需要用到并查集的相关知识:可以参考如下链接
cpp
#include<iostream>
#include<algorithm>
#include<vector>
using namespace std;
vector<int> split(string params_str) {
vector<int> p;
while (params_str.find(" ") != string::npos) {
int found = params_str.find(" ");
p.push_back(stoi(params_str.substr(0, found)));
params_str = params_str.substr(found + 1);
}
p.push_back(stoi(params_str));
return p;
}
vector<string> split_str(string params_str) {
vector<string> p;
while (params_str.find(" ") != string::npos) {
int found = params_str.find(" ");
p.push_back(params_str.substr(0, found));
params_str = params_str.substr(found + 1);
}
p.push_back(params_str);
return p;
}
// 并查集实现
class UnionFind{
vector<int> root;
vector<int> rank;
int cnt;
public:
// 初始化数据结构
UnionFind(int N) : cnt(0){
root.resize(N+1);
rank.reserve(N+1);
for (int i = 0; i < N+1; ++i) {
root[i] = i;
rank[i] = 1;
}
}
int find(int x) {
if (x == root[x]) {
return x;
}
return root[x] = find(root[x]);
}
void union_op(int x, int y) {
root[find(x)] = find(y);
cnt+=1;
}
int get_count(){
return cnt;
}
};
int main()
{
int n,m;
cin >> n >> m;
UnionFind uf(n);
vector<vector<int>> networks;
for (int i = 0; i < m; i++) {
int a,b,c,d;
cin >> a >> b >> c >> d;
if (d == 1) {
if (uf.find(a) != uf.find(b)) {
uf.union_op(a, b);
}
} else {
networks.push_back(vector<int>{a,b,c});
}
}
sort(networks.begin(), networks.end(),[](vector<int> a ,vector<int> b){
return a[2]<b[2];
});
int result = 0;
int i=0;
while(true){
if(i>=networks.size()){
break;
} else {
if (uf.find(networks[i][0]) != uf.find(networks[i][1])) {
uf.union_op(networks[i][0], networks[i][1]);
result += networks[i][2];
if (uf.get_count() == n - 1) {
break;
}
}
}
i+=1;
}
if(uf.get_count() != n - 1){
result = -1;
}
cout<<result<<endl;
return 0;
}
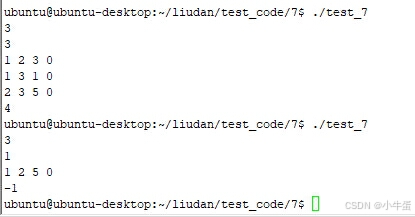