服务器
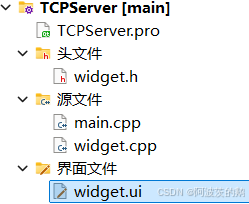
首先要在.pro文件中添加network,否则将不能使用QTcpserver
cpp
QT += core gui network
cpp
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include <QTcpServer>
#include <QTcpSocket>
#define PORT 8000
QT_BEGIN_NAMESPACE
namespace Ui {
class Widget;
}
QT_END_NAMESPACE
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
private slots:
void newClientHandler();
void clientInfoSlot();
void on_sendButton_clicked();
private:
Ui::Widget *ui;
QTcpServer *server;
QTcpSocket *socket;
};
#endif // WIDGET_H
cpp
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
server = new QTcpServer;
server->listen(QHostAddress::AnyIPv4,PORT);
connect(server,&QTcpServer::newConnection,this,&Widget::newClientHandler);
}
Widget::~Widget()
{
delete ui;
}
void Widget::newClientHandler()
{
//建立TCP连接
socket = server->nextPendingConnection();
ui->addresslineEdit->setText(socket->peerAddress().toString());
ui->portlineEdit->setText(QString::number(socket->peerPort()));
connect(socket,&QTcpSocket::readyRead,this,&Widget::clientInfoSlot);
}
void Widget::clientInfoSlot()
{
//获取信号的发出者
QTcpSocket *socket=(QTcpSocket *)sender();
ui->revlineEdit->setText(QString(socket->readAll()));
}
void Widget::on_sendButton_clicked()
{
QByteArray ba;
ba.append(ui->sendlineEdit->text().toLatin1());
socket->write(ba);
}

客户端
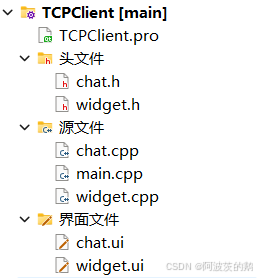
同样的,别忘了在.pro文件中修改
cpp
QT += core gui network
cpp
#ifndef CHAT_H
#define CHAT_H
#include <QWidget>
#include<QTcpSocket>
namespace Ui {
class chat;
}
class chat : public QWidget
{
Q_OBJECT
public:
explicit chat(QTcpSocket *socket,QWidget *parent = nullptr);
~chat();
private slots:
void on_sendButton_clicked();
void on_clearButton_clicked();
void clientReadHandler();
private:
Ui::chat *ui;
QTcpSocket *socket;
};
#endif // CHAT_H
cpp
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include<QTcpsocket>
#include<QHostAddress>
#include<QMessageBox>
#include "chat.h"
QT_BEGIN_NAMESPACE
namespace Ui {
class Widget;
}
QT_END_NAMESPACE
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
private slots:
void on_cancelButton_clicked();
void on_connectButton_clicked();
private:
Ui::Widget *ui;
QTcpSocket *socket;
chat *ch;
};
#endif // WIDGET_H
cpp
#include "chat.h"
#include "ui_chat.h"
chat::chat(QTcpSocket *s,QWidget *parent) :
QWidget(parent),
ui(new Ui::chat)
{
ui->setupUi(this);
socket = s;
connect(socket,&QTcpSocket::readyRead,this,&chat::clientReadHandler);
}
chat::~chat()
{
delete ui;
}
void chat::on_sendButton_clicked()
{
QByteArray ba;
ba.append(ui->lineEdit->text().toLatin1());
socket->write(ba);
}
void chat::on_clearButton_clicked()
{
ui->lineEdit->clear();
}
void chat::clientReadHandler()
{
ui->revlineEdit->setText(QString(socket->readAll()));
}
cpp
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
socket = new QTcpSocket;
}
Widget::~Widget()
{
delete ui;
}
void Widget::on_connectButton_clicked()
{
QString ip = ui->iplineEdit->text();
QString port = ui->portlineEdit->text();
qDebug() << ip;
qDebug() << port;
socket->connectToHost(QHostAddress(ip),port.toShort());
connect(socket,&QTcpSocket::connected,[this]{
QMessageBox::information(this,"Tip","连接成功");
this->hide();
ch = new chat(socket);
ch->show();
});
connect(socket,&QTcpSocket::disconnected,[this]{
QMessageBox::information(this,"Tip","连接失败!");
});
}
void Widget::on_cancelButton_clicked()
{
this->close();
}