| 基类的protected成员 | 派生类的protected成员 | 派生类的protected成员 | 派生类的protected成员 |
| 基类的private成员 | 派生类中不可见 | 派生类中不可见 | 派生类中不可见 |
解释:
- 基类的private无论以什么方式继承都不可见。注意:这里的不可见是指基类的私有成员还是被继承到派生类中,但是语法上限制派生类对象不管在类里面还是类外面都不能去访问它。
- 如果基类成员不想在类外直接被访问并想在派生类中访问,就定义为protected。
- public>protected>private
- 使用关键字class的默认继承方式是private,使用struct默认继承方式是public,但是为了方便阅读代码,最好显示写出继承方式。(class Student : Person->默认私有,struct Student : Person->默认共有)
1.3继承类模版
当父类是类模板时,一定注意要指定作用域
#include<iostream>
#include<vector>
using namespace std;
namespace AA
{
template<class T>
class stack : std::vector<T>
{
public:
void push(const T& x)
{
//push_back(x); //会报错,"push_back": 找不到标识符
//因为stack<int>实例化时,也间接实例化了vector<int>,但是模版是按需实例化,
//push_back成员函数未实例化,所以找不到
//所以当基类是类模板时,需要指定一下类域
vector<T>::push_back(x);
}
//...
};
}
int main()
{
AA::stack<int> st;//自动调用stack的构造l;
st.push(1);
st.push(2);
return 0;
}
3.基类和派生类之间的转换
public继承的派生对象 可以赋值给基类的指针/引用 。基类的指针/引用指向的是派生类中基类的那一部分。(并没有发生类型转换)
基类对象不能赋值给派生类对象
class Person
{
protected :
string _name; // 姓名
string _sex; // 性别
int _age; // 年龄
};
class Student : public Person
{
public :
int _No ; // 学号
};int main()
{
Student sobj ;
// 派⽣类对象可以赋值给基类的指针/引⽤
Person* pp = &sobj;
Person& rp = sobj;return 0;
}
4.继承中的作用域
4.1隐藏规则:
在继承体系中基类和派生类都用独立的作用域。
派生类和基类有同名成员,派生类成员将屏蔽基类对同名成员的直接访问,这种情况叫隐藏。(在派生类中,可以使用基类::基类成员显示访问)
注意如果是成员函数的隐藏,只需要函数名相同就构成子类隐藏父类。
class Person { protected: string _name = "张三"; int _id = 9090; }; class Student : public Person { public: void Print() { cout << _name << endl; cout << _id << endl;//生成子类对应9999 cout << Person::_id << endl;//生成父类对应9090 } protected: int _id = 9999; }; int main() { Student stu; stu.Print(); return 0; }
5.派生类的默认成员函数
5.1 6个常见的默认成员函数
-
派生类的构造函数必须调用基类的构造函数初始化基类的那一部分成员。如果基类没有默认的构造函数,则必须在派生类构造函数的初始化列表阶段显示调用。
class Person
{
public:
Person(const char* name = "peter")
: _name(name)
{
cout << "Person()" << endl;
}
protected:
string _name;
};class Student : public Person
{
public:
//默认生成的构造函数
//1、内置类型->看编译器,不确定
//2、自定义类型->调用默认构造
//3、继承父类成员看做一个中体对象,要求调用父类的默认构造
protected:
int _num;
string _address;
};int main()
{
Student stu;return 0;
}
i. 调试结果符合派生类派生类的构造函数必须调用基类的构造函数初始化基类的那一部分成员
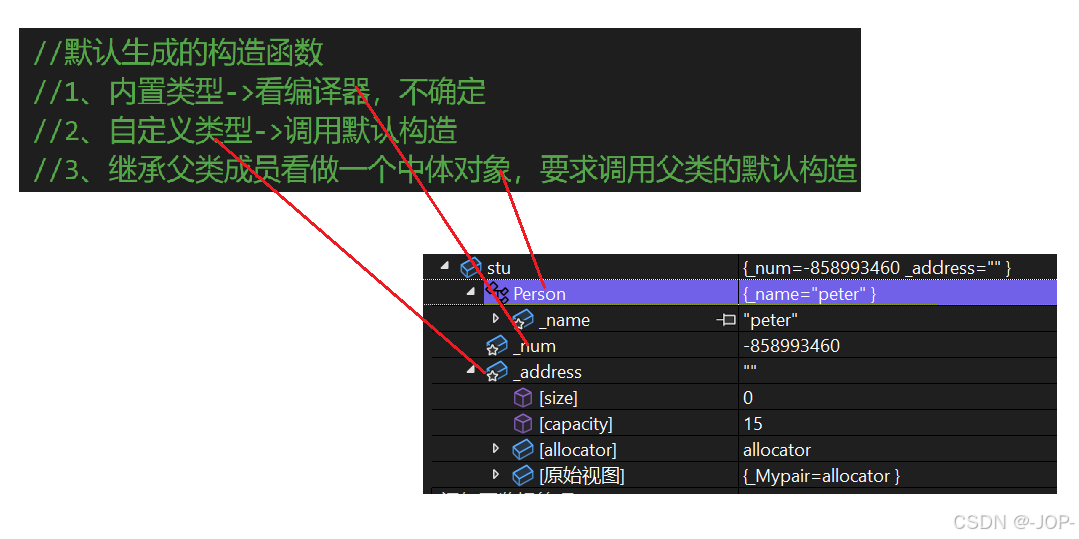
ii. 如果基类没有默认构造函数,则必须在派生类构造函数的初始化列表阶段显示调用
class Person
{
public:
Person(const char* name)
: _name(name)
{
cout << "Person()" << endl;
}
protected:
string _name;
};
class Student : public Person
{
public:
//默认生成的构造函数
//1、内置类型->看编译器,不确定
//2、自定义类型->调用默认构造
//3、继承父类成员看做一个中体对象,要求调用父类的默认构造
Student(const char* name, int num, const char* address)
:Person(name)//显式调用
,_num(num)
,_address(address)
{}
protected:
int _num;
string _address;
};
int main()
{
Student stu("王五",78,"二七区");
return 0;
}
-
派生类的拷贝构造必须调用基类的拷贝构造完成基类的拷贝初始化。
class Person
{
public:
Person(const char* name)
: _name(name)
{
cout << "Person()" << endl;
}
Person(const Person& p)
: _name(p._name)
{
cout << "Person(const Person& p)" << endl;
}
protected:
string _name;
};class Student : public Person
{
public:
//默认生成的构造函数
//1、内置类型->看编译器,不确定
//2、自定义类型->调用默认构造
//3、继承父类成员看做一个中体对象,要求调用父类的默认构造
Student(const char* name, int num, const char* address)
: Person(s)//当做整体对象,调用父类拷贝构造,同时,这里还有基类和派生类的转换
,_num(num)
,_address(address)
{}
//严格来说,Student的拷贝构造不用自己来完成,默认生成就够用
//除非有需要深拷贝的资源。比如:int* ptr = new int[10];
Student(const Student& s)
: Person(s)
, _num(s._num)
,_address(s._address)
{
cout << "Student(const Student& s)" << endl;
}
protected:
int _num;
string _address;
};int main()
{
Student stu1("王五",78,"二七区");
Student stu2(stu1);return 0;
}
-
派生类的operator=必须要调用基类的operator=完成operator=完成基类的赋值。但是要注意:派生类的operator=隐藏了父类的operator=,所以显示调用时,需要指定作用域。
class Person
{
public:
Person(const char* name)
: _name(name)
{
cout << "Person()" << endl;
}
Person(const Person& p)
: _name(p._name)
{
cout << "Person(const Person& p)" << endl;
}
Person& operator=(const Person& p)
{
cout << "Person operator=(const Person& p)" << endl;
if (this != &p)
_name = p._name;
return *this;
}
protected:
string _name;
};class Student : public Person
{
public:
//默认生成的构造函数
//1、内置类型->看编译器,不确定
//2、自定义类型->调用默认构造
//3、继承父类成员看做一个中体对象,要求调用父类的默认构造
Student(const char* name, int num, const char* address)
:Person(name)
,_num(num)
,_address(address)
{}//严格来说,Student的拷贝构造不用自己来完成,默认生成就够用 //除非有需要深拷贝的资源。比如:int* ptr = new int[10]; Student(const Student& s) : Person(s)//当做整体对象,调用父类拷贝构造,同时,这里还有基类和派生类的转换 , _num(s._num) ,_address(s._address) { cout << "Student(const Student& s)" << endl; } //严格来说,Student的赋值重载不用自己来完成,默认生成就够用 //除非有需要深拷贝的资源。比如:int* ptr = new int[10]; Student& operator=(const Student& s) { cout << "Student& operator= (const Student& s)" << endl; if (this != &s) { Person::operator=(s);//显示调用注意加作用域 _num = s._num; _address = s._address; } return *this; }
protected:
int _num;
string _address;
};int main()
{
Student stu1("王五",78,"二七区");
Student stu2(stu1);Student stu3("李四", 90, "高新区"); stu1 = stu3; return 0;
}
-
派生类的析构函数会在被调用完成后自动调用基类的析构函数清理基类成员。因为这样才能保证派生类对象先清理派生类成员再清理基类成员。顺序是:派生类对象析构先调用派生类析构再调用基类的析构。
class Person
{
public:
Person(const char* name)
: _name(name)
{
cout << "Person()" << endl;
}
Person(const Person& p)
: _name(p._name)
{
cout << "Person(const Person& p)" << endl;
}
Person& operator=(const Person& p)
{
cout << "Person operator=(const Person& p)" << endl;
if (this != &p)
_name = p._name;
return *this;
}
~Person()
{
cout << "~Person()" << endl;
}
protected:
string _name;
};class Student : public Person
{
public:
//默认生成的构造函数
//1、内置类型->看编译器,不确定
//2、自定义类型->调用默认构造
//3、继承父类成员看做一个中体对象,要求调用父类的默认构造
Student(const char* name, int num, const char* address)
:Person(name)
,_num(num)
,_address(address)
{}//严格来说,Student的拷贝构造不用自己来完成,默认生成就够用 //除非有需要深拷贝的资源。比如:int* ptr = new int[10]; Student(const Student& s) : Person(s)//当做整体对象,调用父类拷贝构造,同时,这里还有基类和派生类的转换 , _num(s._num) ,_address(s._address) { cout << "Student(const Student& s)" << endl; } //严格来说,Student的赋值重载不用自己来完成,默认生成就够用 //除非有需要深拷贝的资源。比如:int* ptr = new int[10]; Student& operator=(const Student& s) { cout << "Student& operator= (const Student& s)" << endl; if (this != &s) { Person::operator=(s);//显示调用注意加作用域 _num = s._num; _address = s._address; } return *this; } //严格来说,Student的析构函数不用自己来完成,默认生成就够用 //除非有需要显示释放的资源。比如:int* ptr = new int[10]; ~Student() { Person::~Person(); cout << "~Student()" << endl; }
protected:
int _num;
string _address;
};int main()
{
Student stu1("王五",78,"二七区");return 0;
}
当没有显示调用时,顺序是先调用派生类的析构再调用父类的析构。如果显示调用,看代码如何写,并不能保证先子后父。

- 派生类对象初始化先调用基类构造再调用派生类构造。
总结来看,顺序如下:
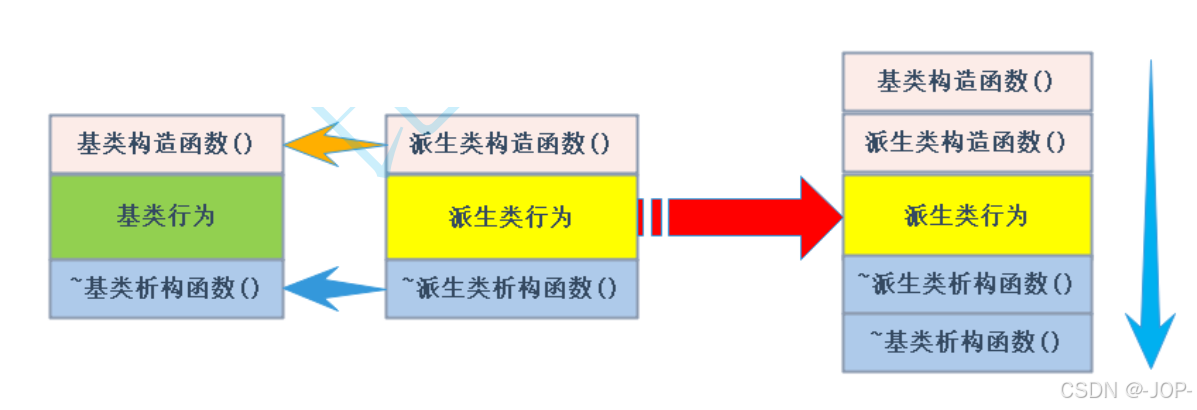
5.2实现一个不能被继承的类
法一:把父类的构造函数私有,子类的构成必须调用基类的构造函数,但是父类的构造被私有化后,子类就无法调用,这样一来,子类就无法实例化出对象。
法二:C++11新增了一个final关键字,子类就不能继承了
class A final//法二加final关键字
{
public:
void func1() { cout << "A::func1" << endl; }
protected:
int a = 1;
private:
// 法一
/*A()
{}*/
};
class B :public A
{
void func2() { cout << "B::func2" << endl; }
protected:
int b = 2;
};
int main()
{
A a;
B b;
return 0;
}
over~