🎉 博客主页:【剑九_六千里-CSDN博客】【剑九_六千里-掘金社区】
🎨 上一篇文章:【HarmonyOS第七章:应用状态共享(PersistentStorage、LocalStorage、AppStorage)】
🎠 系列专栏:【HarmonyOS系列】
💖 感谢大家点赞👍收藏⭐评论✍
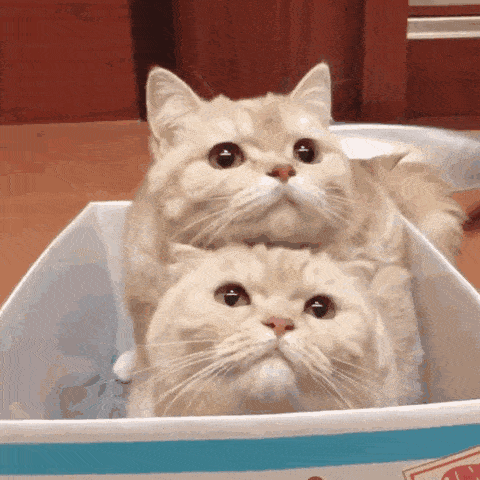

文章目录
- [1. http 介绍](#1. http 介绍)
-
- [1.1. http 基本概念](#1.1. http 基本概念)
- [1.2. http 提供的接口](#1.2. http 提供的接口)
- [1.3. request接口开发步骤](#1.3. request接口开发步骤)
- [2. 基本使用](#2. 基本使用)
-
- [2.1. 配置HTTP使用权限](#2.1. 配置HTTP使用权限)
- [2.2. 导入 http 模块](#2.2. 导入 http 模块)
- [2.3. 页面中使用](#2.3. 页面中使用)
1. http 介绍
1.1. http 基本概念
HTTP
数据请求功能主要由http
模块提供。
使用该功能需要申请ohos.permission.INTERNET
权限。
权限申请请参考访问控制(权限)开发指导。
1.2. http 提供的接口
涉及的接口如下表:
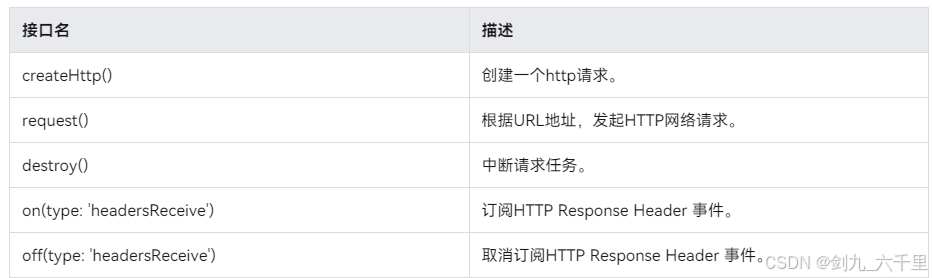
1.3. request接口开发步骤
- 从
@ohos.net.http
中导入http
命名空间。 - 调用
createHttp()
方法,创建一个HttpRequest
对象。 - 调用该对象的
on()
方法,订阅http
响应头事件,此接口会比request
请求先返回。可以根据业务需要订阅此消息 - 调用该对象的
request()
方法,传入http
请求的url
地址和可选参数,发起网络请求。 - 按照实际业务需要,解析返回结果。
- 调用该对象的
off()
方法,取消订阅http
响应头事件。 - 当该请求使用完毕时,调用
destroy()
方法主动销毁。
ts
// 引入包名
import http from '@ohos.net.http';
import { BusinessError } from '@ohos.base';
// 每一个httpRequest对应一个HTTP请求任务,不可复用
let httpRequest = http.createHttp();
// 用于订阅HTTP响应头,此接口会比request请求先返回。可以根据业务需要订阅此消息
// 从API 8开始,使用on('headersReceive', Callback)替代on('headerReceive', AsyncCallback)。
httpRequest.on('headersReceive', (header) => {
console.info('header: ' + JSON.stringify(header));
});
httpRequest.request(
// 填写HTTP请求的URL地址,可以带参数也可以不带参数。URL地址需要开发者自定义。请求的参数可以在extraData中指定
"EXAMPLE_URL",
{
method: http.RequestMethod.POST, // 可选,默认为http.RequestMethod.GET
// 开发者根据自身业务需要添加header字段
header: {
'Content-Type': 'application/json'
},
// 当使用POST请求时此字段用于传递请求体内容,具体格式与服务端协商确定
extraData: "data to send",
expectDataType: http.HttpDataType.STRING, // 可选,指定返回数据的类型
usingCache: true, // 可选,默认为true
priority: 1, // 可选,默认为1
connectTimeout: 60000, // 可选,默认为60000ms
readTimeout: 60000, // 可选,默认为60000ms
usingProtocol: http.HttpProtocol.HTTP1_1, // 可选,协议类型默认值由系统自动指定
usingProxy: false, // 可选,默认不使用网络代理,自API 10开始支持该属性
}, (err: BusinessError, data: http.HttpResponse) => {
if (!err) {
// data.result为HTTP响应内容,可根据业务需要进行解析
console.info('Result:' + JSON.stringify(data.result));
console.info('code:' + JSON.stringify(data.responseCode));
// data.header为HTTP响应头,可根据业务需要进行解析
console.info('header:' + JSON.stringify(data.header));
console.info('cookies:' + JSON.stringify(data.cookies)); // 8+
// 当该请求使用完毕时,调用destroy方法主动销毁
httpRequest.destroy();
} else {
console.error('error:' + JSON.stringify(err));
// 取消订阅HTTP响应头事件
httpRequest.off('headersReceive');
// 当该请求使用完毕时,调用destroy方法主动销毁
httpRequest.destroy();
}
}
);
2. 基本使用
2.1. 配置HTTP使用权限
entry/src/main/module.json5
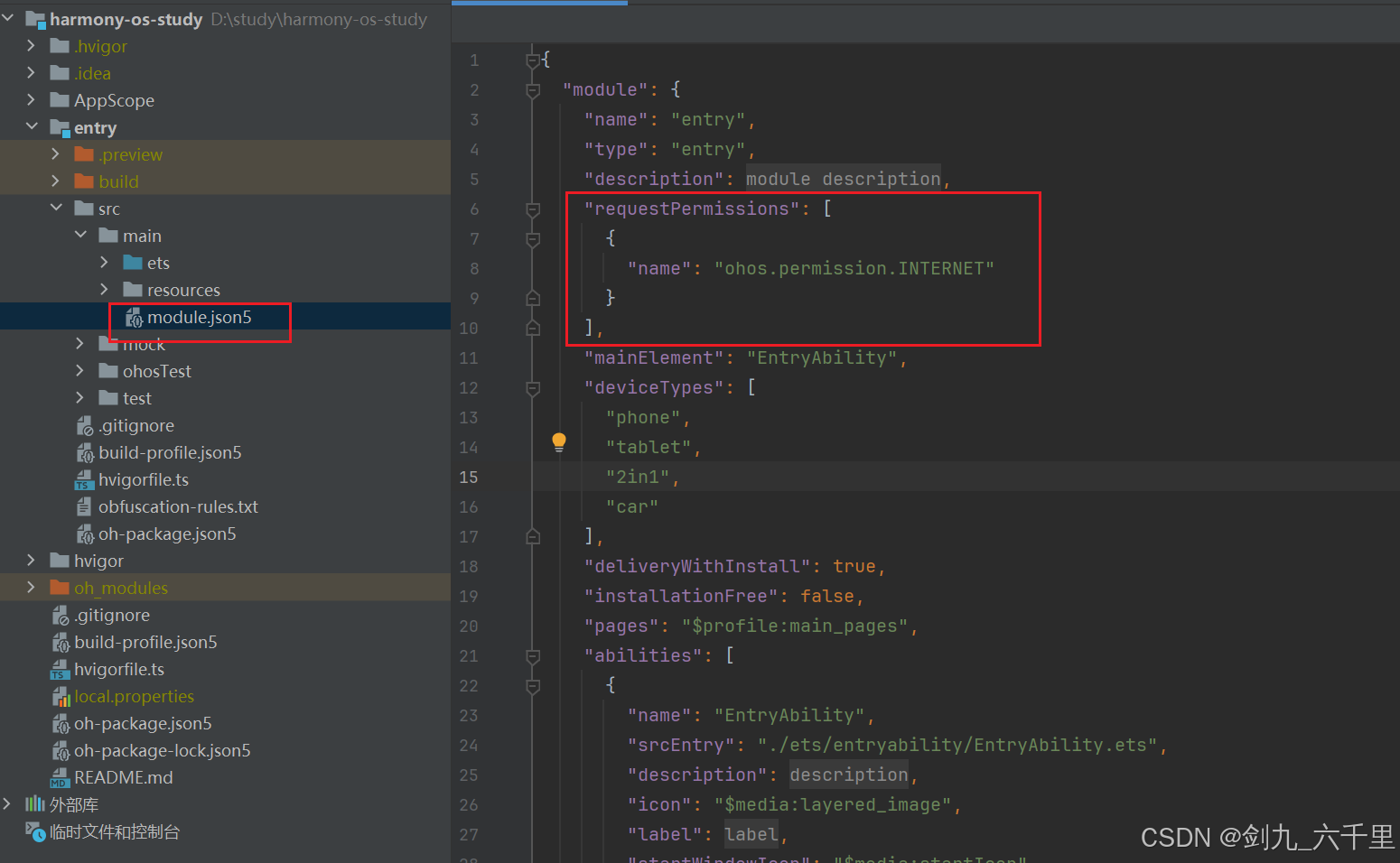
2.2. 导入 http 模块
ts
import http from '@ohos.net.http'
2.3. 页面中使用
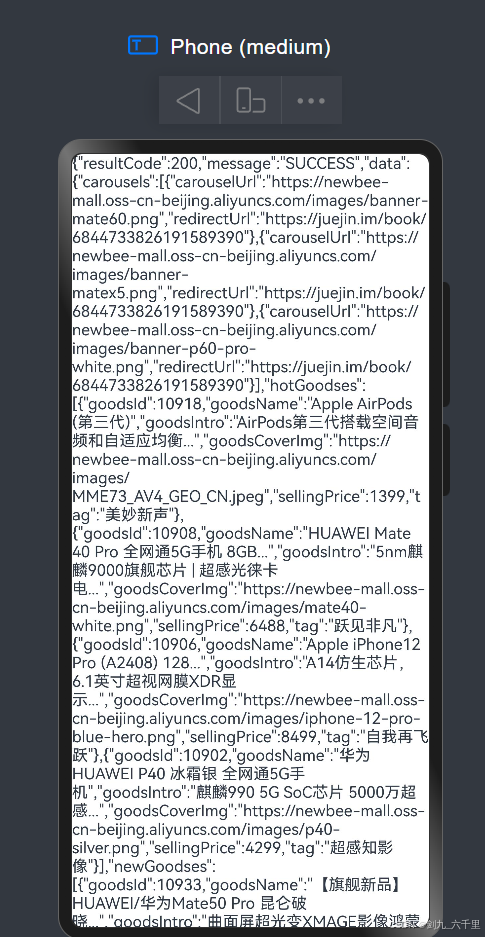
ts
import http from '@ohos.net.http'
@Entry
@Component
struct Index {
@State data: http.HttpResponse = {
result: {},
resultType: http.HttpDataType.OBJECT,
responseCode: 200,
header: "",
cookies: '',
performanceTiming: {
dnsTiming: 0,
tcpTiming: 0,
tlsTiming: 0,
firstSendTiming: 0,
firstReceiveTiming: 0,
totalFinishTiming: 0,
redirectTiming: 0,
responseHeaderTiming: 0,
responseBodyTiming: 0,
totalTiming: 0
},
};
async getData() {
const httpRequest = http.createHttp();
let res = await httpRequest.request(
// 请求地址
"http://backend-api-01.newbee.ltd/api/v1/index-infos",
{
// 请求头
header: {
"Content-Type": "application/json"
},
// 请求方法,默认 http.RequestMethod.GET
method: http.RequestMethod.GET,
// 指定返回数据的类型
expectDataType:http.HttpDataType.OBJECT
}
)
console.log(`${JSON.stringify(res.result)}`, 'res');
this.data = res;
}
build() {
Column() {
Text(`${JSON.stringify(this.data.result)}`)
Button("获取数据").onClick(() => {
this.getData();
})
}.width("100%")
.height("100%")
}
}