简介
- HttpClient
遵循http协议
的客户端编程工具包
- 支持
最新
的http协议
部分依赖自动传递依赖了HttpClient的jar包
- 明明项目中没有引入 HttpClient 的Maven坐标,但是却可以直接使用HttpClient
- 原因是:阿里云的sdk依赖中传递依赖了HttpClient的jar包

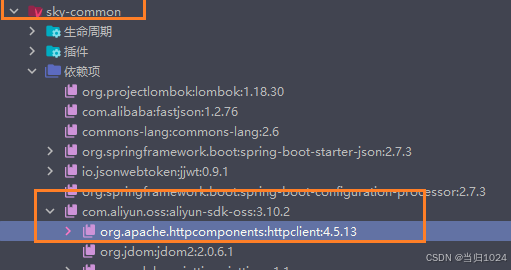
发送get请求
java
@Test
public void testGet() {
// 创建HttpGet对象
HttpGet httpGet = new HttpGet("http://localhost:8080/user/shop/status");
// 创建HttpClient对象 用于发送请求
// try-with-resources 语法 需要关闭的资源分别是 httpClient response
try (CloseableHttpClient httpClient = HttpClients.createDefault();
CloseableHttpResponse response = httpClient.execute(httpGet)) {
// 获取响应状态码
int statusCode = response.getStatusLine().getStatusCode();
System.out.println("响应状态码:" + statusCode); //响应状态码:200
// 获取响应数据
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity);
System.out.println("响应数据:" + result); // 响应数据:{"code":1,"msg":null,"data":1}
} catch (IOException e) {
log.error("请求失败", e);
e.printStackTrace();
}
}
发送post请求
java
/**
* 测试HttpClient 发送post请求 需要提前启动项目 不然请求不到
*/
@Test
public void testPost() {
// 创建HttpPost对象
HttpPost httpPost = new HttpPost("http://localhost:8080/admin/employee/login");
// 这个请求是有请求体的
// 使用JsonObject构建请求体 更加高效简洁
JsonObject jsonObject = new JsonObject();
jsonObject.addProperty("username", "admin");
jsonObject.addProperty("password", "123456");
// 将json对象转为字符串 并设置编码格式 设置传输的数据格式 使用构造器和set方法都是可以设置的
StringEntity stringEntity = null;
try {
stringEntity = new StringEntity(jsonObject.toString());
stringEntity.setContentEncoding("UTF-8");
stringEntity.setContentType("application/json");
} catch (UnsupportedEncodingException e) {
throw new RuntimeException(e);
}
// 设置请求体
httpPost.setEntity(stringEntity);
// 创建HttpClient对象 用于发送请求
// try-with-resources 语法 需要关闭的资源分别是 httpClient response
try (CloseableHttpClient httpClient = HttpClients.createDefault();
CloseableHttpResponse response = httpClient.execute(httpPost)) {
// 获取响应状态码
int statusCode = response.getStatusLine().getStatusCode();
System.out.println("响应状态码:" + statusCode); //响应状态码:200
// 获取响应数据
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity);
System.out.println("响应数据:" + result); // 响应数据:{"code":1,"msg":null,"data":{"id":1,"userName":"admin","name":"管理员","token":"eyJhbGciOiJIUzI1NiJ9.eyJlbXBJZCI6MSwiZXhwIjoxNzI4MzgwOTk5fQ.Rm7UWZbDEU_06DJLfegcP31n-9g8AB-Jxa-49Zw-ttM"}}
} catch (IOException e) {
log.error("请求失败", e);
e.printStackTrace();
}
}
工具类
分装了一个工具类
- 发送get请求
- 使用form表单发送post请求
- 使用json对象发送post请求
java
package com.sky.utils;
import com.alibaba.fastjson.JSONObject;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.NameValuePair;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* Http工具类
*/
@Slf4j
public class HttpClientUtil {
static final int TIMEOUT_MSEC = 5 * 1000;
public static final String UTF_8 = "utf-8";
public static final String DEFAULT_CONTENT_TYPE = "application/json";
public static final String LOG_ERR_TEMPLATE = "{}路径请求出错,错误详情如下";
/**
* 发送GET请求,返回字符串
*/
public static String doGet(String url, Map<String, String> paramMap) throws URISyntaxException, IOException {
String result = "";
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
URIBuilder builder = new URIBuilder(url);
if (paramMap != null) {
for (String key : paramMap.keySet()) {
builder.addParameter(key, paramMap.get(key));
}
}
URI uri = builder.build();
// 创建GET请求
HttpGet httpGet = new HttpGet(uri);
// 发送请求
try (CloseableHttpResponse response = httpClient.execute(httpGet)) {
// 判断响应状态
if (response.getStatusLine().getStatusCode() == 200) {
result = EntityUtils.toString(response.getEntity(), UTF_8);
}
}
} catch (Exception e) {
// 日志记录
logErr(url);
throw e;
}
return result;
}
/**
* 发送GET请求,返回JSONObject
*/
public static JSONObject doGetJson(String url, Map<String, String> paramMap) throws URISyntaxException, IOException {
JSONObject result = null;
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
URIBuilder builder = new URIBuilder(url);
if (paramMap != null) {
for (String key : paramMap.keySet()) {
builder.addParameter(key, paramMap.get(key));
}
}
URI uri = builder.build();
// 创建GET请求
HttpGet httpGet = new HttpGet(uri);
// 发送请求
try (CloseableHttpResponse response = httpClient.execute(httpGet)) {
// 判断响应状态
if (response.getStatusLine().getStatusCode() == 200) {
String resultString = EntityUtils.toString(response.getEntity(), UTF_8);
result = JSONObject.parseObject(resultString);
}
}
} catch (Exception e) {
logErr(url);
throw e;
}
return result;
}
/**
* 发送POST请求,返回字符串 表单请求
*/
public static String doPost(String url, Map<String, String> paramMap) throws IOException {
String resultString = "";
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
// 创建Http Post请求
HttpPost httpPost = new HttpPost(url);
// 创建参数列表
if (paramMap != null) {
List<NameValuePair> paramList = new ArrayList<>();
for (Map.Entry<String, String> param : paramMap.entrySet()) {
paramList.add(new BasicNameValuePair(param.getKey(), param.getValue()));
}
// 模拟表单
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(paramList);
httpPost.setEntity(entity);
}
httpPost.setConfig(builderRequestConfig());
// 执行http请求
try (CloseableHttpResponse response = httpClient.execute(httpPost)) {
resultString = EntityUtils.toString(response.getEntity(), UTF_8);
}
} catch (Exception e) {
logErr(url);
throw e;
}
return resultString;
}
/**
* 发送POST请求,返回JSONObject 表单请求
*/
public static JSONObject doPostJson(String url, Map<String, String> paramMap) throws IOException {
JSONObject result = null;
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
// 创建Http Post请求
HttpPost httpPost = new HttpPost(url);
// 创建参数列表
if (paramMap != null) {
List<NameValuePair> paramList = new ArrayList<>();
for (Map.Entry<String, String> param : paramMap.entrySet()) {
paramList.add(new BasicNameValuePair(param.getKey(), param.getValue()));
}
// 模拟表单
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(paramList);
httpPost.setEntity(entity);
}
httpPost.setConfig(builderRequestConfig());
// 执行http请求
try (CloseableHttpResponse response = httpClient.execute(httpPost)) {
String resultString = EntityUtils.toString(response.getEntity(), UTF_8);
result = JSONObject.parseObject(resultString);
}
} catch (Exception e) {
logErr(url);
throw e;
}
return result;
}
/**
* 发送POST请求,JSON格式数据,返回字符串 json请求
*/
public static String doPost4Json(String url, Map<String, String> paramMap) throws IOException {
String resultString = "";
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
HttpPost httpPost = new HttpPost(url);
if (paramMap != null) {
// 构造json格式数据
JSONObject jsonObject = new JSONObject();
for (Map.Entry<String, String> param : paramMap.entrySet()) {
jsonObject.put(param.getKey(), param.getValue());
}
StringEntity entity = new StringEntity(jsonObject.toString(), UTF_8);
// 设置请求编码
entity.setContentEncoding(UTF_8);
// 设置数据类型
entity.setContentType(DEFAULT_CONTENT_TYPE);
httpPost.setEntity(entity);
}
httpPost.setConfig(builderRequestConfig());
// 执行http请求
try (CloseableHttpResponse response = httpClient.execute(httpPost)) {
resultString = EntityUtils.toString(response.getEntity(), UTF_8);
}
} catch (Exception e) {
logErr(url);
throw e;
}
return resultString;
}
/**
* 发送POST请求,JSON格式数据,返回JSONObject json请求
*/
public static JSONObject doPost4JsonReturnJson(String url, Map<String, String> paramMap) throws IOException {
JSONObject result = null;
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
HttpPost httpPost = new HttpPost(url);
if (paramMap != null) {
// 构造json格式数据
JSONObject jsonObject = new JSONObject();
for (Map.Entry<String, String> param : paramMap.entrySet()) {
jsonObject.put(param.getKey(), param.getValue());
}
StringEntity entity = new StringEntity(jsonObject.toString(), UTF_8);
// 设置请求编码
entity.setContentEncoding(UTF_8);
// 设置数据类型
entity.setContentType(DEFAULT_CONTENT_TYPE);
httpPost.setEntity(entity);
}
httpPost.setConfig(builderRequestConfig());
// 执行http请求
try (CloseableHttpResponse response = httpClient.execute(httpPost)) {
String resultString = EntityUtils.toString(response.getEntity(), UTF_8);
result = JSONObject.parseObject(resultString);
}
} catch (Exception e) {
logErr(url);
throw e;
}
return result;
}
private static RequestConfig builderRequestConfig() {
return RequestConfig.custom()
.setConnectTimeout(TIMEOUT_MSEC)
.setConnectionRequestTimeout(TIMEOUT_MSEC)
.setSocketTimeout(TIMEOUT_MSEC).build();
}
/**
* 日志报错
* @param url 出错的URL
*/
private static void logErr(String url) {
log.error(LOG_ERR_TEMPLATE, url);
}
}