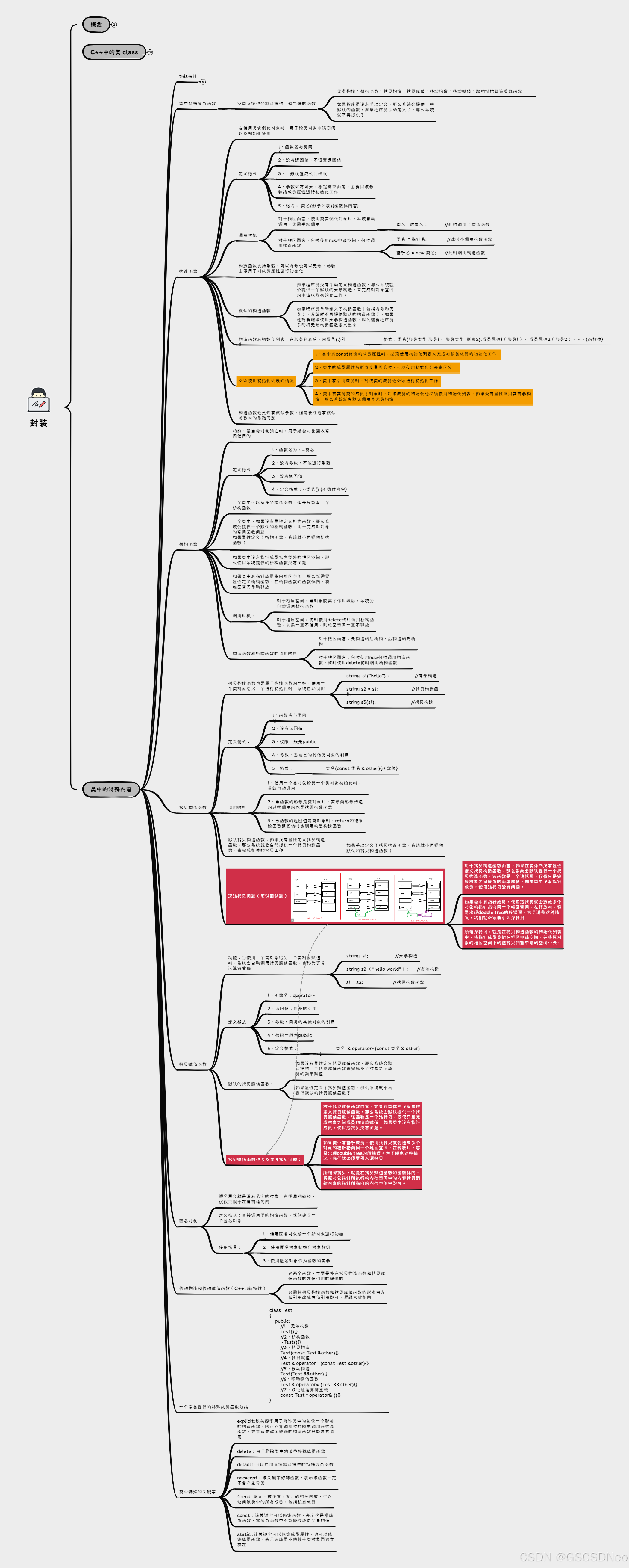
my_string.h
cpp
#ifndef MY_STRING_H
#define MY_STRING_H
#include <iostream>
#include <cstring>
using namespace std;
class My_string
{
private:
char *ptr; //指向字符数组的指针
int size; //字符串的最大容量
int len; //字符串当前容量
public:
//无参构造
My_string():size(20)
{
cout<<"****************无参构造***********"<<endl;
this->ptr = new char[size];
this->ptr[0] = '\0'; //表示串为空串
this->len = 0;
}
//有参构造
My_string(const char* src){
cout<<"****************一个参数有参构造***********"<<endl;
this->len = strlen(src);
this->size = len + 1;
this->ptr = new char[size];
strcpy(this->ptr,src);
}
My_string(int num,char value){
cout<<"****************两个参数有参构造***********"<<endl;
this->len = num;
this->size = len + 1;
this->ptr = new char[len+1];
int i=0;
while(i<num){
this->ptr[i]=value;
i++;
}
this->ptr[num]='\0';
}
//拷贝构造
My_string (const My_string &other){
cout<<"****************拷贝构造***********"<<endl;
len = other.len;
size = other.size;
ptr = new char[size];
strcpy(ptr, other.ptr);
}
//拷贝赋值
My_string &operator=(const My_string &other){
cout<<"****************拷贝赋值***********"<<endl;
if (this == &other) {
return *this; // 直接返回当前对象
}
delete[] ptr;
this->len = other.len;
this->size = other.size;
this->ptr = new char[size];
strcpy(ptr, other.ptr);
return *this;
}
//析构函数
~My_string(){
cout<<"****************析构函数***********"<<endl;
delete []this->ptr;
}
//显示内容
void show();
//判空
void isempty();
//判满
void isfull();
//尾插
void push_back(char value);
//尾删
void pop_back();
//at函数实现
char &at(int index);
//清空函数
void clear();
//返回C风格字符串
char* data();
//返回实际长度
int get_length();
//返回当前最大容量
int get_size();
//君子函数:二倍扩容
void resize();
};
#endif // MY_STRING_H
my_string.cpp
cpp
#include "my_string.h"
#include <cstring>
void My_string::show(){
cout<<ptr<<endl;
}
//判空
void My_string::isempty(){
if(this->len==0){
cout<<"字符串为空"<<endl;
}
return;
}
//判满
void My_string::isfull(){
if(this->len>=this->size){
cout<<"字符串满"<<endl;
resize();
cout<<"重新分配空间"<<endl;
}else{
cout<<"字符串未满"<<endl;
}
return;
}
//尾插
void My_string::push_back(char value){
this->isfull();
this->ptr[len]=value;
len++;
ptr[len]='\0';
}
//尾删
void My_string::pop_back(){
this->len=this->len-1;
ptr[len]='\0';
}
//at函数实现
char & My_string::at(int index){
return ptr[index];
}
//清空函数
void My_string::clear(){
ptr[0]='\0';
this->len=0;
}
//返回C风格字符串
char* My_string::data(){
return this->ptr; // 返回指向字符串的指针
}
//返回实际长度
int My_string::get_length(){
return this->len;
}
//返回当前最大容量
int My_string::get_size(){
return this->size;
}
//君子函数:二倍扩容
void My_string::resize() {
size *= 2;
char* new_ptr = new char[size];
strcpy(new_ptr, ptr);
delete[] ptr;
ptr = new_ptr;
}
main.cpp
cpp
#include "my_string.h"
int main(){
My_string p1;
My_string p2("hello");
p2.show();
My_string p3(5,'A');
p3.show();
My_string p4 = p2;
p4.show();
p3=p2;
p3.show();
p2.show();
cout<<"p2的第3个字符"<<p2.at(3)<<endl;
cout<<"p2C风格的字符串"<<p2.data()<<endl;
p2.isfull();
cout<<"p2的最大长度:"<<p2.get_size()<<endl;
cout<<"p2现在的长度:"<<p2.get_length()<<endl;
p2.clear();
p2.show();
p2.push_back('W');
p2.show();
p2.pop_back();
p2.show();
}