Qt圆角窗口
问题:自己重写了一个窗口,发现用qss设置圆角了,但是都不生效,不过子窗口圆角都生效了。
无边框移动窗口
cpp
bool eventFilter(QObject *watched, QEvent *evt) {
static QPoint mousePoint;
static bool mousePressed = false;
QMouseEvent *event = static_cast<QMouseEvent *>(evt);
if (event->type() == QEvent::MouseButtonPress) {
if (event->button() == Qt::LeftButton) {
mousePressed = true;
mousePoint = event->globalPos() - this->pos();
return true;
} else {
return true;
}
} else if (event->type() == QEvent::MouseButtonRelease) {
mousePressed = false;
return true;
} else if (event->type() == QEvent::MouseMove) {
if (mousePressed && (event->buttons() && Qt::LeftButton)) {
this->move(event->globalPos() - mousePoint);
return true;
}
}
return QWidget::eventFilter(watched, event);
}
1.当前窗口背景隐藏,使用子窗口圆角
红色背景为原来的窗口,里面的子窗口可以看到有圆角窗口了,隐藏掉就行setAttribute(Qt::WA_TranslucentBackground);
实现效果:
窗口还是存在的,只是透明了
代码:
cpp
void StyleSheetWidget::setRoundedRectangle1()
{
setWindowFlags(Qt::FramelessWindowHint);
setAttribute(Qt::WA_TranslucentBackground);//隐藏背景
this->resize(200, 200);
QFrame* title = new QFrame;
QWidget* left = new QWidget;
QWidget* mid = new QWidget;
QWidget* right = new QWidget;
// 布局
QVBoxLayout *layoutMain = new QVBoxLayout;
layoutMain->setContentsMargins(10, 10, 10, 10);
QVBoxLayout *midLayout = new QVBoxLayout;
midLayout->setContentsMargins(0,0,0,0);
QWidget* midWid = new QWidget;
midWid->setLayout(midLayout);
layoutMain->addWidget(midWid);
QHBoxLayout *hLayout_1 = new QHBoxLayout;
hLayout_1->addWidget(left);
hLayout_1->addWidget(mid);
hLayout_1->addWidget(right);
midLayout->addWidget(title);
midLayout->addLayout(hLayout_1);
title->setStyleSheet("background-color:green;border-bottom:3px solid black;");
left->setStyleSheet("background-color:green;");
mid->setStyleSheet("background-color:yellow;");
right->setStyleSheet("background-color:blue;color:white");
QLabel* label = new QLabel();
label->setText("你好啊");
QFormLayout* formLayout = new QFormLayout;
formLayout->addRow(label);
formLayout->addRow(new QLabel("你真的好啊,白云"));
right->setLayout(formLayout);
this->setLayout(layoutMain);
this->setStyleSheet("border-radius: 8px;background-color:red;border: 1px solid #d9d9d9;");
}
2.使用paintEvent()绘制圆角
注意:使用paintEvent绘制圆角时候,布局之间要留一点距离,不然上层的绘制会把下面绘制的圆角给遮住了。如:
cpp
layoutMain->setContentsMargins(10,10,10,10);
设置布局间距离为10的效果:
设置为0效果:
cpp
QVBoxLayout *layoutMain = new QVBoxLayout;
layoutMain->setContentsMargins(0,0,0,0);
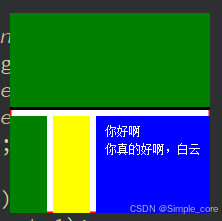
代码:
cpp
void StyleSheetWidget::paintEvent(QPaintEvent *event)
{
QPainter painter(this);
painter.setRenderHint(QPainter::Antialiasing);
painter.setBrush(QColor(255,255,255)); //背景颜色
//painter.setPen(Qt::red);//Qt::transparent); //边框颜色
QPen pen;
pen.setColor(Qt::red);
pen.setWidth(3);
painter.setPen(pen);
QRect rect = this->rect();
rect.setWidth(rect.width());
rect.setHeight(rect.height());
painter.drawRoundedRect(rect, 15, 15);
QWidget::paintEvent(event);
}